Solidity Development I

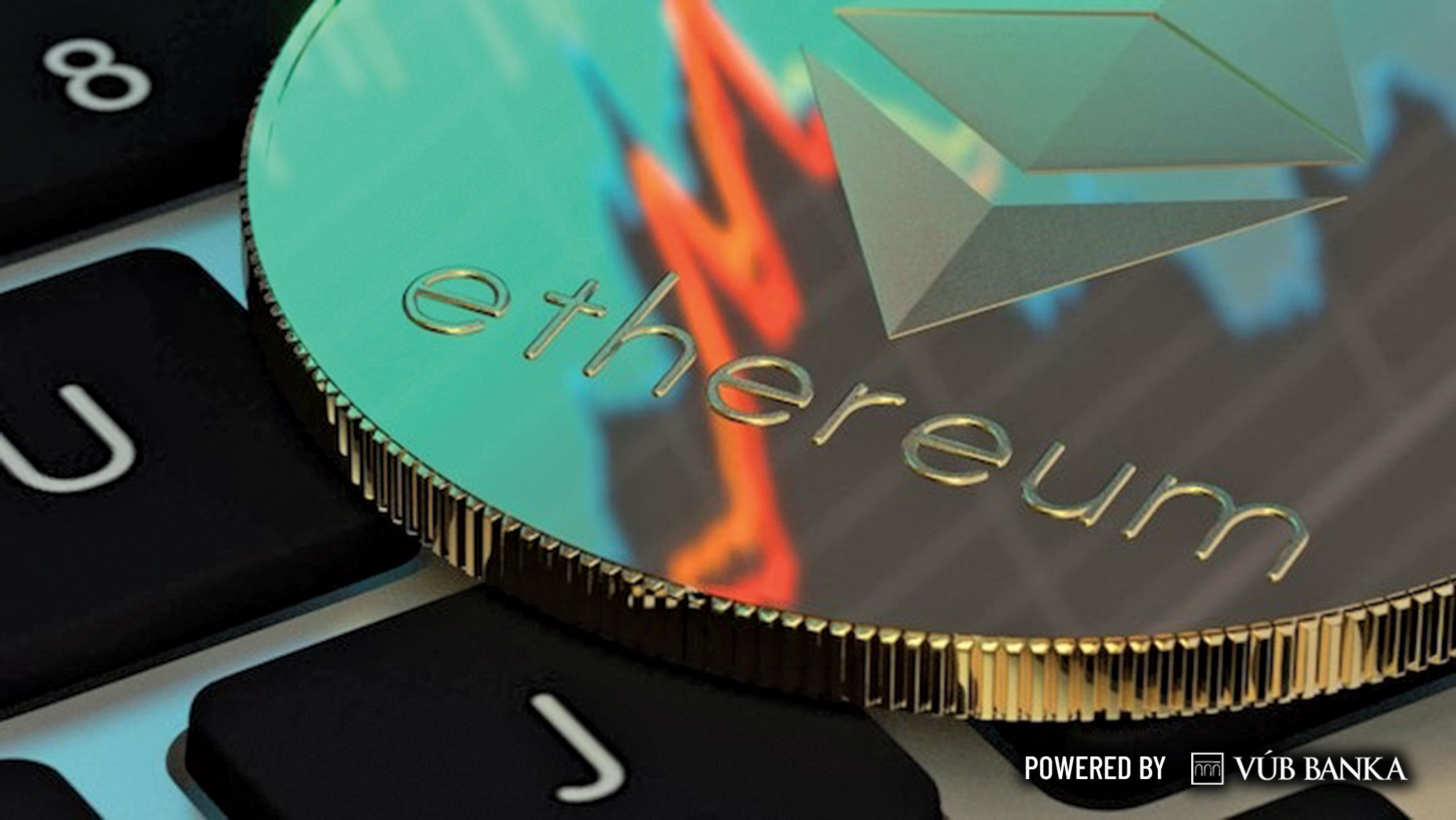
This guide is to help enthusiasts in the blockchain ecosystem learn all about solidity development.
