require() vs import: What You Need to Know in JavaScript
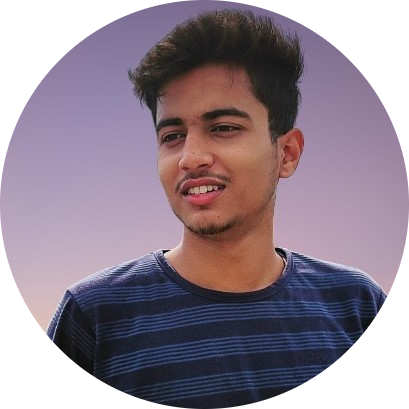
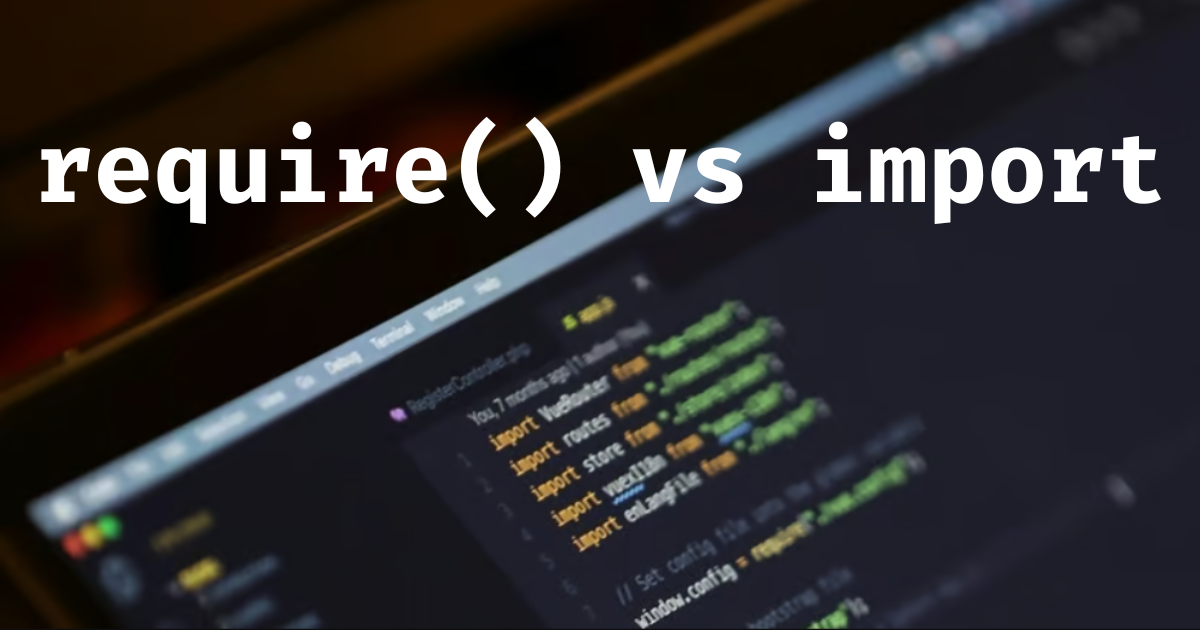
When I first started coding, one of the things that baffled me was seeing some JavaScript files using require()
to import modules while others used import
. This inconsistency across projects left me puzzled. If you’ve ever been confused about this too, keep reading—I’m here to clear things up!
What is CommonJS?
CommonJS is a set of standards used to implement modules in server-side JavaScript, primarily in Node.js environments. In CommonJS, modules are loaded synchronously, meaning the script execution is paused until the module is completely loaded. While this makes for a straightforward approach, it can cause performance issues, especially when loading multiple modules. This is because each module has to load one after the other, potentially delaying the execution of your script.
In CommonJS, you use module.exports
to export functionality from a module and require()
to import it into another file.
Here’s an example of how this looks in code:
// In file multiply.js
module.exports = function multiply(x, y) {
return x * y;
};
// In file main.js
const multiply = require('./multiply.js');
console.log(multiply(5, 4)); // Output: 20
What is ECMAScript (ES6)?
ECMAScript 6, commonly known as ES6, is a newer version of JavaScript released in 2015. One of the key features of ES6 is the ability to import modules asynchronously using the import
and export
statements. Asynchronous loading means that the script can continue to run while the module is being loaded, avoiding bottlenecks and improving performance.
Another advantage of ES6 modules is "tree-shaking," a process where only the parts of the module that are actually used are loaded, leaving out any dead code. This helps optimize the performance of your application by reducing the size of the code loaded into the browser.
Here’s the previous example using import
and export
with ES6:
// In file multiply.js
export const multiply = (x, y) => x * y;
// In file main.js
import { multiply } from './multiply.js';
console.log(multiply(5, 4)); // Output: 20
The Main Differences: require()
vs. import
Module System:
require()
is part of the CommonJS module system, whileimport
is part of the ES6 module system.Usage:
require()
is primarily used in Node.js environments for server-side development, especially in legacy projects that haven't yet adopted ES6. On the other hand,import
is used in both server-side and frontend development, particularly in newer projects and with frontend frameworks like React or Vue.Loading:
require()
loads modules synchronously, whileimport
allows for asynchronous loading, leading to better performance.Optimization: ES6’s
import
can be statically analyzed by tools, enabling optimizations like tree-shaking, which reduces bundle size and improves load times.
Why is import
Better Than require()
?
The import
statement, being asynchronous, can significantly improve performance, especially in larger applications. Since import
can be statically analyzed, tools like linters and bundlers can better optimize your code, leading to smaller, more efficient bundles. Moreover, the import
syntax is generally easier to read and understand, improving the overall developer experience.
When Should You Use require()
vs. import
?
Use
require()
when:You’re working on a legacy Node.js project that predates ES6 and hasn’t been updated.
You need to dynamically load modules at runtime, such as in a configuration file or conditionally loaded modules.
Use
import
when:- Almost any other time, as it’s the current standard and generally more efficient.
Summary
In summary, it's recommended to use import
whenever possible, as it offers more benefits and is the newer, more widely adopted module system. However, there are cases where require()
might still be the better choice, depending on your specific needs and environment. Understanding these differences will help you make more informed decisions and write more efficient, modern JavaScript code.
Subscribe to my newsletter
Read articles from Saurav Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
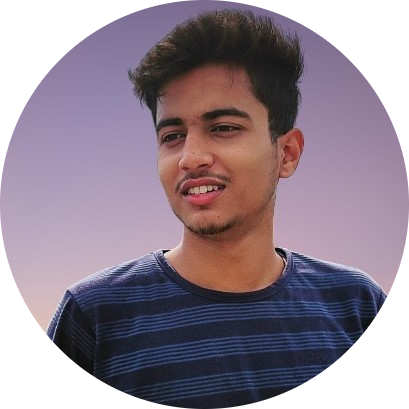
Saurav Kumar
Saurav Kumar
Build WebApps | Powered by Next.js, Node.js, Express.js, MongoDB & many more Styling, Auth, Db, Security, Deployment Tools & Techs