Discovering a Critical Bug in emu8086: Incorrect Handling of Word-Sized Immediate Values
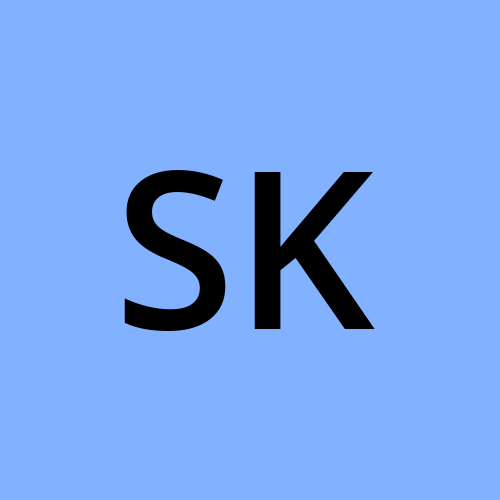
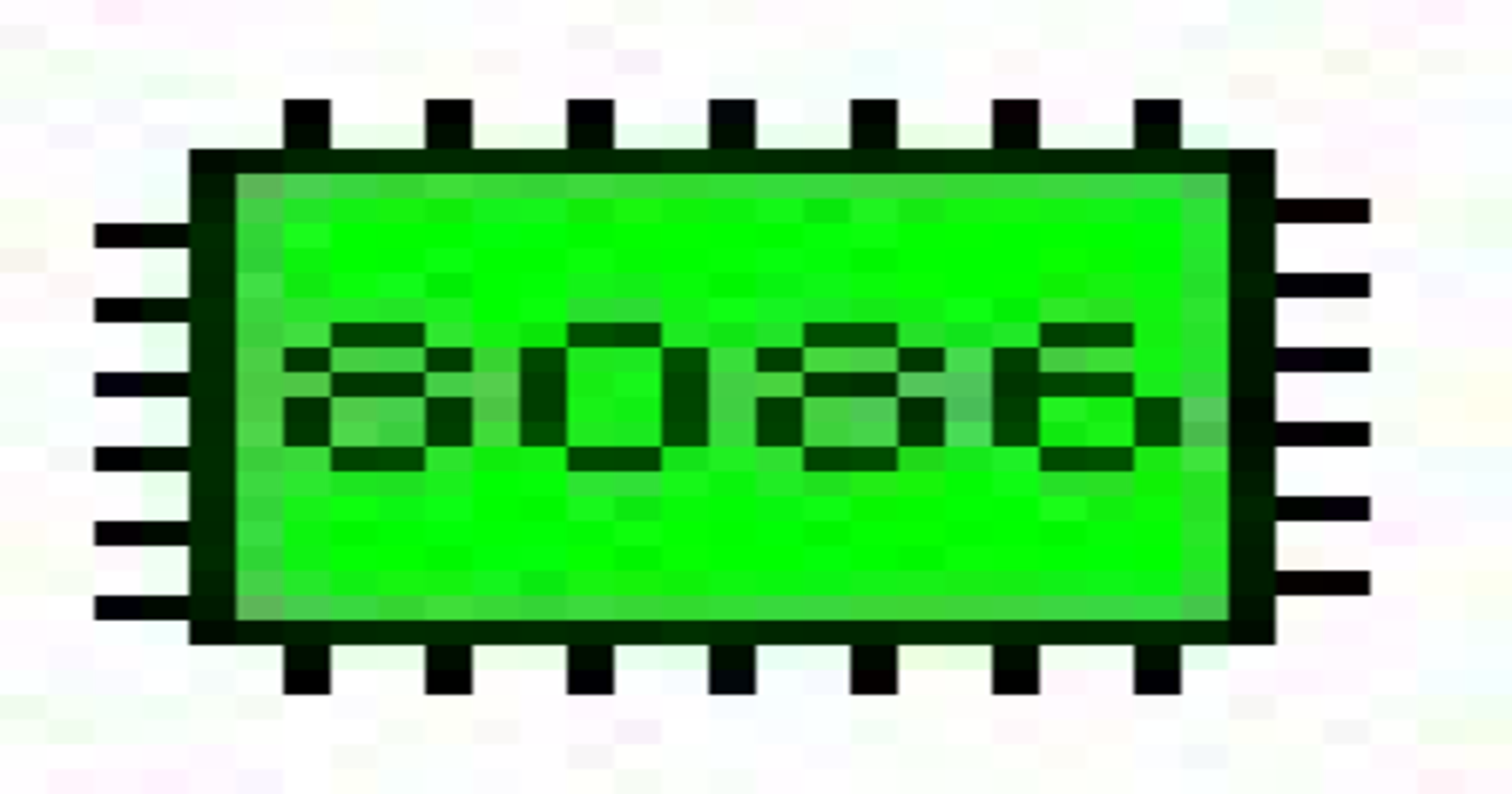
Introduction
Assembly language programming offers unparalleled control over hardware, making emulators like emu8086
invaluable tools for learning and development. However, even the most robust emulators can harbor hidden bugs that disrupt the learning experience and lead to confusion. Recently, I uncovered a significant issue in the emu8086
emulator related to how it handles word-sized immediate values during memory operations. This blog post delves into the nature of this bug, its implications, and steps taken to report it to the developer.
Background: Understanding emu8086 and Segmented Memory
emu8086
is a popular emulator designed to simulate the Intel 8086 microprocessor environment. It is widely used by students and enthusiasts to learn assembly language programming without the need for actual hardware. The emulator replicates the segmented memory architecture of the 8086, where memory addresses are calculated using segment registers (like DS for Data Segment) combined with offset values.
