Unleashing the Power of Speculative RAG
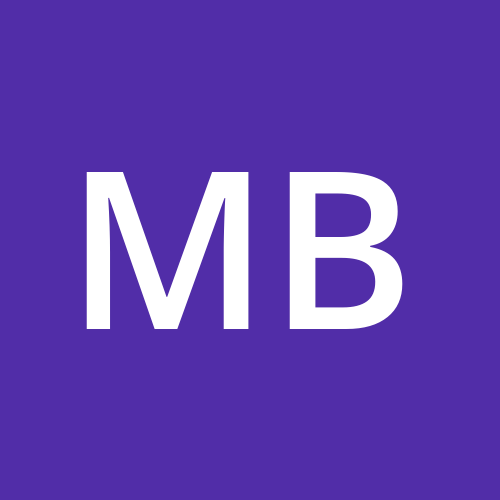
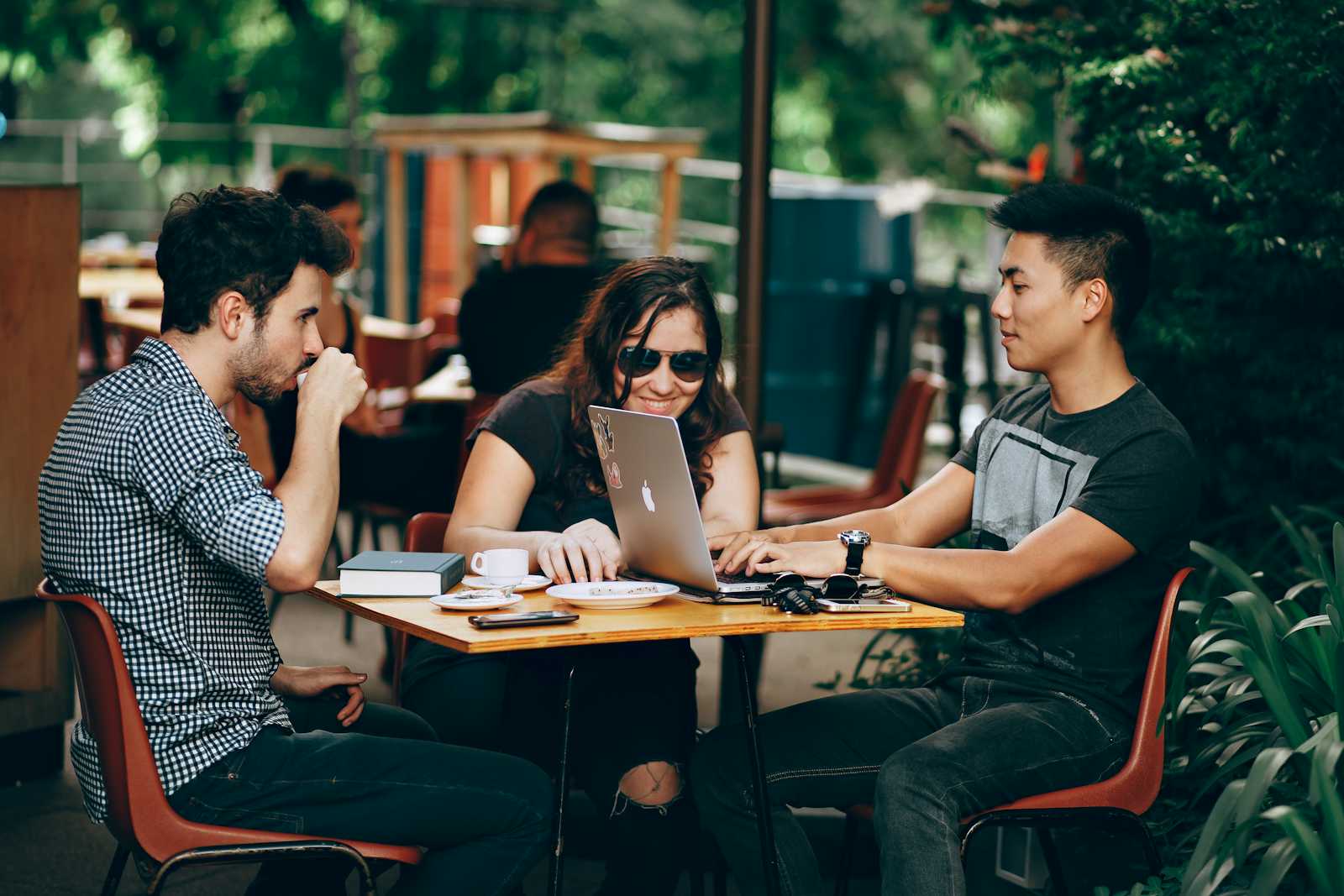
๐๐ FriYAY Demo: Speculative RAG ๐ค๐ฎ
Today we're diving into an intriguing development in the world of artificial intelligence: Speculative Retrieval-Augmented Generation, commonly known as Speculative RAG. This technique represents an interesting step forward in how AI systems can process and respond to complex queries. Let's explore how Speculative RAG works and why it's garnering attention in AI circles.
