TypeScript's Interfaces and Classes for Type Safety
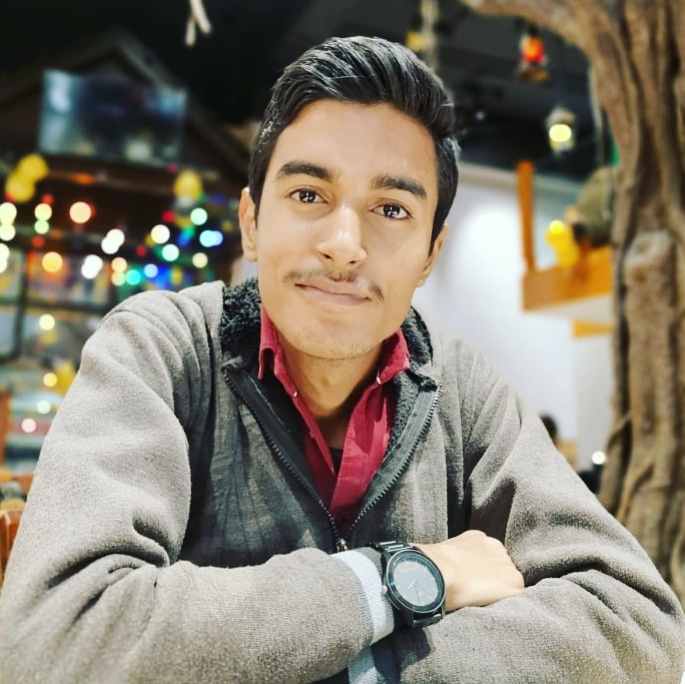
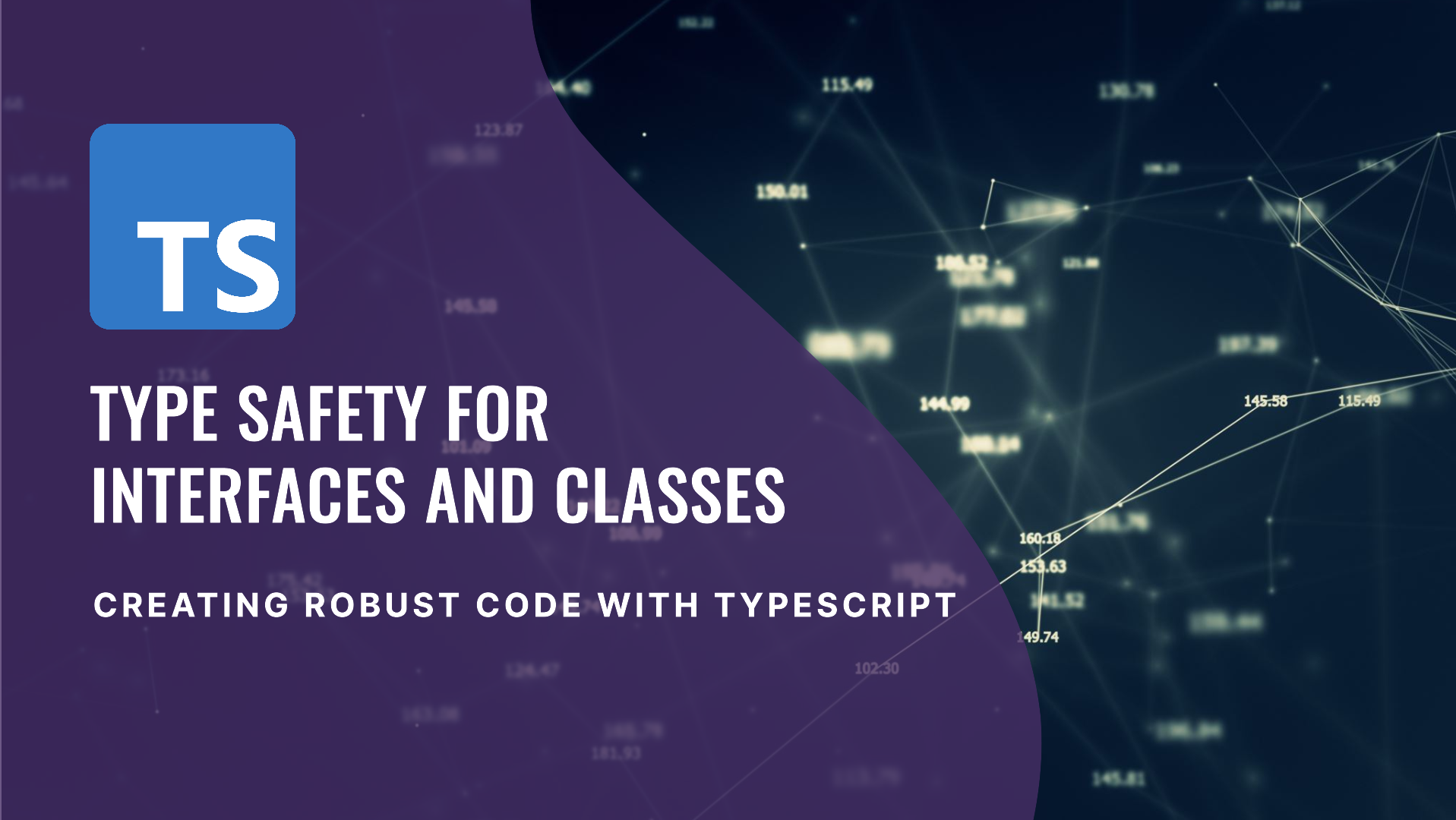
TypeScript, a superset of JavaScript, has gained immense popularity due to its ability to enhance code quality and maintainability through static type checking. Interfaces and classes play a crucial role in achieving this type safety. Let's delve into how these two language constructs work together to create robust and reliable TypeScript applications.