Prerequisites;
What is Base.
What is Solidity.
What are Smart contracts.
Writing a Simple Storage smart contract.
Deployment on Base RPC.
In this article, we will explore various ways to get involved in the Base blockchain ecosystem, whether you are new to web3 or a seasoned enthusiast of decentralized networks. The Base blockchain community embodies innovation, inclusivity, and collaboration, making it an ideal platform for participants of all backgrounds. From its developer-friendly environment to its vibrant community initiatives, Base provides multiple avenues for contributing to the evolution of scalable and secure blockchain solutions. Whether you are interested in building decentralized applications (dApps), simply learning more about blockchain technology, the Base ecosystem offers a supportive space for everyone to play a role in the next phase of blockchain development.
WHAT IS BASE?
Base is an Ethereum Layer 2 (L2) network built on the OP Stack, which is the open-source software developed by Optimism. It aims to offer a secure, low-cost, and developer-friendly environment for building decentralized applications (dApps). Base is designed to leverage Ethereum's security while providing scalability and lower transaction costs, making it an attractive platform for developers who want to build on Ethereum without the associated high gas fees and slower transaction speeds.
Technology Stack and Architecture
Base is built on the Ethereum blockchain as a Layer 2 solution, which means it operates on top of the Ethereum network, providing scalability while maintaining security and decentralization. Base utilizes Optimism's OP Stack, a modular framework that allows for easy integration and scalability on the Ethereum network. This framework helps in reducing gas fees and improving transaction throughput.
Key Features.
Scalability
Base is built to handle a higher volume of transactions compared to the Ethereum mainnet, thanks to its Layer 2 architecture. This allows for faster and cheaper transactions, which is ideal for dApps that require high throughput, such as gaming, decentralized finance (DeFi), and social media platforms.
Security
As a Layer 2 network, Base inherits the security properties of Ethereum. This means that while transactions are processed on Base, they are ultimately settled on Ethereum, benefiting from its robust security and decentralization.
EVM Compatibility
Base is fully compatible with the Ethereum Virtual Machine (EVM), which allows developers to easily deploy their existing Ethereum dApps on Base without significant modifications. This compatibility facilitates seamless migration and integration for projects already operating on Ethereum.
Integration with Coinbase
Base is incubated by Coinbase, a leading cryptocurrency exchange, which provides additional benefits such as access to Coinbase's user base and potential integrations with its suite of products. This affiliation could help drive adoption and trust in the Base network.
WHAT IS SOLIDITY?
Solidity is an object-oriented programming language created specifically by the Ethereum Network team for constructing and designing smart contracts on Blockchain platforms.
It’s used to create smart contracts that implement business logic and generate a chain of transaction records in the blockchain system.
It acts as a tool for creating machine-level code and compiling it on the Ethereum Virtual Machine (EVM).
It has a lot of similarities with C and C++ and is pretty simple to learn and understand. For example, a “main” in C is equivalent to a “contract” in Solidity.
Like other programming languages, Solidity programming also has variables, functions, classes, arithmetic operations, string manipulation, and many other concepts. We can’t also ignore the fact that Solidity is a high-level programming language specifically designed for writing smart contracts on blockchain platforms, with Ethereum being the most prominent example.
A smart contract is a self-executing contract with the terms of the agreement directly written into code. Solidity allows developers to create these contracts by defining rules, conditions, and actions that automatically execute on the blockchain once triggered by predefined events.
Key features of Solidity include:
Smart Contracts: Solidity is primarily used for creating smart contracts. These contracts can automate complex processes, manage assets, and enforce rules without the need for intermediaries.
Decentralized Applications (DApps): Solidity is used to build the logic behind decentralized applications. DApps are applications that run on a blockchain network and have the advantage of transparency, immutability, and security.
Turing Complete: Solidity is a Turing complete language, which means it can handle any computation that can be expressed algorithmically. This allows developers to create a wide range of applications and logic on the blockchain.
Contract-Oriented: Solidity is designed around the concept of contract-oriented programming, where the code is organized into contracts, each with its own data and functions. Contracts can interact with each other, enabling complex systems of interrelated contracts.
