Building with Node.js and Express.js: Everything You Need to Know.
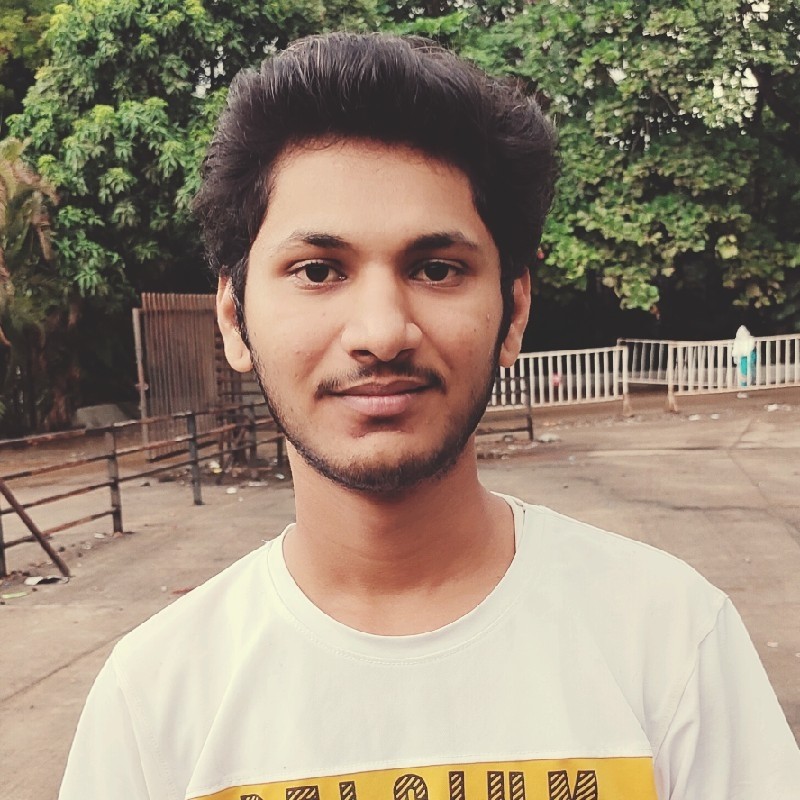
Table of contents
- #Node.js
- Key Features of Node.js:
- How Node.js Works:
- Use Cases:
- Pros of Node.js:
- Cons of Node.js:
- #Express.js
- Key Features of Express.js:
- How Express.js Works:
- Use Cases:
- Pros of Express.js:
- Cons of Express.js:
- #Working With Nodejs And Expressjs
- Step 1: Install Node.js and npm
- Step 2: Initialize a Node.js Project
- Step 3: Install Express.js
- Step 4: Create the Server Code
- Step 5: Start the Server
- Step 6: Using nodemon for Auto-Restart (Optional)
- Step 7: Add More Routes (Optional)
- #Understanding Promises
- Key Concepts of Promises:
- Why Use Promises?
- Example of Using Promises:
- #What is Async/Await
- Key Concepts of async/await:
- Example of async/await:
- Why Use async/await?
- Practical Example:
- #InAddition
- #Some Packages and Their Descriptions
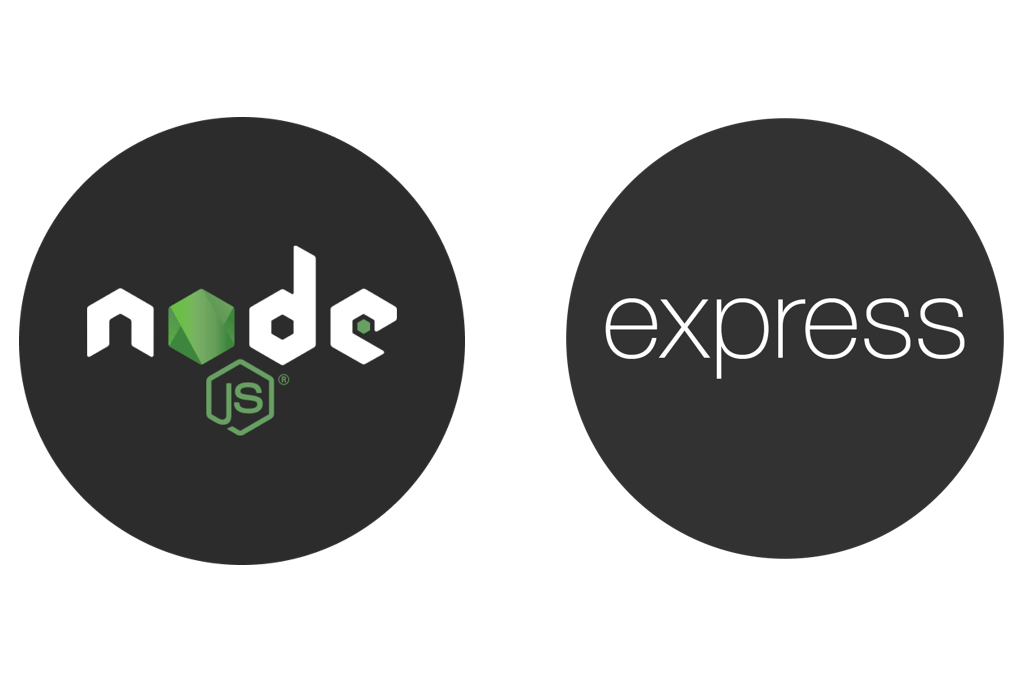
#This Blog Include Information of Environment Nodejs and Framework Express.This Blog is Useful To Understand The Concept and to Learn About Node and Express.Reading This blog Will Help TO Gain Knowledge Help to Enhance Your Skill For Web Development.
#Node.js
Node.js is a powerful, open-source, cross-platform runtime environment that allows developers to execute JavaScript code outside of a web browser. It’s built on Chrome's V8 JavaScript engine and is primarily used to build server-side and network applications.
Key Features of Node.js:
Asynchronous and Event-Driven:
- Node.js uses an event-driven, non-blocking I/O model, which makes it lightweight and efficient. Instead of waiting for operations like file reading or database querying to complete, Node.js continues executing other tasks. This allows it to handle thousands of simultaneous connections without the need for a large number of threads.
