Week 2 DevOps Progress: Practical Applications of Linux Script and AWS Tools
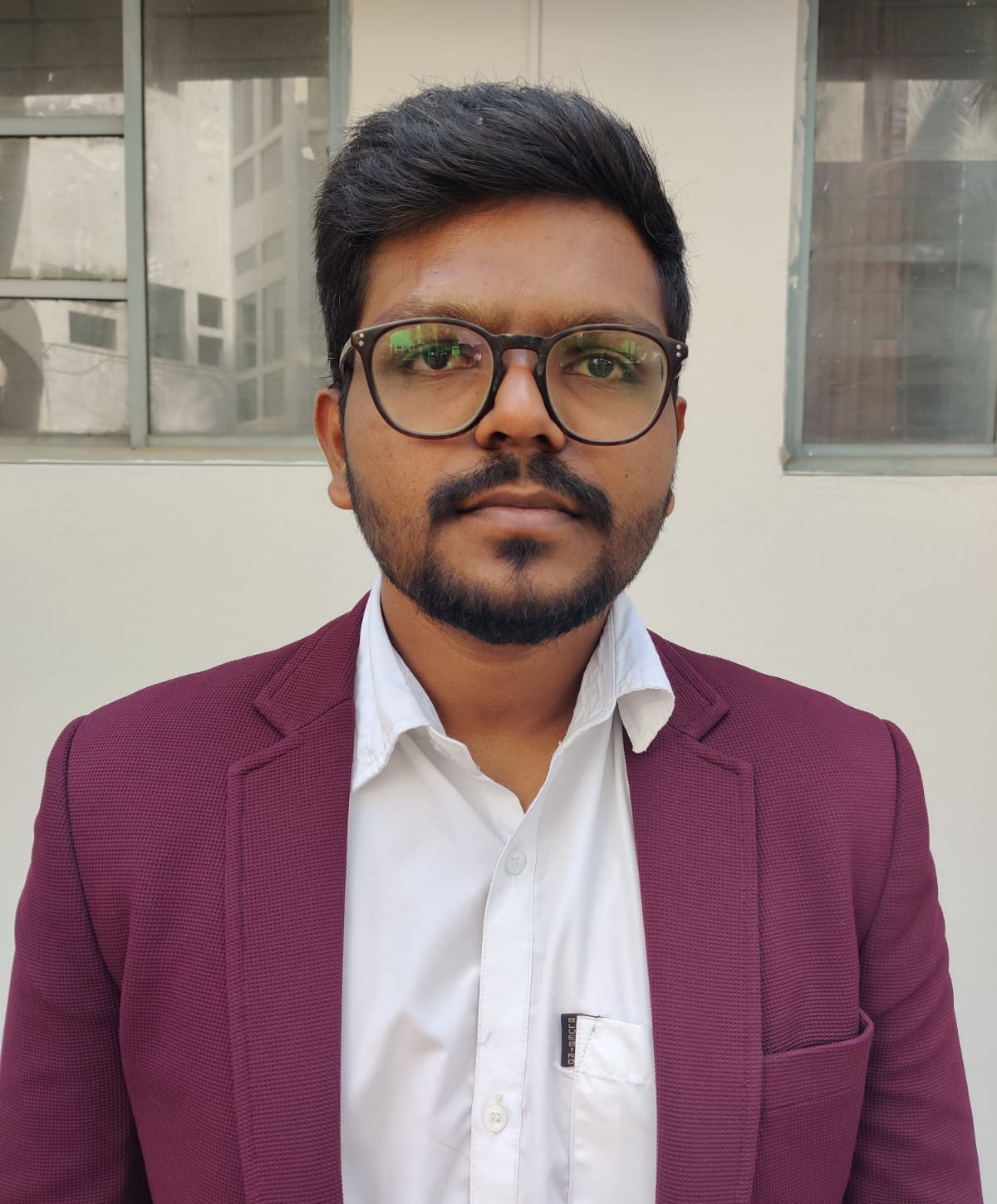
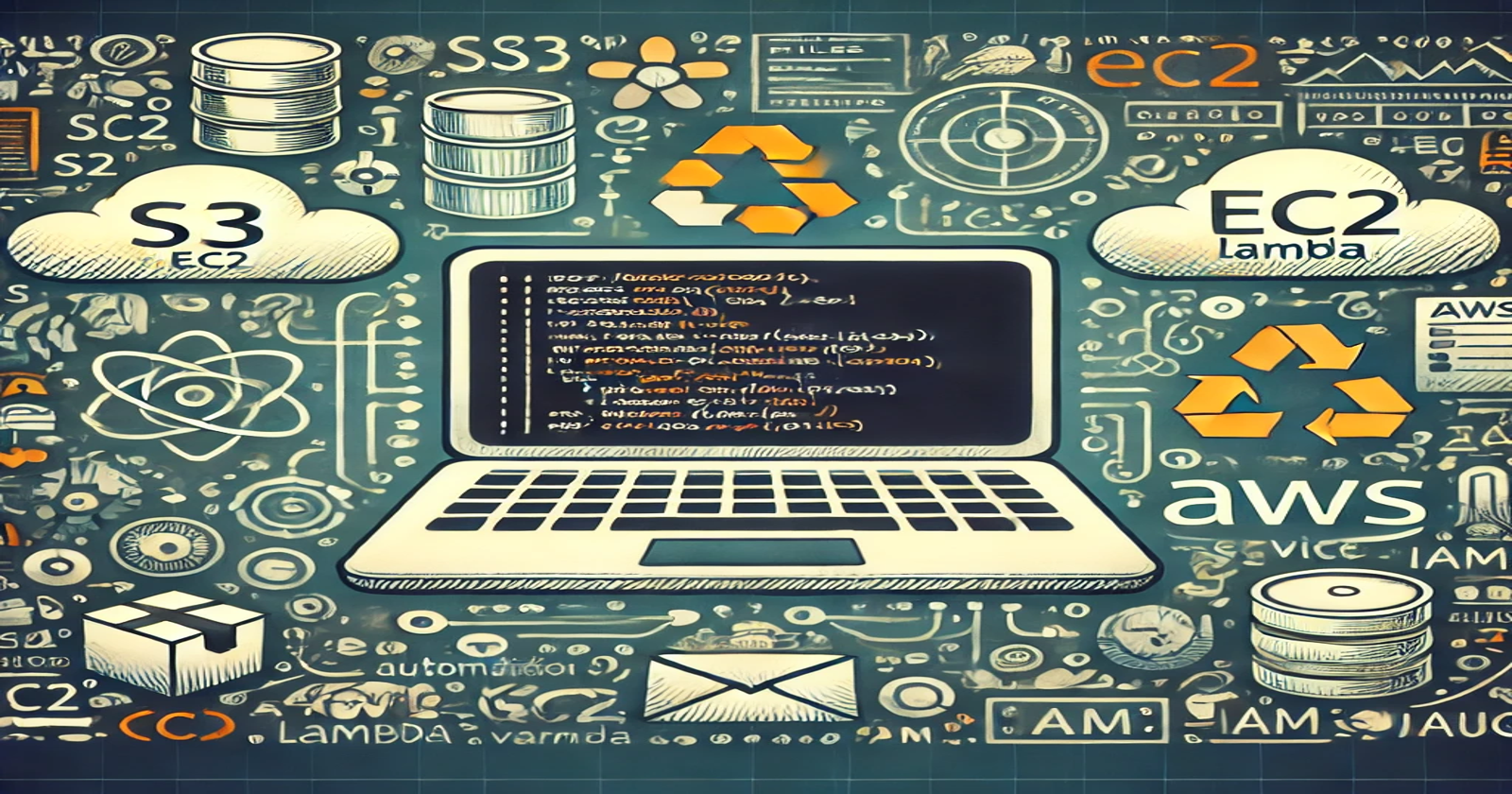
Introduction
This week, I continued my DevOps journey by diving into Linux scripting and exploring different ways to connect and interact with AWS. I learned the basics of Linux scripting and various methods to connect with AWS, including a practical script to report AWS usage for EC2 instances and S3 buckets.
"The main goal of a DevOps engineers is to automate processes "
Understanding Bash Scripting
Bash scripting is a powerful tool for automating repetitive tasks and managing system operations. It’s an essential skill for efficiently handling servers and applications in a DevOps environment.
What is Bash?
Bash (Bourne Again Shell) is a Unix shell and command language that allows you to automate sequences of commands, reducing the need for manual intervention.
