Dealing with Null Reference Exceptions

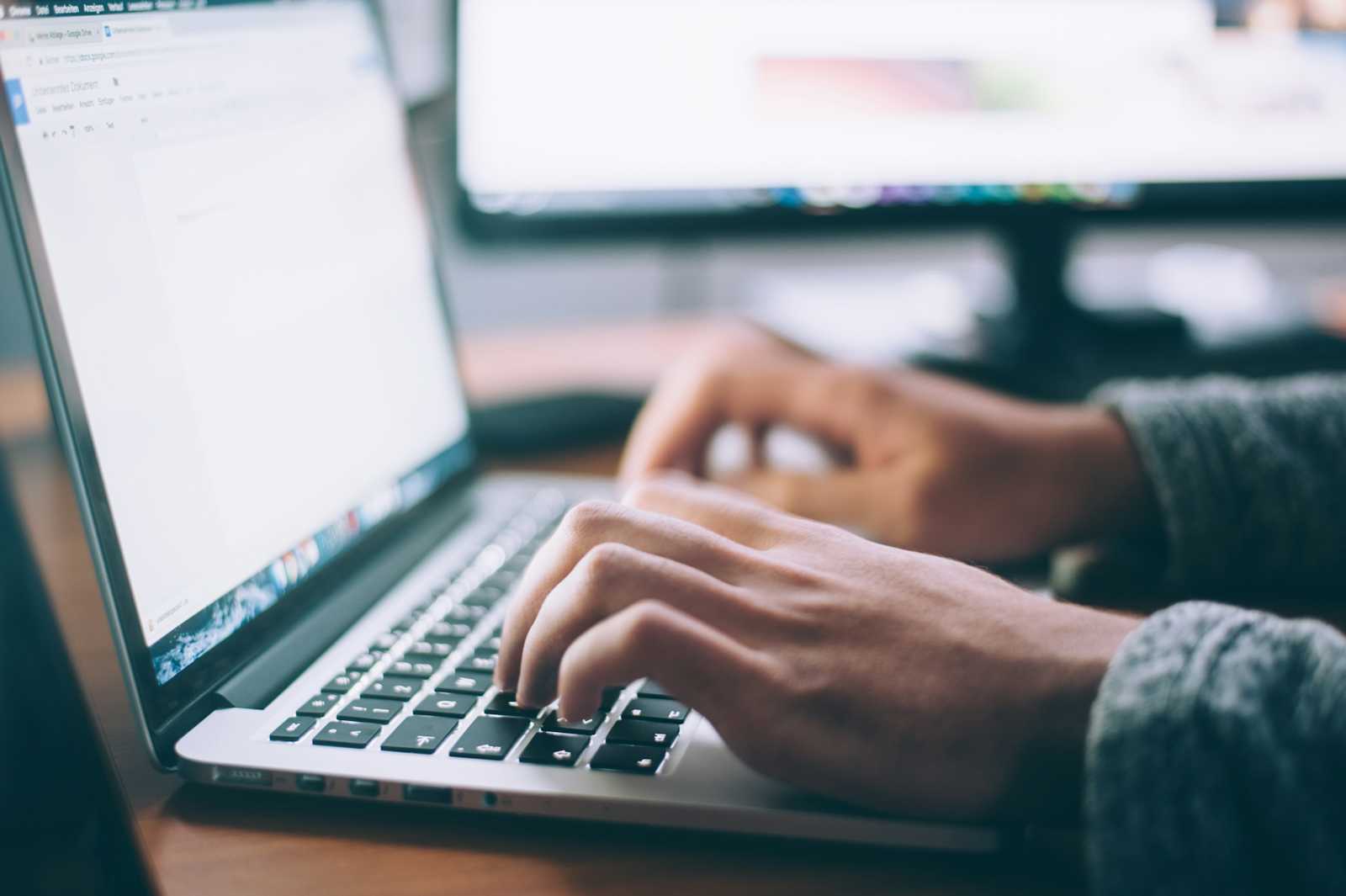
If you are a software Engineer, you might have come accross this error "Object reference not set to an instance of an object". Then you might have asked what does this even mean?