Single-Threaded but Scalable:
- Even though Node.js is single-threaded, it’s designed for scalability. It handles multiple requests by using an event loop mechanism rather than creating a new thread for each request, which is common in other server-side languages like PHP or Java. This makes it memory-efficient and capable of handling a high volume of requests with fewer resources.
Fast Execution:
- Node.js is built on the V8 engine, which compiles JavaScript into machine code before execution, leading to faster execution times. The performance is further enhanced by its non-blocking I/O operations.
NPM (Node Package Manager):
- Node.js comes with a built-in package manager called NPM, which is the largest ecosystem of open-source libraries. NPM allows developers to easily install, share, and manage dependencies within their projects, making development faster and more efficient.
Cross-Platform:
- Node.js applications can run on various platforms, including Windows, macOS, and Linux. This makes it versatile and suitable for a wide range of use cases.
How Node.js Works:
Event Loop: Node.js uses an event loop that continuously checks the event queue and executes callback functions. The event loop allows Node.js to perform non-blocking I/O operations by offloading tasks like file system access, network calls, or database queries to the system's kernel. Once the operation completes, a callback function is pushed onto the event queue, and the event loop picks it up for execution.
Callbacks and Promises: In Node.js, callbacks and promises are often used to handle asynchronous code execution. Callbacks are functions passed into other functions to be called once an asynchronous operation completes, while promises represent the eventual result of an asynchronous operation.
Use Cases:
Web Servers: Node.js is ideal for building fast and scalable web servers. It’s commonly used with Express.js, a popular web framework that simplifies building web applications and APIs.
Real-Time Applications: Due to its event-driven architecture, Node.js is well-suited for real-time applications such as chat applications, gaming servers, or collaborative tools where multiple users interact in real-time.
Microservices: Node.js is often used to create microservices, which are small, independently deployable services that together make up a larger application. Its lightweight nature and fast execution make it ideal for this architecture.
APIs: Node.js is commonly used to build RESTful APIs and GraphQL APIs, which handle large amounts of traffic and data.
Command-Line Tools: Node.js can be used to build command-line tools that automate tasks, manipulate files, or interact with other services.
Pros of Node.js:
High Performance: Its non-blocking I/O model allows for handling a large number of connections simultaneously, making it highly performant.
Scalability: Node.js is well-suited for building scalable applications, particularly real-time applications that require heavy I/O operations.
Large Ecosystem: With NPM, Node.js offers a vast library of modules and packages, speeding up development.
JavaScript Everywhere: With Node.js, developers can use JavaScript both on the client side and the server side, which unifies the development environment and reduces the need for learning multiple languages.
Cons of Node.js:
Single-Threaded Limitations: Although it’s optimized for handling I/O operations, Node.js can struggle with CPU-bound tasks like complex computations, which could block the single thread and degrade performance.
Callback Hell: Handling asynchronous code with multiple nested callbacks can make the code harder to read and maintain. However, this issue can be mitigated using promises and async/await.
Maturity of Libraries: Not all NPM packages are of high quality or well-maintained, and developers may need to carefully select libraries to avoid security or stability issues.
#Express.js
Express.js is a minimal and flexible Node.js web application framework that provides a robust set of features to build web and mobile applications. It simplifies the development process by offering a layer of abstraction over Node.js, enabling developers to create applications and APIs more efficiently.
Key Features of Express.js:
Minimalist Framework:
- Express.js is lightweight, offering just the essential tools and features needed for building web applications. This minimalism gives developers flexibility to structure their applications as they see fit, without imposing strict conventions.
Middleware:
- Middleware functions are at the core of Express.js. They are functions that have access to the request and response objects and can modify or handle them. Middleware can be used to handle different aspects of an HTTP request, such as parsing data, handling authentication, logging, and more.
Routing:
- Express.js provides robust routing capabilities that allow developers to define routes for different HTTP methods and URLs. It supports parameters, query strings, and even regular expressions, making it easy to create RESTful APIs and handle complex routing logic.
Template Engines:
- Express.js supports various template engines like Pug (formerly Jade), EJS, and Handlebars, allowing developers to generate dynamic HTML content on the server side. These engines help render views and deliver them to the client.
Error Handling:
- Express.js comes with built-in error handling capabilities. Developers can define custom error-handling middleware to manage errors in their applications, ensuring graceful degradation and proper response to clients in case of issues.
Integration with Node.js:
- Since Express.js is built on top of Node.js, it inherits all of Node.js's features and capabilities. Developers can seamlessly integrate Express.js with Node.js modules, libraries, and other technologies, like databases, to create full-stack applications.
Scalability:
- Express.js allows developers to create scalable applications by making it easy to break down an application into smaller components, routes, and middleware. It also works well in a microservices architecture.
Asynchronous Programming:
- Like Node.js, Express.js leverages asynchronous, non-blocking code execution, making it well-suited for applications that require high concurrency and efficient I/O operations.
How Express.js Works:
Middleware Stack: In an Express.js application, middleware functions are executed in sequence as part of a stack. When a request is made to the server, it passes through this stack, where middleware can handle authentication, data parsing, logging, and more before reaching the route handler that generates the response.
Routing: Express.js allows developers to define routes for handling specific HTTP requests (GET, POST, PUT, DELETE, etc.). A route consists of a path (e.g.,
/users
) and a callback function that defines what happens when a request is made to that path.Request and Response Objects: Express.js provides
req
(request) andres
(response) objects that contain all the necessary information for handling an HTTP request and sending a response. Developers use these objects to read data from the client, interact with databases, and generate responses.
Use Cases:
RESTful APIs: Express.js is a popular choice for building RESTful APIs, allowing developers to define routes for different endpoints and handle HTTP requests like GET, POST, PUT, and DELETE.
Single Page Applications (SPAs): Express.js can serve the back end for SPAs built with front-end frameworks like React, Angular, or Vue.js. It handles routing, data fetching, and other server-side logic.
Websites: Express.js is commonly used to build server-rendered websites. With template engines, developers can generate dynamic HTML content that is delivered to the client.
Middleware-Oriented Applications: Express.js is ideal for applications that require multiple middleware layers, such as logging, authentication, validation, and data parsing. It simplifies building these middleware chains.
