Basics of Naming Conventions for PHP Development
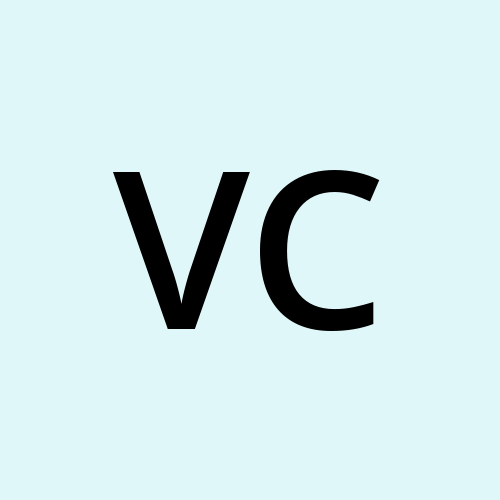
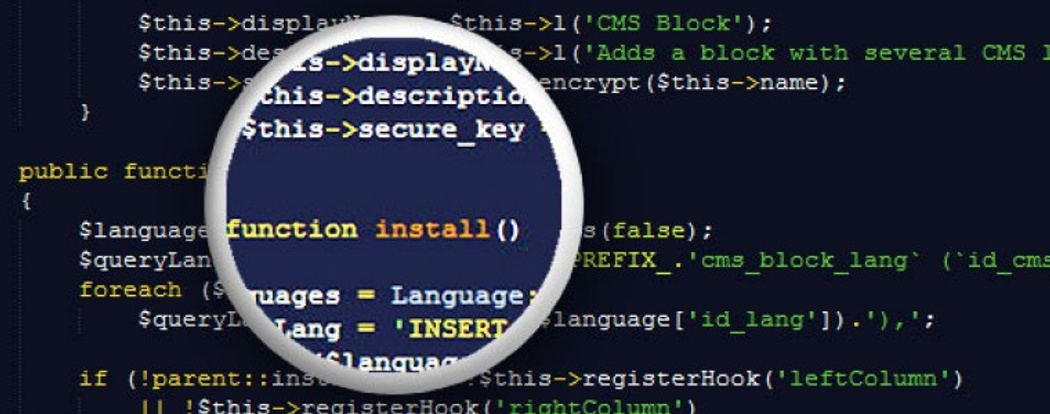
In web development, keeping a definite naming convention is a tough job. Every developer follows the practices he feels are convenient, and you may even identify the code written by a particular developer. In the field of web development, we call it the DNA of the code. But as you know, web development is not meant for a single person’s job; there is always a team effort somewhere down the line. So it becomes necessary that you follow a specific set of naming conventions in your project or even on the whole agency level so that every team member can easily grasp the inners of code by simply looking at a small chunk of code and take over the further development tasks if, in any case, you need to switch developers. I have seen developers feel hesitant to touch the code of peer members just because of the differences in naming conventions.
This article highlights how to make your code beautiful and manageable by following a small set of conventions. For convenience, we’ll use PHP as the example language because it is the most popular web development language out there, and a lot of newbies easily mess up their code by not following conventions.
Note: Here we are covering conventions in an MVC design pattern, and it should be easily followed by an intermediate-level developer.
Variables:
First of all, what is a variable? A variable is what stores data. Data can be of any type, be it a string, integer, floating point value, boolean, or array. I’d even go further to say that we should follow the same conventions when defining array keys and database field names as well. It improves consistency across our whole development stack.
$first_name = “John”; // Right
$last_name = “Doe”; // Right
$n1 = “John”; // Wrong
$n2 = “Doe”; // Wrong
$my_name = “John Doe”; // Wrong
$my_var = “John Doe”; // Wrong
So can you guess what’s wrong with $n1, $n2, $my_name, and $my_var? One word: “ambiguity.”. Ambiguous names are the biggest reason for unreadable and ugly code. So what is “ambiguity”? It’s simply naming a variable in a way that you can’t guess what exactly it stores. Consider, for example, the following snippet:
$i = “Apple iPhone 6S (32GB, Rose Gold)”;
$p = “749.00”;
$t = “749.00”;
Can you guess in the above code what $t is? No? Okay, let’s try the following code:
$item = “Apple iPhone 6S (32GB, Rose Gold)”;
$price = “749.00”;
$total = “749.00”;
Can you guess now? Exactly! This is how you differentiate ambiguous variables from absolute variables. The latter approach is better because it is definitive.
Constants:
Constants, as the name implies, are meant to store values that don’t change throughout the program. So what kind of values are supposed to be constants? They are database credentials, API keys, and mathematical constants as well. There are two types of constants you can find in the PHP language: global and class-based.
Global constants can be defined in a common file, which is included in each request of our program.
define(‘DB_HOST’, ‘localhost’);
define(‘DB_USER’, ‘db_user’);
define(‘DB_PASS’, ‘password$%@#’);
define(‘DB_NAME’, ‘db_app’);
See how we’ve kept the names of constants in all caps? This is to easily differentiate between constants and variables in a program.
