Crafting a Personalized Virtual Rakhi Card with React: A Digital Twist on Tradition
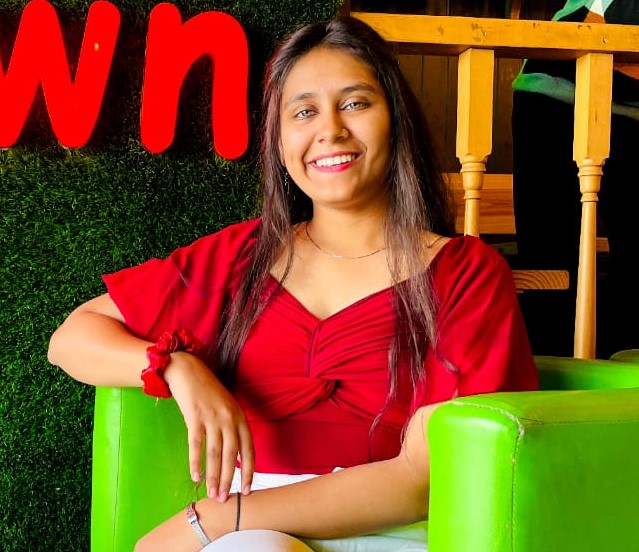
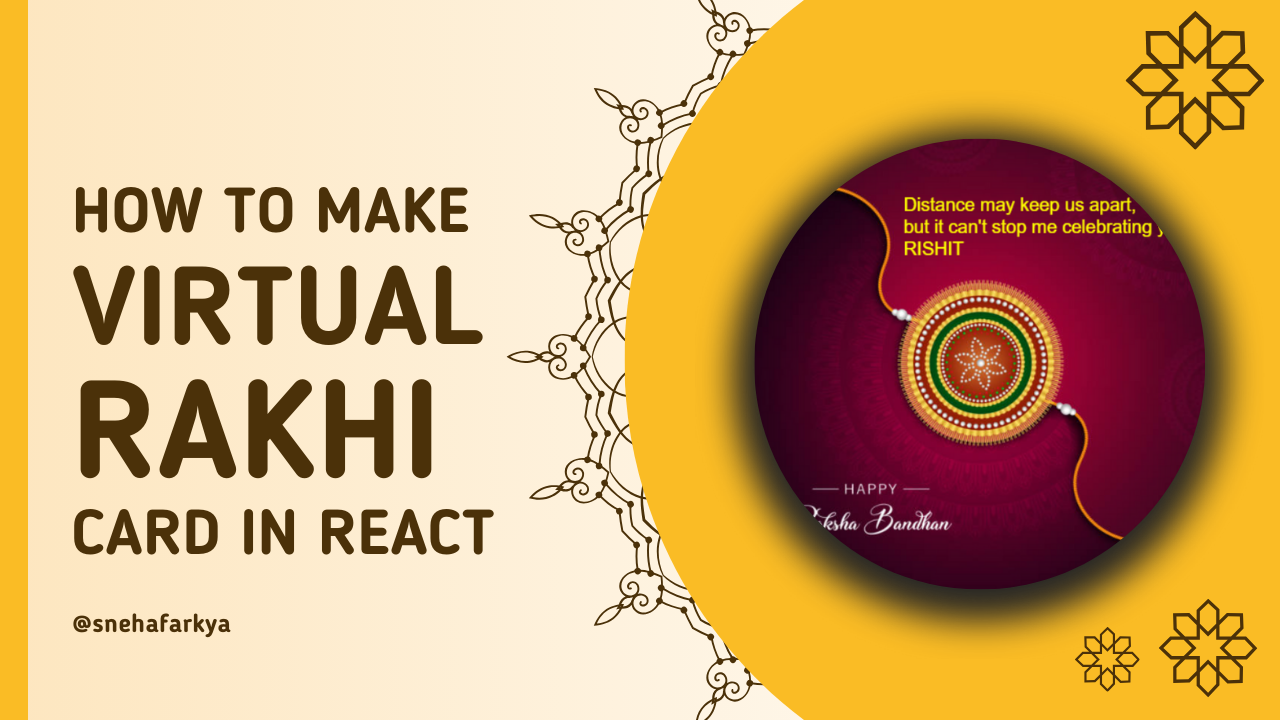
In today’s fast-paced world, where physical distances often separate loved ones, technology bridges the gap creatively and meaningfully. One such beautiful innovation is the concept of virtual rakhi cards, which allows us to celebrate traditional festivals like Raksha Bandhan with our siblings even when we are miles apart. In this blog post, we’ll explore how to create a personalised virtual rakhi card using React.js, adding a unique and modern touch to this age-old tradition.