Microservices: Express.js is lightweight and modular, making it well-suited for creating microservices in a distributed architecture.
Pros of Express.js:
Simplicity: Express.js is easy to learn and use. Its minimalistic nature allows developers to build applications quickly with minimal boilerplate code.
Flexibility: Express.js doesn’t impose a strict application structure, giving developers the freedom to organize their code as they prefer.
Middleware: The use of middleware allows for modular development, where each part of an application can be handled separately, making it easy to maintain and scale.
Integration with Node.js: Express.js benefits from the asynchronous, event-driven nature of Node.js, allowing it to handle high concurrency with low resource usage.
Large Community: Express.js has a large community, so finding solutions, tutorials, and third-party middleware is relatively easy.
Cons of Express.js:
No Built-In Structure: While the flexibility of Express.js can be a strength, it can also be a drawback for larger projects. The lack of a predefined structure can lead to inconsistency across the codebase if not managed carefully.
Callback Hell: Like Node.js, Express.js can suffer from "callback hell" in asynchronous code. However, using promises and async/await can help mitigate this issue.
Manual Work for Common Features: Some features that are built-in in other frameworks (e.g., authentication, session management) may require additional setup or middleware in Express.js, which can increase development time.
#Working With Nodejs And Expressjs
Starting an npm server (typically using Express.js) involves several steps, from initializing a Node.js project to writing server code and running it. Here's a detailed guide on how to set up and start an npm server from scratch.
Step 1: Install Node.js and npm
- Ensure that you have Node.js and npm installed on your system. You can check this by running the following commands in your terminal:
node -v or npm -v
Step 2: Initialize a Node.js Project
Create a Project Directory: Open your terminal and navigate to the directory where you want to create your project. Create a new folder for your project:
mkdir my-npm-server
cd my-npm-server
Initialize the Project: Run the following command to initialize your Node.js project. This will create a
package.json
file that contains information about your project and its dependencies.npm init -y
The
-y
flag will generate thepackage.json
file with default settings.
Step 3: Install Express.js
Install Express.js: Express.js is a minimal web framework for Node.js. Install it by running:
npm install express --save
This will install Express.js and add it to your
package.json
as a dependency.
Step 4: Create the Server Code
Create an
index.js
File: In your project directory, create a new file calledindex.js
. This file will contain the code to start your Express server.Write the Server Code: Add the following code to
index.js
:// Import the Express module const express = require('express');
// Initialize the Express application const app = express();
// Define a port to listen on const port = 3000;
// Define a basic route app.get('/', (req, res) => { res.send('Hello, World!'); });
// Start the server app.listen(port, () => { console.log(Server is running on
http://localhost:${port}
); });
This code does the following:
-Imports the Express module.
-Initializes an Express application (
app
).-Defines a route that responds with "Hello, World!" when accessed via the root URL (
/
).-Starts the server and listens on port 3000.
Step 5: Start the Server
Run the Server: In the terminal, run the following command to start the server:
node index.js
This will start the server, and you'll see a message like:
Server is running on
http://localhost:3000
Access the Server: Open your web browser and go to
http://localhost:3000
. You should see the message "Hello, World!" displayed in your browser.
Step 6: Using nodemon
for Auto-Restart (Optional)
If you want the server to automatically restart when you make changes to the code, you can install nodemon
. This is particularly useful during development.
Install
nodemon
: Install it as a development dependency:npm install nodemon --save-dev
Modify the
package.json
File: Update thescripts
section in yourpackage.json
to include astart
script that usesnodemon
:"scripts": { "start": "nodemon index.js" }
Run the Server with
nodemon
: Now, start the server using:npm start
The server will automatically restart whenever you make changes to your code.
Step 7: Add More Routes (Optional)
You can add more routes to your Express server as needed. Here's an example of adding another route:
app.get('/about', (req, res) => { res.send('This is the About page!'); });
*#Terms To Understand
Anonymous Function | A function that is not named. An anonymous function is often passed into another function as a parameter. |
Application Server | Transforms data into dynamic content and runs the business logic, which is the data storage and transfer rules. |
Asynchronous | A process that runs independently of other processes. |
Callback Function | A function passed into another function as a parameter, which is then invoked inside the outer function to complete an action. Instead of blocking on asynchronous I/O operations, callback functions are used to handle results when the operations complete. |
Database Server | A server dedicated to providing database services. |
Dependencies | Code, usually in the form of libraries and packages, that are called from other modules and reused in a program. |
Event-Driven | Where the flow of a program is determined by particular events such as user input. |
Express.js | A highly configurable web framework for building applications on Node.js. |
Framework | Generates code that cannot be altered to perform common tasks. Examples include Django, Ruby on Rails, and Express.js. |
HTTP Server | A type of software-based server that understands URLs and hypertext transfer protocol. |
Load | Refers to the number of concurrent users, the number of transactions, and the amount of data transferred back and forth between the clients and servers. |
Module | Files containing related, encapsulated JavaScript code that serve a specific purpose. |
Multi-Threaded | Where multiple tasks are executed simultaneously. |
Node.js | A JavaScript runtime environment that runs on Google Chrome’s V8 engine. |
Non-Blocking | Failure of a given thread does not cause failure in another, and the execution of a task is not blocked until execution of another task is completed. |
Npm | Stands for node package manager. It is the default package manager for the Node.js runtime environment. |
Package | A directory with one or more modules bundled together. |
Package.json | Contains metadata information about the project, including dependencies and scripts. |
Payload | The data transmitted between client and server. |
Runtime Environment | Behaves similarly to a mini operating system that provides the resources necessary for an application to run. It is the infrastructure that supports the execution of a codebase. It is the hardware and software environment in which an application gets executed. Node.js is an example of a backend runtime environment. |
Scalability | The application’s ability to dynamically handle the load as is or shrinks without it affecting the application’s performance. |
Server.js | A file that contains the code that handles server creation. |
Single-Threaded | Where only one command is processed at a given point of time. |
Web Server | Ensures client requests are responded to, often using HTTP. |
Web Service | A type of web API that communicates using HTTP requests. It is the web service in the programming interface that sends and receives requests using HTTP among web servers and the client. |
Backend technologies include various types of servers and supporting infrastructures such as programming languages, frameworks, and other hardware.
