Securely Handle Nested AJAX Data in WordPress: A Step-by-Step Sanitization Guide
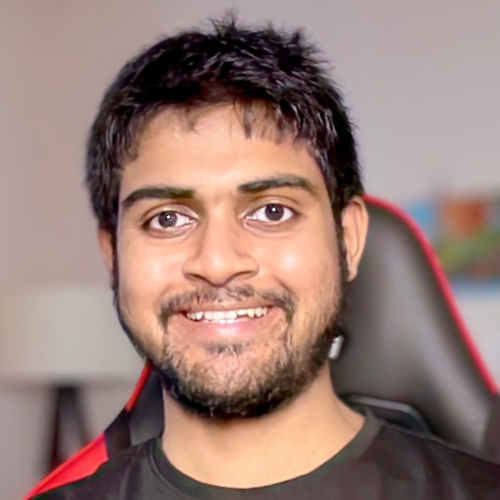
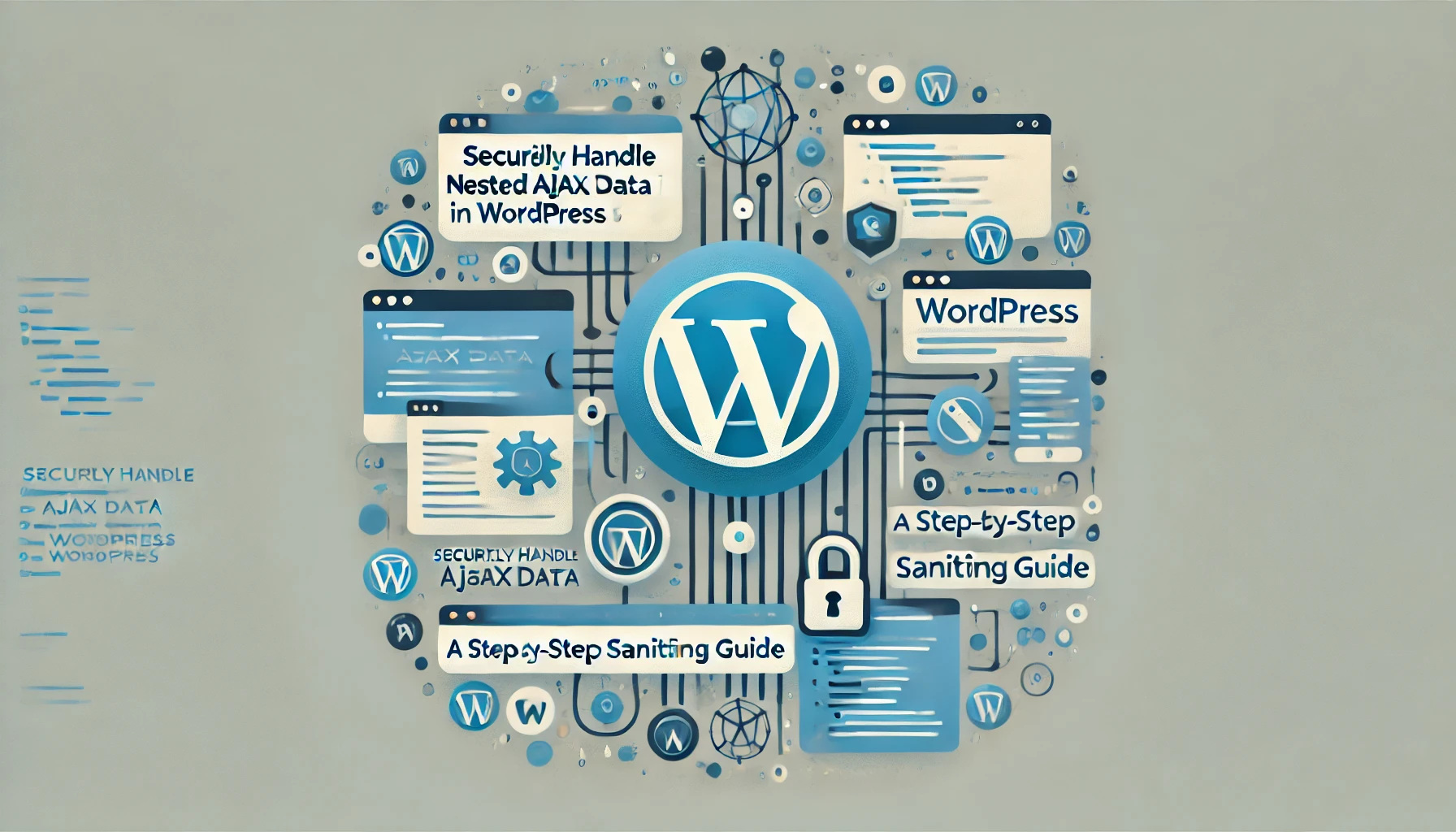
When developing WordPress applications, handling user input securely is paramount. One common scenario is when your AJAX callback receives an array of data, where each element is itself an associative array. In this tutorial, we'll walk through how to properly sanitize this kind of data to ensure it's safe to use in your application.
