Implementing Infinite Scroll in a Blog with JavaScript and Intersection Observer
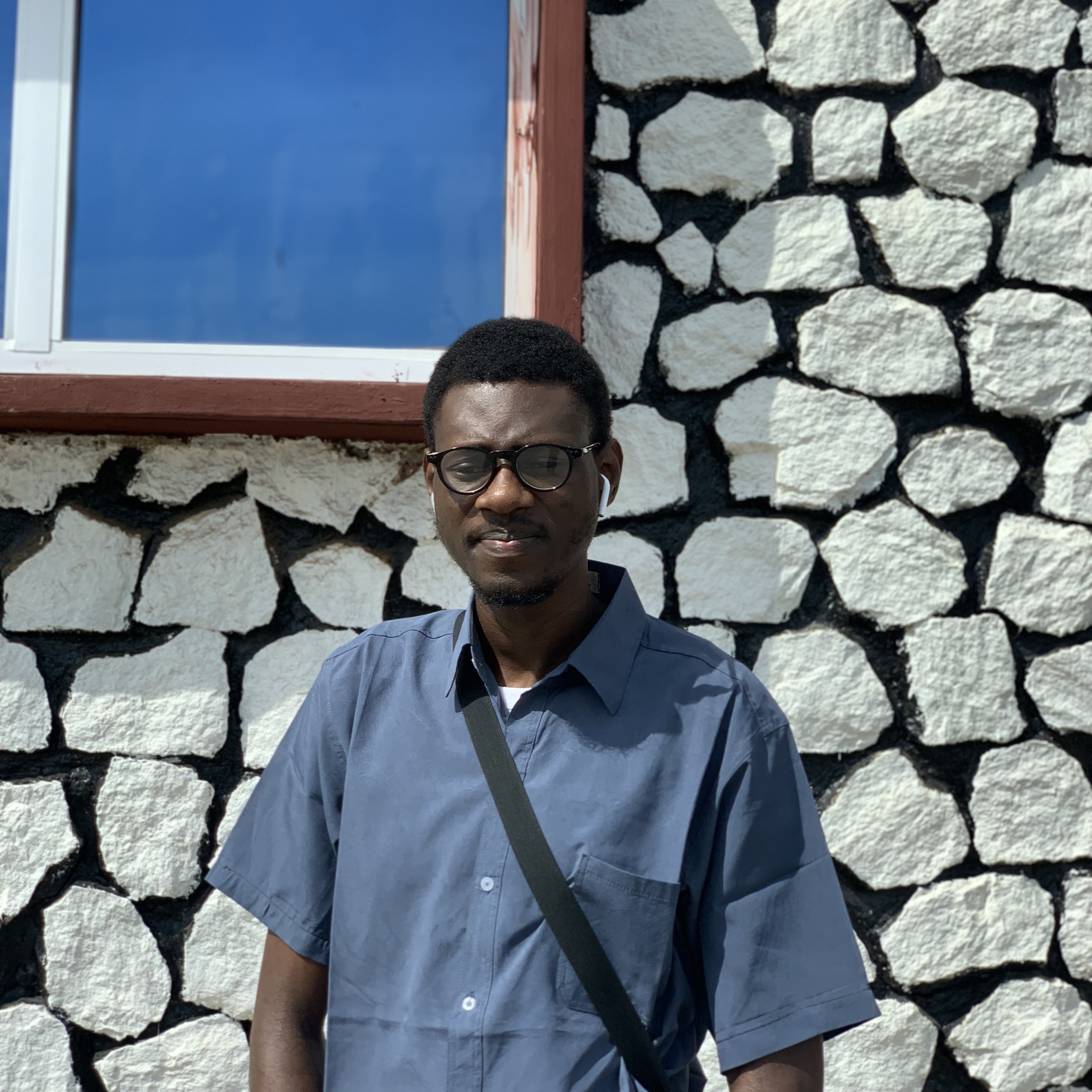
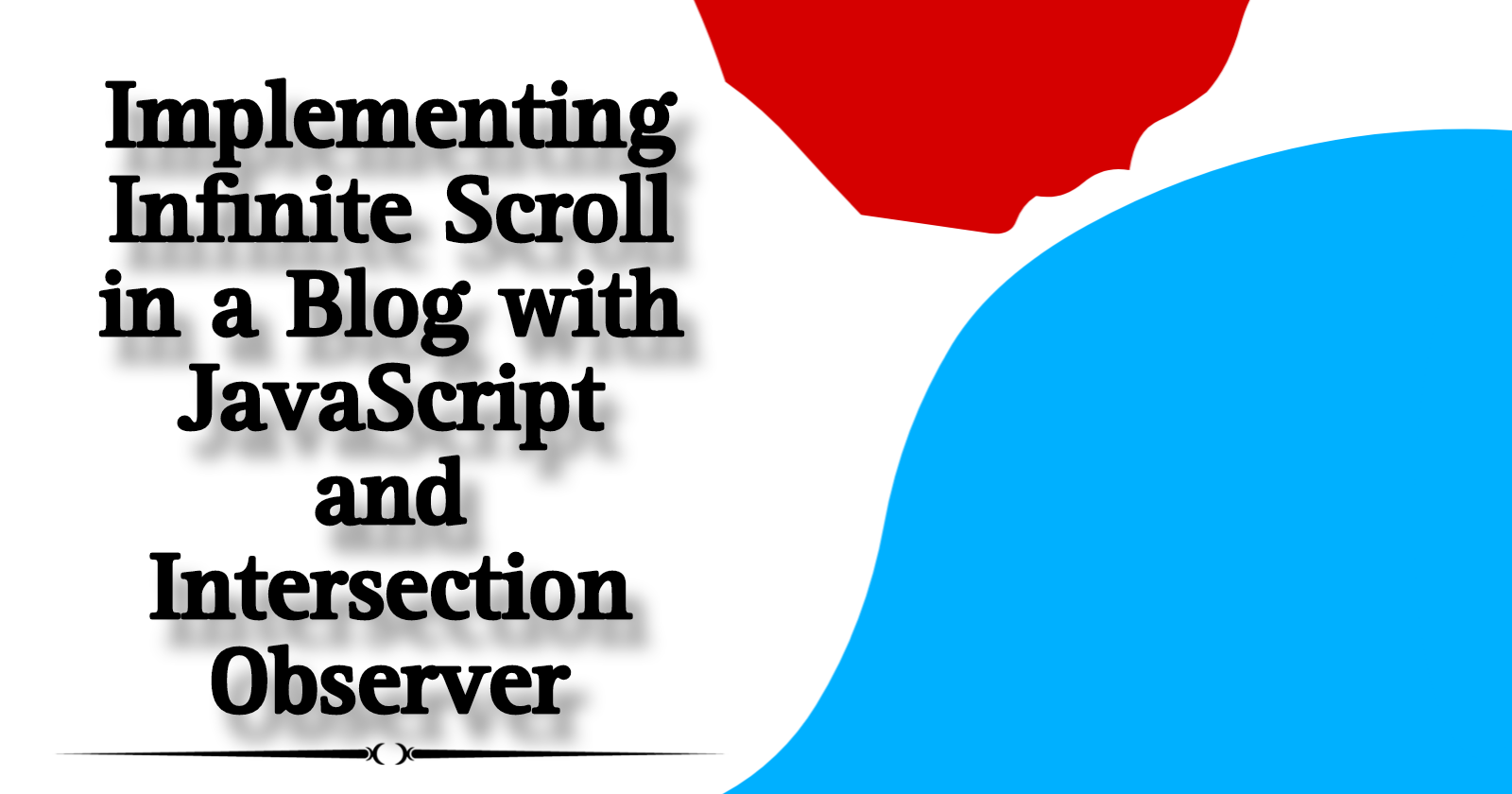
Implementing Infinite Scroll in a Blog with JavaScript and Intersection Observer
Introduction:
Alright, let's talk about one of the coolest tricks you can pull off in web development: Infinite Scroll. Imagine you’re strolling through an endless buffet; every time you take a bite, another dish magically appears. That’s essentially what infinite scroll does for a blog—constantly feeding your users with fresh content without them lifting a finger (or clicking a button). In this guide, we'll touch on how to implement this feature using plain JavaScript and the powerful Intersection Observer API.
But why bother with infinite scroll, you ask? Well, user experience (UX) is king in the digital world. The easier it is for your readers to access and understand content, the longer they’ll stick around. Plus, it’s just plain cool. And who doesn’t want their blog to be the coolest spot on the internet?
