How to Create a Custom Form Validation Hook in React: A Step-by-Step Guide
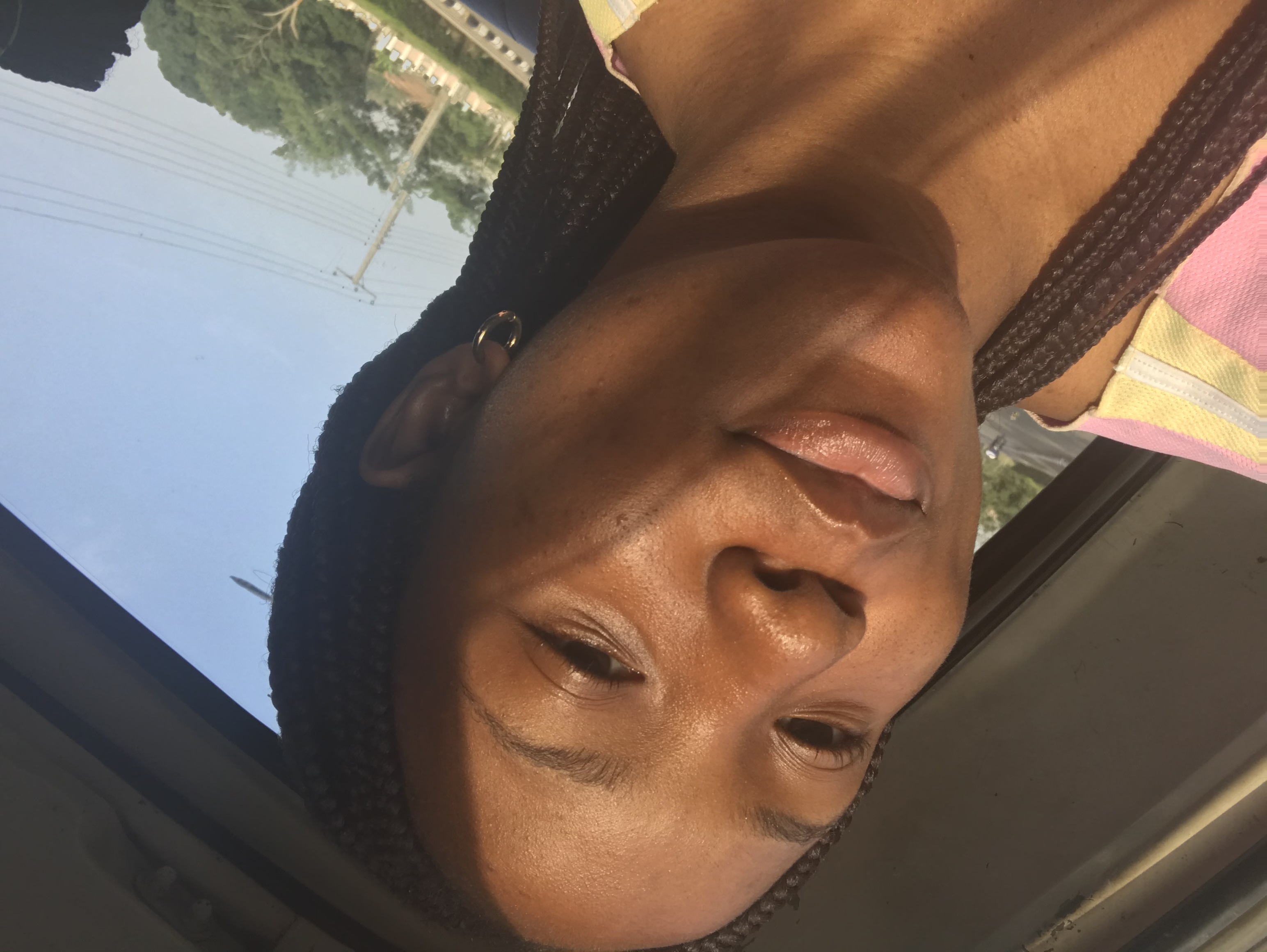
Introduction
Form validation is a common requirement in web applications, and while there are many libraries available to handle it, creating your own custom solution in React gives you full control and a deeper understanding of how it works. In this tutorial, we’ll build a reusable custom form validation hook from scratch. Whether you're a beginner or an experienced developer, this guide will help you create a robust and flexible validation system for your forms.
Why Create a Custom Hook?
Using a custom hook for form validation in React has several advantages:
Reusability: You can apply the same validation logic across multiple forms in your application.
Customization: You can tailor the validation rules to fit the specific needs of your project.
Simplicity: Custom hooks encapsulate logic in a way that keeps your components clean and focused.
Step 1: Setting Up Your React Application
First, ensure that you have a React project set up. If you don't, you can quickly create one using Create React App
npx create-react-app form-validation-hook cd form-validation-hook npm start
Step 2: Creating the Custom Hook
Next, we’ll create a new file for our custom hook,
useFormValidation.js
, in thesrc
directory.```javascript import { useState } from 'react';
const useFormValidation = (initialState, validate) => { const [values, setValues] = useState(initialState); const [errors, setErrors] = useState({});
const handleChange = (event) => { const { name, value } = event.target; setValues({ ...values,
}); };
const handleSubmit = (event) => { event.preventDefault(); const validationErrors = validate(values); setErrors(validationErrors); };
return { values, errors, handleChange, handleSubmit, }; };
export default useFormValidation;
### **Step 3: Implementing the Validation Logic**
The `validate` function will check the input values against your validation rules. This function can be passed into the hook and customized for different forms. Let's create a simple validation function.
