Why Choose Redis? Understanding the Speed and Scalability of In-Memory Databases
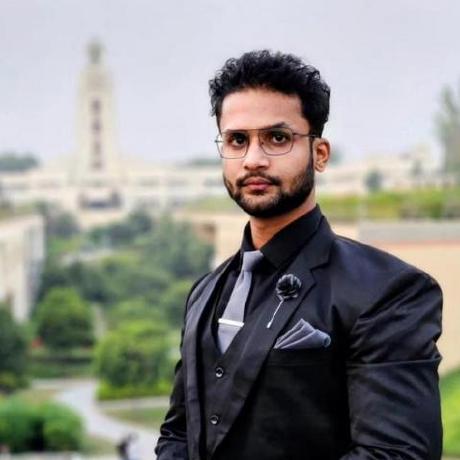
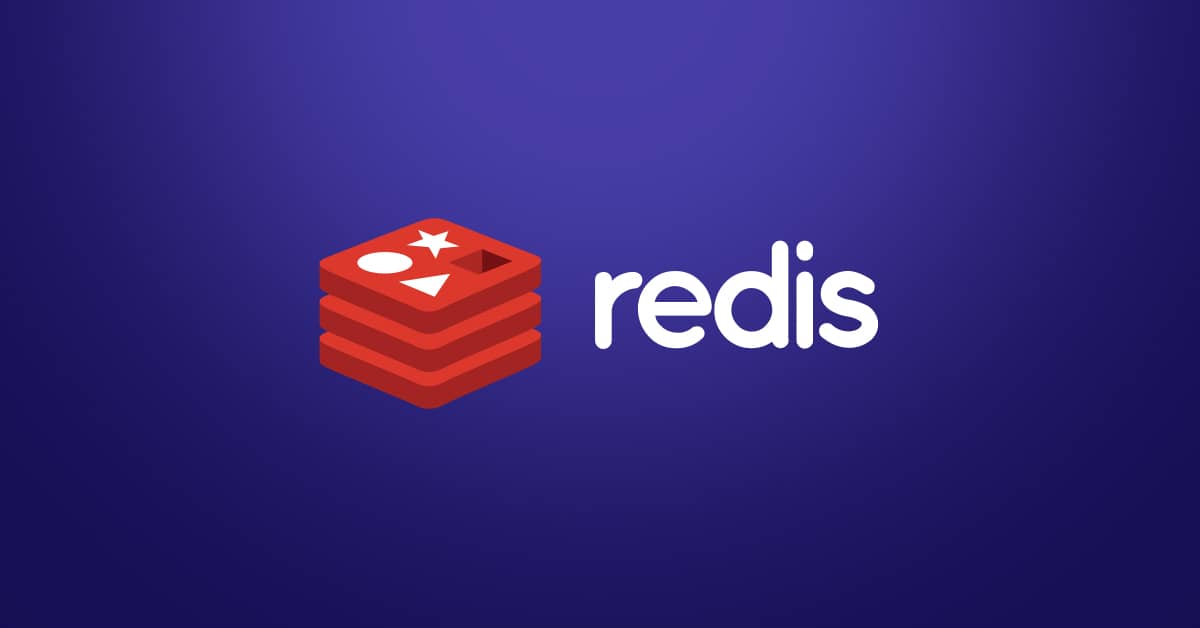
Introduction
In the fast-paced world of software development, performance and scalability are critical. Redis, an open-source, in-memory data structure store, is one tool that consistently meets these demands. Known for its speed and versatility, Redis serves as a database, cache, and message broker, making it a popular choice among developers and companies. This post explores Redis, its uses in the industry, best practices for implementation, and provides some practical code examples to get you started.
What is Redis?
Redis stands for REmote DIctionary Server. It's a powerful, open-source in-memory key-value store that supports various data structures such as strings, hashes, lists, sets, and sorted sets. One of Redis's standout features is its ability to keep all data in memory, enabling lightning-fast read and write operations. Additionally, Redis supports persistence, ensuring that your data isn't lost even after a restart. There are two main persistence mechanisms:
RDB (Redis Database File): Takes point-in-time snapshots of your dataset at specified intervals. This method is efficient for backups but may lose some data if a failure occurs between snapshots.
AOF (Append Only File): Logs every write operation, allowing complete dataset reconstruction. This method is more durable but may be slower than RDB.
Setting Up Redis Locally
Getting started with Redis is straightforward. You can quickly spin up a Redis instance using Docker:
docker run --name my-redis -d -p 6379:6379 redis
docker exec -it container_id /bin/bash
redis-cli
These commands launch a Redis container, allowing you to interact with Redis via the command-line interface (redis-cli
).
Redis in Industry: How It's Used
Redis is highly valued in the industry for specific use cases where speed and scalability are paramount:
Caching: Redis is commonly used as a cache to store frequently accessed data, reducing the load on primary databases and speeding up application responses. By caching data in memory, Redis allows applications to retrieve data quickly without hitting the slower disk-based storage.
Session Management: Redis is often employed to manage user sessions in web applications. It efficiently stores session data, such as user information and preferences, which can be quickly retrieved when the user revisits the site.