Understanding Interfaces
Interfaces in TypeScript are blueprints for objects. They define the structure and properties that an object must have, without providing implementations. They act as contracts, ensuring that objects adhere to a specific shape, making code more predictable and easier to reason about.
Example:
interface Person {
name: string;
age: number;
greet(): void;
}
Understanding Classes
Classes are templates for creating objects. They encapsulate data (properties) and behavior (methods) into a single unit. Classes can implement interfaces, ensuring that they conform to the specified contract.
Example:
class Employee implements Person {
name: string;
age: number;
department: string;
constructor(name: string, age: number, department: string) {
this.name = name;
this.age = age;
this.department 1. github.com github.com = department;
}
greet() {
console.log(`Hello, my name is ${this.name}`);
}
}
Benefits of Interfaces and Classes for Type Safety
Early Error Detection: TypeScript's type checker can identify potential errors at compile time, preventing runtime exceptions and improving code reliability.
Improved Code Readability: Interfaces and classes provide a clear structure, making code easier to understand and maintain.
Enhanced Code Maintainability: When code is well-typed, it's easier to make changes without introducing unintended side effects.
Better Collaboration: In team projects, interfaces and classes can help establish shared conventions and ensure that team members are working with consistent data structures.
Leveraging IDE Features: TypeScript-aware IDEs can provide intelligent code completion, type hints, and refactoring support, significantly boosting developer productivity.
Practical Use Cases
API Contracts: Interfaces can define the expected structure of data exchanged between components or with external services.
Data Validation: Classes can enforce data integrity by ensuring that properties adhere to specific types and constraints.
Component Libraries: Interfaces can define the public API of reusable components, making them easier to use and integrate.
Dependency Injection: Interfaces can abstract away dependencies, making code more modular and testable.
Conclusion
Interfaces and classes are powerful tools in TypeScript's arsenal for achieving type safety. By defining contracts and enforcing structure, they contribute to more reliable, maintainable, and collaborative codebases. By leveraging these language features effectively, you can write TypeScript applications that are not only robust but also a joy to work with.
Subscribe to my newsletter
Read articles from Abhishek Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
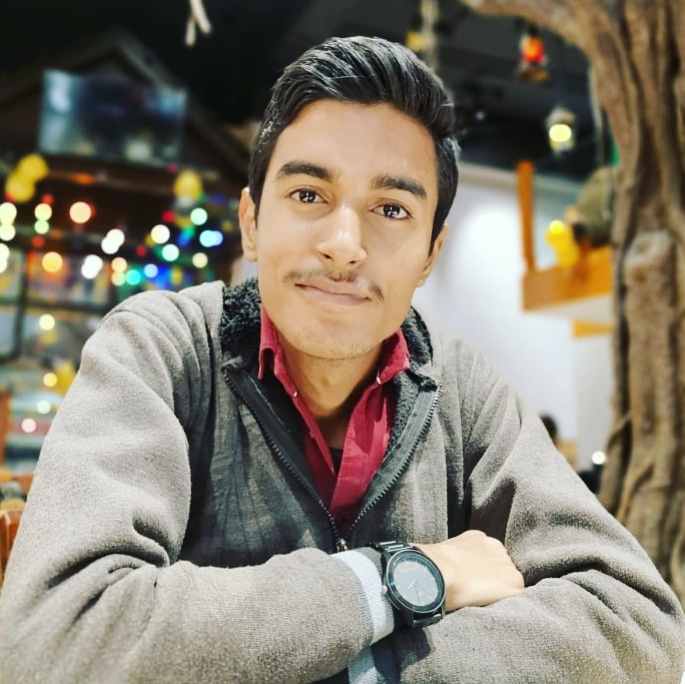
Abhishek Sharma
Abhishek Sharma
Abhishek is a designer, developer, and gaming enthusiast! He love creating things, whether it's building websites, designing interfaces, or conquering virtual worlds. With a passion for technology and its impact on the future, He is curious about how AI can be used to make work better and play even more fun.