Mastering Python’s zip() Function: A Powerful Tool for Working with Multiple Lists

Table of contents
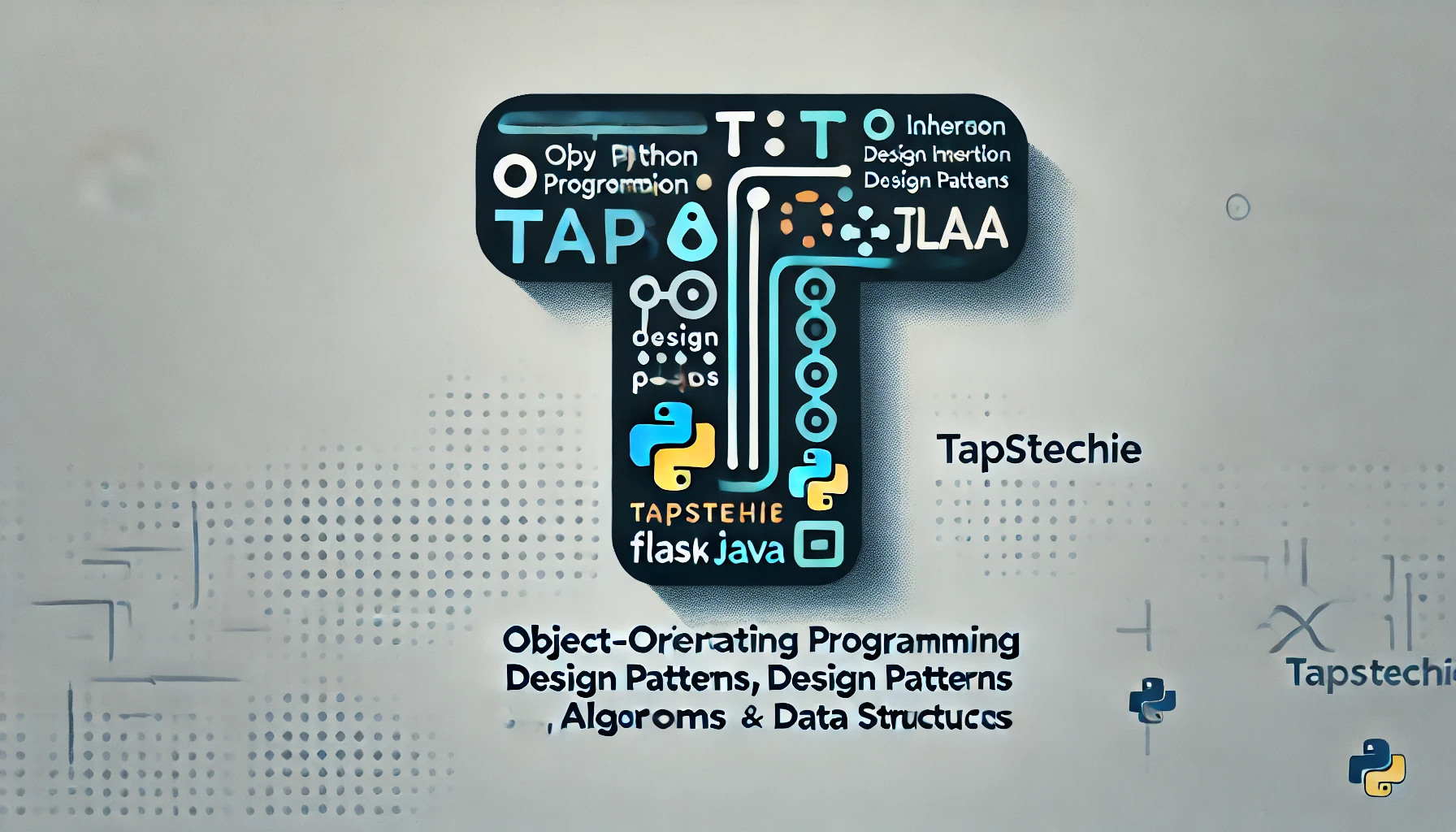
Python's zip()
function is one of those little gems that can make your code cleaner, more efficient, and more readable. Whether you're working with multiple lists, tuples, or any other iterables, zip()
can help you iterate over them in parallel, combine them, or even transform them in creative ways.
In this blog, we’ll explore what zip()
does, how to use it, and some practical examples to showcase its power.
What is the zip()
Function?
The zip()
function takes two or more iterables and aggregates them into tuples, creating a zip object. Each tuple contains one element from each of the iterables. This is incredibly useful when you want to iterate over multiple sequences simultaneously or combine them into a single structure.