let's understand what this error means. For suppose check this below pseudo code where an invoice is geting created from order by mapping the necessary details from order to invoice before invoice invoice creation.
public void MapOrderToInvoice(object order, object invoice){
foreach(var prop in order){
invoice[prop.Key] = order[prop.Key]
}
}
public string CreateInvoice(){
//lets say the value of orderDocument will be result of an api call to fetch order data;
object order = orderDocument;
object invoice = new object();
MapOrderToInvoice(order, invoice);
var invoiceId = _invoiceService.createInvoice(invoice);
return invoiceId;
}
For some reason lets say the order document is not fetched from database, then order variable will be null, so in MapOrderToInvoice method when using foreach loop to iterate over an order object throws an exception with message stating "Object reference not set to an instance of an object" because order is null. Now lets breakdown that statement into 3 parts
Object reference
object reference means a variable which stores address to a point where an object is stored
Not set to
Not set to in this context means, the order variable is not storing the address of an object
an instance of an object
instance means the real order object that will be created, so if you combine these words you understand that the order variable is not storing address to an instance of order object because it is null.
Now let's understand why preventing this error from happening is important
As the code breaks at foreach loop line, the client will get a response like this
"Object reference not set to an instance of an object at line number so and so foreach(var prop in order).... whole call stack"
Then the consumer of this method will not know what to make out of it, this is a very bad user experience.
Now lets understnad how to prevent this, If you see the below code, In CreateInvoice method if order document is null i am ending the execution flow right there and sending the appropriate response to the consumer of this method.
public void MapOrderToInvoice(object order, object invoice){
foreach(var prop in order){
invoice[prop.Key] = order[prop.Key]
}
}
public object CreateInvoice(){
var resonse = {
isSuccesfull : false,
data : "",
errorMessage : ""
}
//lets say the value of orderDocument will be result of an api call to fetch order data;
object order = orderDocument;
if(order == null){
response.errorMessage = "order document is not present to create invoice";
return resposne;
}
JObject invoice = new JObject();
MapOrderToInvoice(order, invoice);
var invoiceId = _invoiceService.createInvoice(invoice);
response.isSuccesfull = true;
response.data = invoiceId;
return response;
}
This is just a simple example i took to demonstrate the use case, but in the real world the codebase and scenarios are very big and complex respectively. so you have to be very careful while writing the code so that the codebase should be more stable.
I want to say to you guys one more thing, there is no need to end the flow always when the object reference variable is null. It should be done only when the missing object instance is very critical to the process. when it is not critical, Write code in such a way so that it prevents that error and continues with the rest of the flow.
Different ways of working to prevent the specified error above in C#
Null-Coalescing Operator
object order = { orderId = "order-2024-0012" orderedDate = "24th sep 2024" } string orderAmount = order["orderAmount"] ?? 1; console.WriteLine(orderAmount); // prints 1 as orderAmount property is not present in order object so a default value 1 is assigned to oderAmount
Null-Conditional Operator (
?.
)object order = null; string orderAmount = order?.["orderAmount"]; Console.WriteLine(orderAmount); //prints null
As the order value is null, when ?. operator is used it tries to access orderAmount property of order object only if order is not null, if order is null then the whole expression is evaluated to null and orderAmount variable will be null.
I would say it's not possible to figure out and prevent all the possible null reference exceptions at compile time. Tools like sonar cloud would be really helpful in analyzing where all code might break due to null reference exception. Utilizing these tools could be really helpful in making the codebase robust and safe.
I write these blogs to share my learnings in my day to day job. Feel free to point any mistakes in the above explanation and give any suggestions. Thank you.
Subscribe to my newsletter
Read articles from chaitanya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
