Interact with GitHub Pull Requests using Python
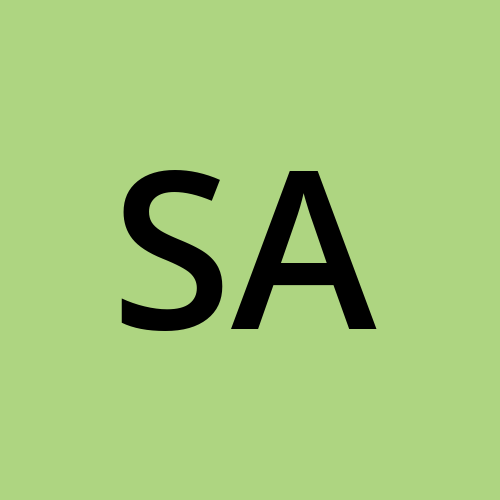
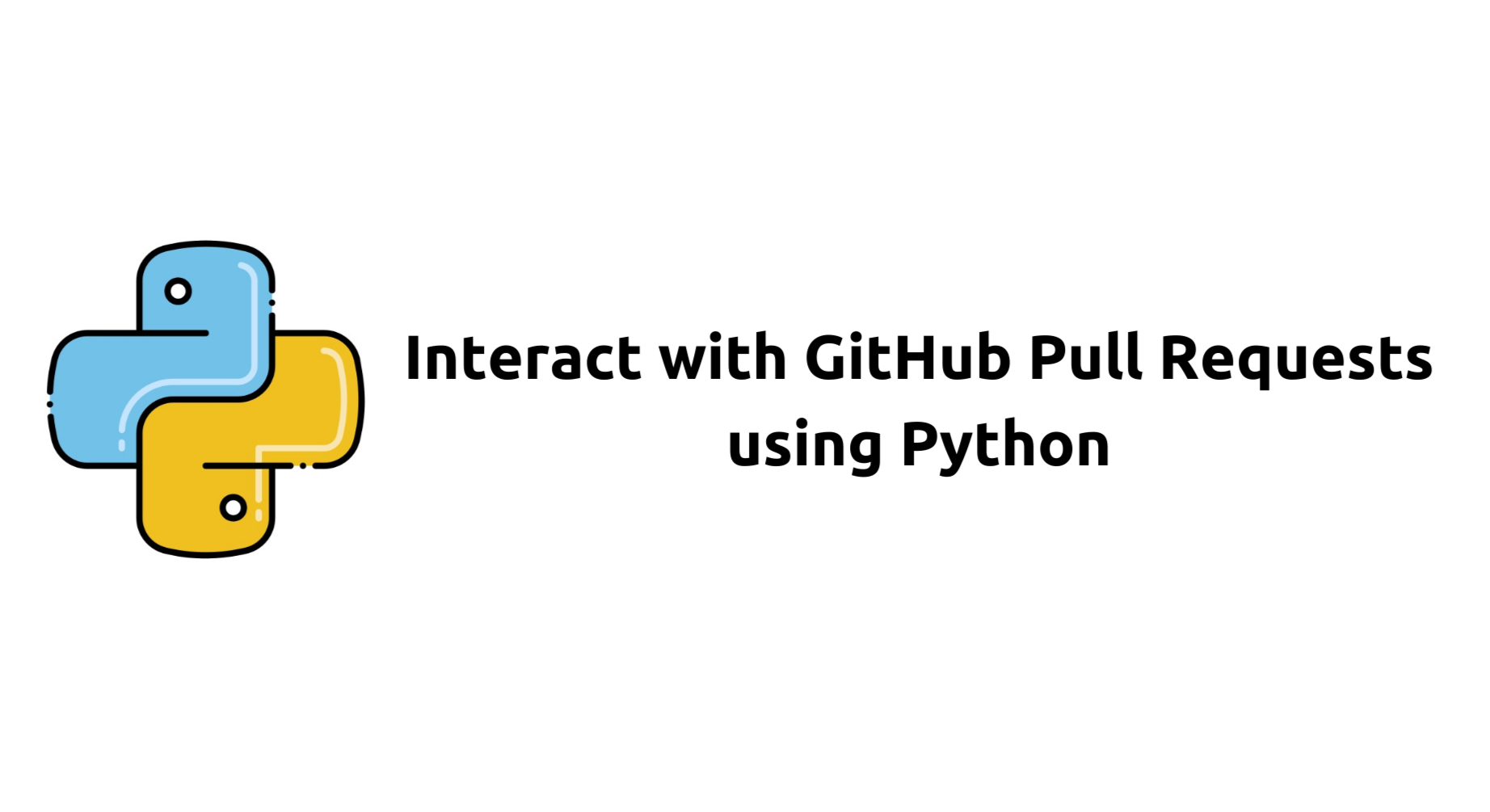
Introduction
In today's software development landscape, collaboration is key, and pull requests serve as the backbone of collaborative coding. GitHub, being one of the most popular platforms for hosting Git repositories, offers a rich API that enables developers to interact with various aspects of their repositories programmatically. In this article, we'll explore how to utilize Python along with the GitHub API to fetch and display pull requests from a repository.