Node.js is the server-side component of JavaScript.
The require statement can be called from anywhere in the app code, is bound dynamically, and is synchronous. The import statement must be called at the beginning of a file, is bound statically, and is not asynchronous.
Client-side JavaScript is used to process front-end user interface elements, and server-side JavaScript is used to enable access to different kinds of servers and web applications.
With server-side JavaScript, Node.js applications process and route web service requests from the client.
To make a function or a value available to Node.js applications that import your module, add a property to the implicit exports object.
Core modules include bare minimum functionality, local modules are those that you create for your application, and the Node.js community creates third-party modules.
A local install means only the application within the directory of the installed can access the package, whereas a global install means that any application on the machine can access the package.
#Understanding Promises
Promises are a fundamental concept in JavaScript for handling asynchronous operations. A promise represents the eventual completion (or failure) of an asynchronous operation and its resulting value.
Key Concepts of Promises:
States of a Promise: A promise can be in one of three states:
Pending: The initial state, neither fulfilled nor rejected.
Fulfilled: The operation was completed successfully.
Rejected: The operation failed.
Creating a Promise: A promise is created using the
Promise
constructor, which takes a function (executor function) with two parameters:resolve
andreject
. These are used to change the state of the promise:javascriptCopy codeconst myPromise = new Promise((resolve, reject) => { // Perform an asynchronous operation let success = true; // This is just an example condition if (success) { resolve('Operation successful!'); } else { reject('Operation failed!'); } });
Handling Promises: Promises can be handled using
.then()
for fulfilled cases and.catch()
for rejected cases:javascriptCopy codemyPromise .then(result => { console.log(result); // Logs: "Operation successful!" }) .catch(error => { console.error(error); // Logs: "Operation failed!" if rejected });
Chaining Promises: You can chain multiple
.then()
calls to handle a sequence of asynchronous operations. Each.then()
returns a new promise, which allows chaining:javascriptCopy codemyPromise .then(result => { console.log(result); return 'Next step'; }) .then(nextResult => { console.log(nextResult); // Logs: "Next step" }) .catch(error => { console.error(error); });
Promise.all() and Promise.race():
Promise.all()
: Executes multiple promises in parallel and resolves when all promises are fulfilled. If any promise is rejected, it rejects.javascriptCopy codePromise.all([promise1, promise2, promise3]) .then(results => { console.log(results); // Array of all fulfilled values }) .catch(error => { console.error(error); // Logs if any promise is rejected });
Promise.race()
: Resolves or rejects as soon as any one of the promises in the array settles (either fulfilled or rejected).javascriptCopy codePromise.race([promise1, promise2, promise3]) .then(result => { console.log(result); // Logs the result of the first settled promise }) .catch(error => { console.error(error); // Logs the first rejection });
Why Use Promises?
Better Readability: Promises provide a more structured way to handle asynchronous operations compared to traditional callbacks, reducing "callback hell" (nested callbacks).
Chaining: Promises allow for chaining of asynchronous operations in a sequential manner, which is easier to manage.
Error Handling: Promises provide a cleaner and more consistent way to handle errors across asynchronous operations.
Example of Using Promises:
Consider an example where we fetch data from an API using a promise:
javascriptCopy codefunction fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
const data = { id: 1, name: 'John Doe' };
if (data) {
resolve(data);
} else {
reject('Data not found');
}
}, 2000); // Simulating a network request with setTimeout
});
}
fetchData()
.then(data => {
console.log('Data fetched:', data);
})
.catch(error => {
console.error('Error:', error);
});
In this example, after 2 seconds, the promise is either resolved with the data or rejected if no data is found.
#What is Async/Await
async/await
is a feature in JavaScript that simplifies working with promises and asynchronous code. It allows developers to write asynchronous code that looks and behaves more like synchronous code, making it easier to read and reason about.
Key Concepts of async/await
:
async
Functions:The
async
keyword is used to define an asynchronous function. It automatically wraps the return value of the function in a promise.When you mark a function with
async
, it means that the function will always return a promise, regardless of whether you explicitly return a promise or some other value.
javascriptCopy codeasync function fetchData() {
return 'Hello, World!';
}
fetchData().then(result => console.log(result)); // Logs: "Hello, World!"
await
Keyword:The
await
keyword is used to pause the execution of anasync
function until a promise is settled (either fulfilled or rejected).await
can only be used insideasync
functions, and it makes the code wait for the promise to resolve and then returns the resolved value.
javascriptCopy codeasync function getData() {
let result = await fetchData(); // Waits until fetchData() resolves
console.log(result); // Logs: "Hello, World!"
}
Error Handling with
async/await
:- You can handle errors in
async/await
by usingtry...catch
blocks, which provide a structured way to catch and manage errors, similar to synchronous code.
- You can handle errors in
javascriptCopy codeasync function getData() {
try {
let result = await fetchData();
console.log(result);
} catch (error) {
console.error('Error:', error);
}
}
Example of async/await
:
Consider an example where you need to fetch data from an API. Without async/await
, you would use promises like this:
javascriptCopy codefunction fetchData() {
return new Promise((resolve) => {
setTimeout(() => {
resolve('Data fetched');
}, 2000);
});
}
fetchData()
.then(data => console.log(data))
.catch(error => console.error(error));
With async/await
, the same operation can be written in a more readable manner:
javascriptCopy codeasync function getData() {
try {
let data = await fetchData();
console.log(data); // Logs: "Data fetched" after 2 seconds
} catch (error) {
console.error(error);
}
}
getData();
In this version, the code appears synchronous (even though it's still asynchronous), which makes it easier to follow.
Why Use async/await
?
Simplifies Promises:
async/await
removes the need to chain.then()
and.catch()
calls, leading to code that is easier to read and maintain.Error Handling:
try...catch
blocks for error handling provide a more familiar and structured way to manage errors compared to.catch()
.Sequential Operations: With
await
, you can easily write code that performs asynchronous operations in sequence, as if they were synchronous:javascriptCopy codeasync function fetchMultipleData() { const data1 = await fetchData(); const data2 = await fetchMoreData(); console.log(data1, data2); }
Avoiding Callback Hell: Like promises,
async/await
helps avoid deeply nested callback structures, improving code readability.