Beyond Bash: Other Automation Tools
In addition to Bash, we can use Python with modules like Boto for AWS management, among other tools. These alternatives provide powerful options for automating complex workflows and integrating with various services, further extending our automation capabilities.
Essential Bash Commands
ls
- List directory contentscd
- Change directorycp
- Copy files or directoriesmv
- Move or rename files or directoriesrm
- Remove files or directoriesecho
- Display a line of textcat
- Concatenate and display file contents
Creating the First Bash Script
Create a new file:
touch myscript.sh chmod +x myscript.sh # Making it executable file # use nano or vim to write
What if we want to change permissions for 100 scripts? Check out the
umask
command to learn how to control and adjust default file permissions for new scripts.Add a shebang and commands:
#!/bin/bash echo "Hello, World!"
What Happens:
#!/bin/bash
it indicates that the script should be executed using the Bash shell located at/bin/bash
.echo "Hello, World!"
is a command within the script that prints the text "Hello, World!" to the terminal when the script is run.
Running the script:
./myscript.sh
Variables and User Input
Variables store data that can be used throughout the script.
Declaring Variables:
NAME="Srinivas" echo "Hello, $NAME!"
Reading User Input:
read -p "Enter your name: " USERNAME echo "Welcome, $USERNAME!"
Conditional Statements
If-Else Statement:
The
if-else
statement executes commands based on whether a condition is true or false.#!/bin/bash # Check if the user is root if [ "$USER" == "root" ]; then echo "You are the root user." else echo "You are not the root user." fi
Loops
Loops are used to repeat tasks until a condition is met or for a set number of iterations.
For Loop:
The
for
loop iterates over a list of items or a range of numbers.#!/bin/bash # Loop through numbers 1 to 5 for i in {1..5}; do echo "Iteration $i" done
Functions
Functions encapsulate code into reusable blocks. This makes scripts more organised, modular, and easier to maintain.
#!/bin/bash # Defining a function named greet function greet() { echo "Hello, $1!" } # Call the function with an argument greet "Srinivas"
Accessing AWS: Different Methods
Managing AWS resources directly through a browser can be inefficient, especially with frequent updates or large-scale operations. Instead, using APIs and command-line tools can make these processes easier. By using AWS APIs and tools, we can automate tasks, manage infrastructure, and integrate AWS services into applications more effectively.
AWS CLI (Command Line Interface)
AWS SDKs and APIs
AWS CloudFormation
AWS CDK (Cloud Development Kit)
Terraform: Terraform is an open-source infrastructure as code software tool that provides a consistent CLI workflow to manage hundreds of cloud services. It allows you to build, change, and version your infrastructure safely and efficiently
AWS CLI (Command Line Interface)
The AWS CLI enables management of AWS services from the terminal.
Installation: Use
pip
or package managers to install.Configuration: Set up credentials and region with
aws configure
.$ aws configure AWS Access Key ID [None]: YOUR_ACCESS_KEY_ID AWS Secret Access Key [None]: YOUR_SECRET_ACCESS_KEY Default region name [None]: example ap-south-1 Default output format [None]: json
- Managing AWS resources efficiently requires more than just occasional checks through the AWS Management Console. To streamline operations and gain insights into resource usage, automating these tasks with scripts can be highly beneficial.
This script uses the AWS Command Line Interface (CLI) to list S3 buckets, describe EC2 instances, list Lambda functions, and retrieve IAM users.
#!/bin/bash # Author: Srinivas # This script will report AWS usage set -x aws s3 ls --region ap-south-1 aws ec2 describe-instances --region ap-south-1 aws lambda list-functions --region ap-south-1 aws iam list-users --region ap-south-1
Key Takeaways
This week, I explored the fundamentals of AWS CLI and its significance in automating and managing cloud resources .As it enables effective interaction with various AWS services directly from the terminal.
I would like to thank Abhishek Veeramalla for the "Devops Zero to Hero Course" playlist on YouTube. His tutorials have been invaluable in enhancing my learning and understanding of AWS CLI and cloud resource management.
Next Steps
Looking ahead, I’m excited to dive into the following topics in the coming weeks:
Starting with JavaScript: Learning JavaScript to add interactivity and dynamic features to web applications.
Git : Mastering version control and collaboration for efficient DevOps practices.
