React: Zero to Hero 4
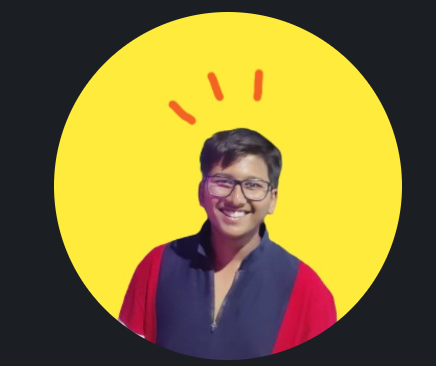
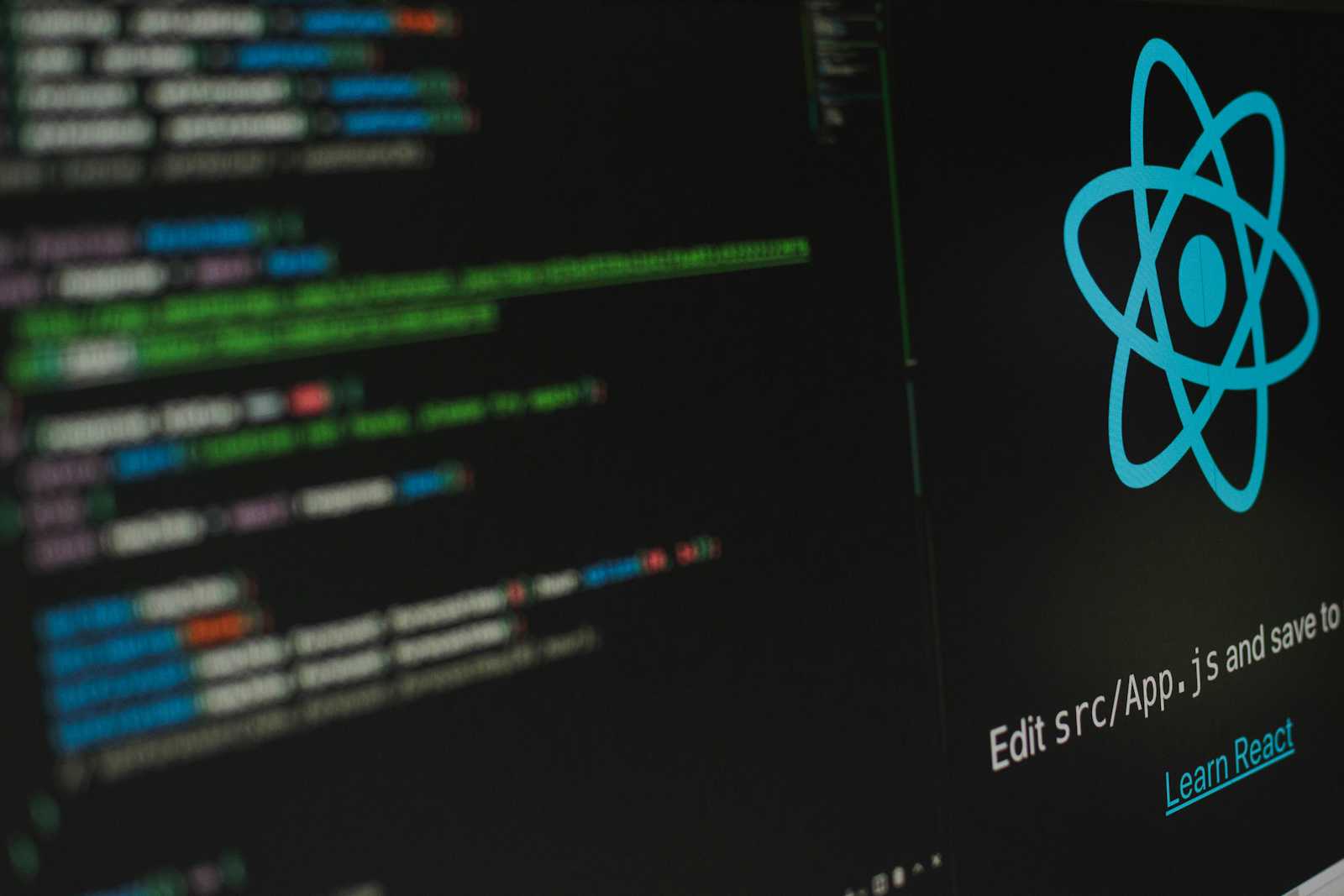
Mastering Routing with React Router
Routing is a fundamental aspect of modern web applications. It enables navigation between different views or pages within an application without requiring a full page reload. For React applications, React Router is the go-to library for managing routing. This post will explore React Router in detail, covering its setup, core concepts, and advanced features to help you master routing in your React apps.
What is React Router?
React Router is a standard library for routing in React. It enables you to define different routes in your application and handle navigation between them. With React Router, you can build single-page applications (SPAs) with dynamic route handling and rendering based on the URL.
Setting Up React Router
To get started with React Router, you need to install it via npm or yarn. Ensure you have a React application set up before proceeding.
Installation
Run the following command to install React Router:
bashCopy codenpm install react-router-dom
or if you are using yarn:
bashCopy codeyarn add react-router-dom
Core Concepts of React Router
React Router provides several key components and concepts that are crucial for routing in React applications. Let’s dive into each of these components.
