Tokens of Appreciation: Decoding the Language of Code
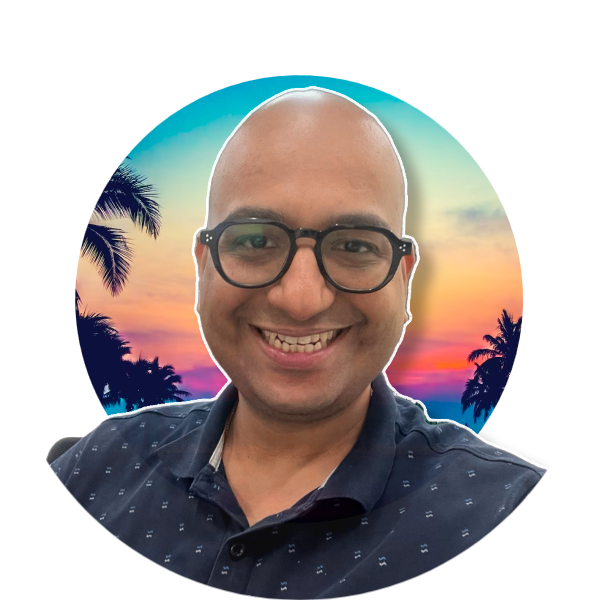
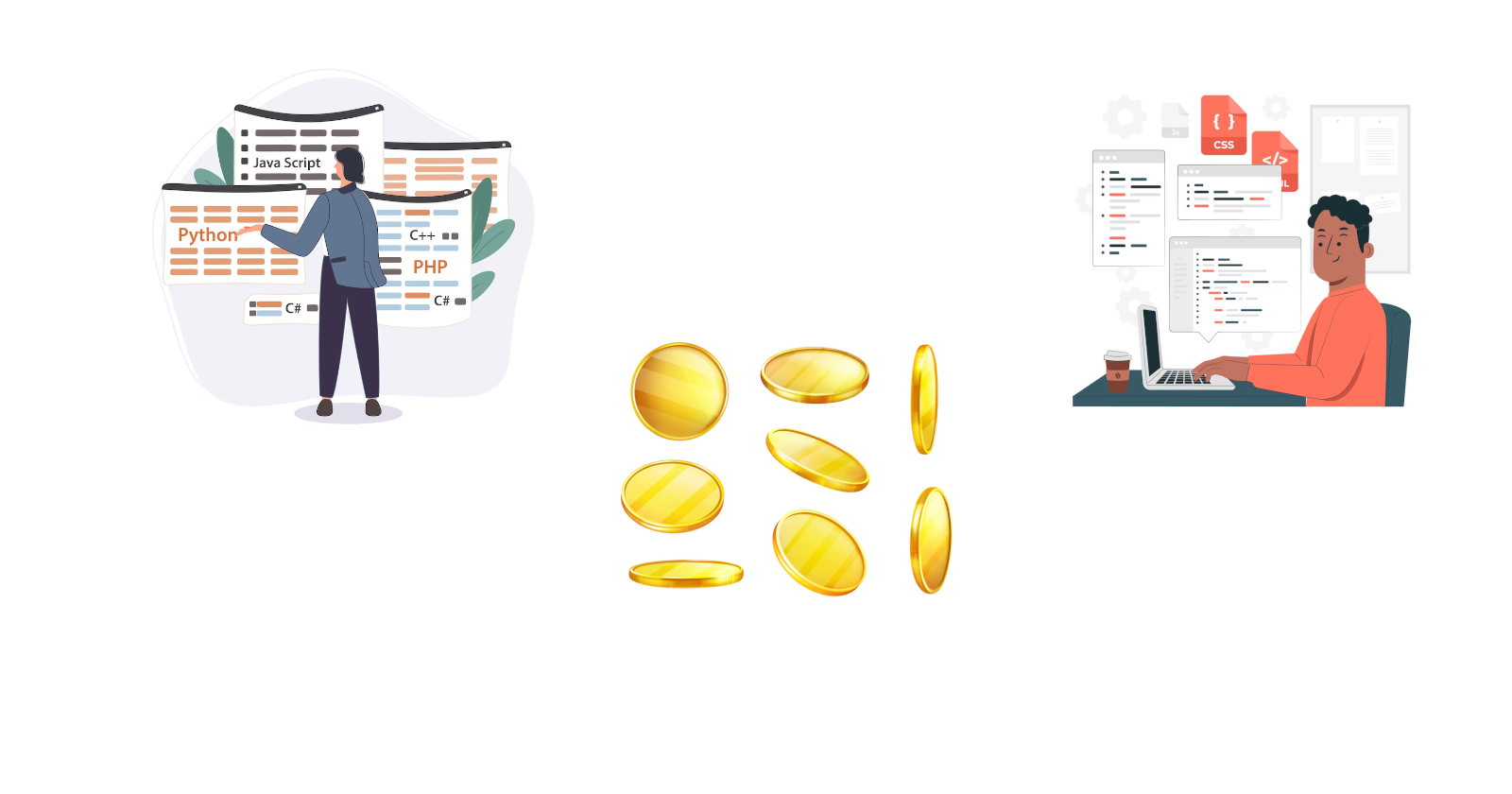
Introduction
A tokenizer, also known as a lexical analyzer, is a fundamental component in the process of creating an interpreter or compiler. It serves as the first step in transforming source code into a format that can be easily processed by subsequent stages of the interpreter. The primary function of a tokenizer is to break down the input source code into a sequence of meaningful units called tokens. These tokens represent the smallest individual elements of the programming language, such as keywords, identifiers, literals, and operators.
In the context of writing an interpreter, the tokenizer acts as a bridge between the raw text input and the parser. It simplifies the parsing process by converting the unstructured text into a structured stream of tokens, each with a specific type and value. This transformation allows the parser to work with a more manageable and meaningful representation of the source code.
Creating a tokenizer in Go involves implementing a systematic approach to recognize and categorize different elements of the programming language. By leveraging Go's powerful string manipulation capabilities and control structures, we can build an efficient and robust tokenizer that forms the foundation of our interpreter.
Example Code
package main
import (
"fmt"
"unicode"
)
type TokenType int
const (
TOKEN_ILLEGAL TokenType = iota
TOKEN_EOF
TOKEN_IDENTIFIER
TOKEN_NUMBER
TOKEN_PLUS
TOKEN_MINUS
TOKEN_ASTERISK
TOKEN_SLASH
)
type Token struct {
Type TokenType
Literal string
}
type Tokenizer struct {
input string
position int
readPosition int
ch byte
}
func NewTokenizer(input string) *Tokenizer {
t := &Tokenizer{input: input}
t.readChar()
return t
}
func (t *Tokenizer) readChar() {
if t.readPosition >= len(t.input) {
