Making Your React App Multilingual
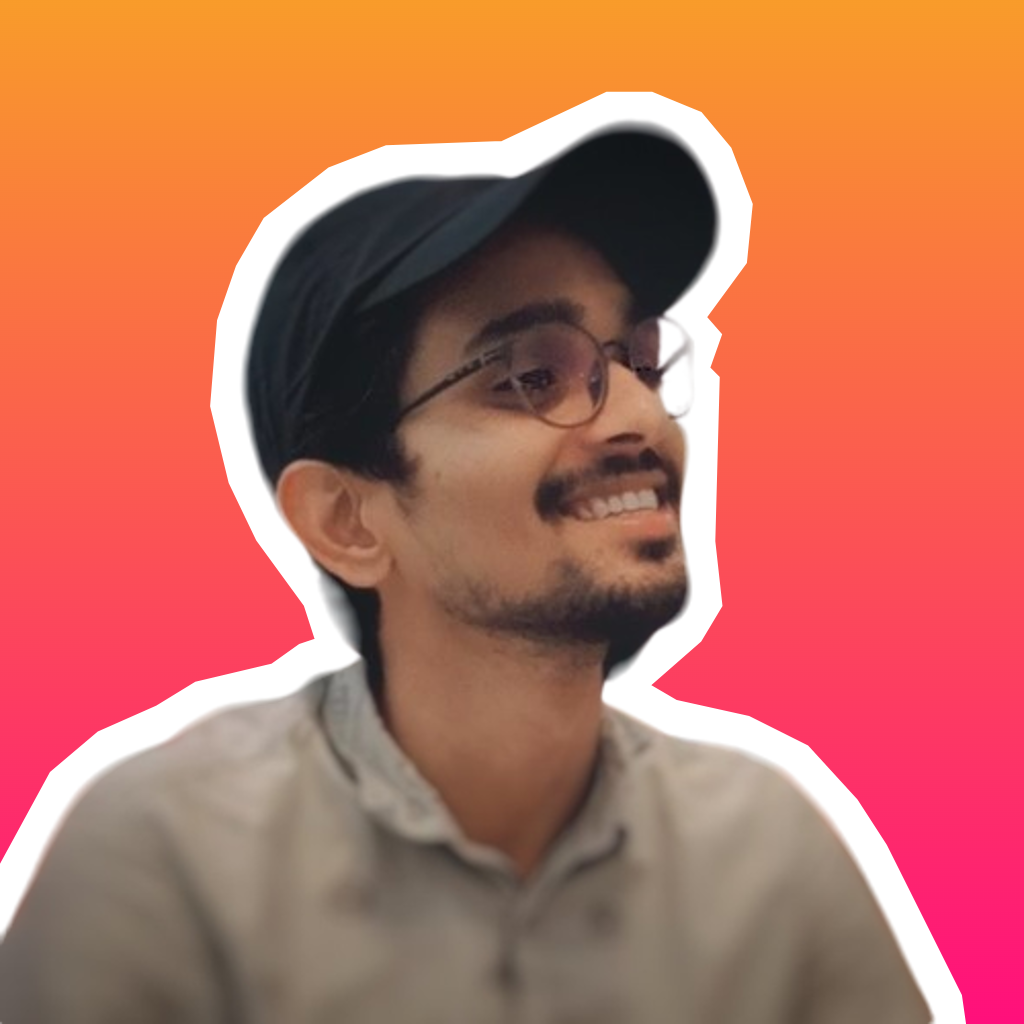
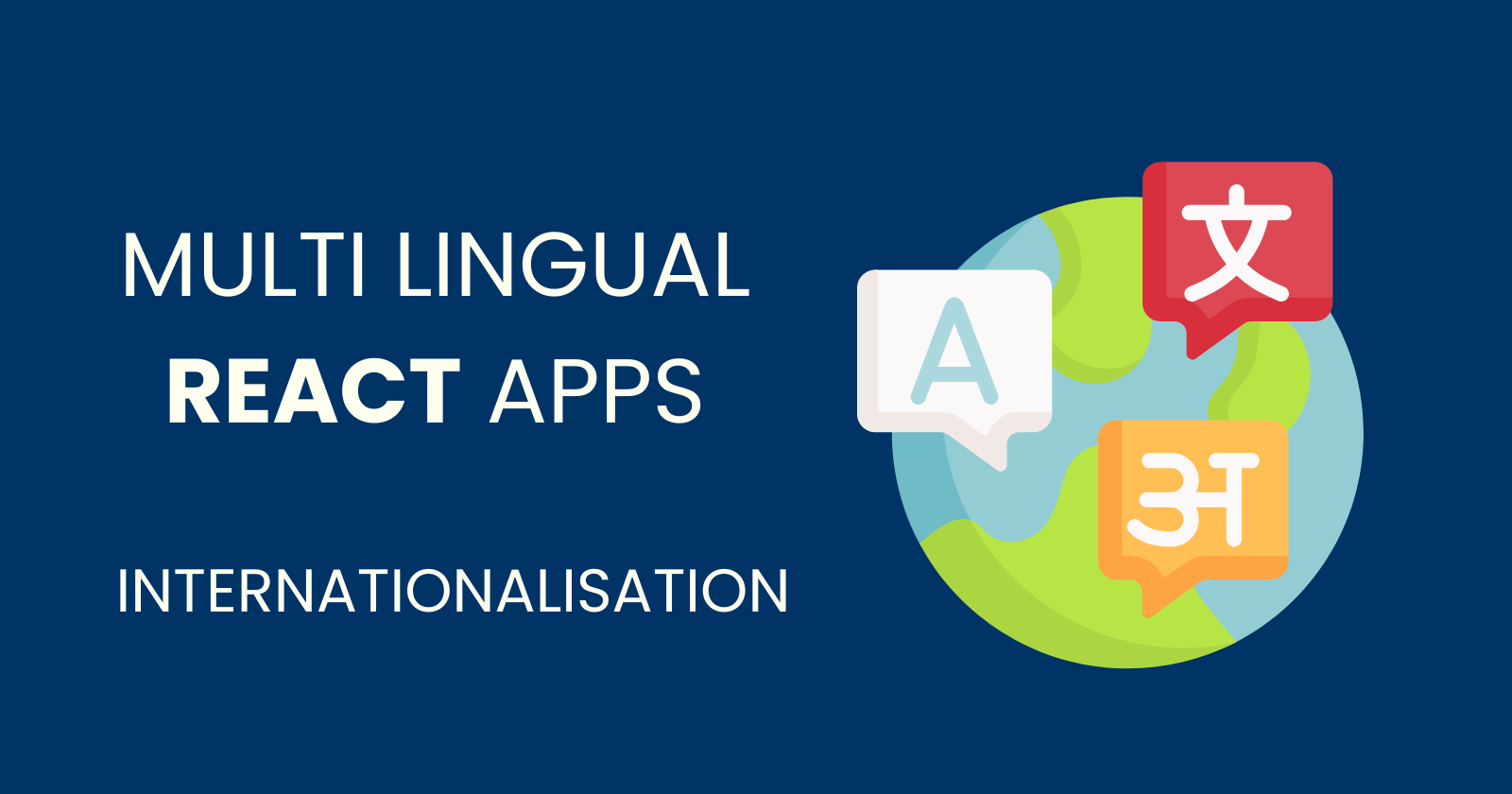
Internationalised software supports the languages and cultural customs of people throughout the world. The Web reaches all parts of the world. Internationalised web apps provide a great user experience for people everywhere.
Localised software adapts to a specific language and culture by translating text into the user's language and formatting data in accordance with the user's expectations. An app is typically localised for a small set of locales.
Typically, web apps are localised to just the language or language-region combination. Examples of such locale codes are:
en for English
en-US for English as spoken in the United States
en-GB for English as spoken in the United Kingdom
es-AR for Spanish as spoken in Argentina
ar-001 for Arabic as spoken throughout the world
ar-AE for Arabic as spoken in United Arab Emirates
Create a new Next.js project by using below command line:
npx create-next-app@latest nextjs-app-router-i18n
Add React Intl dependency
npm i -S react react-intl
Create localisation files
The en.json file
{
"hello": "Hello, world!",
"welcome": "Welcome to our website"
}
The es.json file
{
"hello": "Hola Mundo",
"welcome": "Bienvenido a nuestro sitio web"
}
