How to Build a Multi-Step Form with Vanilla JavaScript
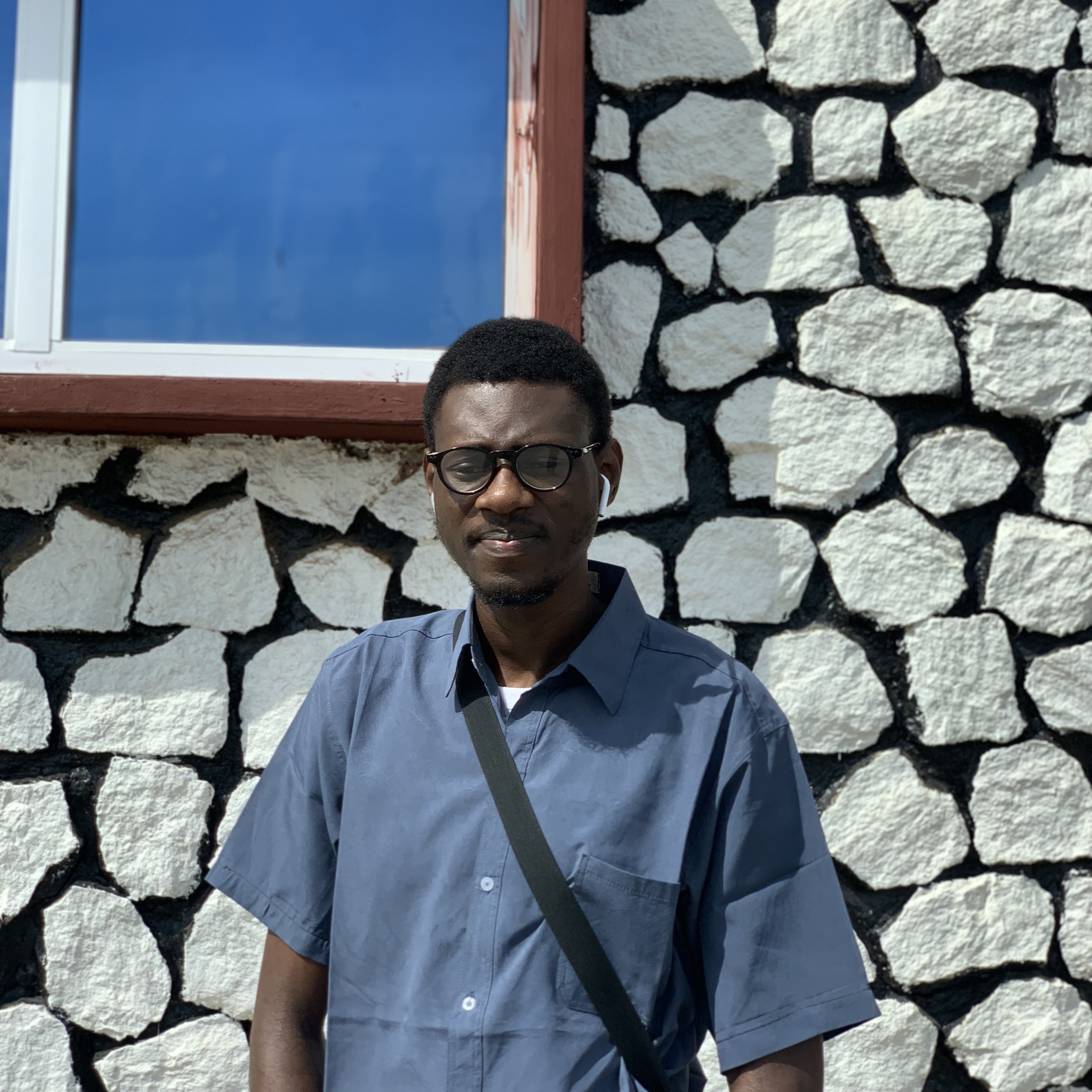
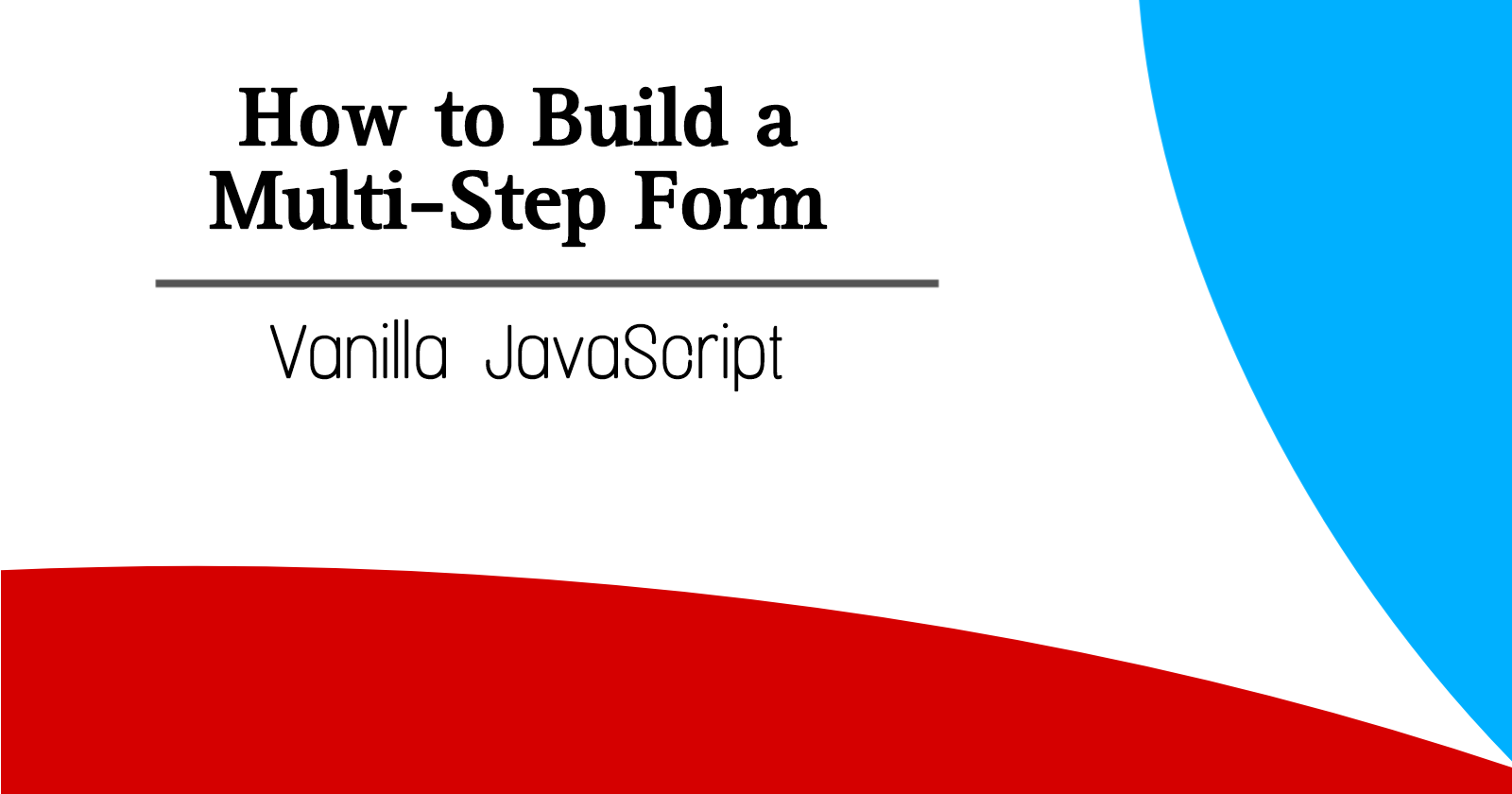
How to Build a Multi-Step Form with Vanilla JavaScript
Hello there, today I will be sharing my little knowledge in building a multi-step form in raw JavaScript.
A smooth, engaging interface not only keeps users on your site but also guides them through complex tasks with ease. I would say a multi-step form is a powerful tool to collect data in bite-sized chunks. Unlike a single, overwhelming form, yes, a multi-step form walks users through each step, reducing immobility and improving completion rates. Today, we'll dive deep into building one from scratch using nothing but vanilla JavaScript.
Purpose: Different people with diverse purposes,but one thing I am sure of is at the end of this guide, you'll understand how to create a multi-step form and also grasp the underlying logic that makes it all work seamlessly.
Prerequisites
Before we get our hands dirty with code, ensure you have the following in place:
- A basic understanding of HTML, CSS, and JavaScript.
- A code editor like Visual Studio Code.
- A modern web browser to view your work in action.
- A touch of curiosity, consistency, and patience.
