Prisma vs. SQL: Why This Modern ORM is a Game-Changer
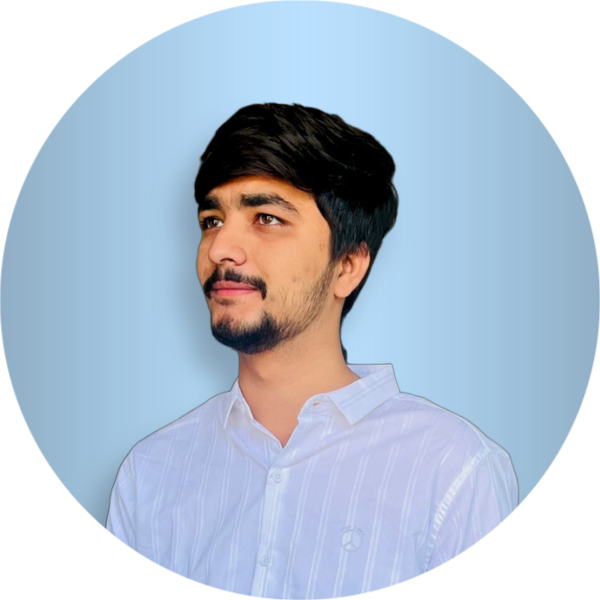
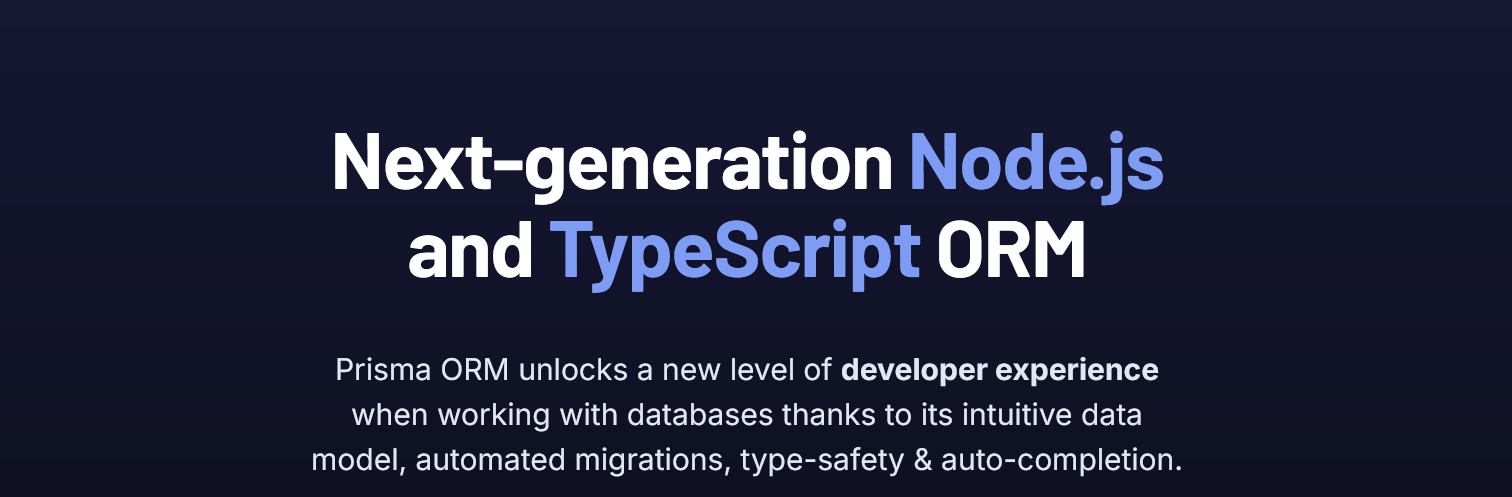
Introduction
If you’ve been working with databases in Node.js, you might have heard about Prisma. It’s a powerful tool that helps developers interact with databases more easily and efficiently. But what exactly is Prisma, and why should you consider using it instead of traditional SQL? In this blog, we’ll dive into Prisma, explore its benefits, and guide you through setting it up in your project.
What is Prisma?
Prisma is a modern database toolkit for Node.js and TypeScript applications. It acts as a bridge between your application and your database, making it easier to perform operations like creating, reading, updating, and deleting data (CRUD operations).
Prisma consists of three main parts:
Prisma Client: An auto-generated and type-safe query builder for Node.js.
Prisma Migrate: A tool for managing your database schema migrations.
Prisma Studio: A visual editor to explore and manage your data.
Why Prisma?
Prisma brings several advantages to the table, especially if you’re used to writing raw SQL queries:
Productivity: With Prisma, you don’t need to write long SQL queries. Instead, you use a query builder that generates these queries for you.
Type Safety: Prisma automatically generates TypeScript types based on your database schema. This means you get compile-time checks, which help catch errors early.
Flexibility: Prisma supports multiple databases, including PostgreSQL, MongoDB, MySQL, SQLite, and others. It’s a versatile tool that can be used in various types of projects.
Prisma vs. SQL: Why Not Just Use SQL?
Raw SQL gives you complete control over your database, which is great for performance and flexibility. However, it comes with its own set of challenges:
Boilerplate Code: Writing SQL queries can be repetitive and time-consuming.
Lack of Type Safety: With raw SQL, errors in queries often show up only at runtime, making debugging harder.
