What is Dispatcher, Thread and Threadpool?
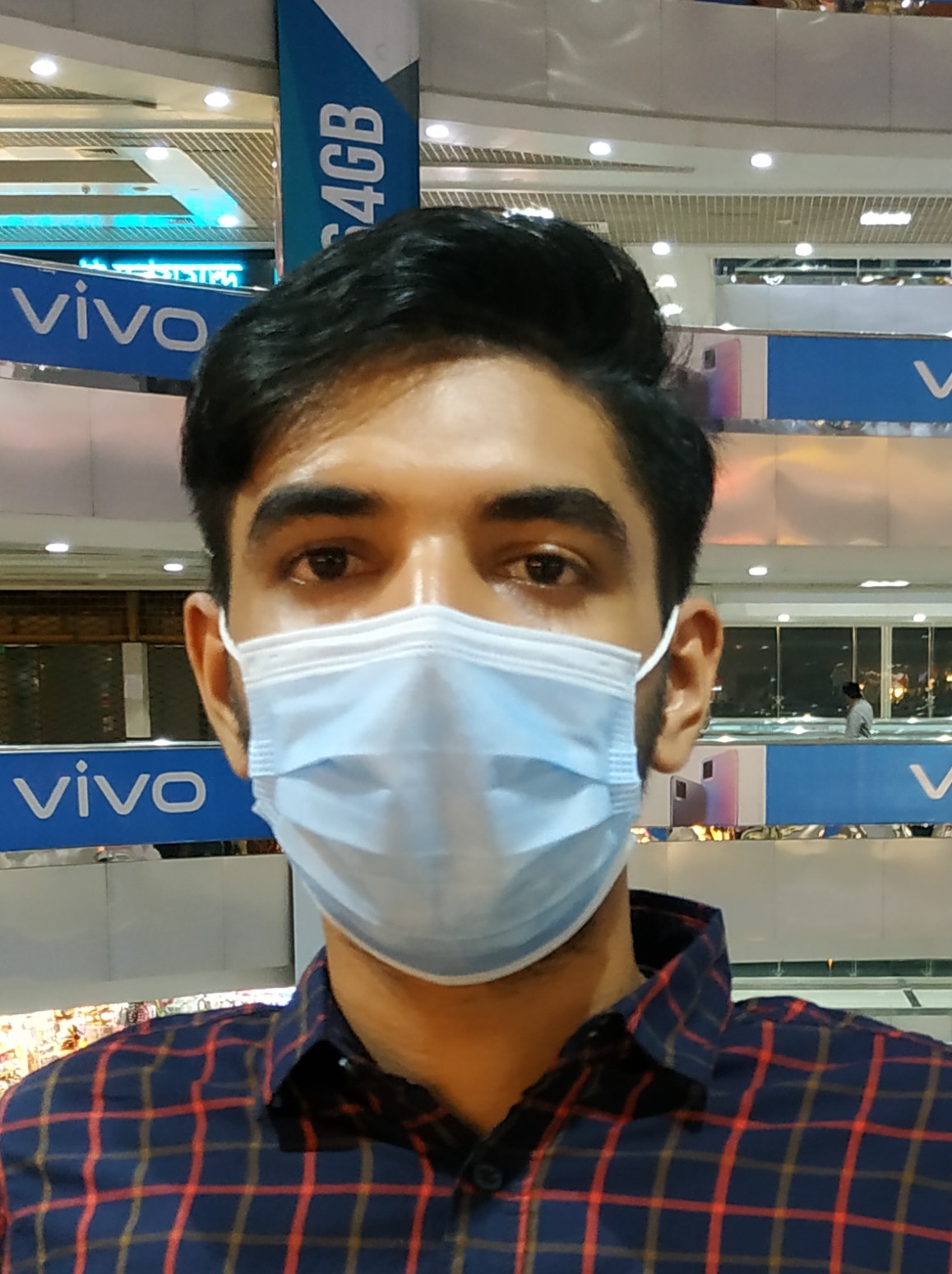
What is a Dispatcher in Kotlin Coroutines?
In Kotlin coroutines, a dispatcher is responsible for determining the thread or thread pool where a coroutine will execute. Dispatchers control the threading behavior of coroutines, allowing you to specify whether a coroutine should run on the main thread, a background thread, or a specific thread pool.
Types of Dispatchers
Kotlin provides several built-in dispatchers that you can use to control the execution context of your coroutines:
Dispatchers.Default
:This dispatcher is optimized for CPU-intensive tasks that require significant processing power (e.g., sorting large lists, parsing JSON, etc.).
