π Refactoring Legacy Code: Strategies for Modernizing Old Projects π οΈ
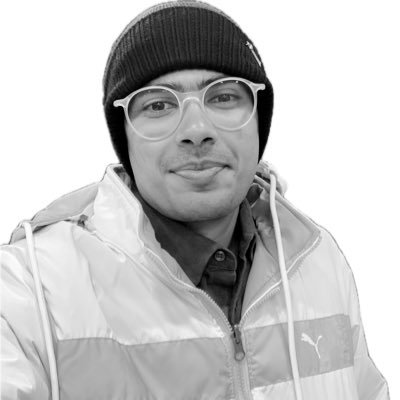
Table of contents
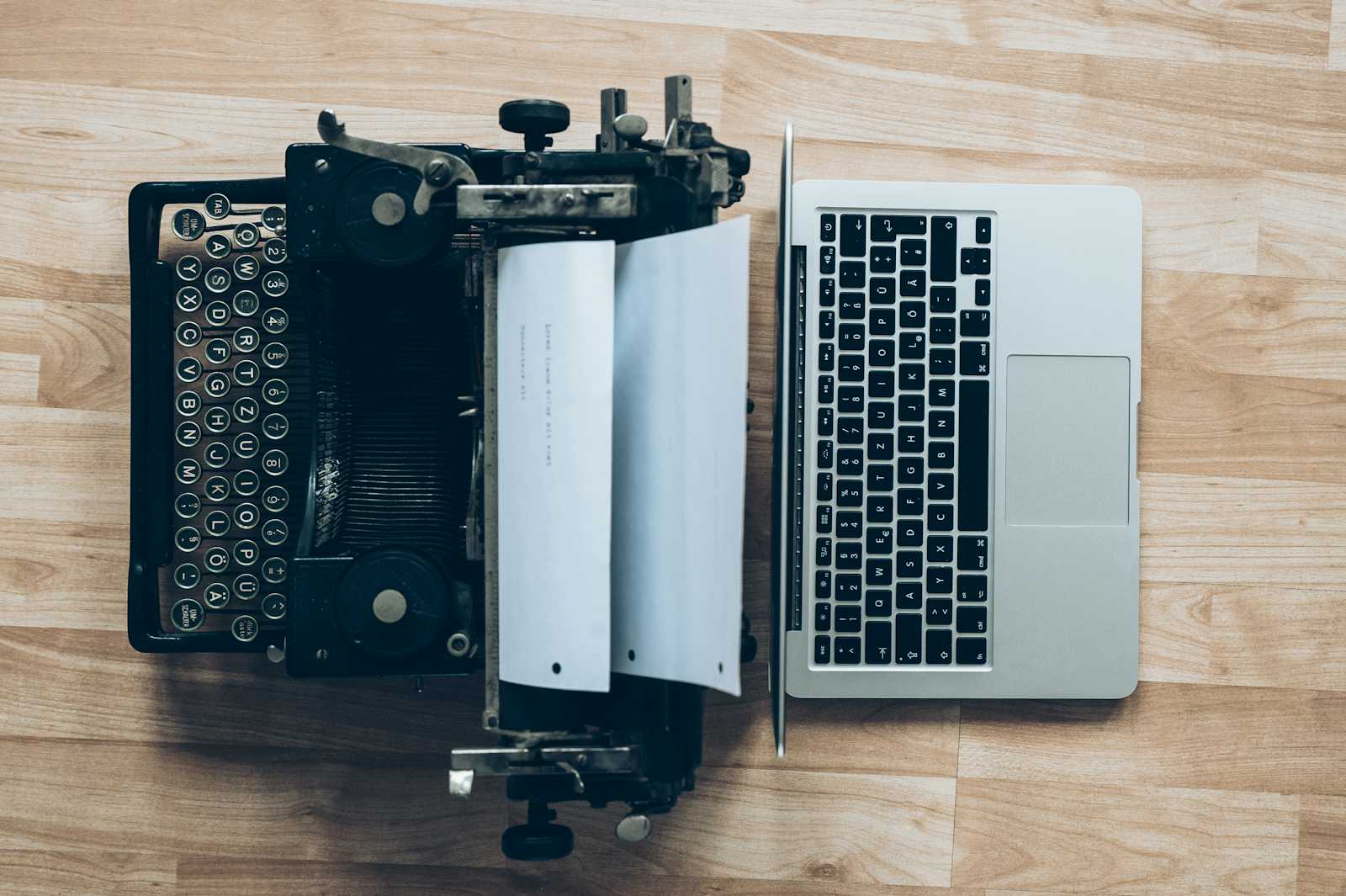
Working with legacy code is a challenge that most developers will face at some point in their careers. Whether youβre maintaining an old project, integrating new features, or improving performance, refactoring legacy code is often necessary to ensure the codebase remains maintainable, efficient, and scalable. In this blog post, we'll explore strategies for refactoring and modernizing legacy codebases, offering tips and techniques to help you tackle this complex task effectively. π‘
π§ Understanding the Legacy Code Challenge
Legacy code is often defined as code that is difficult to maintain or extend due to outdated practices, lack of documentation, or the absence of automated tests. This code may have been written years ago, by different developers, or under tight deadlines, leading to technical debt that accumulates over time. The challenge lies in modernizing the code without introducing new bugs or breaking existing functionality. π¬
π 1. Assess the Current State of the Codebase
Before diving into refactoring, it's essential to assess the current state of the codebase. This involves:
Reviewing Documentation π: If available, review any existing documentation to understand the systemβs architecture, key modules, and dependencies.
Identifying Pain Points π―: Identify areas of the code that are particularly difficult to work with or prone to bugs. These are often the best candidates for refactoring.
Analyzing Dependencies π: Understand the dependencies in the project, including external libraries and frameworks. Determine whether any of these are outdated or need to be replaced.
π» Code Example: Identifying Outdated Dependencies
// Example of outdated dependency in a package.json file
{
"dependencies": {
"express": "^3.0.0", // Old version of Express.js
"mongoose": "^4.0.0" // Old version of Mongoose
}
}
In this example, both Express.js and Mongoose are outdated and should be updated to their latest versions. π
π 2. Prioritize Refactoring Efforts
Refactoring a legacy codebase can be a massive undertaking, so it's crucial to prioritize your efforts. Start with areas that offer the most significant benefits in terms of maintainability, performance, and scalability. Focus on high-impact changes first, such as:
