BigInt: Big brother of Number we didn't knew about in Javascript!!

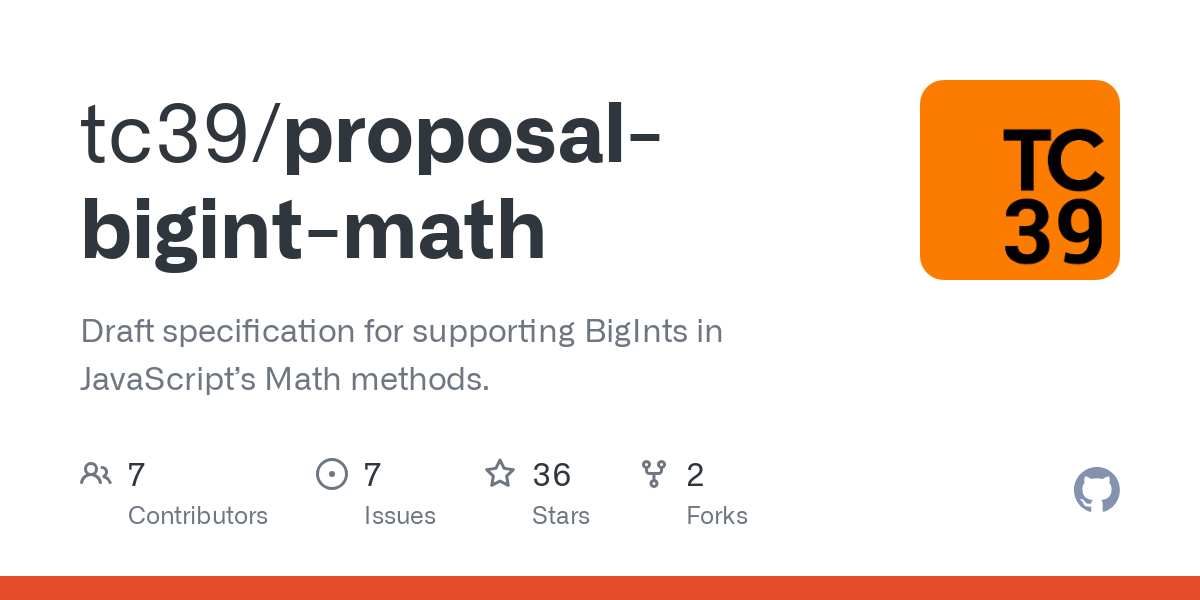
I’ve been using JavaScript for over seven years, and I thought I knew the language well. But recently, I discovered there’s still so much to learn—especially when it comes to the Primitive Types in JavaScript that I wasn’t even aware of!
For example, why was there a need for BigInt? Wasn’t Number
enough? Personally, I never felt we were lacking anything with Number
. But I was wrong because I had never worked with really large numbers. When I say “huge numbers,” I’m talking about something like 9007199254740991
.
Before you ask why this random number is here, let me tell you—it’s the largest number that the Number
type can safely handle. Don’t believe me? Check this out:
const value = Number.MAX_SAFE_INTEGER //9007199254740991;
console.log(value + 2)
//in console: 9007199254740992
As you can see, Number
starts to lose precision with values beyond this limit. That’s where BigInt comes in.
BigInt is like the big gun that handles what Number
cannot. It’s the Hulk with the Infinity Gauntlet of data types! Don’t believe me? Let me show you. Have you ever had to convert values between different number systems like Octal to Decimal in JavaScript? It can get a bit tricky, but BigInt simplifies this to a single line of code!
But before we dive into that, let’s understand more about BigInt.
A BigInt value is a primitive type created by appending
n
to the end of an integer literal or by calling theBigInt()
function (without thenew
operator) and passing it an integer or string value.
That’s the formal definition from the MDN docs—did you get it?
If not, let me clarify: In the image below, notice how the number 12222222
is appended with the character n
. This makes it a BigInt. It’s no longer a regular Number
but a BigInt
. You can achieve the same result with strings using the BigInt()
function like this: BigInt("122222222")
.
typeof 12222222n
//bigint
typeof BigInt("12222222")
//bigint
You can also convert values from different number systems using the same BigInt()
function. If a number is prepended with 0x...
(zero-x), it denotes that the number is in Hexadecimal format.
Here’s a quick guide to different number systems and how they’re represented in JavaScript:
Number System | Represented by | example |
Binary | 0b | 0b100110101, 0b111011 |
Octal | 0o | 0o432144, 0o455244 |
Decimal | none | 2123123, 8765432 |
Hexadecimal | 0x | 0xfb213a, 0xfffff |
const alsoHuge = BigInt(9007199254740991);
// 9007199254740991n
const hugeString = BigInt("9007199254740991");
// 9007199254740991n
const hugeHex = BigInt(0x1fffffffffffff);
// 9007199254740991n
const hugeOctal = BigInt("0o377777777777777777");
// 9007199254740991n
const hugeBin = BigInt(
"0b11111111111111111111111111111111111111111111111111111",
);
// 9007199254740991n
BigInts can be used with all the arithmetic operators that work with normal numbers,
Operator group | Mixed Number with Bigint allowed | example |
Arithmetic (+ , - , * , / , % , ** ) | No | bigint + bigint, bigint * bigint |
Bitwise (>> , << , & , ` | , ^, ~`) | No |
Unary (- ) | No | -bigint (-123123n) |
Increment / Decrement(++, --) | No | ++bigint |
Relational/ Equality (> , < , >= , <= , == , != , === , !== ) | Yes | number > bigint |
Check this out in more detail here MDN docs for BigInt.
Thanks for reading!!!
Subscribe to my newsletter
Read articles from Abhijitsingh Sisodiya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
