Why Use WebSockets? Understanding Their Benefits and How to Implement Them
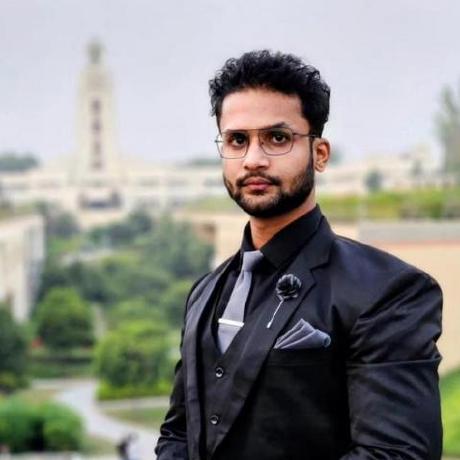
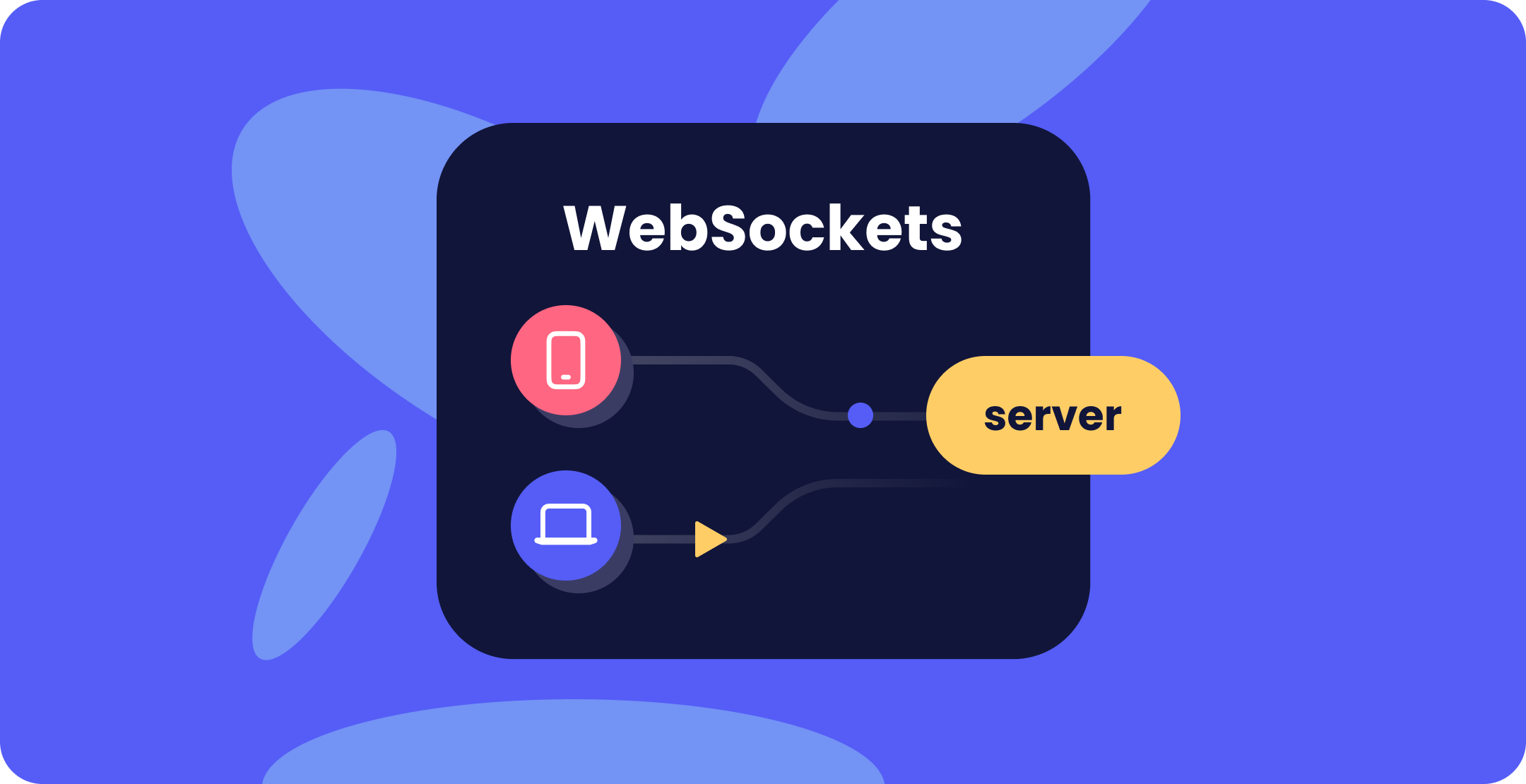
Introduction
In an era where instant communication and real-time updates are the norm, WebSockets have emerged as a powerful tool for enabling seamless, bidirectional communication between clients and servers. Unlike traditional HTTP, which operates on a request-response model, WebSockets maintain an open connection, allowing for continuous data exchange. This article dives into what WebSockets are, why they're used in advanced backend communication, how they differ from HTTP, and provides practical examples of implementing WebSockets in a React and Node.js application.
What are WebSockets?
WebSockets are a protocol that facilitates two-way communication channels over a single, long-lived TCP connection. They allow for real-time data transfer between a client and a server, eliminating the need for constant polling or multiple HTTP requests. Once a WebSocket connection is established, data can be sent back and forth freely and efficiently.
Why Use WebSockets?
WebSockets are particularly valuable in scenarios where low latency and real-time communication are essential. Here are some common use cases:
Real-Time Messaging Applications: Platforms like WhatsApp, Slack, and Facebook Messenger use WebSockets to provide instant messaging capabilities.
Live Notifications: Social media platforms such as Instagram and Twitter use WebSockets to push live notifications and updates to users.
Collaborative Applications: Tools like Google Docs use WebSockets to allow multiple users to edit a document simultaneously, with real-time updates.
Online Gaming: Multiplayer games use WebSockets to keep all players' actions synchronized in real-time.
Stock Trading Platforms: Financial services utilize WebSockets to provide real-time updates on stock prices and market changes.
Coding Platforms: LeetCode, for example, uses WebSockets to provide real-time feedback on code submissions and problem-solving.
