Python Project Setup: Laying the Groundwork for Coding Greatness

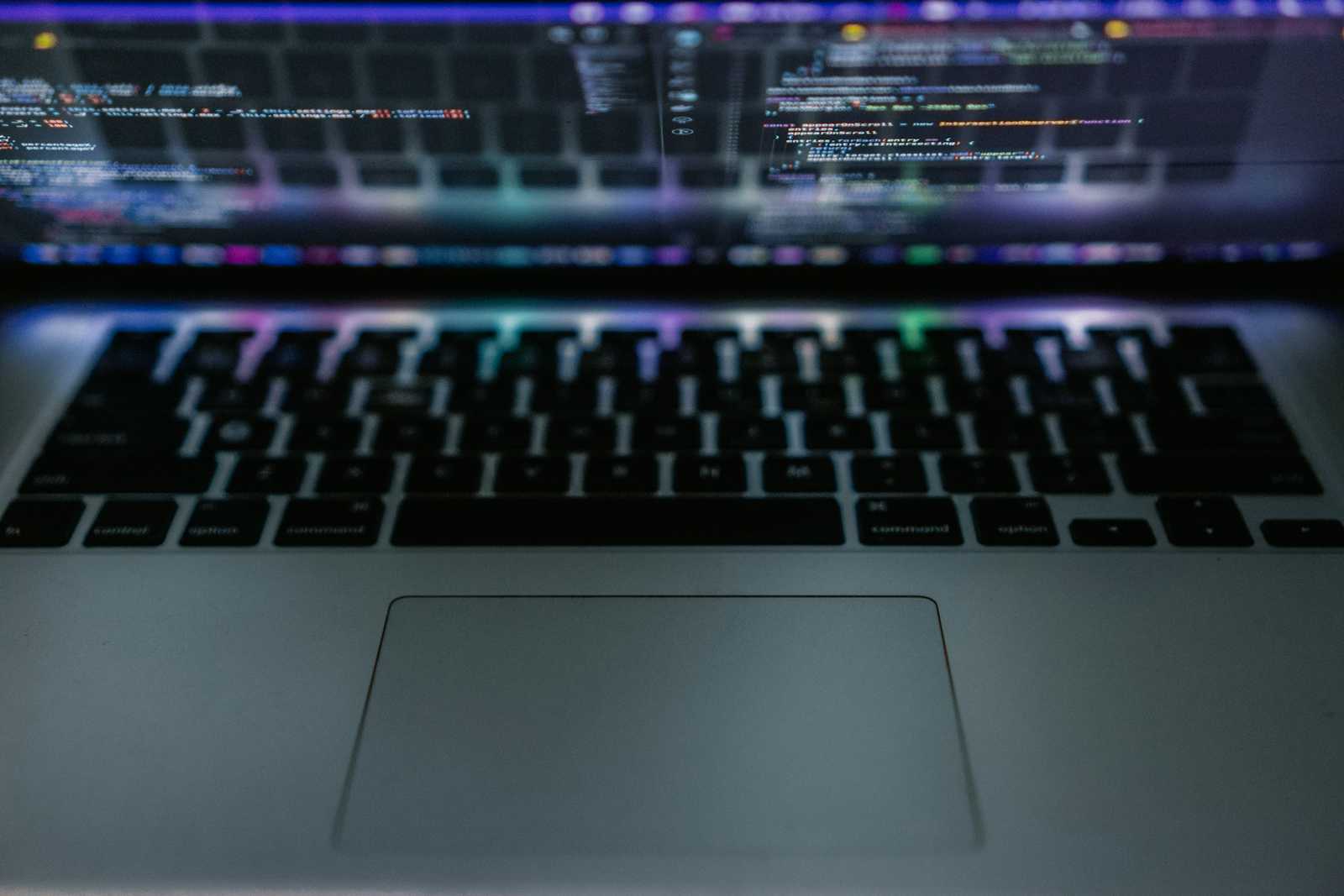
Okay, let's dive into the sometimes-overlooked but oh-so-crucial world of Python project setup. We all love jumping straight into the code, but a solid foundation can make all the difference between a smooth development journey and a frustrating mess. Let's explore the essentials to get your Python projects off on the right foot.
Virtual Environments: Your Project's Cozy Corner
Think of virtual environments like cozy little corners for your Python projects. They keep your project's dependencies separate from others, ensuring smooth sailing and preventing those dreaded "it works on my machine" moments. Let's set one up:
python -m venv venv
Now, activate your environment:
On Windows:
venv\Scripts\activate
On macOS and Linux:
source venv/bin/activate
A Place for Everything: Project Structure
A well-organized project is a joy to work with, so let's create a sensible structure:
mkdir my_project
cd my_project
Inside your project directory, consider this layout:
my_project/
│
├── src/ # Your Python code lives here
│ └── main.py # Main application script
│
├── tests/ # Keep your tests organized
│ └── test_main.py
│
├── docs/ # Documentation is your friend
│ └── README.md
│
├── venv/ # Your trusty virtual environment
├── .gitignore # We'll talk about this soon
└── requirements.txt # Your project's shopping list
This simple structure makes it easy to find what you need and helps others (or future you!) understand your project.
The Shopping List: requirements.txt
requirements.txt
is your project's shopping list. It tells Python which packages (think ingredients) your project needs to run.
As you install packages, keep track of them:
pip install package1 package2 package3
Generate
requirements.txt
:pip freeze > requirements.txt
Now, anyone can recreate your project's environment:
pip install -r requirements.txt
The Bouncer: .gitignore
.gitignore
acts like a bouncer at your project's repository door, keeping out unnecessary files that shouldn't be version controlled. Here's a typical .gitignore
for Python projects:
__pycache__/
*.py[cod]
*$py.class
*.so
.Python
build/
develop-eggs/
dist/
downloads/
eggs/
.eggs/
lib/
lib64/
parts/
sdist/
var/
wheels/
*.egg-info/
.installed.cfg
*.egg
# Virtual environment
venv/
ENV/
# PyCharm stuff
.idea/
*.iml
*.iws
.idea_modules/
# Other stuff you might want to ignore
*.log
.DS_Store
The Time Machine: Git
Git is your project's time machine, letting you track changes and revert to previous versions if needed.
Initialize a Git repository:
git init
Stage your files:
git add .
Make your first commit:
git commit -m "Initial commit: Project setup"
Don't forget to set up your Git identity:
git config --global user.email "your_email@example.com"
git config --global user.name "Your Name"
Leveling Up Your Project
Ready to take your project to the next level? Consider these tips:
- README.md: Write a clear and informative
README.md
file to guide others (and your future self). - Linting and Formatting: Use tools like
flake8
andblack
to keep your code clean and consistent. - Testing: Write tests to catch bugs early and make refactoring a breeze.
- Documentation: Generate beautiful documentation with tools like Sphinx.
- CI/CD: Automate your build and deployment process for a smoother workflow.
Troubleshooting: When Things Go Wrong
Even with the best setup, you might hit a snag. Here are a few common issues:
- "pip is not recognized...": Make sure Python is in your system's PATH.
- "No module named...": Check your virtual environment and
requirements.txt
. - Git errors: Google is your friend! The Python community is incredibly helpful.
Wrapping Up
With a solid project setup, you're well on your way to Python greatness. Remember, organization and planning are key to successful development. Now, go forth and build something amazing!
Subscribe to my newsletter
Read articles from Goran Tomasic directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
