Coroutines vs. Callbacks: The Similarity
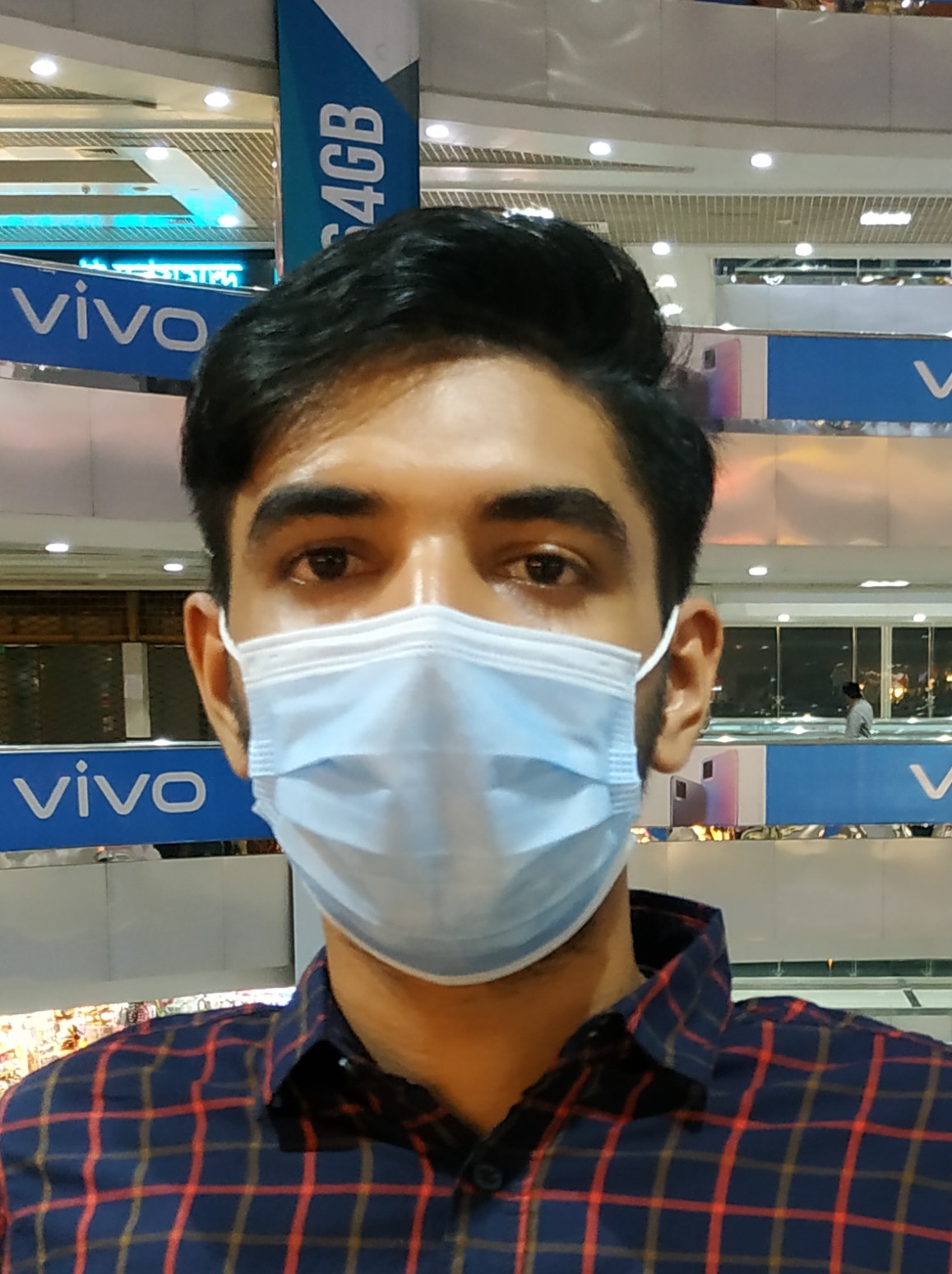
Callbacks: In traditional asynchronous programming, especially in languages like JavaScript, you might pass a callback function to an asynchronous operation. Once the operation completes, the callback is invoked to continue processing. This is essentially a manual way of suspending and resuming execution:
javascriptCopy codefunction fetchData(callback) { setTimeout(() => { callback("Data received"); }, 1000); } fetchData((data) => { console.log(data); // "Data received" after 1 second });
Coroutines: Coroutines automate and abstract away the callback mechanism. Instead of manually passing callbacks, you write code that looks synchronous but is actually asynchronous. Under the hood, the coroutine framework handles the suspension and resumption of tasks, which conceptually resembles callbacks being invoked at the right time:
kotlinCopy codeimport kotlinx.coroutines.* fun main() = runBlocking { val data = fetchData() println(data) // "Data received" after 1 second } suspend fun fetchData(): String { delay(1000L) return "Data received" }
Differences Between Coroutines and Callbacks
Readability and Maintainability:
Callbacks: Asynchronous code written with callbacks can quickly become hard to read, especially when multiple nested callbacks are involved (callback hell). This makes code difficult to understand and maintain.
Coroutines: Coroutines allow you to write asynchronous code in a sequential style, which is much easier to read and maintain. The code looks synchronous, but under the hood, it’s handled asynchronously.
State Management:
Callbacks: With callbacks, managing state between asynchronous calls is manual. You often need to pass state through the callback chain, which can be cumbersome.
Coroutines: Coroutines automatically manage state for you. When a coroutine suspends, the current state (variables, execution point) is captured and restored when the coroutine resumes.
Error Handling:
Callbacks: Handling errors with callbacks often involves checking for errors at each level of the callback chain, which can lead to repetitive and scattered error-handling logic.
