Learning Python for Devops day -1
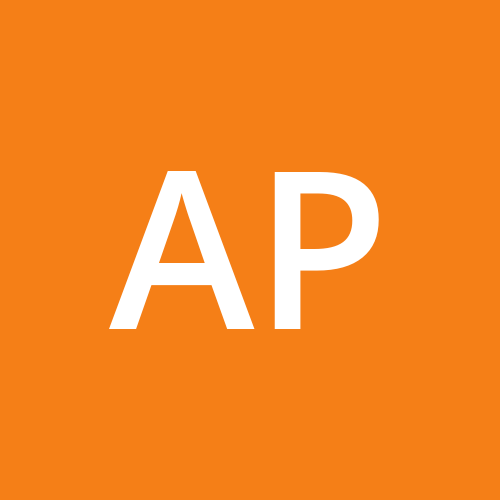
Hello everyone, this is Abhishek Pol, and welcome to my first blog about learning AWS DevOps. I started my journey around two months ago, and I believe it's never too late to share your experiences. Without further ado, let me share what I learned today.
What is Python Python?
Python is an interpreted, object-oriented, high-level programming language. Its simple and easy-to-learn syntax makes it easy to read and maintain. Python supports modules and packages, which help in organizing code and reusing it. The Python interpreter and its large standard library are free to use and available for all major platforms.
Pre-requisites
Indentation
Declaration of variable not required (automatically derive the data type)
Python Shell
We can use any of the following Python Shells to understand this language:
Python Online Shell
- Navigate to Python Online Shell in your browser: https://www.python.org/shell/
Login to EC2 and Install Python3 using yum
Login to your EC2 instance.
Install Python3 using the following command:
sudo yum install python3
To open the Python Interpreter, enter:
python3
Values and Types
A value is one of the basic things a program works with, like a letter or a number.
To get the type of a value, you can use the
type()
function in Python:>>> type('Hello, World!') <class 'str'> >>> type(17) <class 'int'> >>> type('17') <class 'str'> >>> type(3.2) <class 'float'> >>> type('3.2') <class 'str'>
This is similar to the
echo
command in bash:print("Hello, World!")
FYI: Always remember, in the above example, whatever we write inside the print brackets gets printed when we execute the command. If we want to check the type, for example, if it's an integer or a string (integer means numbers and string means letters or alphabets), we use the type() command. Inside the brackets, whatever we write, it shows its type.
variables
Variables are used to store values. An assignment statement creates new variables and gives them values:
# Assigning a value to a variable msg = "Hello world!" # Checking the type of the variable print(type(msg)) # Printing the value of the variable print(msg)
Concatenation (combining strings):
# Assigning values to variables first = 'AWS' last = 'Devops' # Combining strings with a space in between full_name = first + ' ' + last # Printing the combined string print(full_name)
FYI: Concatenation is just combining strings, like making a sentence. In the example above, you can see we assigned values to two variables, and each variable holds a string because it contains letters. To join the two words, we use the
+
symbol. You can also see' '
in the code, which adds a space between the two words. So, for anyone reading this blog, here's a little homework: try printingfull_name
and see the output. Keep experimenting with more words to understand it better!Comments
in Python start with the
#
symbol. This comment contains useful information that is not in the code:# Assigning a value to the variable with a comment v = 5 # velocity in meters/second # Printing the value of the variable print(v)
Boolean Expressions
A boolean expression is an expression that evaluates to either true or false. The following examples use the
==
operator, which compares two operands and returnsTrue
if they are equal andFalse
otherwise:>>> 5 == 5 True >>> 5 == 6 False
True
andFalse
are special values that belong to the typebool
; they are not strings:>>> type(True) <class 'bool'> >>> type(False) <class 'bool'>
The
==
operator is one of several relational operators. Here are the others:x != y
:x
is not equal toy
x > y
:x
is greater thany
x < y
:x
is less thany
x >= y
:x
is greater than or equal toy
x <= y
:x
is less than or equal toy
FYI
Interpreter vs Compiler
Interpreter | Compiler |
Translates program one statement at a time. | Scans the entire program and translates it as a whole into machine code. |
Takes less time to analyze the source code, but overall execution time is slower compared to compilers. | Takes more time to analyze the source code, but overall execution time is faster compared to interpreters. |
No intermediate object code is generated, making it memory efficient. | Generates intermediate object code which requires linking, thus needing more memory. |
Used by programming languages like Python, JavaScript, Ruby. | Used by programming languages like C, C++, Java. |
Python and Java
Java
// Your First Program
class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World inside main function");
}
// This line will cause a compilation error because it's outside the main function
// System.out.println("Hello, World! outside main function");
}
To install Java, use:
sudo yum install java-devel
If you have Java installed on your machine/server, you can execute:
javac HelloWorld.java
to compile the Java code. This will create a HelloWorld.class
file. The program can be executed with:
java HelloWorld
Python
Python follows an indentation approach and does not use curly brackets.
# Example of an if-else statement in Python
x = 10
if x > 5:
print("x is greater than 5")
else:
print("x is not greater than 5")
# This line is outside the if-else block
print("This line is outside the if-else block")
Key Differences:
Java uses curly brackets
{}
to define code blocks.Python uses indentation to define code blocks.