Ansible: Automating configuration management and application deployment.
These tools and skills are essential for modern DevOps practices and web development, and I look forward to integrating them into my workflow.
Connect with Me
- LinkedIn Profile: Srinivas Ch
Resources
- DevOps Zero to Hero YouTube Playlist by Abhishek Veeramalla
Subscribe to my newsletter
Read articles from srinivas ch directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
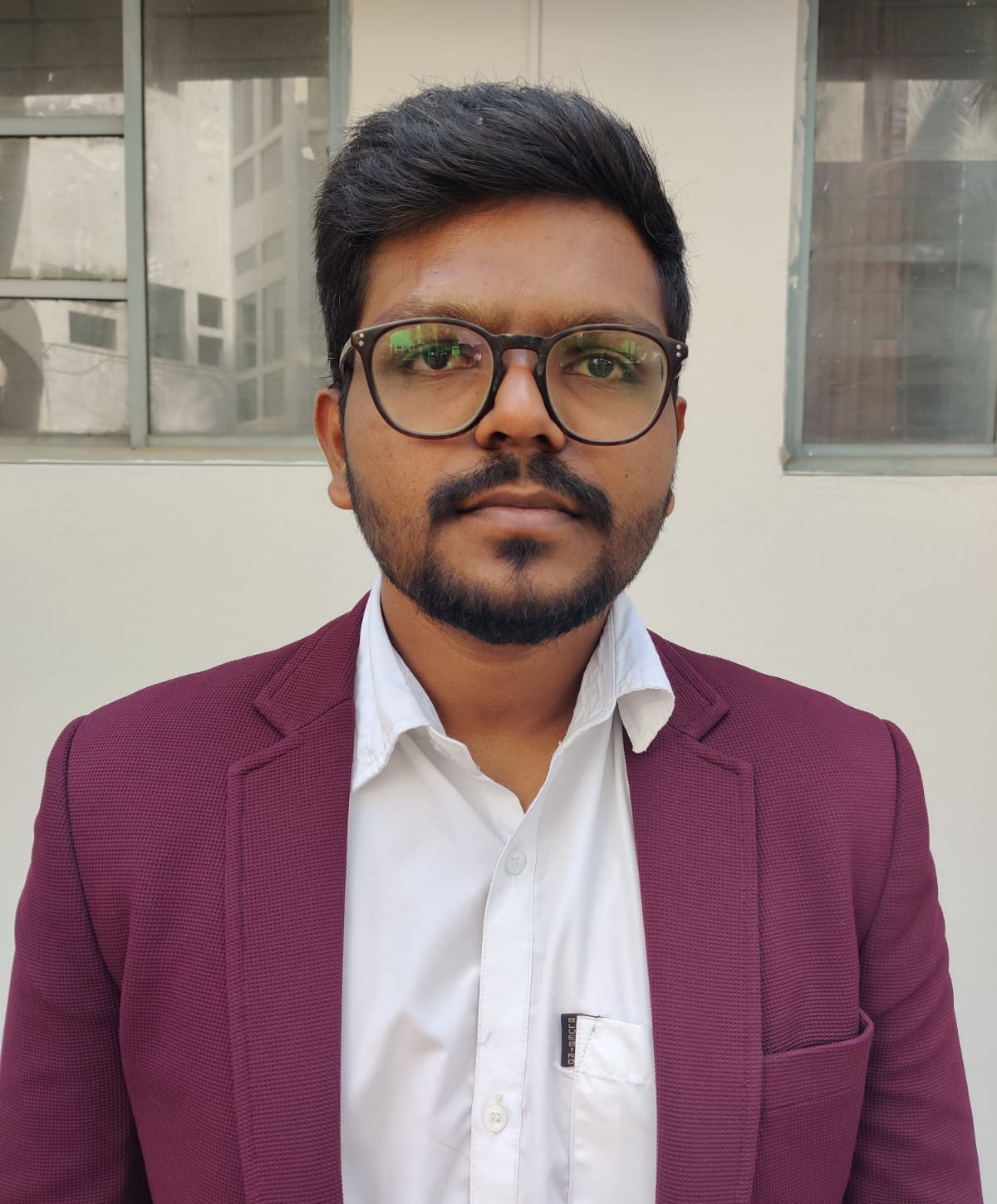