The Art of Building Scalable Backend Architectures
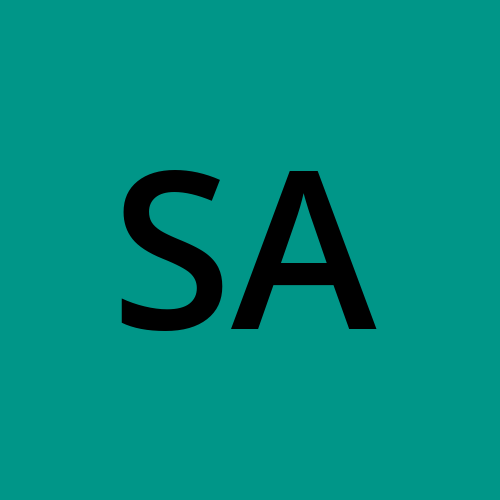
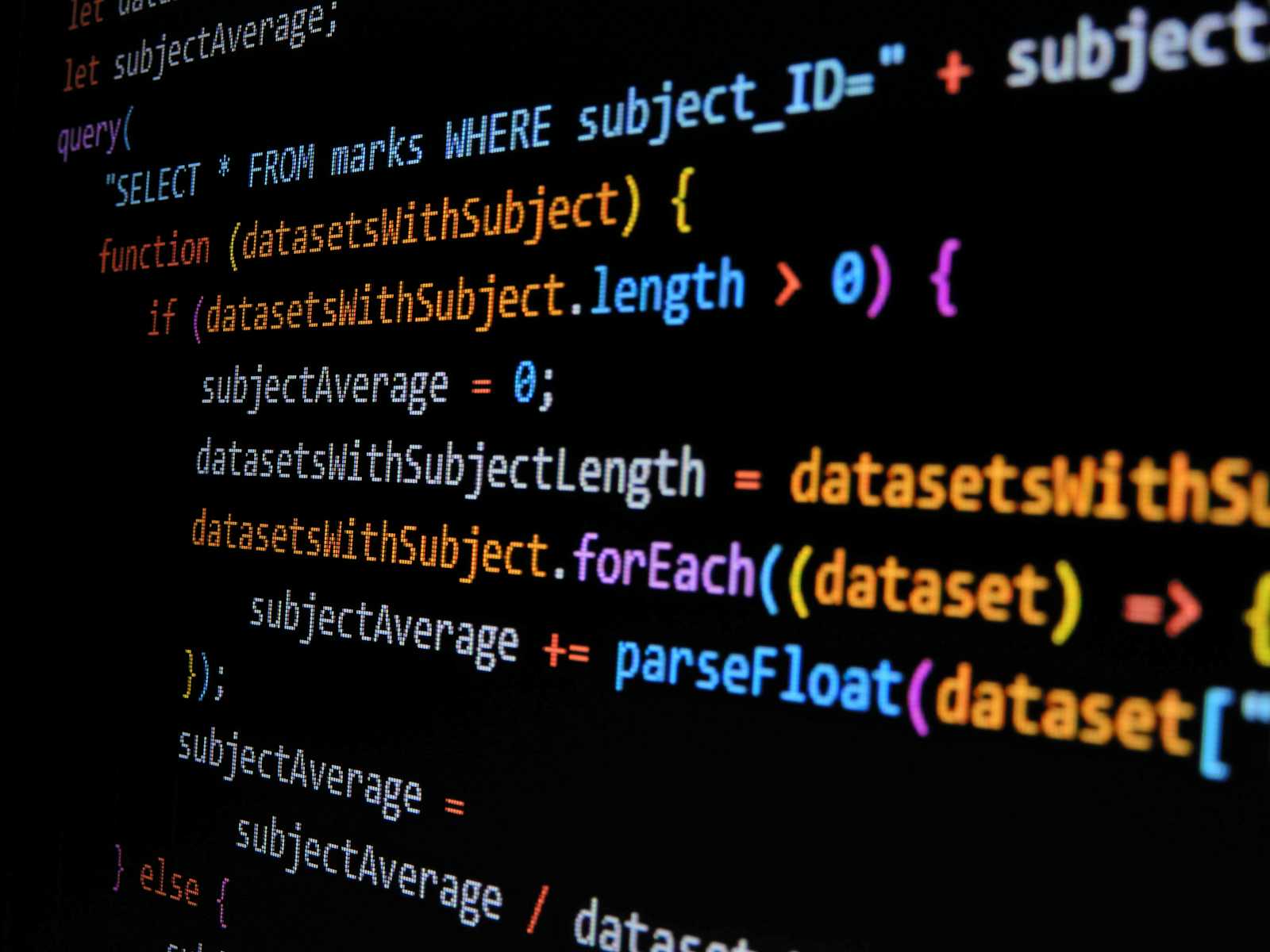
Understanding Backend Architecture
At its core, backend architecture refers to the structure and organization of the server-side components of an application. This includes databases, servers, APIs, and other services that interact to process requests, store data, and deliver responses to the client. The goal of a robust backend architecture is to ensure that these components work together efficiently, even as the application scales to accommodate more users and data.