Basic Syntax
zip(iterable1, iterable2, ...)
iterable1, iterable2, ...
: These are the iterables (like lists or tuples) that you want to combine.
Example 1: Iterating Over Two Lists Simultaneously
Let’s say you have two lists: one containing names and another containing ages. You want to pair each name with its corresponding age and print them.
Traditional Approach with Indexing:
names = ['Alice', 'Bob', 'Charlie']
ages = [25, 30, 35]
for i in range(len(names)):
print(f"{names[i]} is {ages[i]} years old")
The zip()
Approach:
With zip()
, you can do this more elegantly:
names = ['Alice', 'Bob', 'Charlie']
ages = [25, 30, 35]
for name, age in zip(names, ages):
print(f"{name} is {age} years old")
This approach not only reduces the risk of index errors but also makes the code more readable and Pythonic.
Example 2: Creating a Dictionary from Two Lists
Suppose you have two lists: one with keys and another with values, and you want to create a dictionary from them.
Lists:
keys = ['name', 'age', 'city']
values = ['Alice', 25, 'New York']
Creating a Dictionary Using zip()
:
person = dict(zip(keys, values))
Result:
person = {'name': 'Alice', 'age': 25, 'city': 'New York'}
Here, zip()
pairs each key with its corresponding value, making it easy to create a dictionary in a single line of code.
Example 3: Unzipping a List of Tuples
What if you have a list of tuples and want to separate them into individual lists? zip()
can do that too!
List of Tuples:
pairs = [('Alice', 25), ('Bob', 30), ('Charlie', 35)]
Unzipping with zip()
:
names, ages = zip(*pairs)
Result:
names = ('Alice', 'Bob', 'Charlie')
ages = (25, 30, 35)
The *
operator unpacks the list of tuples so that zip()
can separate the elements into two distinct tuples.
Example 4: Combining Multiple Lists
You can use zip()
to combine more than two lists. Let’s say you have three lists, and you want to iterate over them simultaneously.
Lists:
names = ['Alice', 'Bob', 'Charlie']
ages = [25, 30, 35]
cities = ['New York', 'Los Angeles', 'Chicago']
Iterating Over Three Lists:
for name, age, city in zip(names, ages, cities):
print(f"{name}, {age} years old, lives in {city}")
Result:
Alice, 25 years old, lives in New York
Bob, 30 years old, lives in Los Angeles
Charlie, 35 years old, lives in Chicago
This allows you to easily manage multiple data streams in a single loop.
Example 5: Handling Lists of Different Lengths
When the iterables passed to zip()
are of different lengths, zip()
will stop combining when the shortest iterable is exhausted. This behavior can be useful, but if you need to handle lists of different lengths, you should be aware of this limitation.
Uneven Lists:
names = ['Alice', 'Bob']
ages = [25, 30, 35]
Result:
for name, age in zip(names, ages):
print(f"{name} is {age} years old")
Output:
Alice is 25 years old
Bob is 30 years old
Here, the extra element in the ages
list is ignored because the names
list only has two elements. If you need to handle all elements, consider using itertools.zip
_longest()
from the itertools
module, which will fill in the missing values with None
or a specified fill value.
Example Using zip_longest
:
from itertools import zip_longest
for name, age in zip_longest(names, ages, fillvalue='Unknown'):
print(f"{name} is {age} years old")
Output:
Alice is 25 years old
Bob is 30 years old
None is 35 years old
This way, no data is left out, and you can handle uneven lists gracefully.
Conclusion
The zip()
function is a versatile tool that can simplify your code and make it more readable. Whether you're combining lists, creating dictionaries, or handling complex data structures, zip()
allows you to manage multiple sequences with ease. It’s one of those Python features that, once mastered, will significantly streamline your coding process.
Next time you find yourself iterating over multiple lists or needing to pair elements from different sequences, remember the power of zip()
. It’s a small function with big potential!
Keep experimenting with Python and happy coding! 🔧🐍
Subscribe to my newsletter
Read articles from Tarun Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Tarun Sharma
Tarun Sharma
Hi there! I’m Tarun, a Senior Software Engineer with a passion for technology and coding. With experience in Python, Java, and various backend development practices, I’ve spent years honing my skills and working on exciting projects. On this blog, you’ll find insights, tips, and tutorials on topics ranging from object-oriented programming to tech trends and interview prep. My goal is to share valuable knowledge and practical advice to help fellow developers grow and succeed. When I’m not coding, you can find me exploring new tech trends, working on personal projects, or enjoying a good cup of coffee. Thanks for stopping by, and I hope you find my content helpful!