Building a Meme Memory Card Game with AWS Deployment

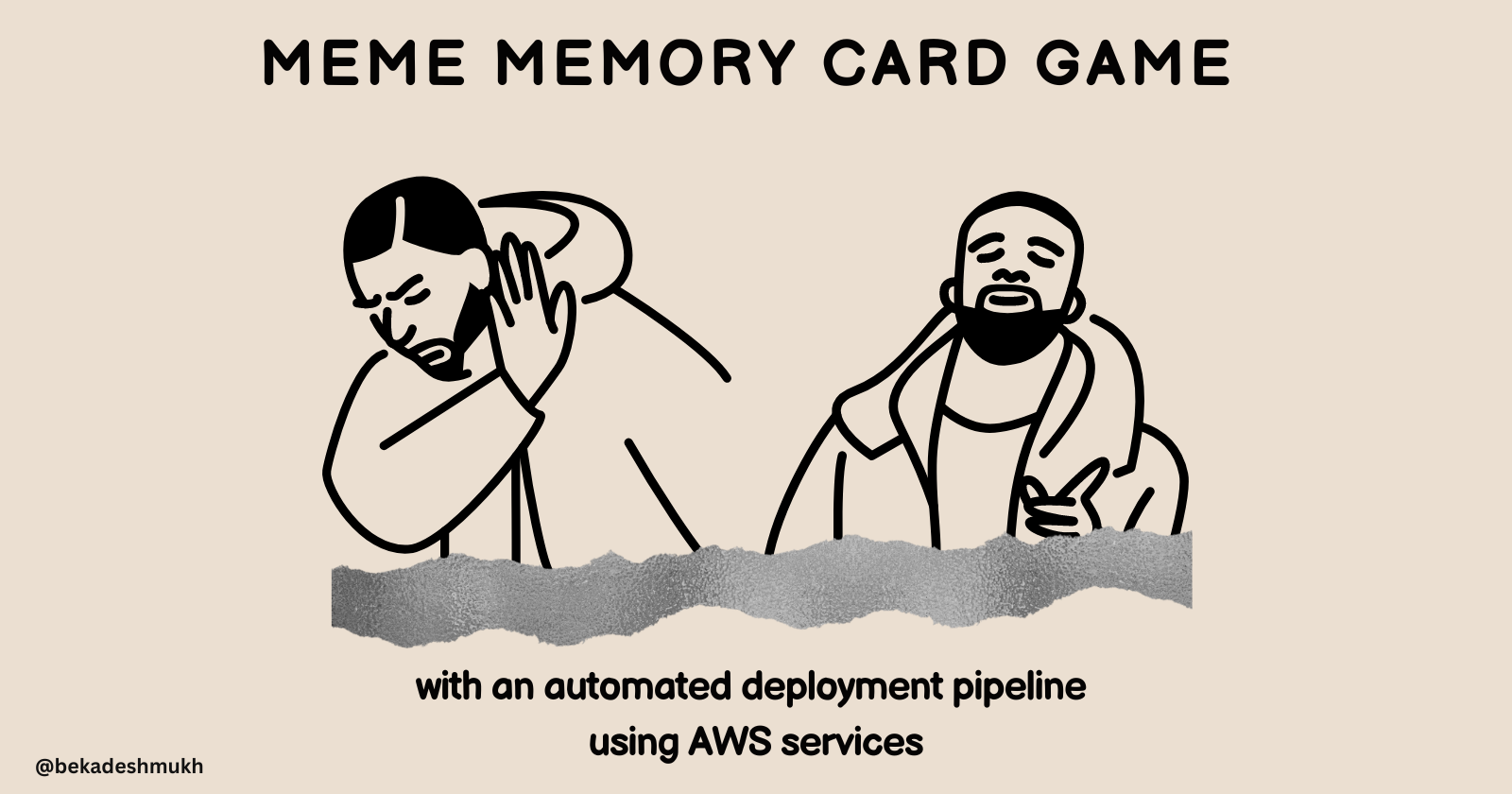
In this project, we'll create a fun memory card game using memes, and set up an automated deployment pipeline using AWS services. Here's what we'll cover:
Developing the game using HTML, CSS, and JavaScript
Setting up an S3 bucket for static website hosting
Creating a CodePipeline to deploy from GitHub to S3
Step 1: Developing the Meme Memory Card Game
Let's start by creating the game files:
Create a new directory for your project:
mkdir meme-memory-game cd meme-memory-game
Create the following files:
index.html
styles.css
script.js
Open
index.html
and add the following code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Meme Matching Game</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<h1>MEME MATCHING GAME</h1>
<p class="instructions">
Welcome to the Meme Matching Game! Flip over two cards at a time and try to find all the matching pairs. Be quick and remember where you saw the cards!