Practical Example:
Consider an example where you need to fetch user data and their posts from an API:
javascriptCopy codeasync function fetchUserData(userId) {
const userResponse = await fetch(`https://api.example.com/users/${userId}`);
const userData = await userResponse.json();
const postsResponse = await fetch(`https://api.example.com/users/${userId}/posts`);
const postsData = await postsResponse.json();
return { userData, postsData };
}
fetchUserData(1)
.then(result => {
console.log('User:', result.userData);
console.log('Posts:', result.postsData);
})
.catch(error => console.error('Error fetching data:', error));
Here, both API calls are made sequentially with await
, and the results are processed together.
#InAddition
Middleware is software that sits between applications, databases, or services and allows those different technologies to communicate. It creates seamless interactions for the end user in a distributed system.
Express is a messaging framework used to handle routes and write middleware. The front end of an application uses Express to facilitate communication between components on the back end without the front-end and back-end services needing to use the same language. The front end communicates with the middleware, not directly with the back end.
Messaging frameworks like Express commonly contain JSON, REST APIs, and web services. Older messaging frameworks may contain extensible markup language (XML) and simple object access protocols (SOAP) instead of JSON and REST APIs, respectively. The messaging framework provides a standardized way to handle data transfer among different applications.
A web server is an example of middleware that connects a website to a database. The web server handles the business logic and routes the data from the database based on the request. A route is the part of the code that associates an HTTP request, such as GET, POST, or DELETE, with a URL and the function that gets called that handles that URL. Routing is used in web development to split an application’s user interface based on rules identified by the browser’s URL.
Router functions are called “middleware” collectively. Middleware is responsible for responding to an HTTP request or calling another function in the middleware chain. Express handles router functions through the Router class, such as Router.get(). As the name suggests, Router.get() handles HTTP GET requests. Other Router functions include Router.post(), Router.put(), Router.delete() in mostly the same way. These methods take two arguments, a URL path and a callback function.
In addition to routing, middleware is also responsible for providing secure connections among services by encrypting and decrypting data, managing application loads by distributing traffic to different servers, and sorting or filtering data before the data is returned to the client.
he authentication process confirms a user's identity using credentials by validating who they claim to be. Authentication assures an application's security by guaranteeing that only those with valid credentials can access the system. Authentication is the responsibility of an application's backend.
Three popular authentication methods in Node.js include:
Session-based
Token-based
Passwordless
Let's explain a little bit about each of these methods and compare them.
Session-based
Session-based authentication is the oldest form of authentication technology. Typically, the flow of a session is as follows:
The user uses their credentials to log in.
The login credentials are verified against the credentials in a database. The database is responsible for storing which resources can be accessed based on the session ID.
The server creates a session with a session ID that is a unique encrypted string. The session ID is stored in the database.
The session ID is also stored in the browser as a cookie.
When the user logs out or a specified amount of time has passed, the session ID is destroyed on both the browser and the database.
Token-based
Token-based security entails two parts: authentication and authorization. Authentication is the process of providing credentials and obtaining a token that proves the user's credentials. Authorization refers to the process of using that token so the resource server knows which resources the user should have access to.
Token-based Authentication
Token-based authentication uses access tokens to validate users. An access token is a small piece of code that contains information about the user, their permissions, groups, and expirations that get passed from a server to the client. An ID token is an artifact that proves that the user has been authenticated.
The token contains three parts, the header, the payload, and the signature. The header contains information about the type of token and the algorithm used to create it. The payload contains user attributes, called claims, such as permissions, groups, and expirations. The signature verifies the token's integrity, meaning that the token hasn’t changed during transit. A JSON web token, pronounced "jot" but spelled JWT, is an internet standard for creating encrypted payload data in JSON format.
A user's browser makes a call to an authentication server and gets access to a web application. The authentication server then passes back an ID token which is stored by the client as an encrypted cookie. The ID token is then passed to the app on the web server as proof that the user has been authenticated.
Token-based Authorization
This flowchart shows the workflow of a token through the authorization process.
The authorization process gets executed when the web application wants to access a resource, for example, an API that is protected from unauthorized access. The user authenticates against the Authorization server. The Authorization server creates an access token (note that the ID token and access token are two separate objects) and sends the access token back to the client, where the access token is stored. Then when the user makes requests or resources, the token is passed to the resource, also called an API server. The token gets passed with every HTTP request. The token contains embedded information about the user's permissions without the need to access those permissions from the authorization server. Even if the token is stolen, the hacker doesn't have access to the user's credentials because the token is encrypted.
Passwordless
With passwordless authentication, the user does not need login credentials, but rather, they gain access to the system by demonstrating they possess a factor that proves their identity. Common factors include biometrics such as a fingerprint, a "magic link" sent to their email address, or a one-time passcode sent to a mobile device. Password recovery systems now commonly use passwordless authentication.
Passwordless authentication is achieved using Public Key and Private Key Encryption. In this method, when a user registers for the app, the user's device generates a private key/public key pair that utilizes a factor that proves their identity, as noted above.
The public key is used to encrypt messages, and the private key is used to decrypt them. The private key is stored on the user's device, and the public key is stored with the application and registered with a registration service.
Anyone may access the public key, but the private key is only known to the client. When the user signs into the application, the application generates a login challenge, such as requesting biometrics, sending a "magic link", or sending a special code via SMS, encrypting it with the public key. The private key allows the message to be decrypted. The app then verifies the sign-in challenge and accepts the response to authorize the user.
In this reading, you learned that:
Authentication is the process of confirming a user's identity using credentials by validating who they claim to be.
Session-based authentication uses credentials to create a session ID stored in a database and the client's browser. When the user logs out, the session ID is destroyed.
Token-based authentication uses access tokens, often JWTs, that get passed between server and client with the data that is passed between the two.
Passwordless authentication uses public/private key pairs to encrypt and decrypt data passed between client and server without the need for a password.
