Running Open Source LLM (Llama3) Locally Using Ollama and LangChain
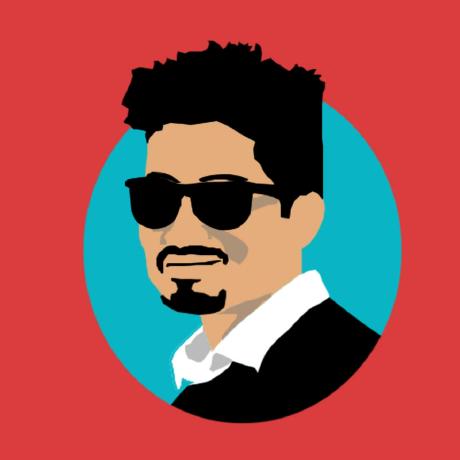
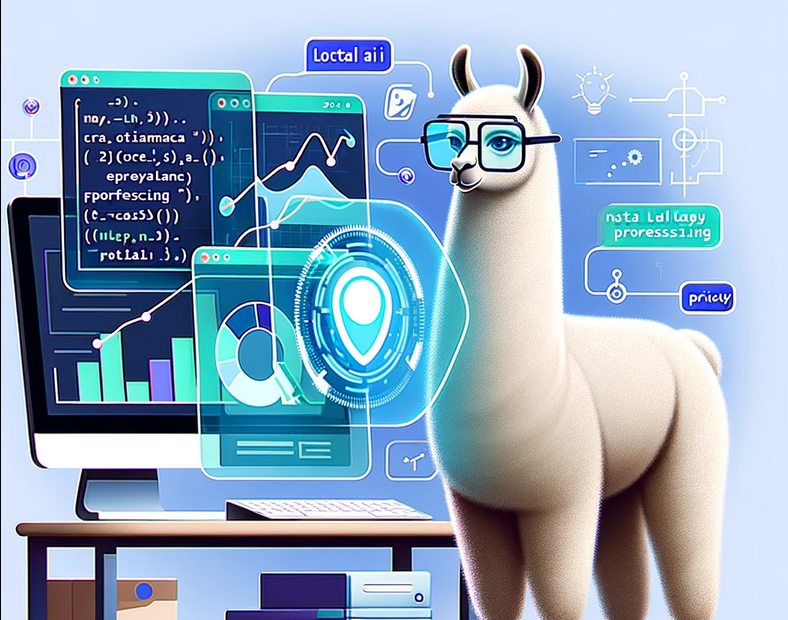
In this post, we will walk through a detailed process of running an open-source large language model (LLM) like Llama3 locally using Ollama and LangChain. We will cover everything from setting up your environment, creating your custom model, fine-tuning it for financial analysis, running the model, and visualizing the results using a financial data dashboard.
1. Setting Up the Environment
To start, we need to set up our development environment. This includes installing necessary dependencies, setting up Python virtual environments, and downloading the Llama3 model using Ollama.
1.1 Install Dependencies
We'll use Python for scripting and various libraries for model management and visualization. Open a terminal and execute the following commands:
# Create a virtual environment
python -m venv llama3_env
source llama3_env/bin/activate # On Windows use `llama3_env\Scripts\activate`
# Install necessary libraries
pip install ollama langchain pandas matplotlib seaborn plotly dash
1.2 Download and Set Up Llama3 Using Ollama
Ollama is a tool designed to simplify the management and deployment of large language models like Llama3. To download and set up Llama3, you need to follow these steps:
Download Ollama: If not already installed, follow Ollama's installation guide for your operating system.
Download Llama3: Use Ollama's CLI to download the Llama3 model.
ollama pull llama3
- Verify Installation: Confirm that Llama3 is correctly set up.
ollama list
This command should list Llama3 among the available models.
2. Getting Started with Ollama and LangChain
2.1 Introduction to Ollama
Ollama is a model deployment platform that helps manage and deploy machine learning models effectively. It abstracts away the complexities of handling large models, making it ideal for ML engineers and data scientists.
2.2 Introduction to LangChain
LangChain is a framework designed to simplify the integration of LLMs into applications. It provides a robust set of tools to build chains of transformations, which are particularly useful when dealing with language models.
2.3 Integrating Llama3 with LangChain
To use Llama3 within LangChain, you need to write a script that loads the model and defines its interaction with data. Below is a basic Python script to get started:
from langchain import LangChain
from ollama import Model
# Initialize LangChain
chain = LangChain()
# Load Llama3 model using Ollama
llama3_model = Model(name="llama3")
# Define a simple chain
chain.add_model(llama3_model)
# Example input to test the setup
input_data = "What are the latest trends in financial technology?"
response = chain.run(input_data)
print(response)
This script initializes a LangChain instance, loads the Llama3 model using Ollama, and runs a sample input through the chain.
3. Building a Custom Model for Financial Analysis
Now that we have set up Llama3 with LangChain, it's time to build a custom model tailored for financial analysis. This section will cover data collection, model fine-tuning, and preparation.
3.1 Project Setup
Create a project directory structure to organize scripts, data, and models.
mkdir llama_financial_analysis
cd llama_financial_analysis
mkdir data models scripts
3.2 Data Collection and Preparation
To fine-tune Llama3 for financial analysis, we need a dataset. You can use publicly available financial data (e.g., stock market data, economic indicators). Here's how to collect and prepare data using Python:
import pandas as pd
import yfinance as yf
# Fetch historical stock price data
tickers = ["AAPL", "GOOGL", "MSFT"]
data = yf.download(tickers, start="2020-01-01", end="2023-01-01")
data.to_csv("data/stock_data.csv")
This script uses the yfinance
library to fetch historical stock prices and saves the data in a CSV file.