</p>
<button id="start-game">Start Game</button>
<div id="game-board" class="game-board">
<!-- Cards will be generated here -->
</div>
</div>
<script src="script.js"></script>
</body>
</html>
- Open
styles.css
and add the following code:
body {
font-family: 'Roboto', sans-serif;
background-color: #282c34;
color: #ffffff;
text-align: center;
margin: 0;
padding: 0;
}
.container {
width: auto;
margin: 40px auto;
padding: 30px;
background-color: #3c404d;
box-shadow: 0 10px 20px rgba(0, 0, 0, 0.25);
border-radius: 15px;
}
h1 {
color: white;
}
.instructions {
font-size: 20px;
margin-bottom: 30px;
color: #adbac7;
}
#game-board {
display: grid;
grid-template-columns: repeat(5, 1fr);
grid-template-rows: repeat(2, 1fr);
gap: 20px;
justify-content: center;
padding: 20px;
}
.card {
width: 211.8px;
height: 166.2px;
background-color: #565c6f;
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.2);
cursor: pointer;
display: flex;
align-items: center;
justify-content: center;
overflow: hidden;
}
.card img {
width: 100%;
height: auto;
visibility: hidden; /* Hide images by default */
display: block;
}
.card.show img {
visibility: visible; /* Show image when card is flipped */
}
#start-game {
background-color: #4caf50;
color: white;
padding: 12px 24px;
border: none;
border-radius: 5px;
cursor: pointer;
font-size: 18px;
transition: background-color 0.3s, transform 0.3s;
}
#start-game:hover {
background-color: #43a047; /* Darker on hover */
transform: scale(1.05); /* Slightly enlarges on hover */
}
Open script.js and add the following code:
document.addEventListener('DOMContentLoaded', () => {
const grid = document.querySelector('#game-board');
const startButton = document.getElementById('start-game');
let cardsChosen = [];
let cardsChosenId = [];
let cardsWon = [];
const cardArray = [
{ name: 'card1', img: 'images/distracted.png' },
{ name: 'card1', img: 'images/distracted.png' },
{ name: 'card2', img: 'images/drake.png' },
{ name: 'card2', img: 'images/drake.png' },
{ name: 'card3', img: 'images/fine.png' },
{ name: 'card3', img: 'images/fine.png' },
{ name: 'card4', img: 'images/rollsafe.png' },
{ name: 'card4', img: 'images/rollsafe.png' },
{ name: 'card5', img: 'images/success.png' },
{ name: 'card5', img: 'images/success.png' },
// ...add more pairs as needed
];
function shuffle(array) {
array.sort(() => 0.5 - Math.random());
}
function createBoard() {
shuffle(cardArray);
grid.innerHTML = '';
cardsWon = [];
for (let i = 0; i < cardArray.length; i++) {
const card = document.createElement('img');
card.setAttribute('src', 'images/blank.png');
card.setAttribute('data-id', i);
card.addEventListener('click', flipCard);
grid.appendChild(card);
}
}
function flipCard() {
let cardId = this.getAttribute('data-id');
if (!cardsChosenId.includes(cardId)) {
cardsChosen.push(cardArray[cardId].name);
cardsChosenId.push(cardId);
this.setAttribute('src', cardArray[cardId].img);
if (cardsChosen.length === 2) {
setTimeout(checkForMatch, 500);
}
}
}
function checkForMatch() {
const cards = document.querySelectorAll('#game-board img');
const firstCardId = cardsChosenId[0];
const secondCardId = cardsChosenId[1];
if (cardsChosen[0] === cardsChosen[1] && firstCardId !== secondCardId) {
cards[firstCardId].style.visibility = 'hidden';
cards[secondCardId].style.visibility = 'hidden';
cards[firstCardId].removeEventListener('click', flipCard);
cards[secondCardId].removeEventListener('click', flipCard);
cardsWon.push(cardsChosen);
} else {
cards[firstCardId].setAttribute('src', 'images/blank.png');
cards[secondCardId].setAttribute('src', 'images/blank.png');
}
cardsChosen = [];
cardsChosenId = [];
if (cardsWon.length === cardArray.length / 2) {
alert('Congratulations! You found them all!');
}
}
startButton.addEventListener('click', createBoard);
});
Now you have a basic meme memory card game! Test it locally by opening the index.html
file in your browser.
Step 2: Setting up an S3 Bucket for Static Website Hosting
Log in to the AWS Management Console and navigate to S3.
Click "Create bucket" and choose a unique name for your bucket (e.g., "meme-memory-game-yourusername").
In the "Object Ownership" section, choose "ACLs disabled".
Uncheck "Block all public access" and acknowledge the warning.
Leave other settings as default and create the bucket.
Once created, go to the bucket's "Properties" tab.
Scroll down to "Static website hosting" and click "Edit".
Choose "Enable" and set the "Index document" to "index.html".
Save the changes.
Go to the "Permissions" tab and add the following bucket policy (replace
YOUR-BUCKET-NAME
with your actual bucket name):
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "PublicReadGetObject",
"Effect": "Allow",
"Principal": "*",
"Action": "s3:GetObject",
"Resource": "arn:aws:s3:::YOUR-BUCKET-NAME/*"
}
]
}
Your S3 bucket is now set up for static website hosting.
Step 3: Creating a CodePipeline to Deploy from GitHub to S3
Navigate to AWS CodePipeline in the AWS Management Console.
Click "Create pipeline".
Choose a name for your pipeline (e.g., "meme-game-pipeline") and click "Next".
For the source provider, choose "GitHub (Version 2)" and connect your GitHub account.
Select your repository and the branch you want to deploy from.
Skip the build stage by choosing "Skip build stage" and click "Skip".
For the deploy stage, choose "Amazon S3" as the deploy provider.
Select your bucket and check "Extract file before deploy" if your repository is zipped.
Review and create the pipeline.
Now, whenever you push changes to your GitHub repository, CodePipeline will automatically deploy the updates to your S3 bucket.
Conclusion
Congratulations! You've built a meme-based memory card game and set up an automated deployment pipeline using AWS services. Your game is now live and will update automatically whenever you push changes to your GitHub repository.
To further improve your game, consider adding more features like:
A timer or move counter
Difficulty levels with more cards
Sound effects or animations
A leaderboard using AWS DynamoDB
Happy coding and meme matching!
Subscribe to my newsletter
Read articles from Beka directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