The fr.json file
{
"hello": "Bonjour le monde",
"welcome": "Bienvenue sur notre site Internet"
}
Import useTranslation
from react-i18next
in your components
// Page.js Entry point
'use client'
import React, {
useState
} from 'react';
import {
FormattedMessage,
IntlProvider
} from 'react-intl';
import messages_en from '../public/locales/en.json';
import messages_fr from '../public/locales/fr.json';
import messages_sp from '../public/locales/sp.json';
import messages_hi from '../public/locales/hindi.json';
import messages_rs from '../public/locales/rus.json';
const messages = {
en: messages_en,
fr: messages_fr,
sp: messages_sp,
hi: messages_hi,
rs: messages_rs,
};
const App = () => {
const [locale, setLocale] = useState('en');
const handleLanguageChange = (e) => {
setLocale(e.target.value);
};
return (
<IntlProvider locale={locale} messages={messages[locale]}>
<div>
<h1><FormattedMessage id="app.title" /></h1>
<select onChange={handleLanguageChange} value={locale}>
<option value="en">English</option>
<option value="fr">French</option>
<option value="sp">Spanish</option>
<option value="hi">Hindi</option>
<option value="rs">Russian</option>
</select>
<p><FormattedMessage id="app.description" /></p>
</div>
</IntlProvider>
);
};
export default App;
Sample code link : To run the code on your browser.
