Essential Techniques for Array Problem Solving You Must Know 👨‍💻
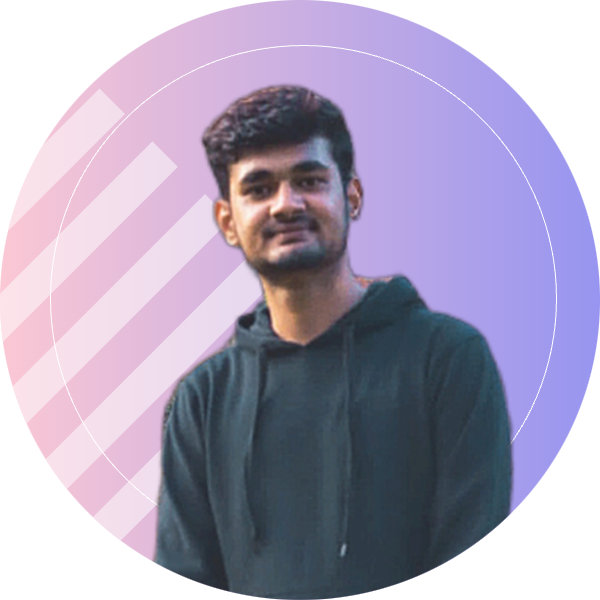
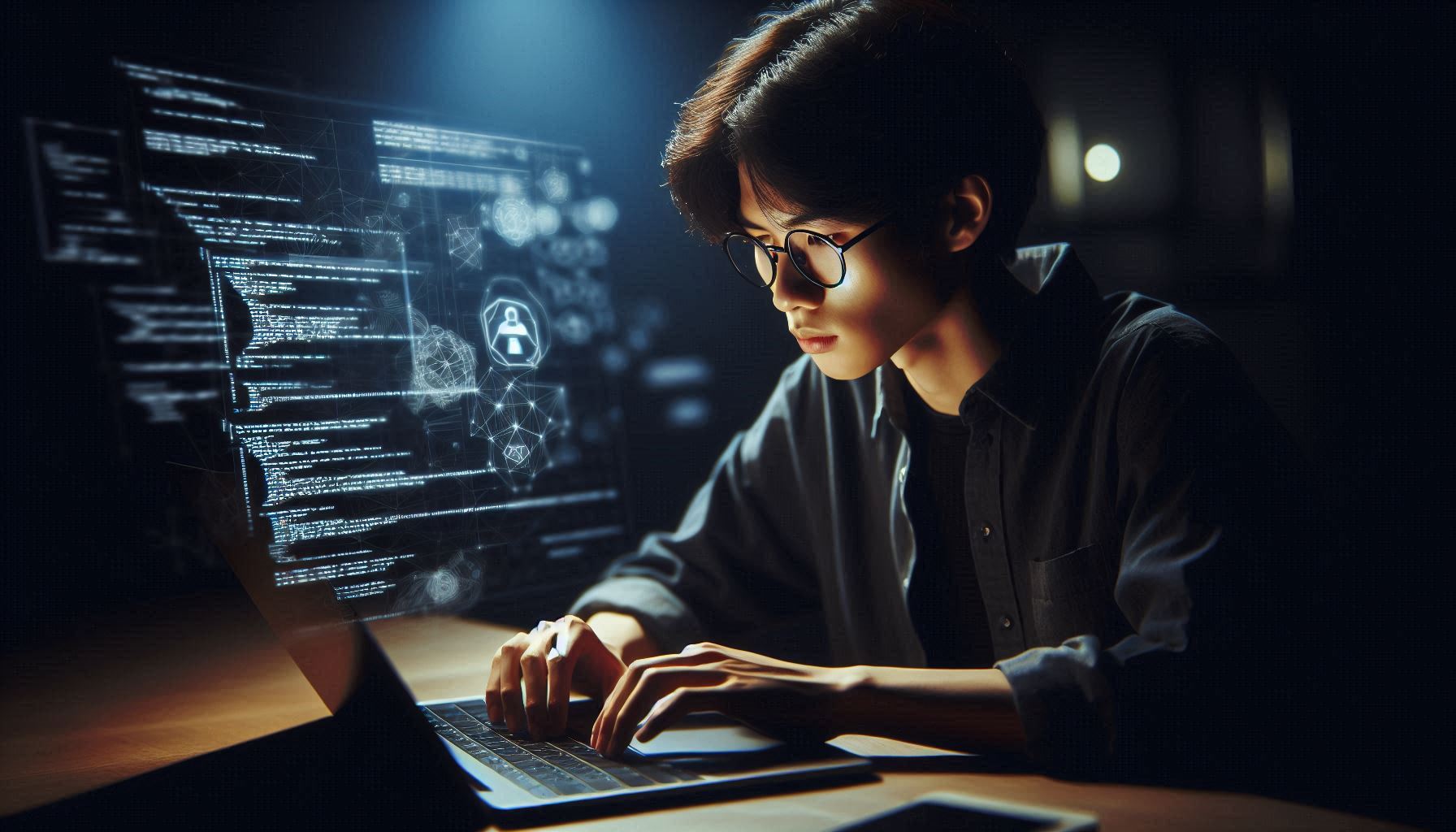
If you are doing competitive programming (CP) or simply solving problem-solving questions on arrays, optimizing the time complexity of your solutions is important, specially when you are dealing with large inputs.
