π Unlocking Hidden Power: Essential Yet Overlooked JavaScript Concepts Every Beginner Should Know π‘
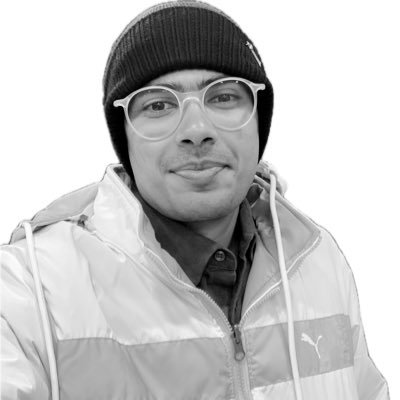
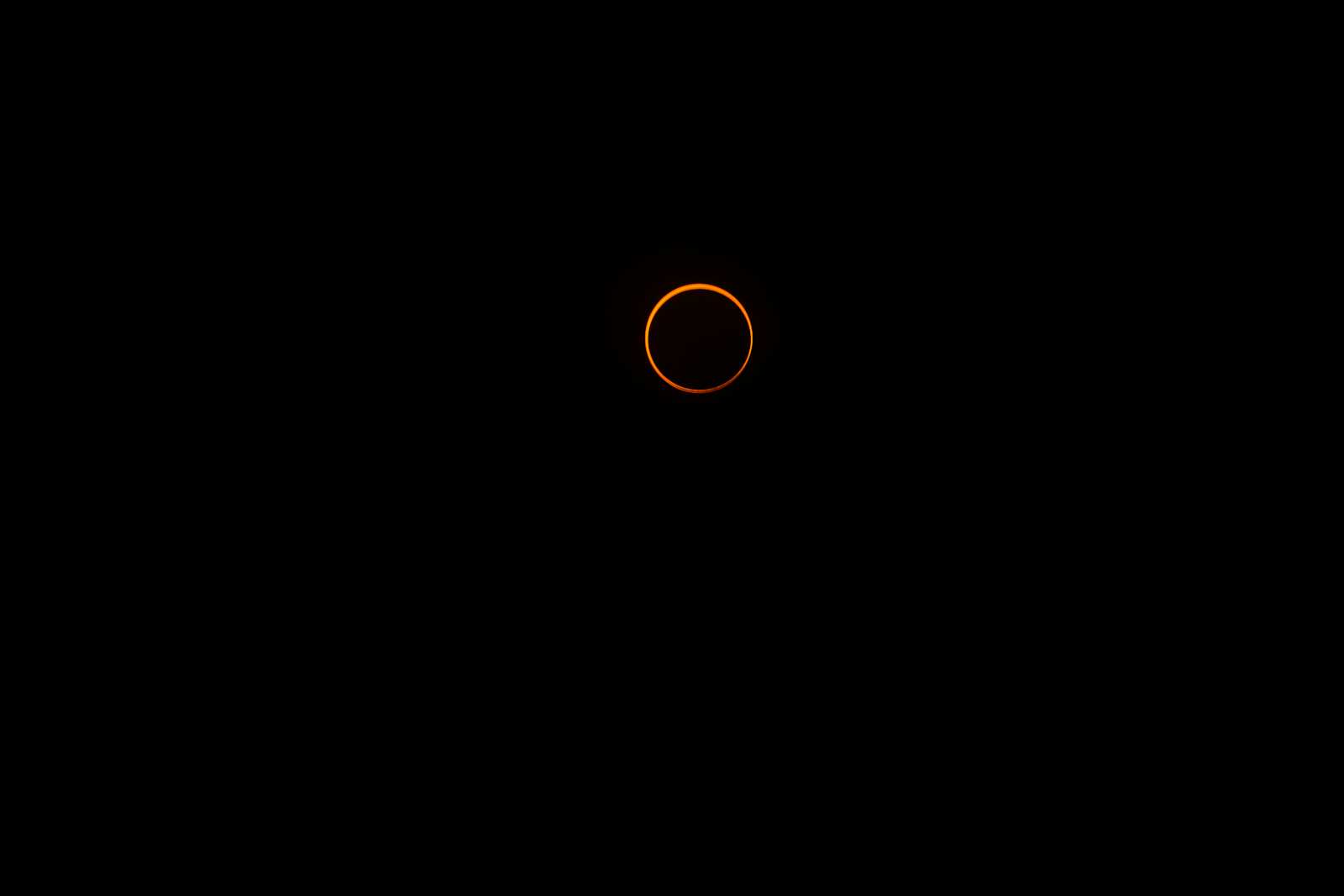
JavaScript is a versatile and powerful language that continues to evolve, with new features and concepts being introduced regularly. While many developers are familiar with the basics and popular features of JavaScript, there are several lesser-known concepts that can significantly enhance your coding skills and make your code more efficient and elegant. In this blog post, weβll explore some of these hidden gems that every JavaScript developer should know. π
1. π§© Closures: Mastering Function Scope
Closures are one of the most powerful yet often misunderstood features in JavaScript. A closure is created when a function is defined inside another function, allowing the inner function to access variables from the outer functionβs scope, even after the outer function has returned.