Java requires a main function to execute code.
Python executes code directly without the need for a main function.
NOTE: Always remember, when using if
or any condition in Python, you must use a colon :
at the end of the statement. For indentation, use the Tab key, not the space bar. This ensures your output prints correctly. If you nest another loop or condition inside an if
statement, you need to use two tabs to avoid any indentation errors.
Conditional Execution
Conditional statements allow us to write useful programs by checking conditions and changing the behavior of the program accordingly. The simplest form is the if
statement:
x = 5
if x > 0:
print('x is positive')
The boolean expression after if
is called the condition. If it is true, then the indented statement gets executed. If not, nothing happens.
Another example of if
:
age = 21
if age >= 18:
print("You can vote!")
If-elif-else Statements
age = 21
if age < 4:
ticket_price = 0
elif age < 18:
ticket_price = 10
else:
ticket_price = 15
print("Ticket price is", ticket_price)
Steps to Run the Code
Create a file named
ticket_
price.py
.Write the above code in the file.
Run it with the command:
python ticket_price.py
FYI: When writing a script in Linux, we use the
vi
command. Similarly, in Python, we usevi
followed by the name of the script we want to execute, ending with.py
. After writing your script, use:wq
to save and exit. On an AWS EC2 Python server, use the name you assigned, like in the example above (ticket_price.py
). In Linux, we usebash
or./
to execute commands. Here, we usepython
orpython3
depending on which version of Python you have installed.
Lists
A list stores a series of items in a particular order. You can access items using an index or within a loop. Lists are mutable, meaning you can change their content. In a list, the first value is at index 0.
Example of List Operations
# Creating a list of bikes
bikes = ['Apache', 'Suzuki', 'Pulsar']
# Modifying an item in the list
bikes[1] = 'BMW'
# Checking the type of the list
print(type(bikes))
# Getting the first item in the list
first_bike = bikes[0]
print(first_bike)
# Attempting to access an out-of-range index
# This will raise an IndexError
# bikes[3]
# Getting the last item in the list
last_bike = bikes[-1]
print(last_bike)
# Getting the length of the list
print(len(bikes))
# Iterating through the list
for bike in bikes:
print(bike)
Adding Items to a List
# Creating an empty list
bikes = []
# Adding items to the list
bikes.append('Apache')
bikes.append('Suzuki')
bikes.append('Pulsar')
# Checking the length of the list
print(len(bikes))
Slicing a List
# Creating a list of AWS topics
aws_topics = ['ec2', 's3', 'rds', 'lambda']
# Slicing the list to get the first two items
first_two = aws_topics[:2]
print(first_two)
# Creating a copy of the list
aws_topics_copy = aws_topics[:]
print(aws_topics_copy)
# Various slicing examples
print(aws_topics[:]) # ['ec2', 's3', 'rds', 'lambda']
print(aws_topics[0:1]) # ['ec2']
print(aws_topics[0:2]) # ['ec2', 's3']
print(aws_topics[:2]) # ['ec2', 's3']
print(aws_topics[1:3]) # ['s3', 'rds']
Summary
Lists are mutable and can store a series of items.
Access items using an index, starting from 0.
Use slicing to get a subset of the list.
Use loops to iterate through the list.
Use
append()
to add items to the list.Use
len()
to get the length of the list.
Tuples
Tuples are immutable. A tuple is a sequence of values. The values can be of any type, and they are indexed by integers, so in that respect, tuples are a lot like lists. Syntactically, a tuple is a comma-separated list of values:
>>> t = 'a', 'b', 'c', 'd', 'e'
Although it is not necessary, it is common to enclose tuples in parentheses:
>>> t = ('a', 'b', 'c', 'd', 'e')
Another way to create a tuple is by using the built-in function tuple()
. With no argument, it creates an empty tuple:
>>> t = tuple()
>>> print(t)
()
You can also create a tuple with values:
>>> t = ('a', 'b', 'c', 'd', 'e')
>>> print(t[0])
'a'
>>> print(t[1:3])
('b', 'c')
But if you try to modify one of the elements of the tuple, you get an error:
>>> t[0] = 'A'
TypeError: 'tuple' object does not support item assignment
Thank you so much for reading my first blog! I hope you learned a lot with me, and yes, keep learning and keep motivating everyone. Have a wonderful day!
Subscribe to my newsletter
Read articles from Abhishek Pol directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
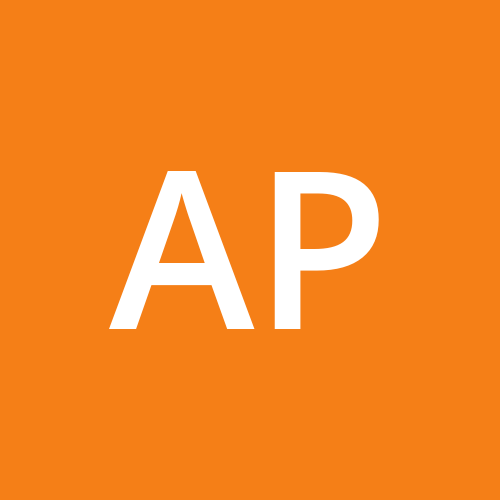