After reading this document, you should be able to:
Define terms related to HTTP methods
Explain guidelines and best practices for writing REST APIs
The internet relies on a client-server architecture. The end-user interfaces with the client, while the servers house the services that operate the applications, the business logic, and the data. Clients communicate with the servers to achieve desired functionality for the end user. Data is transferred between client and server using hypertext transfer protocol, more commonly known as HTTP. This communication usually happens via APIs. In 2000, a set of guidelines for writing these APIs for a client-server architecture was developed called REST APIs.
The acronym "REST" stands for REpresentational State Transfer. Before explaining this term in more detail, let's discuss HTTP methods and some terminology first.
In a client/server architecture, the applications are composed of one or more services that reside on the servers. These services contain resources. The client makes a request for a resource via a request object using a route that has an endpoint within the service. The application sends a response object back in response to the client to honor that request.
A request object contains three parts, a URL, a request header, and a request body. The server uses the URL to identify the service and the endpoint within the service being acted upon. The URL contains
four parts: a protocol, a hostname, a path, and a query string. The request header contains metadata about the resource of the requesting client, such as the user agent, host, content type, content length, and what type of data the client should expect in the response.
The server responds with a response object consisting of a header23, a body24, and a status code. The response object body often contains a JSON payload to provide the data back to the client.
There are a number of HTTP methods that can be used in the REST API that allow interaction between the client and a service. The most common methods are GET,POST, PUT, DELETE, and PATCH.
The name of the method describes what happens to the resource when the method is applied. PUT and DELETE methods result in idempotent data if the same API method is called multiple times.
HTTP has three ways to pass parameters: the URL path parameter, the URL query parameter, and the header parameter. The path and query parameters are passed as part of the URL, but the header parameter is passed by the browser directly to the service.
When the service completes a request, it returns a response. An HTTP status code should be part of that response. The HTTP status code indicates whether the response has been completed or not. Response
code categories are shown in the following table.
Status Code Range | Meaning |
200-299 | Everything is OK |
300-399 | Resource has moved |
400-499 | Client-side error |
500-599 | Server-side error |
As mentioned earlier, REST is a set of guidelines. There are five requirements for an API to be considered
RESTful, plus one optional criterion:
The API leverages a client-server architecture made up of resources that are managed and
delivered via HTTP.Communication between client and server is stateless.
Data is cacheable to improve performance on the client side.
The interface is transferred in a standard format such that the requested resources stored on the server are separated from the representation sent to the client. The representation sent to the client contains sufficient data so that the client can manipulate the representation.
Requests and responses communicate through different layers, such as middleware. The client and server often do not communicate directly with each other.
(Optional) Resources are usually static but can also contain executable code. The code should only be executed when the client requests it.
When the client makes a request, it also must pass information about its state to the server. Every communication between the client and server should contain all the information needed to perform the request. The client, not the service, maintains the state. Each request must contain the requisite information so the server understands the request. So, for example, if the user is viewing a database record and that record needs to be updated, the client must also send which record needs updating. The server doesn't know which record is currently being viewed.
An @app.route() method is used when defining a RESTful service. This method takes two parameters: the route from the URL to the service being acted upon and an optional HTTP method parameter such as POST, GET, etc. The route parameter can include variables, such as <username>. The root of a route is represented by '/'. For example, if you want the route to be www.mywebsite.com/accounts, you only need to specify '/accounts' as the route parameter in the @app.route() function.
REST APIs have the following characteristics:
Resource-based; that is, they describe sets of resources
Only contain nouns, not verbs
Use singular nouns when referring to a singular resource or plural nouns when referring to a collection of resources
Always identified by URLs
NOT RESTful APIs | RESTful equivalents |
GET http://api.myapp.com/getUser/123 | GET http://api.myapp.com/users/123 |
POST http://api.myapp.com/addUser | POST http://api.myapp.com/users |
GET http://api.myapp.com/removeUser/123 | DELETE http://api.myapp.com/users/123 |
URL format guidelines
Should use a slash '/' to denote a hierarchical relationship in the directory structure
Should avoid using a trailing slash, e.g., /resource/
Should use hyphens, not camel case, e.g., /my-resource, not /myResource
Should not use an underscore '_' in the URL, e.g., /my-resource, not /my_resource
Should use lowercase
Should not use a period '.' in a URL
May contain multiple subordinate resources and IDs in the URL, e.g., GET /resource/{id}/subordinate/{id}
Terms and Definitions
Term | Description |
1.Service | A software component that makes up part of an application and serves a specific purpose. Generally, a service takes input from a client or another service and produces an output. |
2.HTTP | The protocol used by a client-server architecture on the internet for fetching or exchanging resources data. |
3.API | An Application Programming Interface is a set of definitions and protocols that allow two services to communicate with each other. The API requests data that can be exchanged between a resource and the results that are returned from that resource. |
4.REST | A set of architectural guidelines that describe how to write an interface (API) between two components, usually a client and server, that describe how these components communicate with each other. REST stands for REpresentational State Transfer. REST describes a standard way to identify and manipulate resources. REST ensures the messages passed between the client and server are self-descriptive and define how the client interacts with the server to access resources on the server. |
5.Resource | A resource is the fundamental concept of a RESTful API. It is an object that has a defined type, associated data, relationships to other resources, and a set of methods that can operate on it. A resource is commonly defined in JSON format but can also be XML. |
