Counter - LeetCode: 30 Days of JavaScript
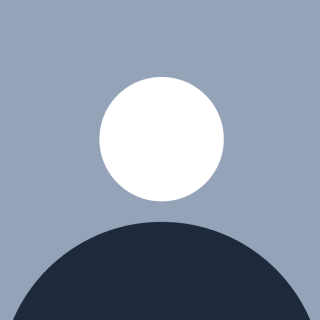
Table of contents
Welcome to day two LeetCode challenge in the "30 Days of JavaScript" series. We will be solving this problem:
Given an integer n
, return a counter
function. This counter
function initially returns n
and then returns 1 more than the previous value every subsequent time it is called (n
, n + 1
, n + 2
, etc).
Problem breakdown
We are expected to:
Write a function that takes an integer
n
.The function should return a
counter
function.The counter function will initially return
n
, increase by 1 each time the function is called, and then, return the incremented value on the next call. For example, if 2 is the initial value, each time you call thecounter
function, it will return 3, 4, 5, 6, and so on.
Solution
// Given an integer n, return a counter function. This counter function initially returns n and then returns 1 more than the previous value every subsequent time it is called (n, n + 1, n + 2, etc).
var createCounter = function (n) {
return function () {
const sum = n++;
return sum;
};
};
const aggregate = createCounter(5);
//console.log(aggregate()); //6
//console.log(aggregate()); // 7
//console.log(aggregate()); // 8
From the code above, we created a function called createCounter
that takes an integer n
. createCounter
returns an anonymous function that does not take any parameter. The returned function will increment the value of n
using the post-increment operator('n++
') and then, return the value of n
.
Furthermore, we assigned createCounter
to a variable called aggregate
, and n
is given the value of 5. So each time aggregate
is called, n
increases by 1.
Conclusion
This article solved the second LeetCode challenge in the "30 Days of JavaScript" series. I look forward to solving the next challenge.
Subscribe to my newsletter
Read articles from Susan Odii directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Susan Odii
Susan Odii
Susan is a Frontend Web Developer. She is passionate about creating beautiful and engaging UI with good user experience. Susan is also a technical writer who writes on Frontend technologies to help other developers get a better understanding of a concept.