Improving Code Readability π: Simplify complex or convoluted code, making it easier for future developers to understand.
Modularizing the Codebase π§©: Break down monolithic code into smaller, reusable modules.
Updating Dependencies π: Upgrade outdated libraries and frameworks to their latest versions.
β¨ Code Example: Simplifying a Complex Function
// Complex legacy function
function processOrder(order) {
if (order.status === 'pending') {
// Process pending order
} else if (order.status === 'completed') {
// Process completed order
} else if (order.status === 'canceled') {
// Process canceled order
} else {
// Handle unknown status
}
}
// Refactored version using a switch statement
function processOrder(order) {
switch (order.status) {
case 'pending':
// Process pending order
break;
case 'completed':
// Process completed order
break;
case 'canceled':
// Process canceled order
break;
default:
// Handle unknown status
}
}
In this example, a complex series of if-else statements is refactored into a more readable switch statement. π οΈ
π§ͺ 3. Introduce Automated Testing
One of the biggest challenges with legacy code is the lack of automated tests. Without tests, it's difficult to ensure that refactoring efforts donβt introduce new bugs. Start by writing unit tests for the most critical parts of the codebase. As you refactor, continue to add tests to cover new code. β
π§βπ» Code Example: Adding Unit Tests
// Legacy function without tests
function add(a, b) {
return a + b;
}
// Adding unit tests using Jest
test('adds 1 + 2 to equal 3', () => {
expect(add(1, 2)).toBe(3);
});
test('adds -1 + -1 to equal -2', () => {
expect(add(-1, -1)).toBe(-2);
});
In this example, unit tests are added to ensure the add
function behaves as expected. π§©
π 4. Incremental Refactoring
Refactoring a large legacy codebase all at once is risky and can lead to significant downtime. Instead, adopt an incremental approach, refactoring small sections of the code at a time. This allows you to make continuous improvements without disrupting the entire project. π
Refactor in Small Batches π οΈ: Tackle one module, function, or file at a time. Test thoroughly after each change.