6.Request | A request is made by a client to a host on a server to access a resource. The client uses parts of a URL to determine the information needed from the resource. Most common request methods include GET, POST, PUT, PATCH, and DELETE but also include HEAD, CONNECT, OPTIONS, TRACE, and PATCH. |
7.Request Object | Contains the HTTP request data. It contains three parts: a URL, a header, and a body. |
8.Route | The combination of an HTTP method and the path to the resource from the root of the path. |
9.Endpoint | The location of the resource specified by a REST API that is being accessed on the server. It is usually identified through the URL in the HTTP method of the API. |
10.Response Object | Contains the HTTP response data in response to a request. It contains a header, a body, and a status. |
11.Response | A response is made by a server and sent to a client to either provide the client with the requested resource, tell the client the requested action has been completed, or let the client know there has been an error processing the request. |
12.URL | A "Uniform Resource Identifier" is used interchangeably with the term URL. They are part of a RESTful API that locates the endpoint of the requested resource and contains the data about how that endpoint should be manipulated. The client issues an HTTP request using the URI/URL to manipulate the resource. They should consist of four parts: the hostname, the path, the header, and a query string. |
13.Request Header | Information passed to the server about the retrieved resource or the requesting client. Examples include: |
14.Request Body | Provides the data being passed to the server. |
15.Protocol | Tells the service how the data is to be transferred between the server and the client. |
16.Hostname | The name of a device on a network, also often called the site name. |
17.Path | The path identifies the location of the resource in the service and its endpoint. For example: https://www.customerservice/customers/{customer_id} |
18.Query String | The part of a URL that contains the query. |
19.User-agent | The type of browser the client is using. |
20.Host | A computer on a network that communicates with other hosts. |
21.Content type | The media type of a resource such as text, audio, or an image. |
22.Content length | The number of bytes of data being sent in a response. |
23.Response Header | Contains metadata about the response, such as a time stamp, caching control, security info, content type, and the number of bytes in the response body. |
24.Response Body | The data from the requested resource is sent back to the client. |
25.Response Status | The return code that communicates the result of the request’s status to the client. |
26.JSON | "JavaScript Object Notation" is a format for storing and transporting data, usually as a way to send data from a service on a server to the client. It consists of key-value pairs and is self-describing. The format of JSON data is the same as the code for creating JavaScript objects, making it easy to convert this data into JavaScript objects but can be written in any programming language. JSON has three data types: scalars (numbers, strings, Booleans, null), arrays, and objects. |
27.Payload | The payload is the data in the body of a response being transported from a server to the client due to an API request. |
28.GET | The GET method is used as a request that retrieves a representation of a resource. GET() should never modify a resource and only return a representation of the requested resource. |
29.POST | HTTP method that sends data to the server to create a resource and should return 201_CREATED status code. |
30.PUT | HTTP method that updates a resource or replaces an existing one. Calling PUT multiple times in a row does not have side effects, whereas POST does. It should return a 200_OK code if the resource exists and can be updated or return a 404_NOT_FOUND code if the resource doesn't exist. |
31.DELETE | HTTP method that deletes a resource and returns 204_NO_CONTENT if the resource exists and can be deleted by the server or if the resource cannot be found, which means it has already been deleted. |
32.PATCH | HTTP method that applies partial modifications to a resource. |
33.Idempotent | Describes an element of a set that remains unchanged when making multiple identical requests. PUT and DELETE methods result in idempotent data if the same API method is called multiple times. |
34.URL Path Parameter | Passed into the operation by the client as a variable in the URL's path. |
35.URL Query Parameter | Contains key-value pairs, usually in JSON format, and are separated from the path by a '?'. If there are multiple key-value pairs, they should be separated by an '&'. The query can be used to pass in a filter to be applied to the results that are returned by the operation. |
36.Header Parameter | Contains additional metadata about the query, such as identifying the client that is calling the operation. |
37.Stateless | All requests from a client to a server for resources happen in isolation from each other. The server is unaware of the application's state on the client, so this information needs to be passed with every request. |
38.Cacheable | The ability to store data on the client so that data can be used in a future request. |
39.Middleware | Software that sits between applications, databases, or services and allows those different technologies to communicate. |
Developers rely on third-party packages to extend Node.js.
You can use the npm application to manage Node.js packages in your Node.js framework installation.
The MVC architecture style divides a back-end application into three parts: the model, the view, and the controller.
REST API frameworks use HTTP methods to communicate with each other.
Express abstracts low-level details.
Routing can be handled at the application level or at the router level.
Five types of middleware are application level, router level, error handling, built-in middleware, and third party.
Template rendering is the ability of the server to fill in dynamic content.
The npm jsonwebtoken package should be required in an Express application to authenticate a user.
Express Web Application Framework
Term | Definition |
Access Token | A small piece of code that contains information about the user, their permissions, groups, and expirations that get passed from a server to the client. |
API Endpoint | The touchpoint where the API connects to the application it is communicating with. |
Application-Level Middleware | Acts as a gatekeeper and is bound to the application. No request to the application server can go past it. |
Authentication | The process of confirming a user’s identity using credentials by validating who they claim to be. Authentication assures an application’s security by guaranteeing that only those with valid credentials can access the system. |
Authorization | In token-based authentication, it is the process that gets executed when a web application wants to access a protected resource. A user authenticates against an authorization server. |
Built-In Middleware | Can be bound to either the entire application or to specific routers. Useful for activities such as rendering HTML pages from the server, parsing JSON input from the front end, and parsing cookies. |
Controller | The layer in an MVC application regulates the flow of the data. It is responsible for processing the data supplied to it by the user and sends that data to the model for manipulation or storage. |