Security: Solidity includes features to help developers write secure code, such as visibility specifiers for functions (public, internal, external, private), events for logging important information, and modifiers for implementing common checks.
Data Types: Solidity supports various data types including integers, strings, booleans, addresses, mappings, arrays, structs, and more. This allows developers to manage different types of data within their contracts.
Inheritance: Solidity supports inheritance, allowing developers to create modular and reusable code. Contracts can inherit properties and methods from other contracts, promoting code reusability.
Gas Fees: In Ethereum and other blockchain networks, executing code costs gas, a unit of measure for computational work. Solidity developers need to be mindful of the gas costs of their operations to optimize the efficiency and cost-effectiveness of their smart contracts.
Development Tools: Solidity has an ecosystem of development tools, such as compilers, integrated development environments (IDEs), testing frameworks, and debuggers, to facilitate the development, testing, and deployment of smart contracts.
Solidity has an ecosystem of development tools, such as compilers, integrated development environments (IDEs), testing frameworks, and debuggers, to facilitate the development, testing, and deployment of smart contracts.
WHAT ARE SMART CONTRACTS?
Solidity smart contract codes are like computer programs that run on a blockchain. A blockchain is a special type of digital ledger that records transactions and data in a secure, transparent, and immutable manner. Smart contracts are stored on the blockchain, and their code is executed automatically when certain conditions are met.
WRITING A SIMPLE STORAGE SMART CONTRACT.
A simple storage smart contract is a basic type of smart contract that is commonly used to demonstrate the fundamental concepts of blockchain and smart contract development. It serves as a starting point for understanding how data can be stored and manipulated on a blockchain platform like Ethereum.
At its core, a simple storage smart contract allows you to store a single value on the blockchain and provides methods to read and update that value. Below is an example of a simple storage smart contract and a detailed explanation of each variables, functions and data.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.24;
contract SimpleStorage {
uint256 private storedData;
event ValueSet(address indexed sender, uint256 newValue);
event ValueIncremented(address indexed sender, uint256 incrementValue);
event ValueDecremented(address indexed sender, uint256 decrementValue);
event ValueMultiplied(address indexed sender, uint256 factor);
event ValueDivided(address indexed sender, uint256 divisor);
event ValueReset(address indexed sender);
event ValueSquared(address indexed sender, uint256 squaredValue);
// Function to set the stored data
function set(uint256 newValue) public {
storedData = newValue;
emit ValueSet(msg.sender, newValue);
}
// Function to get the stored data
function get() public view returns (uint256) {
return storedData;
}
// Function to increment the stored data by a certain value
function increment(uint256 value) public {
storedData += value;
}
// Function to decrement the stored data by a certain value
function decrement(uint256 value) public {
storedData -= value;
}
// Function to multiply the stored data by a factor
function multiply(uint256 factor) public {
storedData *= factor;
emit ValueMultiplied(msg.sender, factor);
}
// Function to divide the stored data by a divisor
function divide(uint256 divisor) public {
require(divisor != 0, "Divisor cannot be zero");
storedData /= divisor;
}
// Function to reset the stored data to zero
function reset() public {
storedData = 0;
emit ValueReset(msg.sender);
}
// Function to get the square root of the stored data
function sqrt() public view returns (uint256) {
return sqrt(storedData);
}
// Internal function to calculate the square root using Newton's method
function sqrt(uint256 x) internal pure returns (uint256 y) {
if (x == 0) return 0;
else if (x <= 3) return 1;
y = x;
uint256 z = (y + x / y) / 2;
while (z < y) {
y = z;
z = (y + x / y) / 2;
}
}
}
Let’s break down the above smart contract code step by step so you can fully understand it.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.24;
This line specifies the license under which the code is released (MIT license).
pragma solidity ^0.8.24;
sets the version of the Solidity programming language that the code is compatible with. In this case, it's version 0.8.0.
