Chapter - 1

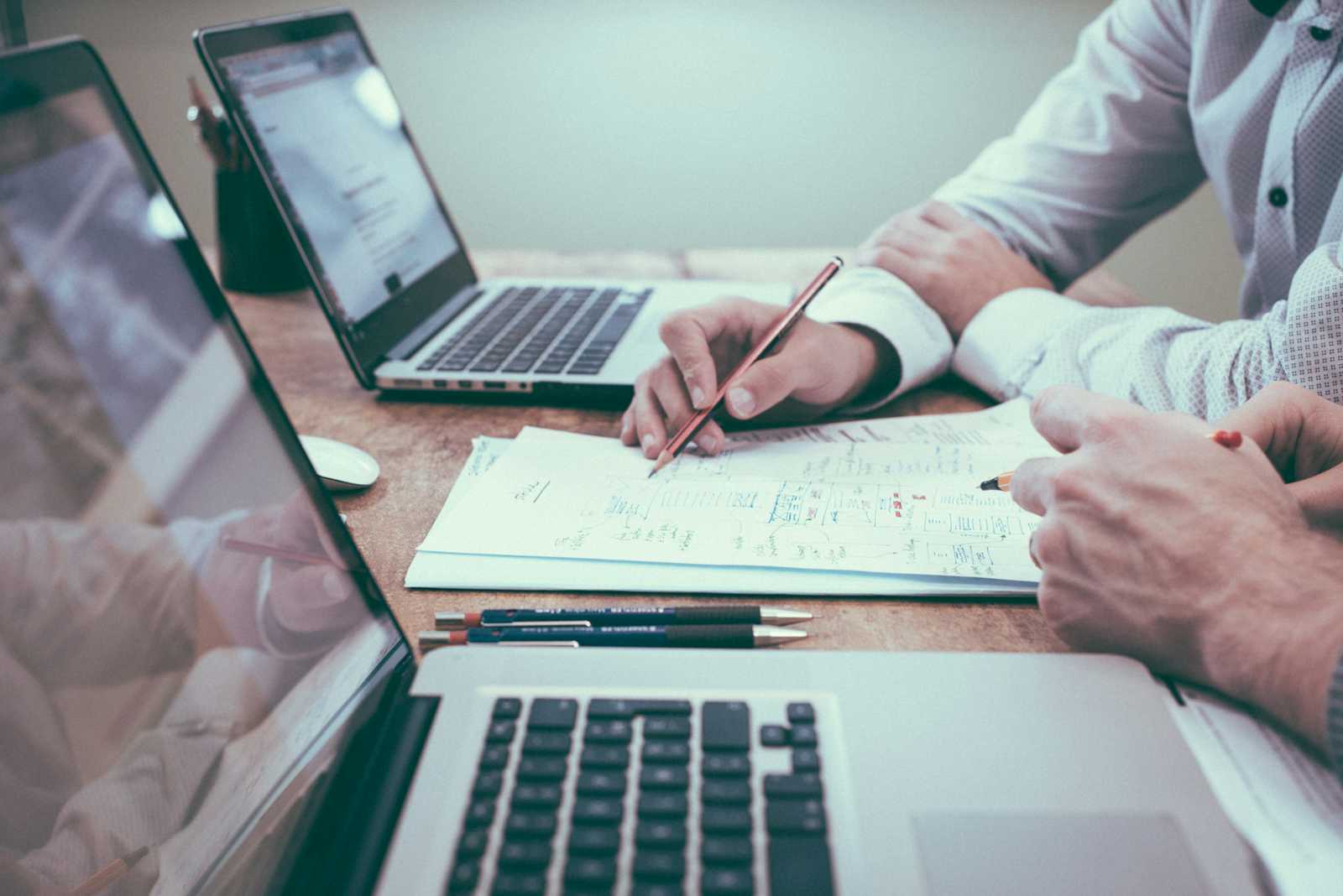
Installation
We will download rust through -> rustup (a command line tool for managing Rust versions and associated tools)
To install the Rust on the Linux:
$ curl --proto '=https' --tlsv1.2 https://sh.rustup.rs -sSf | sh
To check the version of the rust:
$ rustc --version
We will also need linker if we face anly linker issue for which we have to download C compiler.
To download the C compiler (gcc) in Linux:
$ sudo apt install build-essential
To update the Rust version:
$ rust update
To uninstall the Rust:
$ rust self uninstall
In VS Code download the rust-analyzer extension to work with the rust.
Hello World in Rust
fn main(){
println!("Hello World");
}
Save this file with name main.rs
To compile and run the file :
rustc main.rs
It will make a binary executed file "main"
To run the "main" file :
./main
Output -> Hello World
In rust only main fn is run. Other code outside the main will not be executed.
To format the code :
$ rustfmt main.rs
! -> means that we are calling a macro instead of a normal function
Cargo
Like in node we do "npm init" if we want to create a project, in Rust we use Cargo
To create a project "hello"
cargo new hello
It will make the folder with name "hello" which contains config file and the src folder which conatains the main files which are to be executed .
Folder Structure :
Cargo.toml -> It is the config file for the project. It conatins the version, name and the dependencied we are working with.
src
|_ main.rs -> This is the entry point of the project.
To build the cargo project :
$ cargo build
It will create the target folder and Cargo.lock(config file).
To run the project main file :
./target/debug/project
We can also run the project by :
$ cargo run
To check the errors in the project :
$ cargo check
It will compile but do not make the executable file.
Building for release
When our project is ready for release, we can release it using:
$ cargo build --release
It will make the program optimize and faster but it takes more compilation time, so it is better to it use when our project is ready to go to production.
Subscribe to my newsletter
Read articles from Kartik Sangal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