Coroutines: Error handling in coroutines is more streamlined, as you can use
try-catch
blocks just like in synchronous code. This makes it easier to manage exceptions and keep the error-handling logic clean.
Threading:
Callbacks: Typically, callbacks execute in the thread where the asynchronous operation completes. If you want to move the execution to a different thread, you need to handle that explicitly.
Coroutines: Coroutines allow you to easily switch the execution context using
withContext
or by specifying a dispatcher. This makes managing threading concerns much more straightforward.
Cancellation:
Callbacks: Canceling an asynchronous operation using callbacks is generally manual and error-prone. You have to keep track of whether the operation should continue or abort, often leading to additional complexity.
Coroutines: Coroutines have built-in support for cancellation. If a coroutine is canceled, it automatically stops execution at the next suspension point, making cancellation easier to manage.
Under the Hood: Coroutines as Structured Callbacks
Continuation-Passing Style (CPS): Coroutines, at their core, transform your code into a continuation-passing style under the hood. This means that each suspension point in your coroutine is essentially transformed into a callback that the coroutine system manages.
State Machines: The Kotlin compiler converts coroutines into state machines. Each time a coroutine is suspended, its state is saved. When it resumes, it continues from the saved state, which is conceptually similar to a series of callbacks.
Framework Management: The coroutine framework manages these "callbacks" automatically, so you don’t have to. This includes handling resumption, error propagation, and context switching.
Conclusion
So yes, coroutines are like callbacks in the sense that they manage asynchronous operations by suspending and resuming tasks. However, they provide a much more structured, readable, and maintainable approach by abstracting away the manual management of these callbacks, allowing developers to write asynchronous code that looks and feels synchronous.
Subscribe to my newsletter
Read articles from Rafiul Islam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
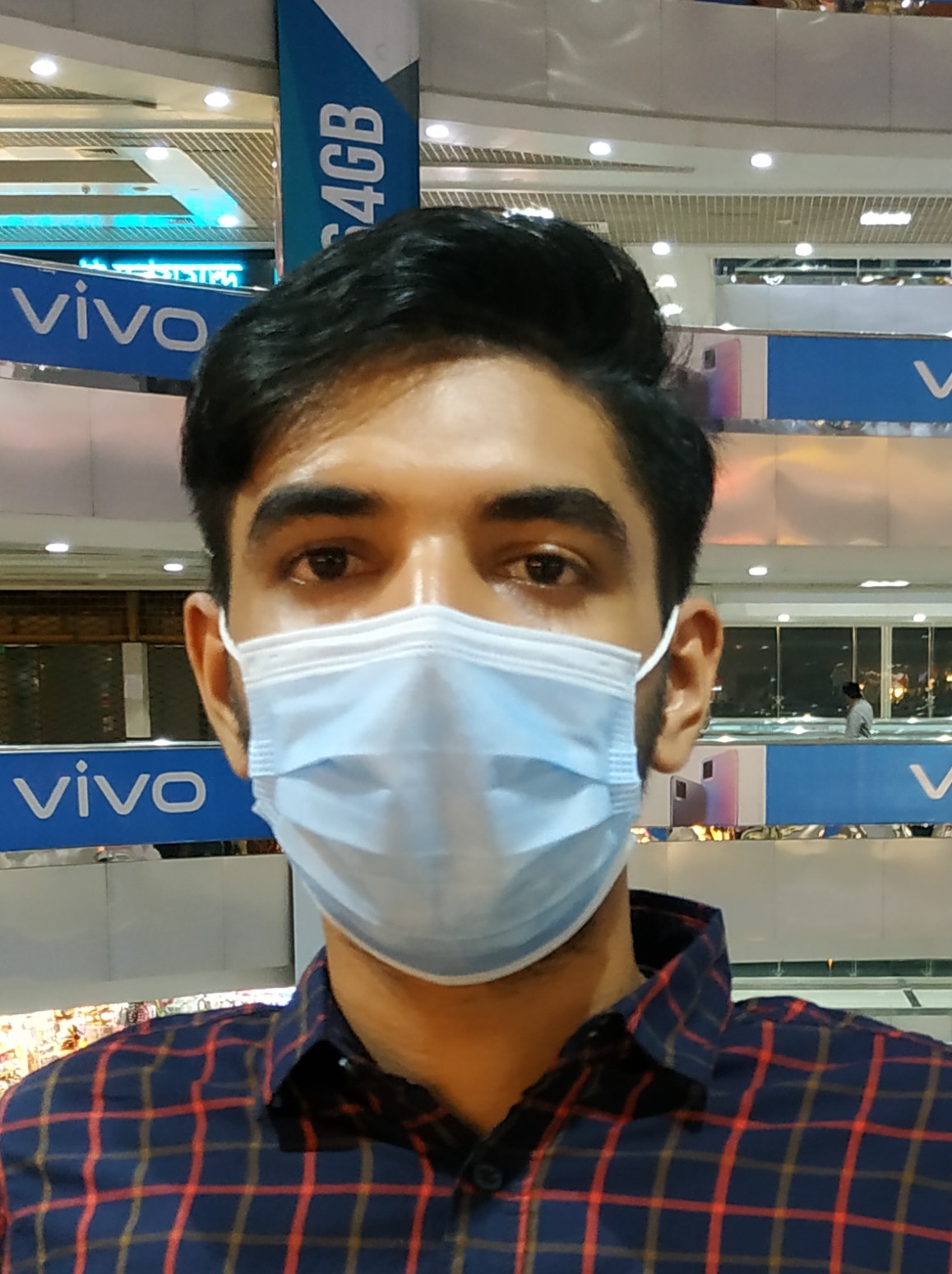