Continuous Integration (CI) π‘οΈ: Use CI tools to automate the testing and deployment of refactored code. This ensures that changes are integrated smoothly and donβt break existing functionality.
βοΈ Code Example: Incremental Refactoring with Feature Branches
# Create a feature branch for refactoring a specific module
git checkout -b refactor-user-module
# Refactor the module and commit changes
git commit -am "Refactor user module for better readability"
# Merge the feature branch back into the main branch
git checkout main
git merge refactor-user-module
In this example, a feature branch is created to refactor a specific module. After testing, the changes are merged back into the main branch. π±
π οΈ 5. Modernize the Architecture
As part of your refactoring efforts, consider modernizing the architecture of the application. This might involve:
Adopting Modern Frameworks π: Replace outdated frameworks with modern alternatives that offer better performance and maintainability.
Implementing Design Patterns π§©: Introduce design patterns such as MVC (Model-View-Controller) to improve code organization and scalability.
Decoupling Components β¨: Break down tightly coupled components into independent, reusable services or modules.
π Code Example: Refactoring to Use Modern Frameworks
// Legacy code using an outdated framework
var express = require('express');
var app = express();
app.get('/', function(req, res) {
res.send('Hello World!');
});
// Refactored code using modern syntax and features
import express from 'express';
const app = express();
app.get('/', (req, res) => {
res.send('Hello World!');
});
In this example, the legacy code is refactored to use modern JavaScript syntax and features. π
π 6. Improve Documentation
Refactoring provides an excellent opportunity to improve or create documentation for the codebase. As you refactor, document the changes you make, the reasons behind them, and how the new code should be used. Good documentation is essential for maintaining code quality over time. π
Comment the Code ποΈ: Add comments to explain complex logic or decisions.
Update README Files π: Ensure that README files reflect the current state of the project and provide clear instructions for setup and usage.
Create a Wiki π₯οΈ: If the project is large, consider creating a wiki or other documentation site to house detailed information about the codebase.
π 7. Monitor Performance
As you refactor, keep an eye on the performance of the application. Use profiling tools to identify bottlenecks and ensure that your changes are not negatively impacting performance. Optimize critical sections of the code for speed and efficiency. β‘
β±οΈ Code Example: Profiling and Optimizing a Function
console.time('processData');
// Optimized code for processing data
processData(data);
console.timeEnd('processData');
In this example, the console.time
function is used to measure the performance of the processData
function, helping to identify areas for optimization. π οΈ
Refactoring legacy code can be a daunting task, but with the right strategies and techniques, it can lead to significant improvements in maintainability, performance, and scalability. By assessing the current state of the codebase, prioritizing refactoring efforts, introducing automated testing, and modernizing the architecture, you can breathe new life into old projects and ensure they continue to meet the demands of modern development. π
Remember, refactoring is an ongoing process, not a one-time task. Continuously improving the codebase will pay off in the long run, making it easier to add new features, fix bugs, and maintain the project over time. π‘
Happy coding! π
Subscribe to my newsletter
Read articles from Vivek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
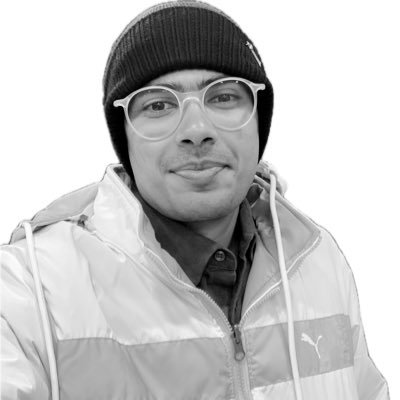
Vivek
Vivek
Curious Full Stack Developer wanting to try hands on β¨οΈ new technologies and frameworks. More leaning towards React these days - Next, Blitz, Remix π¨π»βπ»