Prerequisites:
Basic understanding of Python programming.
Familiarity with GitHub and pull requests.
Step 1: Setting up the Environment
Before we dive into coding, ensure you have Python installed on your system. Additionally, install the requests
library, which we'll use to make HTTP requests to the GitHub API. You can install it via pip:
pip install requests
Step 2: Fetching Pull Requests
We'll begin by defining the owner and repository name of the GitHub project we want to fetch pull requests from. For demonstration purposes, let's consider the Kubernetes repository.
import requests
owner = "kubernetes"
repo = "kubernetes"
Step 3: Making API Requests
We'll construct the URL to fetch pull requests using the GitHub API and make a GET request to retrieve the data.
url = f"https://api.github.com/repos/{owner}/{repo}/pulls"
response = requests.get(url)
Step 4: Handling the Response
We'll check the status code of the response to ensure the request was successful. If successful, we'll parse the JSON response and print information about each pull request.
if response.status_code == 200:
pull_requests = response.json()
for pr in pull_requests:
print(f"Pull Request #{pr['number']}: {pr['title']} by {pr['user']['login']}")
else:
print("Failed to fetch pull requests. Status code:", response.status_code)
Overall Code:
import requests
# GitHub repository information
owner = "kubernetes"
repo = "kubernetes"
# API endpoint for listing pull requests
url = f"https://api.github.com/repos/{owner}/{repo}/pulls"
# Make a GET request to the GitHub API
response = requests.get(url)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON response
pull_requests = response.json()
# Print information about each pull request
for pr in pull_requests:
print(f"Pull Request #{pr['number']}: {pr['title']} by {pr['user']['login']}")
else:
print("Failed to fetch pull requests. Status code:", response.status_code)
Conclusion
In this tutorial, we've demonstrated how to use Python along with the GitHub API to fetch and display pull requests from a repository. This can be immensely helpful for automating tasks related to code review, monitoring contributions, or generating reports. With the power of Python and the flexibility of the GitHub API, developers can streamline their workflow and enhance collaboration in their projects.
Further Exploration
Extend the script to include additional information about pull requests, such as their state, labels, or timestamps.
Implement authentication to access private repositories or increase API rate limits.
Explore other endpoints of the GitHub API to perform actions such as creating or merging pull requests programmatically.
Subscribe to my newsletter
Read articles from Saurabh Adhau directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
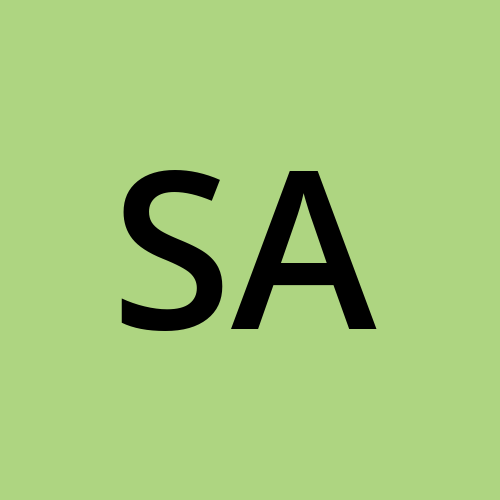
Saurabh Adhau
Saurabh Adhau
As a DevOps Engineer, I thrive in the cloud and command a vast arsenal of tools and technologies: โ๏ธ AWS and Azure Cloud: Where the sky is the limit, I ensure applications soar. ๐จ DevOps Toolbelt: Git, GitHub, GitLab โ I master them all for smooth development workflows. ๐งฑ Infrastructure as Code: Terraform and Ansible sculpt infrastructure like a masterpiece. ๐ณ Containerization: With Docker, I package applications for effortless deployment. ๐ Orchestration: Kubernetes conducts my application symphonies. ๐ Web Servers: Nginx and Apache, my trusted gatekeepers of the web.