What's the Big Deal with Speculative RAG?
Imagine you're at a party, and someone asks a question. You've got a few friends there: the Quick Thinker, the Bookworm, and the Judge. Speculative RAG is like having these three friends work together to give the best possible answer. Let's break it down:
The Quick Thinker (Generalist LLM): This is the friend who quickly decides if the question needs a simple answer or if it's time to call in the big guns.
The Bookworm (Specialist LLM): If the question is tricky, this friend dives into the books (or in our case, the provided documents) and comes up with detailed answers.
The Judge (Evaluator LLM): This friend listens to all the answers from the Bookworm and picks the best one.
Now, let's take a look at the technical implementation of Speculative RAG and see how these components work together in practice.
The Code: Where the Magic Happens
Let's walk through the key parts of our Speculative RAG implementation. Don't worry if you're not a coding guru โ I'll break it down for you!
Setting the Stage
First, we need to import our tools and set up our AI models:
import os
import asyncio
from groq import AsyncGroq
from typing import List, Tuple
# Initialize Groq client
client = AsyncGroq(api_key=os.environ.get("GROQ_API_KEY"))
# Define models
generalist_model = "llama-3.1-8b-instant"
specialist_model = "mixtral-8x7b-32768"
Here, we're using the Groq API to access our AI models. The generalist is quick on its feet, while the specialist is the heavyweight champion of knowledge.
The Quick Thinker in Action
async def generalist_llm(query: str) -> Tuple[bool, str]:
messages = [
{"role": "system", "content": generalist_system_prompt},
{"role": "user", "content": query}
]
response = await call_llm(generalist_model, messages, temperature=0.5, max_tokens=10)
is_complex = response.lower().strip() == 'yes'
return is_complex, f"Generalist decision: {'Knowledge-intensive' if is_complex else 'Simple'}"
This function is our Quick Thinker. It looks at the question and decides if we need to call in the Bookworm or if it can handle it solo. The temperature=0.5
keeps it focused, and max_tokens=10
ensures a quick yes/no response.
The Bookworm's Deep Dive
async def specialist_llm(query: str, document: str) -> Tuple[str, str, str]:
messages = [
{"role": "system", "content": specialist_system_prompt},
{"role": "user", "content": f"Query: {query}\n\nDocument:\n{document}"}
]
response = await call_llm(specialist_model, messages, temperature=0.8, max_tokens=2048)
parts = response.split("Rationale:", 1)
draft = parts[0].strip()
rationale = parts[1].strip() if len(parts) > 1 else "No explicit rationale provided."
return draft, rationale, f"Specialist generated a draft."
Here's where the Bookworm shines. It takes the question and a document, then generates a detailed answer (draft) and explains its thinking (rationale). We use a higher temperature=0.8
to encourage creative thinking and allow for longer responses with max_tokens=2048
.
The Judge's Wise Decision
async def evaluator_llm(query: str, drafts_and_rationales: List[Tuple[str, str]]) -> Tuple[int, str, str]:
drafts_text = "\n\n".join([f"Draft {i+1}:\n{draft}\nRationale:\n{rationale}"
for i, (draft, rationale) in enumerate(drafts_and_rationales)])
messages = [
{"role": "system", "content": evaluator_system_prompt},
{"role": "user", "content": f"Query: {query}\n\nDrafts and Rationales:\n{drafts_text}"}
]
response = await call_llm(generalist_model, messages, temperature=0.3, max_tokens=512)
lines = response.split('\n')
best_draft_num = int(lines[0].split(':')[1].strip()) - 1
rationale = '\n'.join(lines[1:]).strip()
return best_draft_num, rationale, f"Evaluator selected Draft {best_draft_num + 1} as the best."