Segmented Memory Architecture Recap
In the x86 architecture, memory addresses are specified using a combination of a segment and an offset:
Effective Address=Segment×16+Offset\text{Effective Address} = \text{Segment} \times 16 + \text{Offset}Effective Address=Segment×16+Offset
For example, with DS = 0x0900
and SI = 0x00FF
, the effective address calculates to:
0x0900×0x10+0x00FF=0x9000+0x00FF=0x90FF0x0900 \times 0x10 + 0x00FF = 0x9000 + 0x00FF = 0x90FF0x0900×0x10+0x00FF=0x9000+0x00FF=0x90FF
Understanding this calculation is crucial for accurately manipulating memory in assembly programs.
The Bug: Incorrect Handling of Word-Sized Immediate Values
Description of the Issue
While working on a simple assembly program to move data to and from memory, I encountered unexpected behavior in emu8086
. Specifically, when moving word-sized immediate values into memory, the emulator incorrectly handles cases where the most significant byte (MSB) is 0x00
. Instead of storing both bytes, it only stores the least significant byte (LSB), leading to corrupted data when attempting to retrieve the stored word.
Code Example Demonstrating the Bug
Here's a snippet of the assembly code that reveals the issue:
assemblyCopy code; Initialize segment and registers
mov bx, 0xfff0
mov [bx], 0x1020 ; Expected: DS:FFF0 = 0x20, DS:FFF1 = 0x10
mov cx, 0x0040
mov [bx-1], cx ; Expected: DS:FFEF = 0x40, DS:FFEE = 0x00
mov bx, 0xfff3
mov [bx], 0x30 ; Expected: DS:FFF3 = 0x30
mov [bx-1], 0x0005 ; Expected: DS:FFF2 = 0x05, DS:FFF1 = 0x00
mov dx, [bx-1] ; Expected: DX = 0x0005
mov [bx-1], 0x0607 ; Expected: DS:FFF2 = 0x07, DS:FFF1 = 0x06
mov [bx-1], 0x0800 ; Expected: DS:FFF2 = 0x00, DS:FFF1 = 0x08
Observed Behavior
mov [bx], 0x1020
: Correctly stores0x20
atDS:FFF0
and0x10
atDS:FFF1
.mov [bx-1], 0x0005
: Stores0x05
atDS:FFF2
, but ignores the MSB0x00
atDS:FFF1
, leaving it unchanged or incorrectly modified.mov dx, [bx-1]
: Instead of loading0x0005
, it incorrectly loads0x3050
from memory due to the corrupted storage.
However, when the MSB is non-zero, as in mov [bx-1], 0x0607
, the emulator behaves correctly, storing both bytes (0x07
at DS:FFF2
and 0x06
at DS:FFF1
).
Analysis: Why Does This Happen?
Real CPU Behavior
In a genuine x86 CPU, the MOV
instruction with a word-sized immediate value will always store both bytes, regardless of their values. The operation adheres to little-endian format, ensuring that the LSB is stored at the lower memory address and the MSB at the higher address.
Emulator Behavior
emu8086
, however, appears to have a flaw in handling word-sized immediate values where the MSB is 0x00
. Instead of storing both bytes, it omits the MSB, leading to incomplete data storage. This behavior deviates from the actual CPU's operation, resulting in corrupted data during retrieval.
Impact of the Bug
This bug undermines the reliability of emu8086
for learning and development purposes. Users relying on accurate memory operations may encounter unexpected behavior, leading to confusion and potential frustration. Moreover, such discrepancies can hinder the learning process, as the emulator does not faithfully replicate the CPU's behavior in all scenarios.
Steps to Reproduce the Bug
Set Up the Environment: Ensure you have
emu8086
installed and configured with the default data segment (DS = 0x0700
).Write the Assembly Code: Use the provided code snippet that moves word-sized immediate values into memory.
Run the Program: Execute the program within
emu8086
and observe the memory contents after eachMOV
operation.Verify the Results: Compare the stored values against expected results, noting discrepancies when the MSB is
0x00
.
Comparison with Real CPU Execution
Running the same code on actual hardware or a more accurate emulator like Bochs or QEMU should yield correct storage of both bytes. For instance:
mov [bx-1], 0x0005
on real hardware:DS:FFF2 = 0x05
DS:FFF1 = 0x00
mov dx, [bx-1]
would correctly load0x0005
intoDX
.
Reporting the Bug
To ensure that emu8086
is improved and remains a reliable tool for learners, reporting this bug to the developer is essential.
Subscribe to my newsletter
Read articles from Sameera Khatoon directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
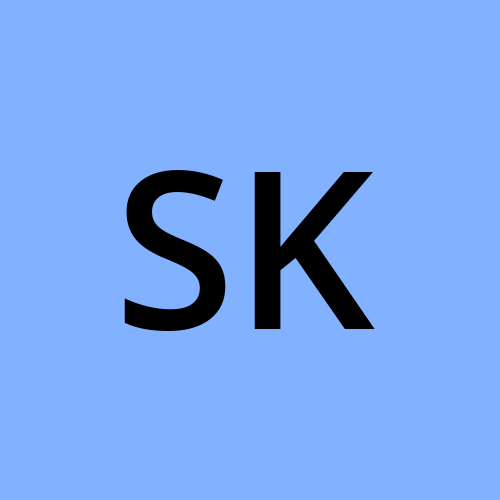