Pre-requisites:
Before we jump in, make sure you’ve got the following ready:
- A code editor like Visual Studio Code or Sublime Text
- Basic knowledge of HTML, CSS, and JavaScript
- A blog or webpage setup where you want to implement infinite scroll Now, things are about to get interesting.
Setting the Stage: The HTML Structure
First, let’s set up the basic HTML structure of our blog page. We need a container to hold the blog posts, and a loader to indicate when new content is being fetched.
<div class="blog-container">
<!-- Blog posts will be appended here -->
</div>
<div class="loader" id="loader">Loading more posts...</div>
This setup is simple but effective. The .blog-container
div will hold our blog posts and the .loader
div will give users a visual cue that more content is on the way.
Styling the Scroll: The CSS
Now that we have the HTML structure, let’s make it look decent. We’ll add some basic CSS to style our blog posts and the loader.
.blog-container {
width: 80%;
margin: 0 auto;
padding: 20px;
}
.blog-post {
background-color: #f4f4f4;
padding: 20px;
margin-bottom: 20px;
border-radius: 5px;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1);
}
.loader {
text-align: center;
font-size: 20px;
margin: 20px 0;
display: none; /* Hide initially */
}
The .blog-post
class gives our blog entries a clean, minimalist look, while the .loader
is kept hidden until it’s needed. Now, things are starting to take shape.
Adding Life: JavaScript and Intersection Observer
Here’s where the magic happens. We’ll use JavaScript to dynamically load more content as the user scrolls. The Intersection Observer API will help us detect when the loader enters the viewport, signaling that it’s time to fetch more posts.
// Get references to our elements
const by blog container document.querySelector('.blog-container');
const loader = document.getElementById('loader');
let currentPage = 1;
let isLoading = false;
// Function to simulate fetching posts
const fetchPosts = (page) => {
isLoading = true;
loader. style.display = 'block';
setTimeout(() => {
for (let i = 1; i <= 5; i++) {
const post = document.createElement('div');
post.className = 'blog-post';
post.innerHTML = `<h2>Post ${((page - 1) * 5) + i}</h2><p>This is the content of post ${(page - 1) * 5 + i}.</p>`;
blogContainer.appendChild(post);
}
loader.style.display = 'none';
isLoading = false;
}, 1000);
};
// Intersection Observer callback
const handleIntersection = (entries) => {
if (entries[0].isIntersecting && !isLoading) {
currentPage++;
fetchPosts(currentPage);
}
};
// Create an observer instance
const observer = new IntersectionObserver(handleIntersection);
observer.observe(loader);
// Initial load
fetchPosts(currentPage);
Let’s break this down. We start by grabbing references to our .blog-container
and #loader
elements. We also set up a few variables: currentPage
to track the current page number, and isLoading
to prevent multiple fetches at once.
The fetchPosts
function simulates an API call to fetch more posts. In reality, you’d replace this with a real API request. The setTimeout
function delays the process to mimic network latency, giving us time to show the loader.
The Intersection Observer API does the heavy lifting. We create an observer and pass it a callback function (handleIntersection
). This function checks if the loader is visible (isIntersecting
) and whether we’re currently loading more posts. If both conditions are met, we increment the page number and fetch more posts.
Now, I think things are getting clearer now—you're seeing how each piece fits together to create a seamless experience for your users. Isn't this amazing?
Testing and Fine-Tuning
By now, you should have a functional infinite scroll. But it’s crucial to test thoroughly. Make sure the scroll works smoothly on different devices and browsers. Also, consider adding error handling for situations where the API might fail or the user has no internet connection.
Conclusion:
Infinite scroll isn't just a fancy feature; it’s a way to keep your audience engaged without interrupting their experience. By using the Intersection Observer API, we’ve implemented a solution that’s both efficient and elegant. As Antoine de Saint-Exupéry once said, “Perfection is achieved not when there is nothing more to add, but when there is nothing left to take away.” That’s the beauty of infinite scroll—just the right amount of content at the right time.
Now that you've got this down, what's stopping you from implementing it in your next project?!