Note: PHP constants don’t use the $ prefix as the variables do.
Now an example of class constants:
class Circle{
const PI = 3.14;
public function circumference($radius){
return 2 * self::PI * $radius;
}
public function area($radius){
return self::PI * $radius * $radius;
}
}
When you need to use a class constant inside the class itself, you use self::CONSTANT_NAME but to use a constant outside the class, you can simply call it by replacing self with the name of the class, e.g. Circle::PI
Class constants are targeted for contained libraries, such as when integrating multiple 3rd-party APIs, since a library can have all its constants inside its classes so that they don’t get conflicted with the global constants. So suppose a class decides to use PI up to 6 precisions, but you are using PI up to 2 precisions, You can achieve this simply by making a global PI constant and one class-specific constant. Easy, huh! ( I know this is a stupid example, in the real world, you’d never need to use 2 versions of PI.)
Functions:
A function is a piece of code that does a specific task. Each programming language consists of a lot of functions that do different tasks. So what conventions are mostly used in functions?
There are two of them most commonly used: lowercase with underscore separators and camelcase.
Consider the following two examples:
function get_name(){
// Do something
}
function getName(){
// Do something
}
So which one is best? I’d say none, both are equally good, but we prefer to use the camel-case convention because for variables, I prefer the underscore separations, and thus using camel-case for functions gives a visual ease of differentiating between a function and a variable.
But (yeah, there is a but), always keep in mind that a function should always start with a “verb,” which defines what that function is trying to do in conjunction with the name of the “entity” being affected by this function.
getName() // Right
namesList() // Wrong
listNames() // Right
sendEmail() // Right
deleteUserById($id) // Right
connectToDatabase() // Right
databaseConnection() // Wrong
prepareOutput() // Right
See, how the above examples consist of “verb” and “entity” in the functions and which ways are correct.
This covers the global/procedural functions, what about “class methods”? For those, who don’t know what a "class method” is, it is simply a function inside a class—we'll cover it in greater detail in our upcoming articles. Here is how you handle the naming convention for class methods:
class User{
public function delete($id){
// Do something
}
}
$user = new User();
$user->delete(2); // Delete the user with ID = 2
So I’m contradicting myself for “class methods," right? Yes, there is a logical explanation for this. When you use a class, a class itself represents an “entity” of our program, so suffixing the “entity” in a function name doesn’t make sense because it becomes self-explanatory in the case of class methods.
Note: An “entity” is what a program is composed of. It is a universal term and is applicable across all kinds of programs. A blog website has the following entities: articles, authors, topics, and tags, whereas an e-commerce platform consists of the following entities: products, categories, orders, manufacturers, and customers.
Classes:
Each class should be declared in its file. Class names must be in "StudlyCaps.”
class ModelUser{
}
Private and protected properties in a class MUST BE prefixed with a single underscore, for example:
class Circle{
public PI = 3.14;
private $_radius;
private function _calculateArea(){
return self::PI * $_radius * $_radius;
}
}
Parentheses:
Parenthesis is important to follow correctly. There are only two conventions for parenthesis that are widely used these days. You can read more about all the styles in existence here:
K&R Style Parentheses and Allman Parentheses.
K&R Style Parentheses:
if($a == 1){
// do something
}
Allman Parentheses:
if($a == 1){
// do something
}
We prefer to use K&R Style parentheses because they save one line and seem natural. But a lot of people prefer Allman parentheses. It doesn’t matter which one you prefer; they both are equally good, What matters is ensuring that you stick to the one you prefer.
Epilogue:
The key to following conventions is consistency. You MUST ensure that you stick to a particular set of conventions. A lot of agencies have developed their coding guidelines, and you can follow the ones we follow here at Hashbyte. If you wish, you can mix and mingle to create yourself a new set of conventions.
Happy Coding!
Subscribe to my newsletter
Read articles from Veenit Chauhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
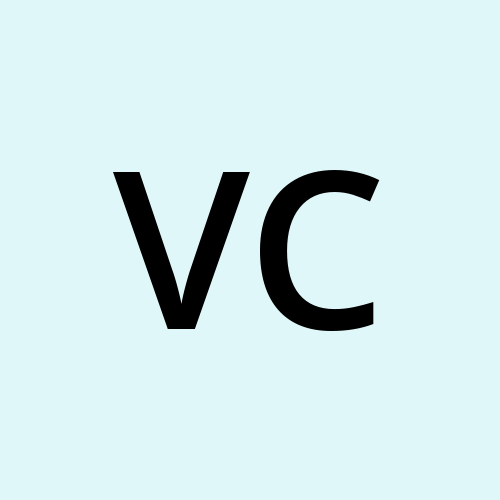