Conclusion
Next.js offers pretty good support for internationalisation, though recent routing updates have added some complexity. We've shown how to integrate the React Intl library with Next.js, utilizing both the App Router and Pages Router for routing.
Subscribe to my newsletter
Read articles from Ankur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
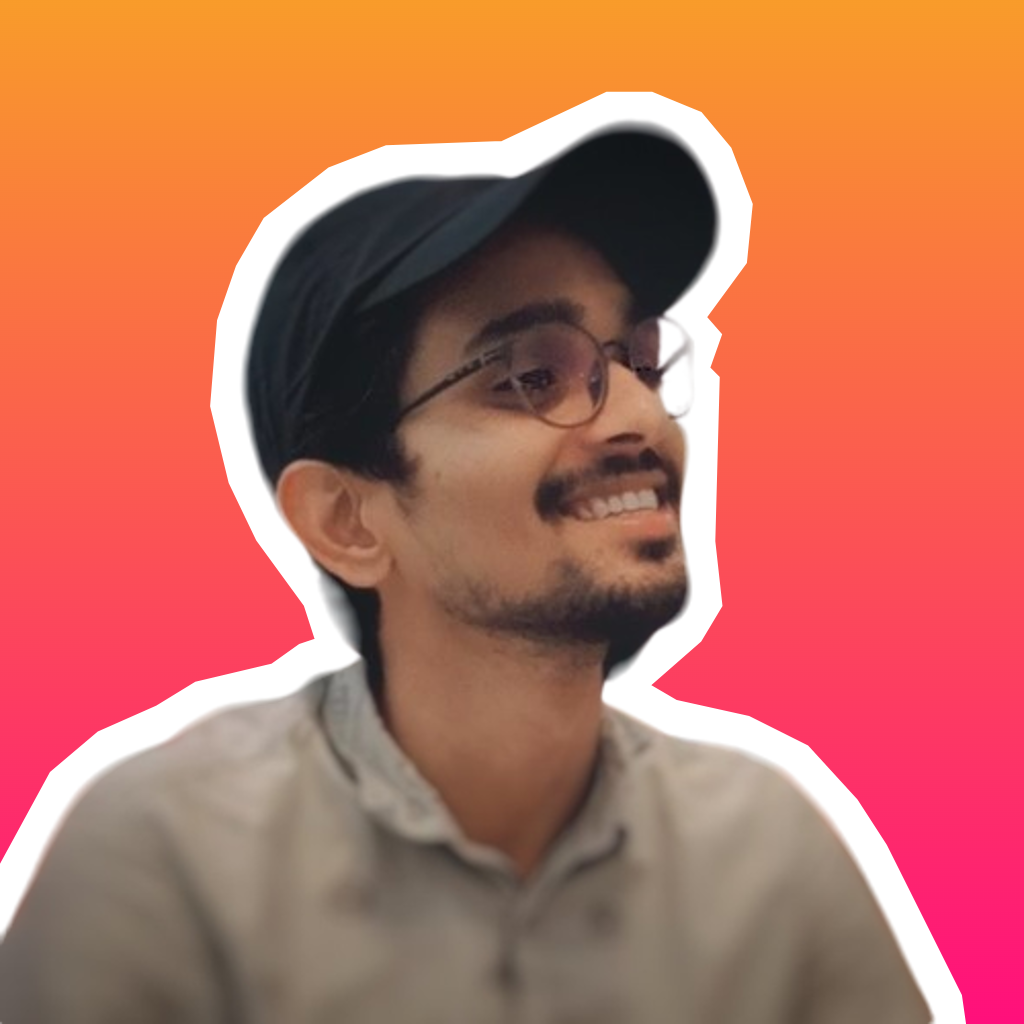