The Significance of Raksha Bandhan
Raksha Bandhan is a cherished Indian festival celebrating the bond between brothers and sisters. On this special day, sisters tie a rakhi (a sacred thread) around their brothers' wrists, symbolising protection and care. Brothers, in return, promise to safeguard their sisters and offer gifts as a token of their love and commitment. However, with the demands of modern life, we might not always be physically present with our loved ones on this occasion.
Why a Virtual Rakhi Card?
Virtual rakhi cards are a brilliant way to maintain this tradition even when separated by distance. They offer a platform to send personalized messages and images, allowing siblings to feel connected despite the physical separation. Creating a virtual rakhi card with React.js not only adds a personal touch but also leverages modern web technologies to make the experience interactive and engaging.
Building the Virtual Rakhi Card with React
In this tutorial, we’ll create a virtual rakhi card application using React.js. Our application will allow users to input custom text, overlay it on a predefined image, and download or copy the URL of the final image. Here’s a step-by-step guide to building this application.
1. Setting Up the Project
First, set up a new React project if you haven’t already:
npx create-react-app virtual-rakhi
cd virtual-rakhi
2. Creating the React Component
Create a new component VirtualRakhi.js
in the src
folder. This component will handle text input, canvas drawing, and image generation.
Note: Put your image in public folder.
We begin by setting up a React component that will manage the state and interactions of the application. Here’s the code for the basic setup:
import React, { useState, useRef, useEffect } from "react";
function VirtualRakhi() {
const [text, setText] = useState("");
const canvasRef = useRef(null);
State (
text
): Holds the text input by the user. This state updates whenever the user types in the input field.Ref (
canvasRef
): Provides a reference to the canvas element in the DOM, enabling us to draw on it and manipulate its content.
Drawing on the Canvas
The function drawTextOnCanvas
is responsible for rendering the image and user input text on the canvas. Here’s how it works:
const drawTextOnCanvas = () => {
const canvas = canvasRef.current;
const ctx = canvas.getContext("2d");
// Clear the canvas
ctx.clearRect(0, 0, canvas.width, canvas.height);
// Load a local image
const img = new Image();
img.src = `${process.env.PUBLIC_URL}/rakhii.jpg`; // Replace with your image file name
img.crossOrigin = "anonymous"; // Allow cross-origin if needed
img.onload = () => {
ctx.drawImage(img, 0, 0, canvas.width, canvas.height);
// Set text properties
ctx.font = "20px Arial";
ctx.fillStyle = "yellow";
ctx.textAlign = "left";
// Draw the first part of the text
ctx.fillText("Distance may keep us apart,", canvas.width / 3, canvas.height / 6);
ctx.fillText("but it can't stop me celebrating you,", canvas.width / 3, canvas.height / 6 + 24);
// Draw the user input text
ctx.font = "bold 20px Arial"; // Set font to bold
ctx.fillText(text.toUpperCase(), canvas.width / 3, canvas.height / 6 + 48);
};
};
canvasRef.current
: Retrieves the canvas element from the DOM.getContext("2d")
: Gets the 2D drawing context, which allows us to perform drawing operations.clearRect
: Clears the canvas before drawing to prevent overlapping content.Image
Object: Loads and draws the background image.fillText
: Draws both the static and user-input text onto the canvas.
Handling User Actions
We need functions to handle downloading the image and copying its URL:
const handleDownload = () => {
const canvas = canvasRef.current;
const link = document.createElement("a");
link.download = `${text}'s-virtual-rakhi.png`;
link.href = canvas.toDataURL();
link.click();
};
const handleSaveName = () => {
drawTextOnCanvas(); // Redraw the canvas with the new text
};
const handleCopyUrl = () => {
const canvas = canvasRef.current;
const imageUrl = canvas.toDataURL("image/png");
// Copy the URL to the clipboard
navigator.clipboard.writeText(imageUrl).then(() => {
alert("Image URL copied to clipboard!");
});
};
handleDownload
:toDataURL()
: Converts the canvas content to a data URL, which is used to download the image.link.download
: Sets the filename for the downloaded image.link.click
()
: Triggers the download process.
handleSaveName
: Refreshes the canvas with the updated text when the "Print Name" button is clicked.handleCopyUrl
:navigator.clipboard.writeText(imageUrl)
: Copies the data URL of the image to the clipboard, enabling users to paste it wherever needed.