The Judge (Evaluator) reviews all the drafts, picks the best one, and explains why. We use a lower temperature=0.3
here because we want a more deterministic, focused decision.
Putting It All Together
The heart of our Speculative RAG system is the speculative_rag
function:
async def speculative_rag(query: str, documents: List[str]) -> Tuple[str, str]:
process_log = []
# Step 1: Quick Thinker decides
is_complex, gen_log = await generalist_llm(query)
process_log.append(gen_log)
if is_complex:
# Step 2: Bookworm generates drafts
tasks = [process_document(query, doc) for doc in documents]
drafts_and_rationales = await asyncio.gather(*tasks)
process_log.append(f"Specialist generated {len(drafts_and_rationales)} drafts.")
# Step 3: Judge selects the best draft
best_draft_num, eval_rationale, eval_log = await evaluator_llm(query, drafts_and_rationales)
process_log.append(eval_log)
# Step 4: Craft final response using the best draft
best_draft = drafts_and_rationales[best_draft_num][0]
final_response, final_log = await final_response_llm(query, best_draft, eval_rationale)
process_log.append(final_log)
else:
# For simple queries, Quick Thinker handles it
final_response = await call_llm(generalist_model, [
{"role": "system", "content": "You are a helpful assistant. Please answer the following query concisely."},
{"role": "user", "content": query}
], temperature=0.7, max_tokens=512)
process_log.append(f"Simple query: Generalist provided the response.")
return final_response, "\n".join(process_log)
This function orchestrates the whole show. It's like the party host making sure everyone plays their part:
The Quick Thinker assesses the question.
If it's complex, the Bookworm generates answers for each document.
The Judge picks the best answer.
We use the best answer to craft a final, polished response.
If the question is simple, the Quick Thinker handles it directly. Efficiency at its finest!
Why This is Cool
Speculative RAG is like having a team of AI experts working together to answer your questions. It's smart enough to handle simple queries quickly and to dive deep when needed. Plus, it can use multiple documents to generate comprehensive answers.
The best part? It's all happening behind the scenes. To the user, it looks like they're just asking a question and getting a great answer. But we know the truth โ there's a whole party of AI friends working hard to make it happen!
Wrapping Up
And there you have it, folks! We've just taken a whirlwind tour of Speculative RAG. We've seen how it combines the speed of a quick-thinking generalist with the depth of a knowledgeable specialist and the wisdom of a fair judge.
This approach opens up exciting possibilities for more efficient, accurate, and context-aware AI interactions. Whether you're building a chatbot, a question-answering system, or just exploring the frontiers of AI, Speculative RAG is definitely a technique worth keeping in your toolbox.
Remember, the key to great AI is not just about having a big brain โ it's about knowing when to think quick, when to dig deep, and when to call for a second opinion. Speculative RAG does all of that and more!
So, next time you're chatting with an AI and it gives you a surprisingly good answer, you can smile and think, "I know what's going on behind the curtain!" And who knows? Maybe you'll be inspired to create your own AI party with Speculative RAG as the guest of honor.
Until next time, keep coding, keep learning, and keep pushing the boundaries of what's possible with AI!
Grab the code at replit
Subscribe to my newsletter
Read articles from Martin Bowling directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
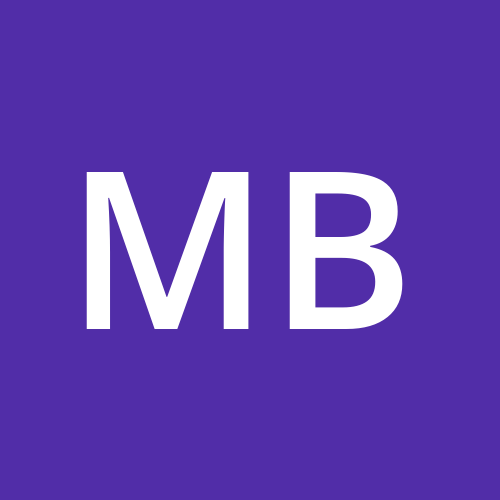