Setting Up the HTML Structure
The backbone of any form is its HTML structure. We’ll start by laying out the basic form fields and the progress tracker that will guide users through the steps.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Multi-step form</title>
<link rel='stylesheet' href='https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css'>
<link rel='stylesheet' href='https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.0.3/css/font-awesome.css'>
</head>
<body>
<div class="container-fluid">
<div class="row justify-content-center">
<div class="col-11 col-sm-10 col-md-10 col-lg-6 col-xl-5 text-center p-0 mt-3 mb-2">
<div class="card px-0 pt-4 pb-0 mt-3 mb-3">
<h2 id="heading">Sign Up Your User Account</h2>
<p>Fill all form fields to go to the next step</p>
<form id="form">
<!-- progressbar -->
<ul id="progressbar">
<li class="active" id="account"><strong>Account</strong></li>
<li id="personal"><strong>Personal</strong></li>
<li id="payment"><strong>Image</strong></li>
<li id="confirm"><strong>Finish</strong></li>
</ul>
<!-- form fields here -->
</form>
</div>
</div>
</div>
</div>
</body>
</html>
Don't be scared by the lengthy the HTML snippet, it basically lays out the structure, including a progress bar at the top that visually indicates the user's position in the form. Each form field will reside within a <fieldset>
, which we’ll toggle on and off as the user navigates through the steps.
Styling the Form with CSS
With the structure in place, it’s time to make the form visually appealing. The following CSS ensures that our form not only looks good but also functions well across different screen sizes(it facilitates its responsiveness)
*{
margin: 0;
padding: 0;
}
html{
height: 100%;
}
#heading{
text-transform: uppercase;
color: #3a85b7;
font-weight: normal;
}
#msform{
text-align: center;
position: relative;
margin-top: 20px;
}
#msform fieldset{
background: white;
border: 0 none;
border-radius: 0.5rem;
width: 100%;
margin: 0;
padding-bottom: 20px;
}
#msform .action-button{
width: 100px;
background: #3ab7a0;
font-weight: bold;
color: white;
cursor: pointer;
padding: 11px 6px;
margin: 11px 0px 10px 6px;
float: right;
}
.card {
border: none;
position: relative;
}
.fs-title {
font-size: 25px;
color: #3ab7a0;
margin-bottom: 15px;
font-weight: normal;
text-align: left
}
#progressbar {
margin-bottom: 30px;
overflow: hidden;
color: darkgrey
}
#progressbar .active {
color: #3ab7a0
}
.progress-bar {
background-color:#3ab7a0
}
The styles above control the appearance of the progress bar, the form fields, and the navigation buttons. Combining “modern” design principles with functional CSS ensures that users won’t just complete the form—they’ll enjoy the process.
Adding Functionality with JavaScript
Now comes the brain of our form—JavaScript. The JavaScript code will control the form’s navigation, ensuring users move from one step to the next smoothly.
$(document).ready(function(){
var current_fs, next_fs, previous_fs; //fieldsets
var opacity;
var current = 1;
var steps = $("fieldset").length;
setProgressBar(current);
$(".next").click(function(){
current_fs = $(this).parent();
next_fs = $(this).parent().next();
//Add Class Active
$("#progressbar li").eq($("fieldset").index(next_fs)).addClass("active");
//show the next fieldset
next_fs.show();
//hide the current fieldset with style
current_fs.animate({opacity: 0}, {
step: function(now) {
opacity = 1 - now;
current_fs.css({
'display': 'none',
'position': 'relative'
});
next_fs.css({'opacity': opacity});
},
duration: 500
});
setProgressBar(++current);
});
$(".previous").click(function(){
current_fs = $(this).parent();
previous_fs = $(this).parent().prev();
//Remove class active
$("#progressbar li").eq($("fieldset").index(current_fs)).removeClass("active");
//show the previous fieldset
previous_fs.show();
//hide the current fieldset with style
current_fs.animate({opacity: 0}, {
step: function(now) {
opacity = 1 - now;
current_fs.css({
'display': 'none',
'position': 'relative'
});
previous_fs.css({'opacity': opacity});
},
duration: 500
});
setProgressBar(--current);
});
function setProgressBar(curStep){
var percent = parseFloat(100 / steps) * curStep;
percent = percent.toFixed();
$(".progress-bar").css("width",percent+"%")
}
});
This JavaScript code manages the logic for moving between the different steps of the form. It controls the animation for hiding and showing each step, updates the progress bar, and ensures that the form is both functional and user-friendly.
Understand Better: Look at it this way, think of this form as navigating a well-designed building. Each room (or step) is marked, and as you move through the rooms, the building’s map (our progress bar) updates to show you where you are. Just like in a building, you can always go back if you missed something in a previous room.
Now I think things are getting clearer now: the code snippets work in unison to deliver a smooth, elegant user experience. By breaking the form into manageable pieces, users feel less overwhelmed, and your form completion rates could see a noticeable improvement.
Conclusion
Creating a multi-step form with vanilla JavaScript isn’t just about gathering user data—it’s about enhancing the user experience by making the process as painless as possible. By following the steps laid out in this guide, you should be able to craft forms that not only work well but also look great and keep users engaged.
“Good design is obvious. Great design is transparent.” — Joe Sparano
In the end, remember that the best forms are the ones that users barely notice—because they just work.