Understanding the Data Structure
Let's say you have an AJAX request that sends the following data structure to your server:
Array
(
[0] => Array
(
[orderId] => 31766
[email] => junaid@allnextver.com
)
[1] => Array
(
[orderId] => 31762
[email] => junaid@allnextver.com
)
[2] => Array
(
[orderId] => 31761
[email] => junaid@allnextver.com
)
)
In this structure, each element is an array containing an orderId
and an email
. Properly sanitizing this data is crucial for preventing vulnerabilities such as XSS (Cross-Site Scripting).
Step-by-Step: Sanitizing the Data
1. Setting Up Your AJAX Callback
In your WordPress plugin or theme's functions.php
file, you'll need to set up an AJAX handler. Here’s how you can create a function that sanitizes the incoming data:
add_action('wp_ajax_send_order_data', 'send_order_data');
add_action('wp_ajax_nopriv_send_order_data', 'send_order_data'); // For non-logged-in users
function send_order_data() {
// Check the nonce for security
check_ajax_referer('your_nonce_action', 'nonce');
$sanitized_data = array();
// Check if selectedOrderData is set and is an array
if (isset($_POST['selectedOrderData']) && is_array($_POST['selectedOrderData'])) { //phpcs:ignore
foreach ($_POST['selectedOrderData'] as $order) {
if (is_array($order)) {
$sanitized_order = array();
// Sanitize each property of the order array
$sanitized_order['orderId'] = sanitize_text_field(wp_unslash($order['orderId']));
$sanitized_order['email'] = sanitize_email(wp_unslash($order['email']));
// Add the sanitized order to the result array
$sanitized_data[] = $sanitized_order;
}
}
}
// Log the sanitized data for debugging
error_log(print_r($sanitized_data, true), 0);
// Send a success response
wp_send_json_success('Data logged successfully.');
}
2. Explanation of the Code
Nonce Check: The
check_ajax_referer()
function verifies that the AJAX request is valid and not forged. Replace'your_nonce_action'
with your actual nonce action name.Data Checking: The code checks whether the
selectedOrderData
POST variable is set and is an array.Loop Through Orders: It uses a
foreach
loop to iterate over each order in theselectedOrderData
array.Sanitization: Each property (
orderId
andemail
) of the associative array is sanitized using:sanitize_text_field()
for theorderId
, which strips out any unwanted HTML tags.sanitize_email()
for theemail
, which ensures the email address is properly formatted.
Logging: The sanitized data is logged for debugging purposes using
error_log()
. This can help you verify that the data has been sanitized correctly.Response: Finally, a success response is sent back to the client.
3. Example AJAX Request
Here's how you might structure your AJAX request from JavaScript:
jQuery(document).ready(function($) {
$('#sendOrderButton').on('click', function() {
const selectedOrderData = [
{ orderId: '31766', email: 'junaid@allnextver.com' },
{ orderId: '31762', email: 'junaid@allnextver.com' },
{ orderId: '31761', email: 'junaid@allnextver.com' },
];
$.ajax({
url: ajaxurl, // This should be defined in your script localization
method: 'POST',
data: {
action: 'send_order_data',
nonce: your_nonce_value, // Replace with your actual nonce value
selectedOrderData: selectedOrderData
},
success: function(response) {
console.log(response.data); // Handle success
},
error: function(jqXHR, textStatus, errorThrown) {
console.error('Error:', errorThrown); // Handle error
}
});
});
});
4. Testing
To test the functionality:
Make sure you trigger the AJAX request (e.g., by clicking a button).
Check the server logs for the sanitized output.
Verify that the data in your logs reflects the sanitized input.
Conclusion
Sanitizing data received via AJAX in WordPress is essential for maintaining security and data integrity. By using functions like sanitize_text_field()
and sanitize_email()
, you can ensure that the data is safe for processing. This tutorial provides a clear example of how to handle nested arrays in AJAX callbacks and highlights best practices for sanitization.
Feel free to adapt this example to suit your needs and remember to always validate and sanitize user inputs in your applications!
Subscribe to my newsletter
Read articles from Junaid Bin Jaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
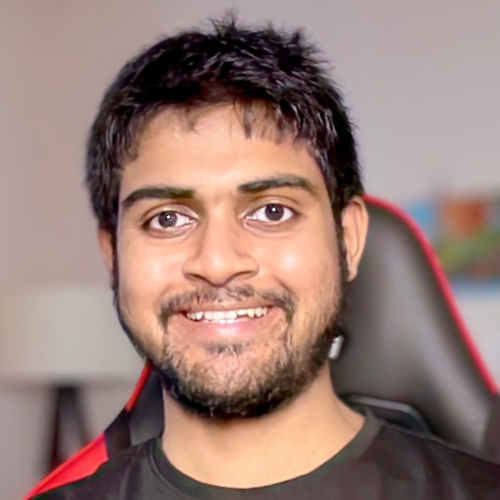
Junaid Bin Jaman
Junaid Bin Jaman
Hello! I'm a software developer with over 6 years of experience, specializing in React and WordPress plugin development. My passion lies in crafting seamless, user-friendly web applications that not only meet but exceed client expectations. I thrive on solving complex problems and am always eager to embrace new challenges. Whether it's building robust WordPress plugins or dynamic React applications, I bring a blend of creativity and technical expertise to every project.