* ```javascript
const validate = (values) => {
let errors = {};
if (!values.email) {
errors.email = 'Email address is required';
} else if (!/\S+@\S+\.\S+/.test(values.email)) {
errors.email = 'Email address is invalid';
}
if (!values.password) {
errors.password = 'Password is required';
} else if (values.password.length < 6) {
errors.password = 'Password must be at least 6 characters';
}
return errors;
};
export default validate;
Step 4: Integrating the Hook into a Form
Now, let's see how to use our custom
useFormValidation
hook in a form component.import React from 'react'; import useFormValidation from './useFormValidation'; import validate from './validate'; const initialState = { email: '', password: '', }; const LoginForm = () => { const { values, errors, handleChange, handleSubmit } = useFormValidation(initialState, validate); return ( <form onSubmit={handleSubmit}> <div> <label>Email</label> <input type="email" name="email" value={values.email} onChange={handleChange} /> {errors.email && <p>{errors.email}</p>} </div> <div> <label>Password</label> <input type="password" name="password" value={values.password} onChange={handleChange} /> {errors.password && <p>{errors.password}</p>} </div> <button type="submit">Login</button> </form> ); }; export default LoginForm;
Step 5: Testing the Form
Once you've integrated the hook into your form, try entering invalid data to see how the validation works. The hook should update the form with appropriate error messages.
Conclusion
With this custom hook, you've created a reusable, flexible, and easy-to-manage form validation system for your React applications. You can expand this by adding more complex validation rules, handling additional input types, or even integrating it with other form libraries. This approach keeps your components clean and allows you to manage validation logic separately, making your code more maintainable.
Subscribe to my newsletter
Read articles from Kpeale Legbara directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
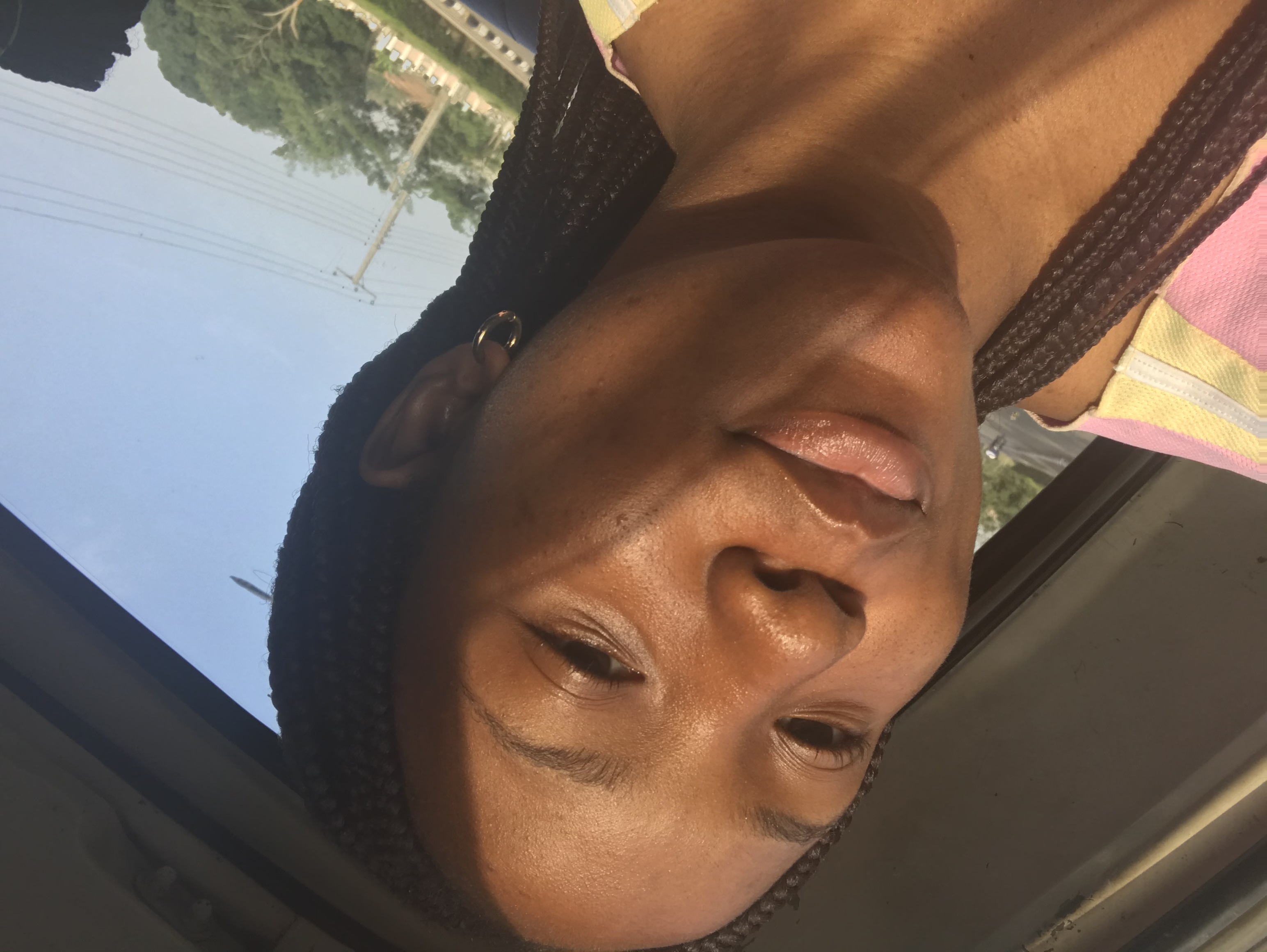