Choosing the Right Architecture: Monolith vs. Microservices
One of the first decisions you’ll make when designing a backend is whether to go with a monolithic or microservice architecture. Each has its pros and cons
Monolithic Architecture: This is a single, unified codebase where all components are tightly coupled. Monolithic architectures are simpler to develop and deploy, making them a good choice for small to medium-sized applications. However, as the application grows, a monolithic architecture can become cumbersome, leading to longer deployment times and more complex codebases.
Microservices Architecture: In a microservices architecture, the application is broken down into small, independent services that communicate with each other via APIs. This approach offers greater flexibility and scalability, as each service can be developed, deployed, and scaled independently. However, managing a microservices architecture requires more sophisticated tools and practices, such as containerization, service discovery, and distributed tracing.
Database Design: Relational vs. NoSQL
The database is the backbone of any backend architecture. Choosing the right type of database is critical to ensuring the performance and scalability of your application.
Relational Databases: Relational databases like MySQL, PostgreSQL, and Oracle DB are well-suited for applications with structured data and complex queries. They enforce ACID (Atomicity, Consistency, Isolation, Durability) properties, making them reliable for transactions that require high data integrity.
NoSQL Databases: NoSQL databases like MongoDB, Cassandra, and Redis are designed for unstructured or semi-structured data. They offer greater flexibility and scalability, particularly for applications with large amounts of data or high read/write operations. NoSQL databases are often used in conjunction with relational databases in a polyglot persistence architecture.
API Design: REST vs. GraphQL
APIs are the glue that connects your backend to the frontend and other services. When designing an API, you’ll typically choose between REST and GraphQL:
REST (Representational State Transfer): REST is the most common API design pattern, offering a simple and predictable way to structure APIs. REST APIs are based on standard HTTP methods (GET, POST, PUT, DELETE) and are ideal for applications where simplicity and ease of use are priorities.
GraphQL: GraphQL is a query language for APIs that allows clients to request exactly the data they need. This can reduce over-fetching and under-fetching of data, making GraphQL a powerful tool for complex applications with diverse data requirements. However, GraphQL can introduce additional complexity in terms of query optimization and caching.
Security: Protecting Your Backend
Security is a critical consideration in backend development. A single vulnerability can expose your entire application to attacks. Here are some key security practices:
Authentication and Authorization: Implement strong authentication mechanisms such as OAuth2, JWT (JSON Web Tokens), or multi-factor authentication (MFA) to ensure that only authorized users can access your application.
Input Validation and Sanitization: Always validate and sanitize user inputs to prevent common security threats like SQL injection and cross-site scripting (XSS).
Encryption: Use encryption to protect sensitive data both at rest and in transit. This includes using HTTPS for all communications between clients and servers.
Regular Security Audits: Perform regular security audits and code reviews to identify and address potential vulnerabilities.
Scaling Your Backend
As your application grows, your backend needs to scale to handle increased traffic and data. Here are some strategies for scaling your backend:
Horizontal Scaling: Horizontal scaling involves adding more servers to handle increased load. This is often done using loadbalancers to distribute traffic across multiple servers.
Vertical Scaling: Vertical scaling involves adding more resources (CPU, RAM) to your existing servers. While simpler to implement, vertical scaling has limits and can be more costly in the long run.
Caching: Implement caching strategies to reduce the load on your backend. Caching can be done at various levels, including the database (e.g., using Redis or Memcached), API responses, and static assets.
Asynchronous Processing: Offload long-running tasks to background workers using message queues like RabbitMQ or Kafka. This allows your backend to handle more requests without getting bogged down by time-consuming processes.
Conclusion
Building a scalable backend architecture is both an art and a science. It requires careful planning, the right choice of tools and technologies, and a deep understanding of your application’s requirements. By adhering to best practices in architecture, database design, API development, security, and scaling, you can create a backend that not only meets today’s needs but is also ready for tomorrow’s challenges. Whether you’re just starting out or are a seasoned developer, mastering the intricacies of backend development is a journey worth taking. The rewards are not just in the code you write but in the powerful, reliable, and scalable applications you create.
Subscribe to my newsletter
Read articles from Sabbir Ahmed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
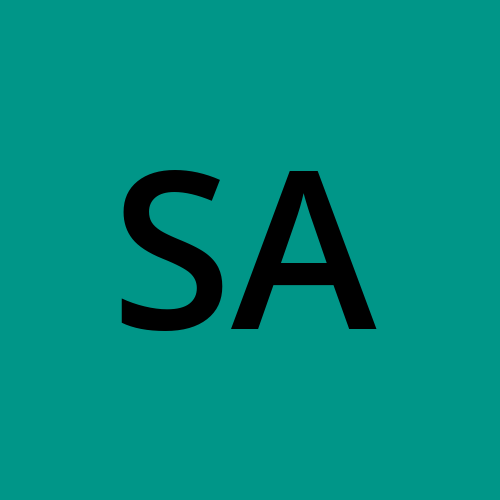