1. BrowserRouter
The BrowserRouter
component is the primary router component that uses HTML5 history API to keep the UI in sync with the URL. It should be used at the top level of your application.
jsxCopy codeimport { BrowserRouter as Router } from 'react-router-dom';
function App() {
return (
<Router>
{/* Your routes go here */}
</Router>
);
}
export default App;
2. Route
The Route
component is used to define a path and the component that should be rendered when the path matches the URL. Each Route
should be placed inside a Router
.
jsxCopy codeimport { Route, Switch } from 'react-router-dom';
import Home from './Home';
import About from './About';
function App() {
return (
<Router>
<Switch>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
</Switch>
</Router>
);
}
export default App;
3. Switch
The Switch
component is used to render only the first Route
that matches the current URL. This ensures that only one route is rendered at a time.
jsxCopy code<Switch>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
</Switch>
4. Link
The Link
component is used to navigate between different routes. It renders an anchor (<a>
) tag, but instead of a full page reload, it uses React Router's internal mechanisms to change the URL and update the view.
jsxCopy codeimport { Link } from 'react-router-dom';
function Navigation() {
return (
<nav>
<Link to="/">Home</Link>
<Link to="/about">About</Link>
</nav>
);
}
export default Navigation;
5. NavLink
The NavLink
component is similar to Link
but with additional features. It can apply styles based on whether the link is active, making it useful for navigation menus.
jsxCopy codeimport { NavLink } from 'react-router-dom';
function Navigation() {
return (
<nav>
<NavLink exact to="/" activeClassName="active">Home</NavLink>
<NavLink to="/about" activeClassName="active">About</NavLink>
</nav>
);
}
export default Navigation;
6. useParams
The useParams
hook is used to access URL parameters within a component. This is useful for dynamic routing where parts of the URL are variable.
jsxCopy codeimport { useParams } from 'react-router-dom';
function UserProfile() {
const { userId } = useParams();
return <div>User Profile for User ID: {userId}</div>;
}
// Route definition
<Route path="/user/:userId" component={UserProfile} />
7. useHistory
The useHistory
hook allows you to programmatically navigate to different routes or manage history within your components.
jsxCopy codeimport { useHistory } from 'react-router-dom';
function Login() {
const history = useHistory();
const handleLogin = () => {
// Perform login logic
history.push('/dashboard');
};
return <button onClick={handleLogin}>Login</button>;
}
export default Login;
8. useLocation
The useLocation
hook provides access to the current location object, which contains information about the current URL.
jsxCopy codeimport { useLocation } from 'react-router-dom';
function CurrentLocation() {
const location = useLocation();
return <div>Current URL: {location.pathname}</div>;
}
export default CurrentLocation;
Advanced Routing Patterns
Nested Routes
React Router supports nested routes, which means you can have routes within routes. This is useful for applications with complex layouts.
jsxCopy codeimport { Route, Switch } from 'react-router-dom';
import Dashboard from './Dashboard';
import UserProfile from './UserProfile';
function App() {
return (
<Router>
<Switch>
<Route path="/dashboard" component={Dashboard} />
<Route path="/user/:userId" component={UserProfile} />
</Switch>
</Router>
);
}
// Inside Dashboard component
function Dashboard() {
return (
<div>
<h2>Dashboard</h2>
<Route path="/dashboard/profile" component={UserProfile} />
</div>
);
}
Redirects
You can redirect users to a different route programmatically or conditionally using the Redirect
component or useNavigate
hook.
jsxCopy codeimport { Redirect } from 'react-router-dom';
// Redirect component usage
function LoginRedirect() {
return <Redirect to="/login" />;
}
// useNavigate hook usage
import { useNavigate } from 'react-router-dom';
function SomeComponent() {
const navigate = useNavigate();
navigate('/some-path');
}
Route Guards
Route guards (or private routes) are used to restrict access to certain routes based on conditions, such as user authentication.
jsxCopy codeimport { Route, Redirect } from 'react-router-dom';
function PrivateRoute({ component: Component, ...rest }) {
const isAuthenticated = /* logic to check authentication */;
return (
<Route
{...rest}
render={props =>
isAuthenticated ? (
<Component {...props} />
) : (
<Redirect to="/login" />
)
}
/>
);
}
Dynamic Routing
Dynamic routing involves creating routes based on data or user input. This is useful for applications that need to handle routes generated at runtime.
jsxCopy codeimport { Route, useParams } from 'react-router-dom';
function Product({ products }) {
const { productId } = useParams();
const product = products.find(p => p.id === parseInt(productId, 10));
if (!product) return <div>Product not found</div>;
return <div>{product.name}</div>;
}
// Route definition
<Route path="/product/:productId" render={(props) => <Product {...props} products={productList} />} />
Conclusion
React Router is a powerful and flexible library for handling routing in React applications. By understanding and utilizing its core concepts, such as BrowserRouter
, Route
, Link
, and hooks like useParams
and useHistory
, you can build dynamic, navigable SPAs. Additionally, mastering advanced features like nested routes, redirects, and route guards will help you create robust and user-friendly applications.
Thank you for reading till here. If you want learn more then ping me personally and make sure you are following me everywhere for the latest updates.
Yours Sincerely,
Sai Aneesh
Subscribe to my newsletter
Read articles from Sai Aneesh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
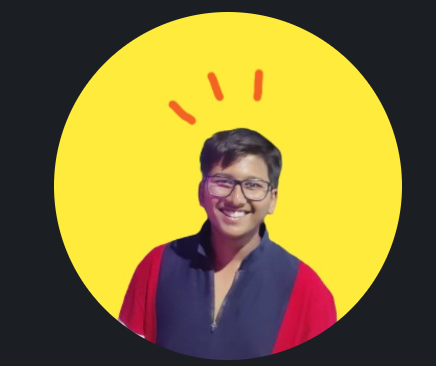