Error-Handling Middleware | Can be bound to either the entire application or to specific routers. Error-handling middleware always takes four arguments: error, request, response, and the next function that it needs to be chained to. Even if you don’t use the next parameter, you still define it in the method signature. |
Express.js | A web application framework based on the Node.js runtime environment, however, Express abstracts low-level details. |
Framework | A framework is a skeleton on which an application is built for a specific environment. The framework is the fundamental structure that supports the application. |
HTTP Headers | Additional information about and contained in an HTTP response or request. |
HTTP Request | A method called by a client and sent to a server requesting access to a resource on the server. |
HTTP Response | A method called by a server and sent to a client in response to an HTTP request. |
ID Token | An artifact that proves that a user has been authenticated and contains information about authorized API routes. |
JSON Payload | Data that is transferred in JSON format between the client and server in the header of an HTTP method. |
JWT | A JSON Web token. An internet standard for creating encrypted payload data in JSON format. |
Middleware | Includes functions that have access to the request and response objects and the next() function. |
Model | The layer in an MVC application responsible for managing the data of the application. It interacts with the database and handles the data logic. |
MVC | Short for “Model-View-Controller”. It is an architectural pattern that divides an application into three components: model, view, and controller. |
Node Framework | A framework that works in conjunction with Node.js. A framework is a skeleton on which an application is built for a specific environment. The framework is the fundamental structure that supports the application. |
npm | An application that manages Node.js packages in your Node.js framework installation. |
Passwordless Authentication | A type of authentication that uses public/private key pairs to encrypt and decrypt data passed between client and server without the need for a password. |
Private Key | In cryptography, it is a key that is known only to a specific client used to decrypt messages. Used in conjunction with a public key. |
Public Key | In cryptography, it is a key that can be used by anyone to encrypt messages for a specific client. Used in conjunction with a private key. |
REST | “Representational state transfer” is a set of guidelines for creating stateless client/server interfaces using HTTP methods. |
REST API | An API used for communicating between clients and servers that conforms to REST architecture principles. |
Route | The code that associates an HTTP request method and a URL. |
Router-Level Middleware | Bound to a router and not bound to an application. You can use specific middleware for a specific route instead of having all requests go through the same middleware. Then you bind the application routes to each router. |
Session-based Authentication | A type of authentication where a user provides login credentials that are verified against stored credentials in a database on a server. A session ID is provided to the client and stored in the browser as a cookie. |
Statelessness | Implies that each HTTP request happens in isolation in relation to other requests. The state of the client is not stored on the server; the state is passed to the server by the client for each request. |
Template Rendering | The ability of the server to fill in dynamic content in an HTML template. |
Token | Contains three parts, the header, the payload, and the signature. The header contains information about the type of token and the algorithm used to create it. The payload contains user attributes, called claims, such as permissions, groups, and expirations. The signature verifies the token’s integrity, meaning that the token hasn’t changed during transit. |
Token-based Authentication | A type of authentication that uses access tokens, often JWTs, which get passed between server and client with the data that is passed between the two. |
TypeScript | A language that builds on top of JavaScript and provides type safety which assists with the development of large-scale applications by reducing the complexity of component development in JavaScript. |
View | The layer in an MVC application responsible for rendering the presentation of the data that is passed to it by the model. |
xml2js | Node.js package to parse a string of XML elements into a JavaScript object. |
#Some Packages and Their Descriptions
Package/Method | Description | Code Example | ||
http/createServer | http package is used for establishing remote connections to a server or to create a server which listens to client. | 1.const http = require('http'); 2.const requestListener = function(req, res) { 3.res.writeHead(200); 4.res.end('Hello, World!'); 5.} 6.const port = 8080; 7.const server = http.createServer(requestListener); 8.console.log('server listening on port: '+ port); 9.server.listen(port); | ||
new Date() | new Date() method returns the current date as an object. You can invoke methods on the date object to format it or change the timezone. | 1.module.exports.getDate = function getDate() { 2. let aestTime = new Date().toLocaleString("en-US", {timeZone: "Australia/Brisbane"}); 3.return aestTime; 4.} | ||
import() | The import statement is used to import modules that some other module has exported. A file that includes reusable code is known as a module. | 1.// addTwoNos.mjs 2.function addTwo(num) { 3.return num + 4; 4.} 5.export { addTwo }; 6.// app.js 7.import { addTwo } from './addTwoNos.mjs'; 8.// Prints: 8 9.console.log(addTwo(4)); | ||
require() | The built-in NodeJS method require() is used to incorporate external modules that are included in different files. The require() statement essentially reads and executes a JavaScript file before returning the export object. | 1.module.exports = 'Hello Programmers'; 2.let msg = require('./messages.js'); 3.console.log(msg); |
###Hope you like the blog ,Plz help me If find any mistake at Shreyash Kale or to make changes in blog .Thank you!###
This article provides an in-depth overview of Node.js and the Express.js framework, detailing their features, use cases, and how to set up a server with Express. Node.js is highlighted for its asynchronous, event-driven architecture, scalability, performance, and extensive NPM ecosystem. Express.js is noted for its minimalist design, middleware capabilities, robust routing, and seamless integration with Node.js. The article also covers key concepts like asynchronous programming with Promises and async/await, REST API principles, and various authentication methods. Finally, practical steps for setting up an Express.js server and essential terms and packages are explained to aid webImportance and relevance of using Node.js with Express.js
Subscribe to my newsletter
Read articles from Shreyash Kale directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
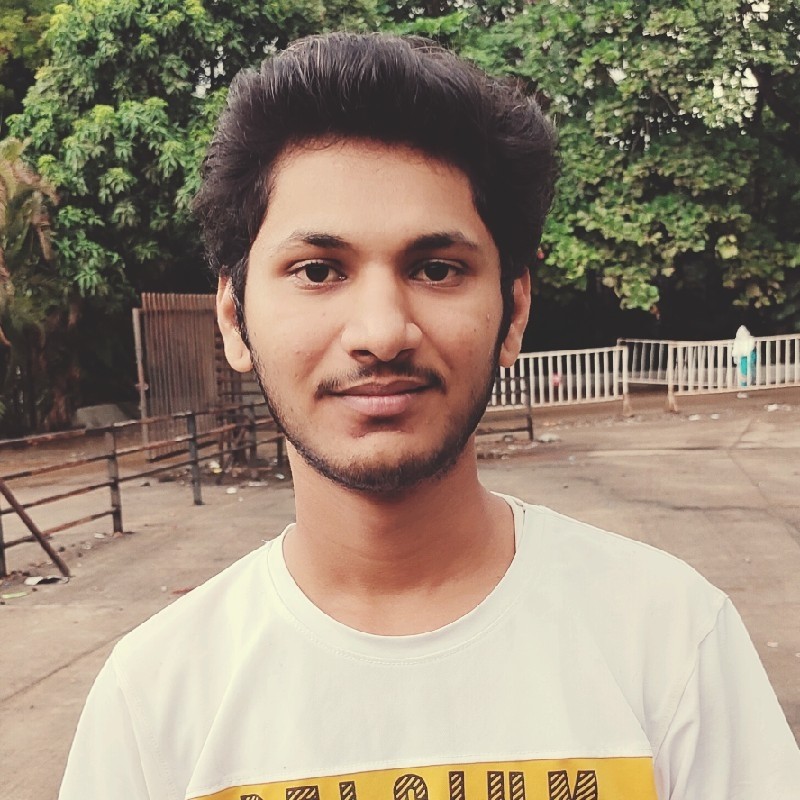
Shreyash Kale
Shreyash Kale
I am a computer engineer student ,currently in my final year . like to contribute articles, and talk about tech .