It uses a shared pool of threads, known as a thread pool, which is typically based on the number of CPU cores available. This allows it to run multiple coroutines in parallel efficiently.
-
Designed for I/O-bound tasks like reading from or writing to files, making network requests, or interacting with databases.
It uses a thread pool optimized for I/O operations, which can handle a larger number of threads because I/O tasks often involve waiting (e.g., waiting for a network response), allowing threads to be reused effectively.
Dispatchers.Main
:This dispatcher is used for tasks that need to run on the main (UI) thread, such as updating the UI in Android apps.
Since the main thread handles user interactions and UI updates, you should only run lightweight tasks on it to avoid freezing the UI.
Dispatchers.Unconfined
:This dispatcher starts the coroutine in the current thread, but it can later resume in a different thread, depending on the suspension point.
It’s generally used for specific use cases where you need to avoid the overhead of context switching but should be used cautiously.
What Does the Dispatcher Do?
Thread Assignment:
- The primary role of a dispatcher is to assign the coroutine to an appropriate thread or thread pool. For example, if you use
Dispatchers.IO
, the dispatcher ensures that the coroutine runs on a thread that is optimized for I/O operations.
- The primary role of a dispatcher is to assign the coroutine to an appropriate thread or thread pool. For example, if you use
Context Switching:
- When a coroutine suspends (e.g., waiting for a network request), the dispatcher can switch the coroutine's execution to another thread when it resumes. This context switching is managed by the coroutine framework and the dispatcher, allowing efficient use of threads.
Load Balancing:
- Dispatchers help balance the load across threads. For example,
Dispatchers.Default
might spread CPU-intensive tasks across multiple CPU cores, ensuring that no single thread is overloaded.
- Dispatchers help balance the load across threads. For example,
How Do Threads and Thread Pools Fit In?
Thread:
A thread is the smallest unit of processing that can be scheduled by the operating system. Threads run code and can operate concurrently, which allows multiple tasks to be performed simultaneously.
Each thread has its own stack and local variables but shares memory with other threads in the same process. This shared memory allows threads to communicate but can also lead to issues like race conditions if not handled carefully.
Thread Pool:
A thread pool is a collection of threads that can be reused to execute multiple tasks. Instead of creating a new thread for each task (which is resource-intensive and slow), tasks are submitted to the pool, and the pool manages the reuse of threads.
Advantages:
Efficiency: Thread pools reduce the overhead of creating and destroying threads.
Resource Management: They limit the number of concurrent threads, preventing the system from being overwhelmed.
Task Queueing: Tasks are queued if all threads are busy, ensuring that they will be executed as soon as a thread becomes available.
How Dispatchers Use Threads and Thread Pools
Dispatchers.Default
: Uses a thread pool with a number of threads based on the available CPU cores. It optimizes for CPU-bound tasks by spreading them across multiple threads.Dispatchers.IO
: Uses a thread pool with a large number of threads, as I/O tasks often involve waiting. This allows the system to efficiently manage many I/O-bound coroutines by reusing threads.Dispatchers.Main
: Tied to the main thread, typically in UI frameworks like Android. This dispatcher ensures that the coroutine runs on the main thread to update the UI.Dispatchers.Unconfined
: Doesn’t confine the coroutine to a specific thread or thread pool. It runs on the current thread but can switch threads when it suspends and resumes, depending on the suspension point.
Example: Using Dispatchers
Here’s a simple example demonstrating how different dispatchers work:
kotlinCopy codeimport kotlinx.coroutines.*
fun main() = runBlocking {
// Running on Default dispatcher (CPU-bound task)
val defaultJob = launch(Dispatchers.Default) {
println("Running on Default: ${Thread.currentThread().name}")
// Simulate CPU-bound work
repeat(5) { i ->
println("Processing $i on Default")
delay(1000)
}
}
// Running on IO dispatcher (I/O-bound task)
val ioJob = launch(Dispatchers.IO) {
println("Running on IO: ${Thread.currentThread().name}")
// Simulate I/O-bound work
repeat(5) { i ->
println("Reading $i on IO")
delay(1000)
}
}
// Running on Main dispatcher (UI thread)
val mainJob = launch(Dispatchers.Main) {
println("Running on Main: ${Thread.currentThread().name}")
// Simulate UI work
repeat(5) { i ->
println("Updating UI $i on Main")
delay(1000)
}
}
defaultJob.join()
ioJob.join()
mainJob.join()
}
Summary
Dispatchers in Kotlin coroutines determine which thread or thread pool a coroutine runs on.