t.ch = 0
} else {
t.ch = t.input[t.readPosition]
}
t.position = t.readPosition
t.readPosition++
}
func (t *Tokenizer) NextToken() Token {
var tok Token
t.skipWhitespace()
switch t.ch {
case '+':
tok = Token{Type: TOKEN_PLUS, Literal: string(t.ch)}
case '-':
tok = Token{Type: TOKEN_MINUS, Literal: string(t.ch)}
case '*':
tok = Token{Type: TOKEN_ASTERISK, Literal: string(t.ch)}
case '/':
tok = Token{Type: TOKEN_SLASH, Literal: string(t.ch)}
case 0:
tok.Literal = ""
tok.Type = TOKEN_EOF
default:
if isLetter(t.ch) {
tok.Literal = t.readIdentifier()
tok.Type = TOKEN_IDENTIFIER
return tok
} else if isDigit(t.ch) {
tok.Literal = t.readNumber()
tok.Type = TOKEN_NUMBER
return tok
} else {
tok = Token{Type: TOKEN_ILLEGAL, Literal: string(t.ch)}
}
}
t.readChar()
return tok
}
func (t *Tokenizer) readIdentifier() string {
position := t.position
for isLetter(t.ch) {
t.readChar()
}
return t.input[position:t.position]
}
func (t *Tokenizer) readNumber() string {
position := t.position
for isDigit(t.ch) {
t.readChar()
}
return t.input[position:t.position]
}
func (t *Tokenizer) skipWhitespace() {
for t.ch == ' ' || t.ch == '\t' || t.ch == '\n' || t.ch == '\r' {
t.readChar()
}
}
func isLetter(ch byte) bool {
return 'a' <= ch && ch <= 'z' || 'A' <= ch && ch <= 'Z' || ch == '_'
}
func isDigit(ch byte) bool {
return '0' <= ch && ch <= '9'
}
func main() {
input := "x + 5 * y"
tokenizer := NewTokenizer(input)
for {
tok := tokenizer.NextToken()
fmt.Printf("%+v\n", tok)
if tok.Type == TOKEN_EOF {
break
}
}
}
Explanation
The code defines a
Tokenizer
struct that holds the input string and current position.TokenType
enum represents different types of tokens.Token
struct contains the type and literal value of each token.NextToken()
method is the core of the tokenizer, identifying and returning the next token.Helper methods like
readChar()
,readIdentifier()
, andreadNumber()