3.3 Fine-Tuning Llama3 for Financial Analysis
To fine-tune Llama3, we'll use the prepared financial data. Fine-tuning requires adjusting the model's parameters based on our specific dataset.
from ollama import FineTuner
# Load data
financial_data = pd.read_csv("data/stock_data.csv")
# Initialize FineTuner
tuner = FineTuner(model="llama3", data=financial_data, output_dir="models/finetuned_llama3")
# Fine-tune the model
tuner.fine_tune()
The script above loads financial data and uses Ollama's FineTuner
to fine-tune Llama3. The output is a fine-tuned model stored in the models
directory.
4. Running the Model for Predictions
Once the model is fine-tuned, we can run it to generate predictions. In this example, the model will predict future stock prices based on historical data.
finetuned_model = Model.load("models/finetuned_llama3")
input_data = "Predict the stock price trend for Apple Inc. over the next quarter."
prediction = finetuned_model.predict(input_data)
print(prediction)
This script loads the fine-tuned model and generates a prediction based on the input prompt related to Apple's stock price.
5. Creating a Financial Data Visualization Dashboard
Visualizing the predictions is crucial for understanding trends and making informed decisions. We'll create a dashboard using Dash, a Python framework for building analytical web applications.
5.1 Setting Up the Dashboard Framework
First, install Dash if you haven't already:
pip install dash
Then, create a new Python script (dashboard.py
) to build the dashboard:
import dash
from dash import dcc, html
import plotly.express as px
import pandas as pd
predictions = pd.read_csv("data/predictions.csv")
app = dash.Dash(__name__)
# Define the layout of the dashboard
app.layout = html.Div(children=[
html.H1(children='Financial Analysis Dashboard'),
dcc.Graph(
id='stock-trend',
figure=px.line(predictions, x='Date', y='Predicted Price', title='Stock Price Predictions')
)
])
# Run the app
if __name__ == '__main__':
app.run_server(debug=True)
This script sets up a Dash app that reads the prediction results and visualizes them using a line chart. The predictions are displayed on the dashboard as a Plotly graph.
5.2 Adding Interactive Features
To enhance the user experience, add interactive elements like dropdowns and sliders:
app.layout = html.Div(children=[
html.H1(children='Financial Analysis Dashboard'),
dcc.Dropdown(
id='ticker-dropdown',
options=[
{'label': 'Apple', 'value': 'AAPL'},
{'label': 'Google', 'value': 'GOOGL'},
{'label': 'Microsoft', 'value': 'MSFT'}
],
value='AAPL'
),
dcc.Graph(id='stock-trend')
])
@app.callback(
dash.dependencies.Output('stock-trend', 'figure'),
[dash.dependencies.Input('ticker-dropdown', 'value')]
)
def update_graph(selected_ticker):
filtered_data = predictions[predictions['Ticker'] == selected_ticker]
figure = px.line(filtered_data, x='Date', y='Predicted Price', title=f'Stock Price Predictions for {selected_ticker}')
return figure
The dashboard now includes a dropdown menu to select different tickers, dynamically updating the displayed graph based on user input.
6. Deployment and Testing
6.1 Local Testing
Run the dashboard locally to ensure all functionalities work as expected:
python dashboard.py
Visit http://127.0.0.1:8050
in your web browser to interact with the dashboard.
6.2 Deployment Options
Consider deploying your dashboard to a cloud platform for public access:
Heroku: Deploy Dash apps on Heroku.
AWS: Use AWS services like Elastic Beanstalk for deployment.
Docker: Containerize your app for easier deployment across different environments.
6.3 Monitoring and Maintenance
Regularly update the model with new financial data for accurate predictions.
Monitor the dashboard's performance and user interactions to identify areas for improvement.
7. Conclusion
In this guide, we covered setting up a local environment to run a large language model using Ollama and LangChain, fine-tuning the model, and visualizing the results through an interactive dashboard. This tutorial provides a solid foundation for leveraging LLMs in specialized fields such as finance, enabling data scientists and backend engineers to explore the potential of advanced AI models.
Troubleshooting Tips
Ensure all dependencies are correctly installed and environment variables are configured.
Check model compatibility and versioning when integrating with LangChain.
This tutorial provides a comprehensive end-to-end guide for implementing LLM-based financial analysis using Python, Ollama, and LangChain, complete with data visualization. By following this guide, you can extend the capabilities of LLMs in your own projects and fields of expertise.
For more such guides and tutorials, visit AhmadWKhan.com.
Happy Coding!
Subscribe to my newsletter
Read articles from Ahmad W Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
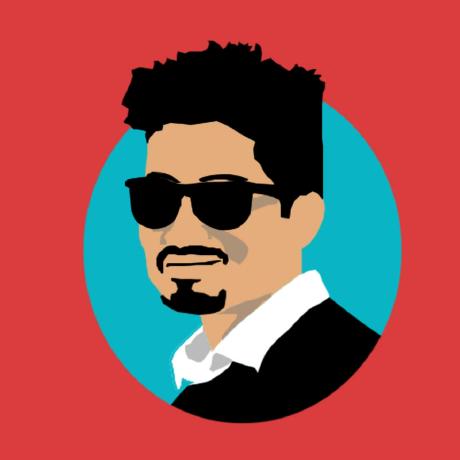