Conditionals and Loops

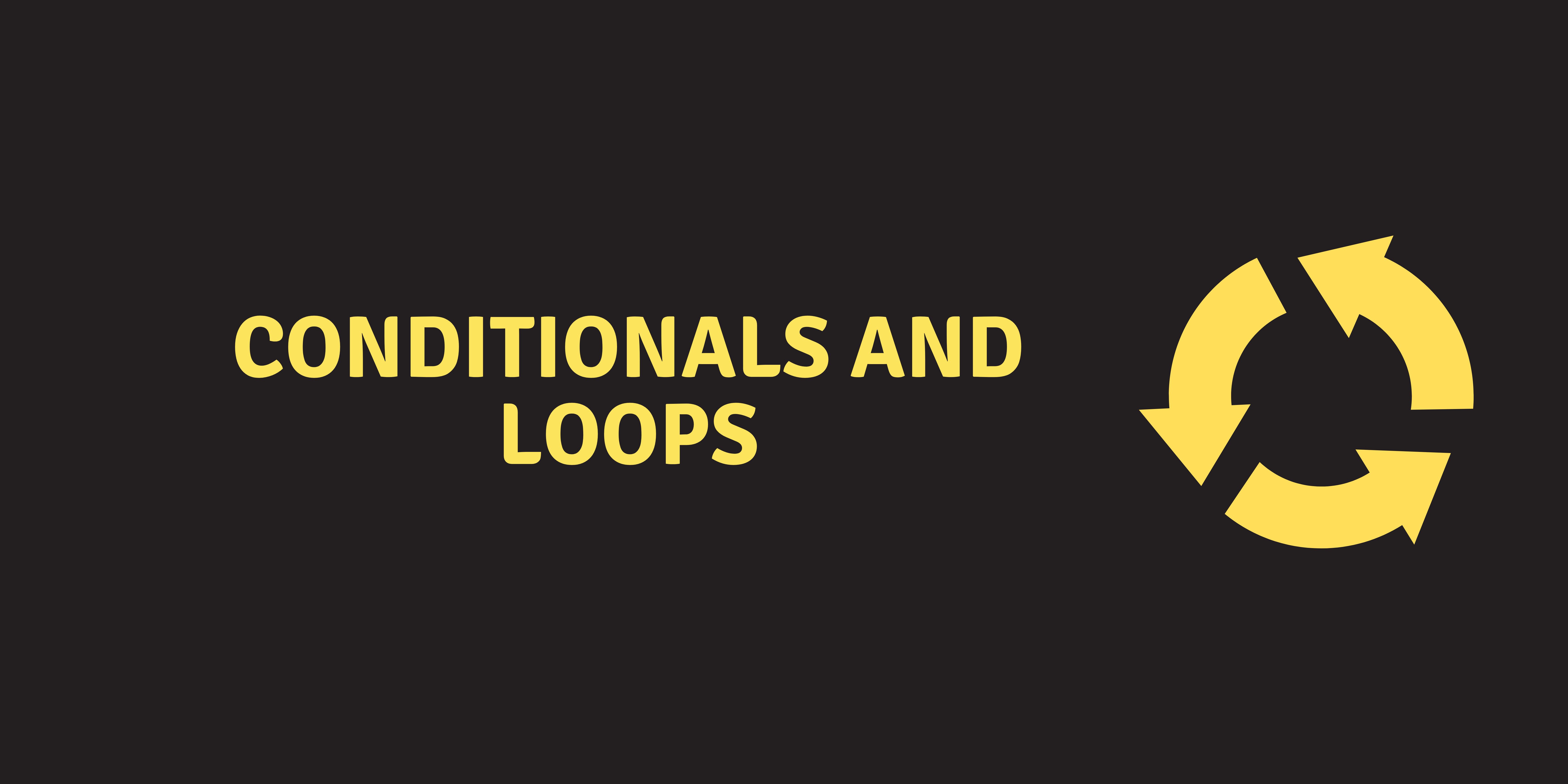
Condition: It provides check for the statement.
