Integrating Sitecore Next.js with Ninetailed
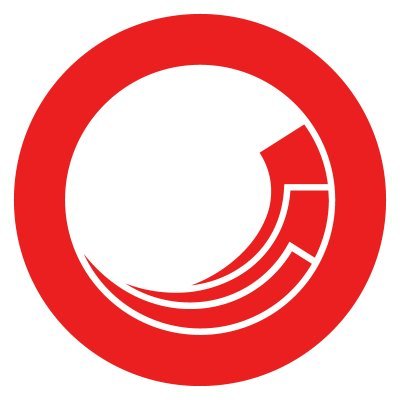
Integrating Sitecore Next.js with Ninetailed involves a few key steps to set up both the Sitecore and Ninetailed environments and then connect them using the Next.js framework. Ninetailed is a personalization and experimentation tool that allows you to deliver personalized experiences to your users. Here's a step-by-step guide to achieve this integration:
1. Set Up Your Sitecore Environment
Ensure you have a working Sitecore environment with Sitecore Headless Services and Sitecore Next.js SDK installed. Your environment should be configured to support Next.js applications.
2. Install Next.js and Ninetailed Packages
In your Next.js project, install the required Ninetailed packages:
npm install @ninetailed/experience.js @ninetailed/nextjs
3. Configure Ninetailed in Your Next.js App
In your Next.js application, you will need to initialize Ninetailed to connect your application to the Ninetailed API.
Create a new file (e.g., ninetailed.config.js
) in your project root and set up the Ninetailed client:
import { Ninetailed } from '@ninetailed/experience.js';
const ninetailed = new Ninetailed({
clientId: process.env.NINETAILED_CLIENT_ID, // Your Ninetailed client ID
apiKey: process.env.NINETAILED_API_KEY, // Your Ninetailed API key
});
export default ninetailed;
Ensure you have the environment variables NINETAILED_CLIENT_ID
and NINETAILED_API_KEY
set up in your .env.local
file or your hosting environment.
4. Integrate Ninetailed with Sitecore Content
To leverage personalization from Ninetailed in your Next.js application, you’ll need to fetch data from Sitecore and use Ninetailed to manage personalization.
Modify your data fetching methods (such as getStaticProps
or getServerSideProps
) to include Ninetailed data. For example:
import ninetailed from '../ninetailed.config';
export async function getStaticProps(context) {
// Fetch data from Sitecore using Sitecore SDK or HTTP request
const sitecoreData = await fetchSitecoreData(context.params.slug);
// Fetch personalization data from Ninetailed
const experienceData = await ninetailed.getExperience();
return {
props: {
sitecoreData,
experienceData,
},
};
}
5. Implement Personalized Components
Use the Ninetailed data to personalize components in your Next.js pages. Create a personalized component that checks the Ninetailed experience data and renders content accordingly:
import { useExperience } from '@ninetailed/nextjs';
const PersonalizedComponent = ({ sitecoreData, experienceData }) => {
const { experience } = useExperience(experienceData);
const personalizedContent = experience.isPersonalized
? experience.variant // Render variant content
: sitecoreData.defaultContent; // Render default content
return <div>{personalizedContent}</div>;
};
export default PersonalizedComponent;
6. Enable and Configure Personalization in Sitecore
Ensure that the Sitecore components or placeholders you want to personalize are enabled for personalization. Set up rules and conditions in Sitecore for Ninetailed to work with.
7. Deploy Your Next.js Application
Deploy your Next.js application to your hosting environment (e.g., Vercel, Netlify, or any other hosting service). Ensure all environment variables and configurations for Sitecore and Ninetailed are correctly set up in the production environment.
8. Test and Validate Integration
Test Personalized Content: Ensure that the content personalized through Ninetailed is rendered correctly based on the conditions set up in your Ninetailed dashboard.
Check Sitecore Data Rendering: Make sure the default and variant contents are fetched from Sitecore and displayed as expected.
9. Monitor and Optimize
Use the analytics tools provided by Ninetailed to monitor the performance and effectiveness of your personalized experiences. Adjust and optimize your personalization rules and content as needed.
Conclusion
By following these steps, you can successfully integrate Sitecore Next.js with Ninetailed, allowing you to deliver personalized and dynamic experiences to your users. Make sure to regularly update both your Sitecore environment and Ninetailed configuration to leverage new features and maintain a smooth integration.
Subscribe to my newsletter
Read articles from Sandeep Bhatia directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
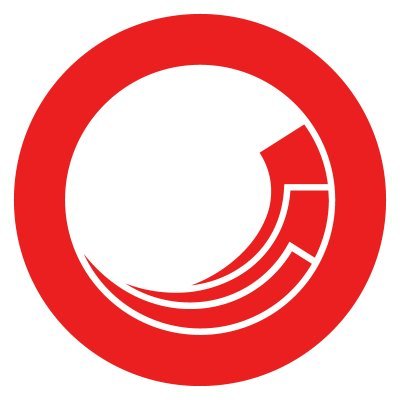
Sandeep Bhatia
Sandeep Bhatia
I am a developer from India.