Subscribe to my newsletter
Read articles from Modefoluwa Adeniyi-Samuel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
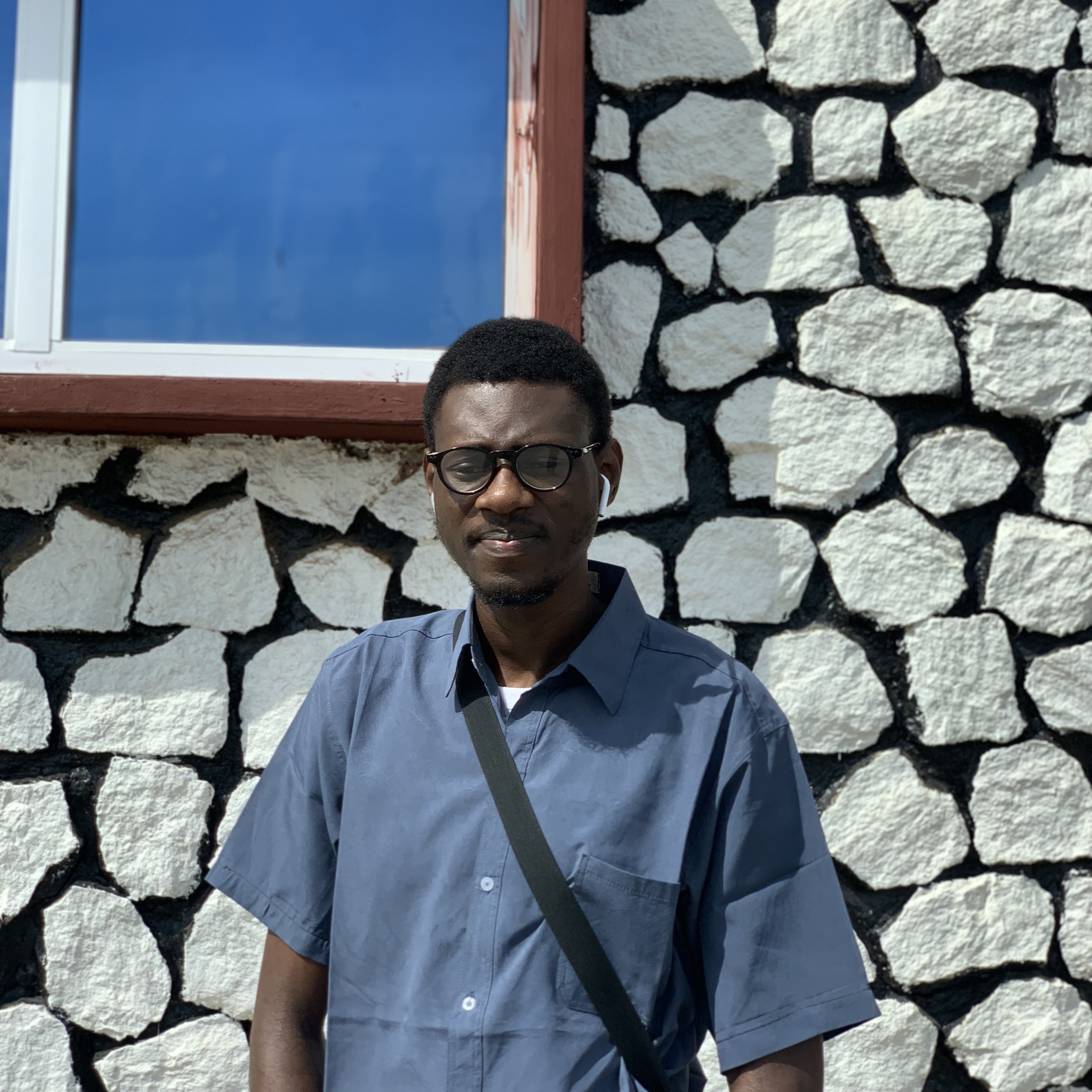
Modefoluwa Adeniyi-Samuel
Modefoluwa Adeniyi-Samuel
Hi there! I'm Modefoluwa, a committed technical writer, Web3 enthusiast, and a Computer Engineering student. With a knack for simplifying complex concepts, I specialize in creating tutorials, guides, and articles that empower developers and non-technical users alike. As a learning full-stack developer, I’m actively expanding my technical skill set to better understand the technologies I write about. My journey started with a love for storytelling, which evolved into crafting impactful content for the ever-evolving tech landscape. When I’m not writing or coding, you’ll find me exploring emerging technologies, brainstorming creative ideas, or engaging with communities that share my love for innovation. My goal? To bridge the gap between technology and its users through clear, concise, and engaging communication - cheers.