- if-else statement:- It is used to check the condition, it checks the boolean condition True or false.
Syntax:-
if (boolean expression True or false) {
//Body
} else {
//Do this
}
Example:-
public class IfElse {
public static void main(String[] args) {
int salary = 20000;
if (salary > 10000) {
salary = salary + 2000;
} else {
salary = salary + 1000;
}
System.out.println(salary);
}
}
//Output
22000
- Multiple if-else statement:- It executes one condition from multiple statements.
Syntax:-
if (condition 1) {
// code to be executed if condition 1 is true
} else if (condition 2) {
// code to be executed if condition 2 is true;
} else if (condition 3) {
// code to be executed if condition 3 is true;
} else {
// code to be executed if all conditions are false;
}
Example:-
public class MultipleIfElse {
public static void main(String[] args) {
int salary = 20000;
if (salary <= 10000) {
salary = salary + 1000;
} else if (salary <= 20000) {
salary = salary + 2000;
} else {
salary = salary + 3000;
}
System.out.println(salary);
}
}
//Output
25000
Loop:- Loops are used to iterate a part of program several times.
- for loop:- It is generally used when we know how many times loop will iterate.
Syntax:-
for (initialization; condition; increment/decrement) {
//body
}
Example:- Print numbers from 1 to 5.
public class ForLoop {
public static void main(String[] args) {
for (int num = 1; num <= 5; num++) {
System.out.println(num);
}
}
}
//Output
1
2
3
4
5
- While Loop:- It is used when we don't know how many time the loop will iterate.
Syntax:-
while (condition) {
//code to be executed
// increment/decrement
}
Example:-
public class whileloop {
public static void main(String[] args) {
int num = 1;
while (num <= 5) {
System.out.println(num);
num = num + 1;
}
}
}
//Output
1
2
3
4
5
- do while loop:- It is used when we want to execute our statement at least one time. It is called exit control loop because it checks the condition after execution of statement.
Syntax:-
do {
// code to be executed
// update statement -> increment/decrement
} while (condition);
Example:-
public class DoWhileLoop {
public static void main(String[] args) {
int n = 1;
do {
System.out.println(n);
n++;
} while (n <= 5);
}
}
//Output
1
2
3
4
5
While Loop | Do while loop |
Used when no of iteration is not fixed | Used when we want to execute the statement at least ones |
Entry controlled loop | Exit controlled loop |
No semicolon required at the end of while (condition) | Semicolon is required at the end of while (condition) |
Switch statement:- A switch is a multiple-choice decision-making statement.
Syntax:-
switch (expression) {
case one:
// Code block
break;//---> terminate the sequence
case two:
// Code block
break;
// Add more cases as needed
default://---> default will execute when none of the above does
// Code block
}
- If break is not used then it will continue with other cases.
public class SwitchWithoutBreak {
public static void main(String[] args) {
int choice = 2;
switch (choice) {
case 1:
System.out.println("Case 1");
// No break, falls through to the next case
case 2:
System.out.println("Case 2");
// No break, falls through to the next case
case 3:
System.out.println("Case 3");
// No break, falls through to the next case
default:
System.out.println("Default Case");
// No break needed at the end
}
}
}
- Duplicate cases are not allowed.
code one:
//code block
break;
code one://----> Duplicate cases not allowed
//code block
break;
Nested Switch case: Nested-Switch statements refer to Switch statements inside of another Switch Statements.
switch (expression) {
case one:
// code block
break;
case two:
switch (expression) {
case one:
// code block
break;
case two:
// code block
break;
default:
// code block
break;
}
break;
default:
// code block
}
Thanks for reading:)
Subscribe to my newsletter
Read articles from Saswat Pal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Saswat Pal
Saswat Pal
Hi there! I'm a computer science student with a keen interest in Java programming and Data Structures & Algorithms. Currently, I am honing my skills in these areas and also familiar with C, C++. As an aspiring developer, I am always open to new opportunities and collaborations that allow me to learn and grow. Let's connect and create something great together!