Threads are the basic units of execution, and thread pools manage multiple threads efficiently.
Dispatchers.Default and Dispatchers.IO use thread pools to manage CPU-bound and I/O-bound tasks, respectively.
Dispatchers.Main ensures coroutines run on the main thread, which is crucial for UI updates.
Dispatchers allow you to control the threading behavior of your coroutines, making it easier to write efficient and responsive applications.
Subscribe to my newsletter
Read articles from Rafiul Islam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
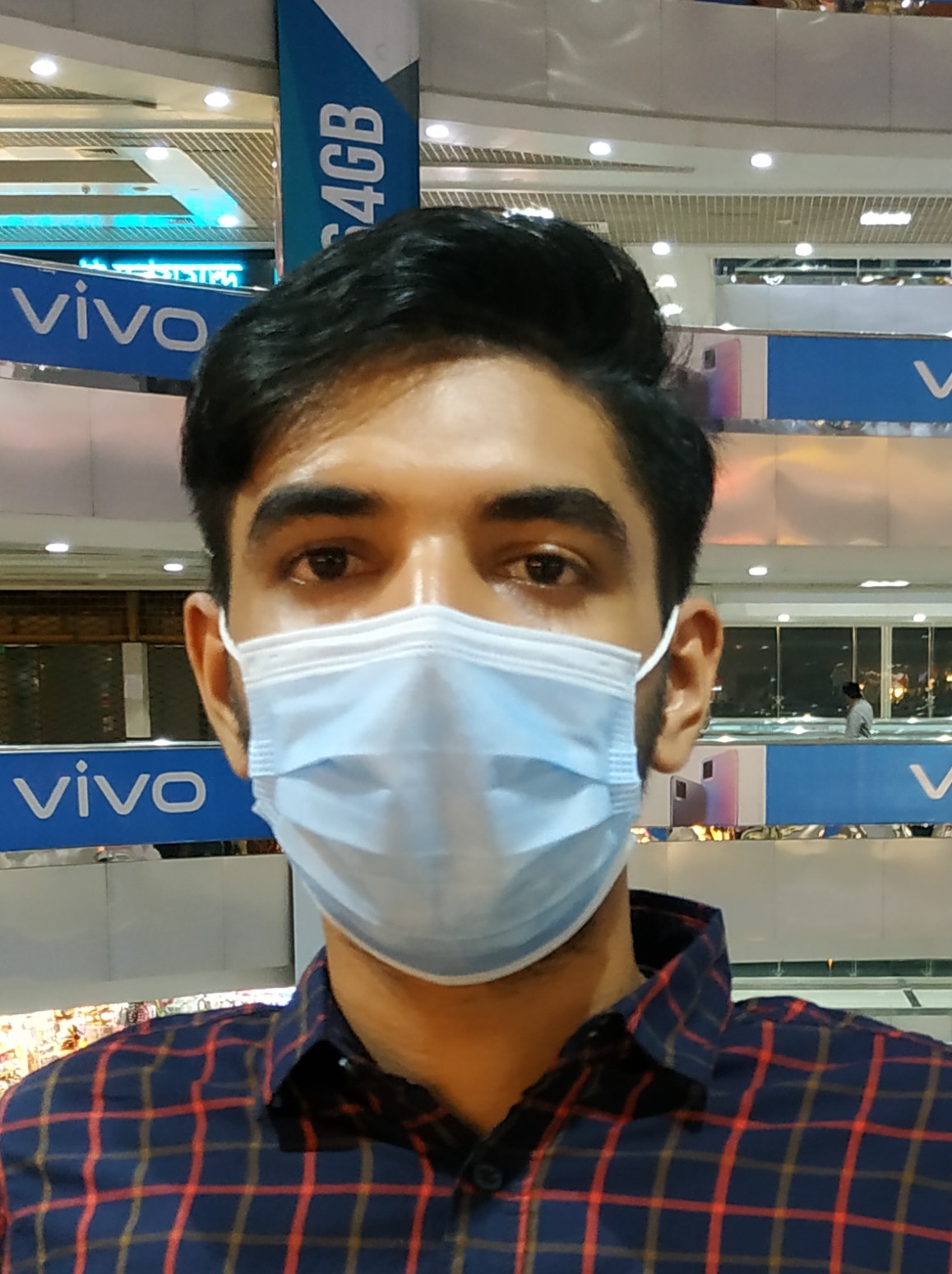