WebSockets vs. HTTP: What’s the Difference?
Understanding the key differences between WebSockets and HTTP is crucial to appreciate why WebSockets are essential for specific applications:
Communication Model:
HTTP: Works on a request-response model. The client sends a request, and the server responds. Once the response is delivered, the connection is closed.
WebSockets: Maintain an open, continuous connection. This allows both client and server to send messages to each other at any time without having to establish a new connection.
Efficiency:
HTTP: Requires multiple connections for continuous communication, leading to higher latency and overhead.
WebSockets: Use a single connection for ongoing communication, reducing latency and bandwidth usage.
Data Transfer:
HTTP: Limited to text-based data transfer.
WebSockets: Support both text and binary data, providing more flexibility.
Implementing a Simple WebSocket Example
To demonstrate how WebSockets work, let's create a simple WebSocket-based chat application using React (TypeScript) for the frontend and Node.js (TypeScript) for the backend.
Setting Up the Backend with Node.js
First, we’ll set up a basic WebSocket server using Node.js and the ws
library.
Initialize a Node.js Project:
mkdir websocket-server cd websocket-server npm init -y npm install ws typescript @types/ws npx tsc --init
Create the WebSocket Server:
Create a file named
server.ts
and add the following code:import { WebSocketServer } from 'ws'; const wss = new WebSocketServer({ port: 8080 }); wss.on('connection', (ws) => { console.log('New client connected'); ws.on('message', (message) => { console.log(`Received message: ${message}`); // Echo back the message to the client ws.send(`Server: ${message}`); }); ws.on('close', () => { console.log('Client disconnected'); }); }); console.log('WebSocket server is running on ws://localhost:8080');
This code sets up a WebSocket server on port 8080. It listens for new client connections, receives messages from clients, and echoes those messages back to the sender.