Two of the most effective techniques for improving efficiency in array and string manipulation are the Two-Pointer and Sliding Window approaches. In this blog I will try to explain you what these techniques are, the types of problems they are best suited for, and provide C++ templates for implementing them in your code.
So let’s just get started !!
What is the Two-Pointer Approach?
The two-pointer approach involves using two indices (pointers) to traverse a data structure, usually starting from different ends or moving towards each other to meet a specific condition. This technique is particularly useful when working with sorted arrays or when you need to evaluate pairs or triplets of elements.
Use Cases
Finding pairs or triplets that satisfy certain conditions (e.g., sum to a specific value).
Checking for palindromes in a string.
Merging two sorted arrays.
void twoPointerTemplate(vector<int>& arr, int target) {
int left = 0;
int right = arr.size() - 1;
while (left < right) {
int sum = arr[left] + arr[right];
if (sum == target) {
// Logic for when the target is found
break;
} else if (sum < target) {
left++; // Move the left pointer to the right
} else {
right--; // Move the right pointer to the left
}
}
}
Now let’s talk about the sliding window technique.
What is the Sliding Window Technique?
The sliding window technique is a method used to keep track of a subset of elements in an array or string. By moving the window across the dataset, you can efficiently compute sums, maximums, minimums, or any other property over the subset without recalculating from scratch.
Use Cases
Finding the maximum or minimum sum of a subarray of fixed size.
Identifying the longest substring with unique characters.
Calculating the sum of elements within a dynamic window.
C++ Template for Sliding Window Technique
void slidingWindowTemplate(vector<int>& arr, int k) {
int n = arr.size();
int windowSum = 0, maxSum = 0;
// Initialize the first window
for (int i = 0; i < k; i++) {
windowSum += arr[i];
}
maxSum = windowSum;
// Slide the window across the array
for (int i = k; i < n; i++) {
windowSum += arr[i] - arr[i - k];
maxSum = max(maxSum, windowSum);
}
}
Conclusion
Two-Pointer and Sliding Window techniques are essential for efficient problem-solving with arrays and strings. Mastering these methods helps reduce time complexity and improves your problem-solving skills. Practice these techniques regularly to integrate them seamlessly into your coding approach.
For further reading or practice, you can read or solve questions from the links below.
Thanks for reading!!
Subscribe to my newsletter
Read articles from Musab Rayan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
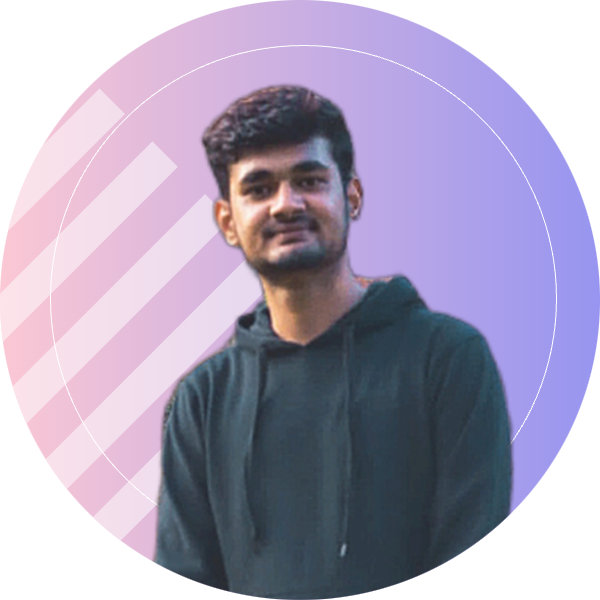
Musab Rayan
Musab Rayan
Hello, I'm Musab Rayan, a third-year CSE student at BSA Crescent Institute of Science and Technology. I love building websites! Since I was a kid, I've been curious about how they work, and now that curiosity drives me into the exciting world of tech. Currently honing my skills in DSA, I aspire to carve a successful career as a software engineer with a focus on innovation.