contract SimpleStorage {
uint256 private storedData;
contract SimpleStorage
defines the start of a new smart contract named "SimpleStorage."uint256 private storedData;
declares a private variable calledstoredData
of typeuint256
(unsigned integer of 256 bits) to hold the stored value. The variable is marked as private, meaning it can only be accessed within the contract itself.
event ValueSet(address indexed sender, uint256 newValue);
event ValueIncremented(address indexed sender, uint256 incrementValue);
event ValueDecremented(address indexed sender, uint256 decrementValue);
event ValueMultiplied(address indexed sender, uint256 factor);
event ValueDivided(address indexed sender, uint256 divisor);
event ValueReset(address indexed sender);
event ValueSquared(address indexed sender, uint256 squaredValue);
These lines define a series of events. An event is a way to log and notify external observers about specific actions happening within the contract.
Events provide transparency and allow external parties to track contract interactions.
function set(uint256 newValue) public {
storedData = newValue;
emit ValueSet(msg.sender, newValue);
}
function set(uint256 newValue) public
defines a public function namedset
that takes anewValue
of typeuint256
as an argument.Inside the function,
storedData
is updated with the new value, and then theValueSet
event is emitted with the sender's address (msg.sender
) and the new value.
function get() public view returns (uint256) {
return storedData;
}
function get() public view returns (uint256)
defines a public view function namedget
.This function does not modify the contract's state and can be called by anyone. It returns the current value of
storedData
.
function increment(uint256 value) public {
storedData += value;
}
function increment(uint256 value) public
defines a public function namedincrement
that takes avalue
of typeuint256
as an argument.The function adds the
value
to the current value ofstoredData
.
function decrement(uint256 value) public {
storedData -= value;
}
function decrement(uint256 value) public
defines a public function nameddecrement
that takes avalue
of typeuint256
as an argument.The function subtracts the
value
from the current value ofstoredData
.
function multiply(uint256 factor) public {
storedData *= factor;
emit ValueMultiplied(msg.sender, factor);
}
function multiply(uint256 factor) public
defines a public function namedmultiply
that takes afactor
of typeuint256
as an argument.The function multiplies the current value of
storedData
by the providedfactor
, and then emits theValueMultiplied
event.
function divide(uint256 divisor) public {
require(divisor != 0, "Divisor cannot be zero");
storedData /= divisor;
}
function divide(uint256 divisor) public
defines a public function nameddivide
that takes adivisor
of typeuint256
as an argument.The function divides the current value of
storedData
by the provideddivisor
, but it also includes arequire
statement to ensure thedivisor
is not zero.
function reset() public {
storedData = 0;
emit ValueReset(msg.sender);
}
function reset() public
defines a public function namedreset
.The function sets the value of
storedData
to zero and emits theValueReset
event.
function sqrt() public view returns (uint256) {
return sqrt(storedData);
}
function sqrt() public view returns (uint256)
defines a public view function namedsqrt
.This function returns the square root of the current value of
storedData
by calling the internalsqrt(uint256 x)
function.
function sqrt(uint256 x) internal pure returns (uint256 y) {
if (x == 0) return 0;
else if (x <= 3) return 1;
y = x;
uint256 z = (y + x / y) / 2;
while (z < y) {
y = z;
z = (y + x / y) / 2;
}
}
}
This section defines an internal pure function named
sqrt(uint256 x)
for calculating the square root using Newton's method.The function iteratively refines an estimate
z
of the square root until it converges to the accurate value.The function is
internal
which means it can only be used within the contract itself and ispure
as it doesn't modify state.
Below is the code on an IDE.
DEPLOYMENT ON BASE.
Navigate to your metamask and add Base Sepolia Testnet to the list of networks. Click this to get some gas fees for deployment on base testnet.
After this, you should have been rewarded with 0.1Base Sepolia ETH!
Navigate back to your wallet.
Next is to connect your wallet to remix for smooth deployment.
Check for the address.
And verify on Base Sepolia Etherscan. Paste Address.
Conclusion
Congratulations on successfully creating your very own simple storage smart contract on the Base network! .
Subscribe to my newsletter
Read articles from Adeola Aregbesola directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