Rendering the Component
The JSX part of the component manages the rendering of the input field, buttons, and canvas:
return (
<div className="flex justify-center flex-col md:w-fit w-[380px] mx-auto md:items-center py-10 gap-6">
<h1 className="text-3xl font-semibold">
Send this virtual rakhi to your <span className="uppercase font-bold text-amber-900">siblings!</span>
</h1>
<div className="flex md:justify-center md:items-center gap-4">
<input
type="text"
value={text}
onChange={(e) => setText(e.target.value)}
className="p-2 bg-transparent text-amber-950 rounded-lg font-semibold focus:outline-none border-2 placeholder:text-amber-950 border-amber-950"
placeholder="Write your sibling's name"
/>
<button onClick={handleSaveName} className="bg-amber-950 border-2 border-amber-950 text-white p-2 rounded-md shadow-xl hover:bg-amber-900">
Print Name
</button>
</div>
<canvas
ref={canvasRef}
width={500}
height={500}
className="rounded-xl"
/>
<button onClick={handleDownload} className="bg-amber-950 border-2 w-fit mx-auto border-amber-950 text-white p-2 rounded-md shadow-xl hover:bg-amber-900">
Download Image
</button>
<button onClick={handleCopyUrl} className="bg-amber-950 border-2 w-fit mx-auto border-amber-950 text-white p-2 rounded-md shadow-xl hover:bg-amber-900">
Copy Image URL
</button>
</div>
);
}
HTML Structure:
<input>
: For user input of the sibling's name.<button>
: Triggers actions like saving the name, downloading the image, or copying the image URL.<canvas>
: Displays the image with overlaid text.
Understanding the Canvas Element
The canvas element in HTML provides a versatile space for drawing graphics dynamically using JavaScript. Here’s why it’s significant:
Dynamic Graphics: Allows for real-time drawing and manipulation of graphics.
2D Context: Supports basic graphics operations like drawing shapes, text, and images.
Web Applications: Useful for interactive applications such as games, photo editors, and data visualizations.
In our project, the canvas element is used to overlay user input text on a static image, creating a personalized virtual rakhi card.
3. Styling the Component
Ensure your App.css
or equivalent stylesheet includes basic styling for your component to enhance its appearance. I am using Tailwind CSS for styling.
4. Testing and Deployment
Test Locally: Run
npm start
to test the functionality on your local development server.Deploy: Deploy your application to Vercel or any other hosting provider. Here is the live link to the deployed application: Virtual Rakhi App.
Real-Life Example
Imagine you are working in a different city, and Raksha Bandhan is around the corner. You want to send your sibling a heartfelt message but can't be there in person. Using this virtual rakhi card, you can easily create a personalized card with your sibling's name and a special message. Simply enter the name, click the button to generate the card, and then either download the image or copy the URL to share it. This way, you can make your sibling feel special and loved even from afar.
Conclusion
Creating a virtual rakhi card with React is a wonderful way to blend tradition with modern technology. It allows us to stay connected and celebrate important occasions even when we’re not physically present. By following the steps outlined above, you can create your own interactive and personalized rakhi card, adding a unique touch to your celebrations.
Feel free to try out the live application and see how you can make your Raksha Bandhan celebrations more special: Virtual Rakhi App.
You can just experiment with this example and explore Canvas in more depth.
Want to read more?
Find me on this socials, I would love to connect with you:
Have a wonderful day🌻!
Subscribe to my newsletter
Read articles from Sneha Farkya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
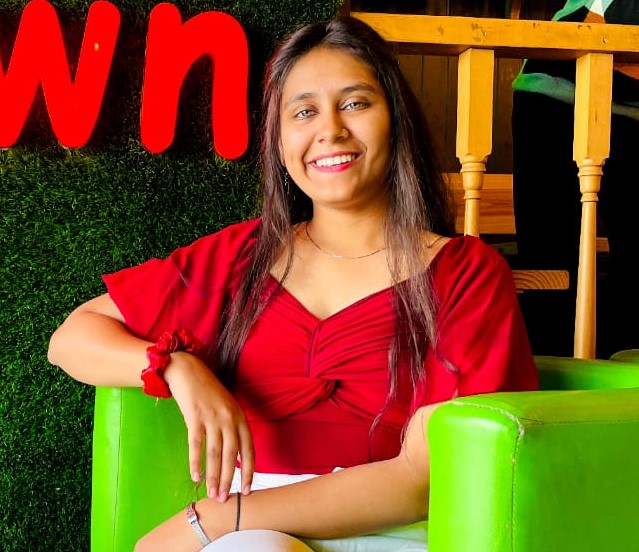
Sneha Farkya
Sneha Farkya
This is Sneha Farkya from India. I am a front-end developer and technical writer with a strong interest in creating interactive and visually appealing web interfaces. I am constantly exploring new technologies and keeping up with the latest trends. I like to solve people's problems and believe in giving bac to community