Real-Time Analytics: Redis's ability to handle a high volume of reads and writes makes it ideal for real-time analytics. Companies use Redis to store metrics, logs, and event data that need to be processed in real-time.
Message Queuing: Redis supports messaging with its Pub/Sub capabilities and list structures. It can be used to implement message queues for asynchronous processing, allowing applications to handle tasks in the background.
Best Practices for Using Redis
While Redis is powerful, it should be used appropriately to maximize its benefits:
Not a Primary Database: While Redis can be used as a primary database, it's best suited for use cases requiring high-speed access and not for long-term storage. Combine Redis with a persistent database for more extensive, non-volatile data storage.
Data Eviction Policies: Configure appropriate data eviction policies based on your use case to handle memory limits effectively. Redis supports various policies like
LRU
(Least Recently Used),LFU
(Least Frequently Used), andTTL
(Time to Live).Persistence Configuration: Choose the right persistence mode (RDB, AOF, or a combination) based on your application's durability and performance requirements.
Monitoring and Scaling: Use monitoring tools to track Redis performance and scale your infrastructure to handle increased loads as your application grows.
Example: Using Redis for Caching and Queues
Let’s dive into some practical code examples using Redis. These examples illustrate how Redis can be used as a cache and a queue system.
Basic Redis Commands
Redis operates as a key-value store. Here are some essential commands:
SET mykey "Hello"
GET mykey
DEL mykey
Using Redis for Caching User Data
HSET user:100 name "John Doe" email "user@example.com" age "30"
HGET user:100 name
HGET user:100 email
Implementing Queues with Redis
Queues are fundamental for background processing tasks. Redis provides simple commands to manage queues:
Pushing to a Queue:
LPUSH problems 1 LPUSH problems 2
Popping from a Queue:
RPOP problems
Blocked Pop (with timeout): This command waits for an item to be added to the list if it's empty, making it useful for worker processes that need to wait for tasks.
BRPOP problems 0
Building a Simple Queue System with Node.js
Let's create a simple system using Node.js and Redis to handle coding problem submissions, similar to a LeetCode platform.
Setting Up the Express Server:
Create a Node.js project and install necessary packages:
mkdir express-server cd express-server npm init -y npx tsc --init npm install express @types/express redis
Add the following code to
index.ts
:import express from "express"; import { createClient } from "redis"; const app = express(); app.use(express.json()); const client = createClient(); client.on('error', (err) => console.log('Redis Client Error', err)); app.post("/submit", async (req, res) => { const { problemId, code, language } = req.body; try { await client.lPush("problems", JSON.stringify({ code, language, problemId })); res.status(200).send("Submission received and stored."); } catch (error) { console.error("Redis error:", error); res.status(500).send("Failed to store submission."); } }); async function startServer() { try { await client.connect(); console.log("Connected to Redis"); app.listen(3000, () => { console.log("Server is running on port 3000"); }); } catch (error) { console.error("Failed to connect to Redis", error); } } startServer();
Creating a Worker Service:
In a separate
worker
folder, set up a Node.js project to process submissions:mkdir worker cd worker npm init -y npx tsc --init npm install redis
Add the following code to
index.ts
:import { createClient } from "redis"; const client = createClient(); async function processSubmission(submission: string) { const { problemId, code, language } = JSON.parse(submission); console.log(`Processing submission for problemId ${problemId}...`); console.log(`Code: ${code}`); console.log(`Language: ${language}`); // Simulate processing delay await new Promise(resolve => setTimeout(resolve, 2000)); console.log(`Finished processing submission for problemId ${problemId}.`); } async function startWorker() { try { await client.connect(); console.log("Worker connected to Redis."); while (true) { try { const submission = await client.brPop("problems", 0); // @ts-ignore await processSubmission(submission[1]); } catch (error) { console.error("Error processing submission:", error); } } } catch (error) { console.error("Failed to connect to Redis", error); } } startWorker();
Conclusion
Redis is a versatile tool that excels in scenarios where speed, scalability, and simplicity are required. Whether used for caching, session management, or as a message broker, Redis enables developers to build high-performance, reliable applications. By understanding its core concepts and best practices, you can effectively integrate Redis into your projects to handle demanding workloads.
Redis is a powerful ally in building scalable, high-performance applications. From caching to message queuing, Redis provides developers with the tools needed to optimize data access and processing. By following best practices and leveraging Redis's capabilities, you can enhance your applications' efficiency and responsiveness.
Happy coding! 🚀
Subscribe to my newsletter
Read articles from Swami Buddha Chaitanya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
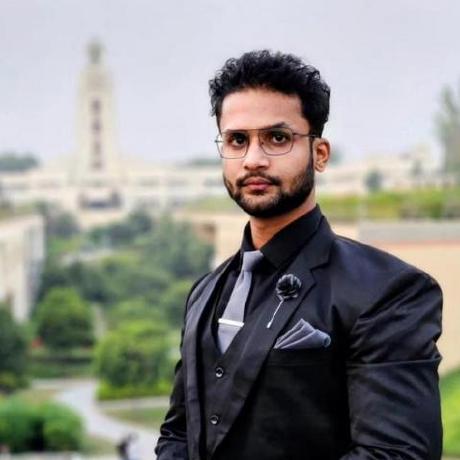