Setting Up the Frontend with React (TypeScript)
Next, let's create a simple React application that connects to our WebSocket server.
Initialize a React Project:
npx create-react-app websocket-client --template typescript cd websocket-client npm install
Create a WebSocket Component:
Add a new component
WebSocketChat.tsx
in thesrc
folder with the following code:import React, { useState, useEffect } from 'react'; const WebSocketChat: React.FC = () => { const [ws, setWs] = useState<WebSocket | null>(null); const [messages, setMessages] = useState<string[]>([]); const [input, setInput] = useState<string>(''); useEffect(() => { const socket = new WebSocket('ws://localhost:8080'); socket.onopen = () => { console.log('Connected to WebSocket server'); }; socket.onmessage = (event) => { setMessages(prevMessages => [...prevMessages, event.data]); }; socket.onclose = () => { console.log('Disconnected from WebSocket server'); }; setWs(socket); return () => { socket.close(); }; }, []); const sendMessage = () => { if (ws && input) { ws.send(input); setInput(''); } }; return ( <div> <h2>WebSocket Chat</h2> <div> {messages.map((msg, index) => ( <p key={index}>{msg}</p> ))} </div> <input type="text" value={input} onChange={(e) => setInput(e.target.value)} /> <button onClick={sendMessage}>Send</button> </div> ); }; export default WebSocketChat;
This component establishes a WebSocket connection to the server, listens for incoming messages, and allows users to send messages.
Integrate the Component into Your App:
Update the
App.tsx
file to include theWebSocketChat
component:import React from 'react'; import WebSocketChat from './WebSocketChat'; const App: React.FC = () => { return ( <div> <WebSocketChat /> </div> ); }; export default App;
WebSockets in Industry
WebSockets are widely used across various industries to enable real-time communication and enhance user experience. Here are some notable examples:
LeetCode and Coding Platforms: These platforms use WebSockets to provide real-time updates on code execution, test case results, and collaboration features.
Social Media Apps (e.g., Instagram, Twitter): WebSockets are used to push live notifications, update feeds in real-time, and handle instant messaging.
Stock Trading Platforms: Real-time stock price updates and order book changes are delivered using WebSockets.
Collaborative Tools (e.g., Google Docs, Trello): WebSockets enable multiple users to interact with shared documents and boards simultaneously, ensuring that everyone sees the latest changes instantly.
Online Gaming: Multiplayer games rely on WebSockets to synchronize player actions and game state in real-time.
Conclusion
WebSockets provide a robust and efficient solution for real-time, bidirectional communication, making them indispensable in today's interactive web applications. Whether you're building a chat app, pushing live updates, or enabling real-time collaboration, WebSockets offer a seamless way to enhance user experience. By understanding the power of WebSockets and implementing them effectively, you can build applications that are not only responsive but also engaging and interactive.
Happy coding! 🎉
Subscribe to my newsletter
Read articles from Swami Buddha Chaitanya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
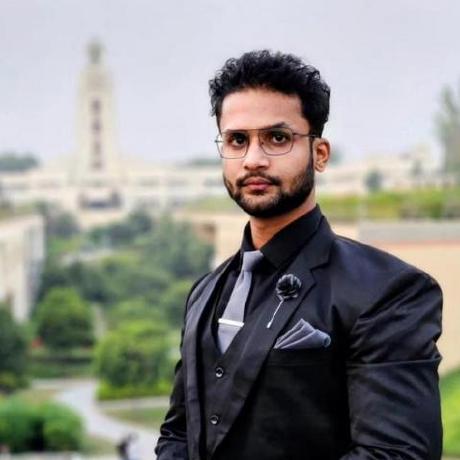