Day 11 Task: Error Handling in Shell Scripting

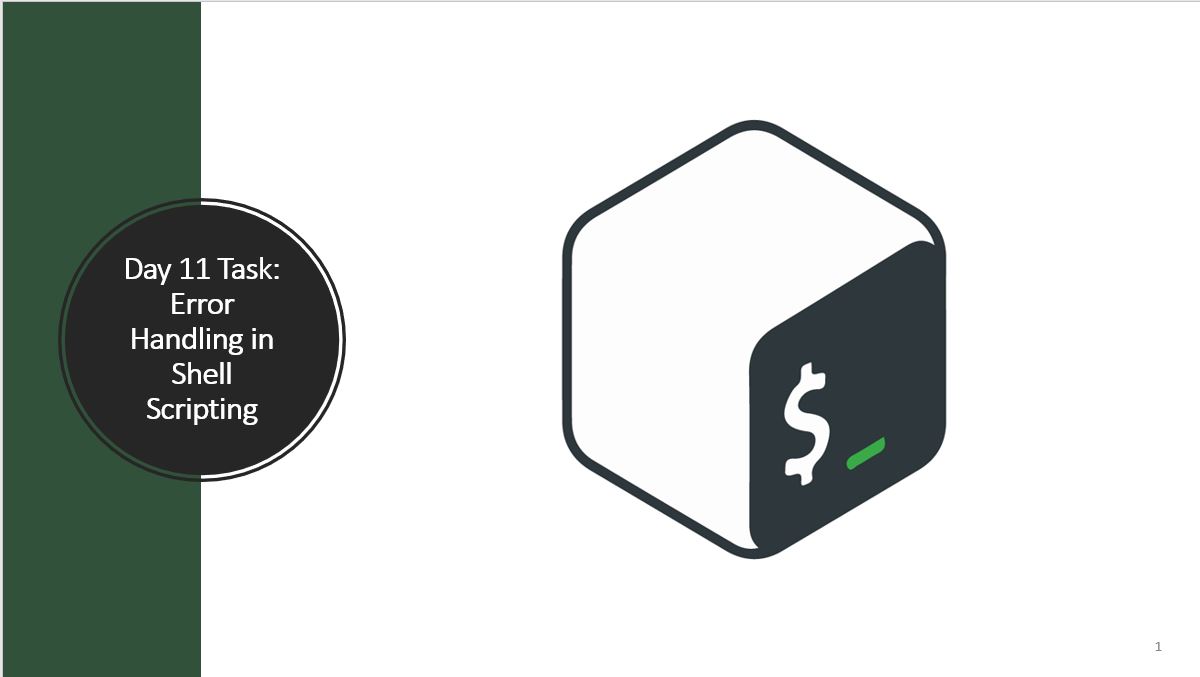
Learning Objectives
Understanding how to handle errors in shell scripts is crucial for creating robust and reliable scripts. Today, you'll learn how to use various techniques to handle errors effectively in your bash scripts.
Topics to Cover
Understanding Exit Status: Every command returns an exit status (0 for success and non-zero for failure). Learn how to check and use exit statuses.
Using
if
Statements for Error Checking: Learn how to useif
statements to handle errors.Using
trap
for Cleanup: Understand how to use thetrap
command to handle unexpected errors and perform cleanup.Redirecting Errors: Learn how to redirect errors to a file or
/dev/null
.Creating Custom Error Messages: Understand how to create meaningful error messages for debugging and user information.
Tasks
Task 1: Checking Exit Status
In a shell script, the exit status of a command indicates whether the command was successful or not. By convention:
An exit status of 0 means the command was successful.
Any non-zero exit status (usually 1) indicates that the command failed.
How to Check Exit Status
Using $?:
- After a command is executed, you can check its exit status using the special variable $?. This variable holds the exit status of the last executed command.
#!/bin/bash
# Store the first argument as the directory path
directory_path=$1
# Create the directory
mkdir -p "$directory_path"
# Check if the directory was created successfully
if [ $? -eq 0 ]; then
echo "Directory created successfully at: $directory_path"
else
echo "Failed to create directory at: $directory_path"
exit 1
fi
Output
ubuntu@ip-172-31-19-250:~/Scripts$ vim task11.sh
ubuntu@ip-172-31-19-250:~/Scripts$ ./task11.sh /home/ubuntu/Vibhuti
Directory created successfully at: /home/ubuntu/Vibhuti
ubuntu@ip-172-31-19-250:~/Scripts$
Task 2: Using if
Statements for Error Checking
- Modify the script from Task 1 to include more commands (e.g., creating a file inside the directory) and use
if
statements to handle errors at each step.
#!/bin/bash
# Check if the user provided an argument (the directory path)
if [ -z "$1" ]; then
echo "Usage: $0 <directory_path>"
exit 1
fi
# Store the first argument as the directory path
directory_path=$1
# Create the directory
touch "$directory_path"
# Check if the directory was created successfully
if [ $? -eq 0 ]; then
echo "File created successfully at: $directory_path"
else
echo "Failed to create file at: $directory_path"
exit 1
fi
Output:
ubuntu@ip-172-31-19-250:~/Scripts$ vim task11.sh
ubuntu@ip-172-31-19-250:~/Scripts$ ./task11.sh /home/ubuntu/hello.txt
File created successfully at: /home/ubuntu/hello.txt
Task 3: Using trap
for Cleanup
- Write a script that creates a temporary file and sets a
trap
to delete the file if the script exits unexpectedly.
#!/bin/bash
tempfile=$(mktemp)
function cleanup() {
echo "Cleaning up....."
rm -f ${tempfile}
echo "Temporary file deleted" ${tempfile}
}
trap cleanup EXIT
echo "This is temporary file" > ${tempfile}
cat ${tempfile}
exit 1
ubuntu@ip-172-31-19-250:~/Scripts$ ./cleanup.sh
This is temporary file
Cleaning up.....
Temporary file deleted /tmp/tmp.tHBEOuQgCO
Task 4: Redirecting Errors
- Write a script that tries to read a non-existent file and redirects the error message to a file called
error.log
.
$cat hello.txt 2> error.log
ubuntu@ip-172-31-19-250:~/Scripts$ cat error.log
cat: hello.txt: No such file or directory
Task 5: Creating Custom Error Messages
- Modify one of the previous scripts to include custom error messages that provide more context about what went wrong.
#!/bin/bash
mkdir /tmp/mydir
if [ $? -ne 0 ]; then
echo "Error: Directory /tmp/mydir could not be created. Check if you have the necessary permissions."
fi
ubuntu@ip-172-31-19-250:~/Scripts$ ./custom.sh
mkdir: cannot create directory ‘/tmp/mydir’: File exists
Error: Directory /tmp/mydir could not be created. Check if you have the necessary permissions.
Follow for more updates:)
Subscribe to my newsletter
Read articles from Vibhuti Jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vibhuti Jain
Vibhuti Jain
Hi, I am Vibhuti Jain. A Devops Tools enthusiastic who keens on learning Devops tools and want to contribute my knowledge to build a community and collaborate with them. I have a acquired a knowledge of Linux, Shell Scripting, Python, Git, GitHub, AWS Cloud and ready to learn a lot more new Devops skills which help me build some real life project.