And with that, you’re all set! Go forth and build something exceptional.
Subscribe to my newsletter
Read articles from Modefoluwa Adeniyi-Samuel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
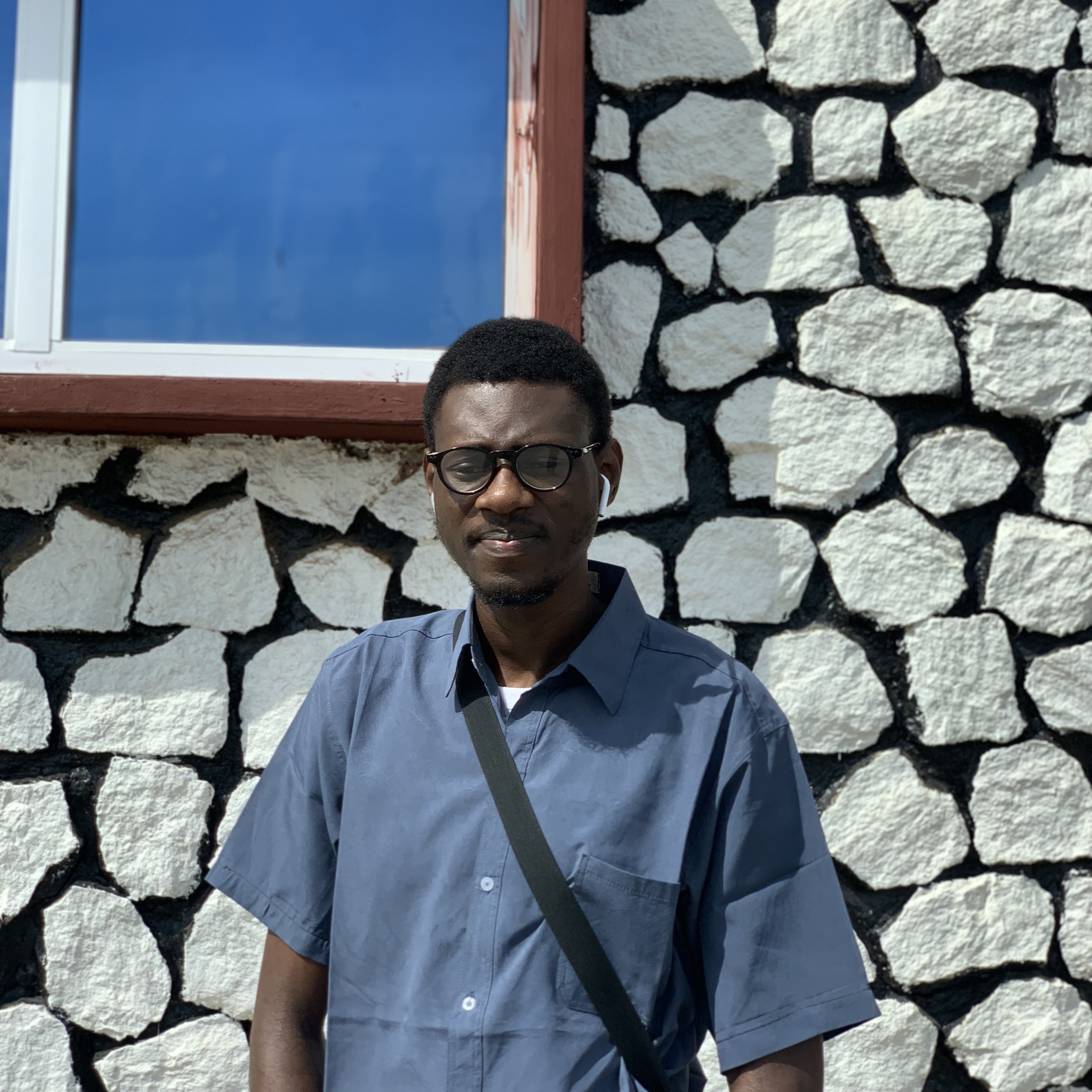
Modefoluwa Adeniyi-Samuel
Modefoluwa Adeniyi-Samuel
Hi there! I'm Modefoluwa, a committed technical writer, Web3 enthusiast, and a Computer Engineering student. With a knack for simplifying complex concepts, I specialize in creating tutorials, guides, and articles that empower developers and non-technical users alike. As a learning full-stack developer, I’m actively expanding my technical skill set to better understand the technologies I write about. My journey started with a love for storytelling, which evolved into crafting impactful content for the ever-evolving tech landscape. When I’m not writing or coding, you’ll find me exploring emerging technologies, brainstorming creative ideas, or engaging with communities that share my love for innovation. My goal? To bridge the gap between technology and its users through clear, concise, and engaging communication - cheers.