Maintenance: As your project grows, maintaining raw SQL queries can become complex and error-prone.
Prisma addresses these challenges by providing a higher level of abstraction while still allowing you to use raw SQL when needed.
Setting Up Prisma in Your Project
Ready to give Prisma a try? Let’s walk through the setup process.
Step 1: Prerequisites
Before we start, make sure you have Node.js and npm (or yarn) installed. You should also have a basic understanding of JavaScript/TypeScript and SQL.
Step 2: Installing Prisma
Start by setting up a new Node.js project. Open your terminal and run:
npm init -y
npm install @prisma/client
npx prisma init
This will install Prisma and create a prisma
folder with a schema.prisma
file, where you’ll define your database schema.
Step 3: Configuring the Prisma Schema
The schema.prisma
file is where you define your database models (like tables in SQL). Here’s an example of a simple User model:
model User {
id Int @id @default(autoincrement())
name String
email String @unique
posts Post[]
}
model Post {
id Int @id @default(autoincrement())
title String
content String
author User @relation(fields: [authorId], references: [id])
authorId Int
}
This schema defines two models: User
and Post
, with a one-to-many relationship.
Step 4: Connecting to Your Database
In the schema.prisma
file, you’ll also configure your database connection. Replace the datasource
block with your database connection string:
datasource db {
provider = "postgresql" // or "mysql","mongodb","sqlite", etc.
url = env("DATABASE_URL")
}
Make sure to set the DATABASE_URL
in your .env
file.
Prisma Migrate: Managing Database Migrations
Prisma Migrate helps you evolve your database schema over time.
Creating a Migration
To create your first migration, run:
npx prisma migrate dev --name init
This will create the migration files and apply them to your database.
Prisma Client: Basic CRUD Operations
Now that your database is set up, let’s explore how to perform basic CRUD operations using Prisma Client.
1. Create:
To create a new record in the database, you can use the create
method. Here’s how you can create a new user:
const { PrismaClient } = require('@prisma/client');
const prisma = new PrismaClient();
async function createUser() {
const newUser = await prisma.user.create({
data: {
name: 'kishan',
email: 'kishan@example.com',
},
});
console.log('User Created:', newUser);
}
createUser();
This will insert a new user named kishan into the User
table.
2. Read: Fetching Data
To retrieve data from the database, you can use the findMany
or findUnique
methods. Here’s how to fetch all users:
async function fetchUsers() {
const users = await prisma.user.findMany();
console.log('All Users:', users);
}
fetchUsers();
You can also fetch a single user by a unique field like id
or email
:
async function fetchUserByEmail(email) {
const user = await prisma.user.findUnique({
where: { email: email },
});
console.log('User Found:', user);
}
fetchUserByEmail('kishan@example.com');
3. Update: Modifying Data
To update an existing record, use the update
method. Here’s how to update a user’s name:
async function updateUser(id) {
const updatedUser = await prisma.user.update({
where: { id: id },
data: { name: 'Kishan Updated' },
});
console.log('User Updated:', updatedUser);
}
updateUser(1); // Assuming the user with ID 1 exists
This will update the name of the user where ID 1.
4. Delete: Removing Data
To delete a record from the database, use the delete
method. Here’s how to delete a user by their ID:
async function deleteUser(id) {
const deletedUser = await prisma.user.delete({
where: { id: id },
});
console.log('User Deleted:', deletedUser);
}
deleteUser(1); // Assuming the user with ID 1 exists
This will remove the user where ID 1 from the database.
Best Practices for Using Prisma
Schema Organization: Keep your Prisma schema organized, especially as your project grows.
TypeScript: Use TypeScript to take full advantage of Prisma’s type safety.
Migration Management: Regularly apply and test migrations in development to avoid issues in production.
Conclusion
Prisma simplifies working with databases in Node.js applications, offering productivity, type safety, and flexibility. Whether you’re a beginner or an experienced developer, Prisma is a valuable tool that can help you build and maintain scalable applications with ease.
Further Resources
To dive deeper into Prisma, check out these resources:
Subscribe to my newsletter
Read articles from Kishan Kareliya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
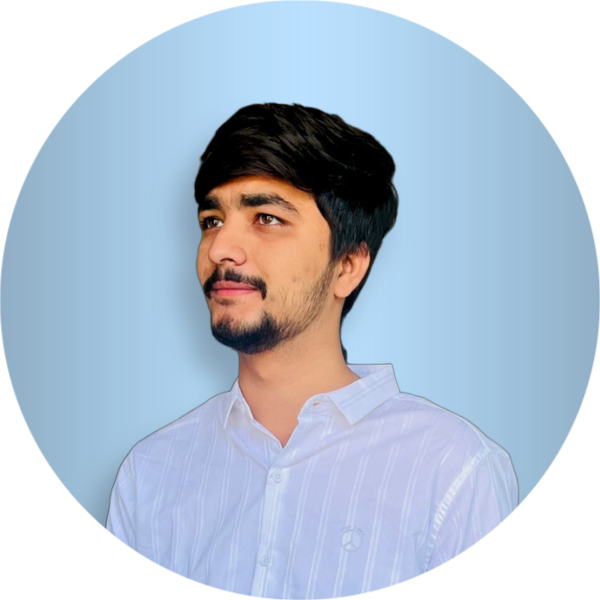