assist in token recognition.The main loop in the
main()
function demonstrates how to use the tokenizer.
Use cases
Compiler Development: Tokenizers are essential in building compilers for programming languages, breaking down source code into tokens for further processing.
Syntax Highlighting: Text editors and IDEs use tokenizers to identify different parts of code for accurate syntax highlighting.
Code Analysis Tools: Static analysis tools employ tokenizers to break down code for detecting patterns, bugs, or style violations.
Domain-Specific Languages (DSLs): When creating DSLs, tokenizers help in parsing and interpreting custom language constructs.
Natural Language Processing: In NLP, tokenizers are used to break down text into words or subwords for further linguistic analysis.
Next topics to learn
Parser implementation
Abstract Syntax Tree (AST) construction
Symbol table management
Type checking
Code generation
Takeaway
A tokenizer is a crucial component in building interpreters and compilers, serving as the first step in processing source code. By breaking down the input into meaningful tokens, it simplifies subsequent stages of interpretation or compilation. Implementing a tokenizer in Go involves creating a structured approach to recognize and categorize language elements. This process not only forms the foundation for more complex language processing tasks but also provides insights into the structure and design of programming languages. Mastering tokenizer implementation is an essential skill for anyone interested in language design, compiler construction, or developing tools for code analysis and manipulation.
Reference
I recently came across this book on Writing An Interpretter in Go, I highly recommend it!
Image attribution
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
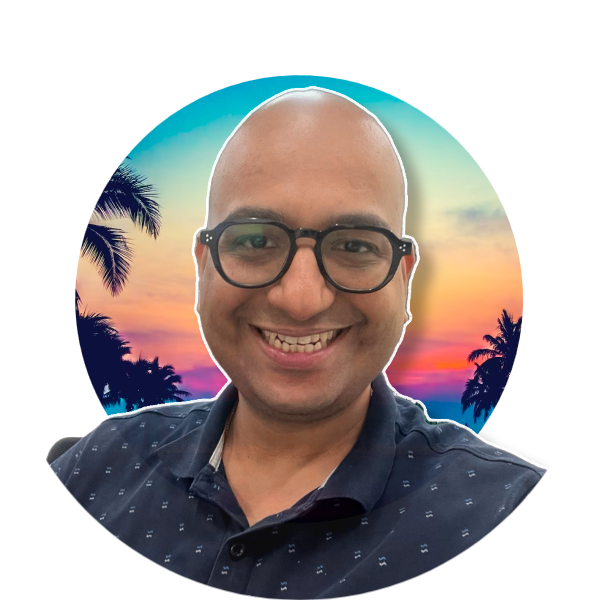
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.