Example:
function outerFunction() {
let outerVariable = 'I am from the outer scope';
function innerFunction() {
console.log(outerVariable); // Can access the outerVariable
}
return innerFunction;
}
const myClosure = outerFunction();
myClosure(); // Output: I am from the outer scope
Why it's useful: Closures allow for data encapsulation and are frequently used in callbacks, event handlers, and functional programming.
2. π Memoization: Optimizing Function Performance
Memoization is an optimization technique that stores the results of expensive function calls and returns the cached result when the same inputs occur again. This can greatly improve the performance of functions with heavy computations or frequent calls.
Example:
function memoize(fn) {
const cache = {};
return function(...args) {
const key = JSON.stringify(args);
if (cache[key]) {
return cache[key];
} else {
const result = fn(...args);
cache[key] = result;
return result;
}
};
}
const factorial = memoize(function(n) {
if (n <= 1) return 1;
return n * factorial(n - 1);
});
console.log(factorial(5)); // Calculated
console.log(factorial(5)); // Cached
Why it's useful: Memoization can boost performance by avoiding redundant calculations, making it ideal for scenarios like dynamic programming or expensive computations.
3. π² Currying: Breaking Down Functions
Currying is a technique of transforming a function that takes multiple arguments into a sequence of functions that each take a single argument. This allows for creating more modular and reusable code.
Example:
function multiply(a) {
return function(b) {
return a * b;
};
}
const double = multiply(2);
console.log(double(5)); // Output: 10
const triple = multiply(3);
console.log(triple(5)); // Output: 15
Why it's useful: Currying promotes function reusability and can lead to cleaner and more readable code, especially in functional programming.
4. π°οΈ Debouncing and Throttling: Controlling Function Execution
Debouncing and throttling are techniques used to control the rate at which a function is executed, particularly useful in scenarios like event handling where functions can be triggered frequently.
Debouncing ensures that a function is executed only after a certain amount of time has passed since the last time it was invoked.
Throttling ensures that a function is executed at most once in a specified time period.
Debouncing Example:
function debounce(fn, delay) {
let timeoutId;
return function(...args) {
clearTimeout(timeoutId);
timeoutId = setTimeout(() => {
fn(...args);
}, delay);
};
}
const handleResize = debounce(() => {
console.log('Window resized');
}, 300);
window.addEventListener('resize', handleResize);
Why it's useful: Debouncing and throttling help to optimize performance by limiting the number of times a function is executed in response to events like scrolling, resizing, or typing.
5. π The Module Pattern: Encapsulating Code
The Module Pattern is a design pattern used to create encapsulated modules, keeping certain parts of your code private while exposing only what is necessary. This is particularly useful for organizing code in larger projects.
Example:
const CounterModule = (function() {
let counter = 0;
function increment() {
counter++;
}
function getCounter() {
return counter;
}
return {
increment,
getCounter
};
})();
CounterModule.increment();
console.log(CounterModule.getCounter()); // Output: 1
Why it's useful: The Module Pattern allows you to encapsulate implementation details, reducing the risk of naming conflicts and keeping your global namespace clean.
These lesser-known JavaScript concepts can elevate your coding skills and make your code more efficient, maintainable, and elegant. π Whether youβre optimizing performance with memoization, enhancing code readability with currying, or controlling function execution with debouncing and throttling, these techniques are powerful tools in any developer's arsenal.
So, next time you write JavaScript, try incorporating these hidden gems into your code and watch how they transform your development process!
Happy coding! π
Subscribe to my newsletter
Read articles from Vivek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
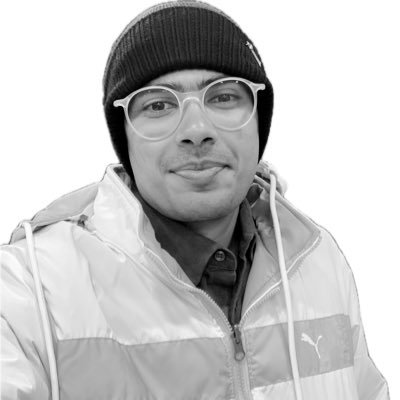
Vivek
Vivek
Curious Full Stack Developer wanting to try hands on β¨οΈ new technologies and frameworks. More leaning towards React these days - Next, Blitz, Remix π¨π»βπ»