A Comprehensive Guide to Hypothesis in Python: From Coding Practice to Advanced API Testing

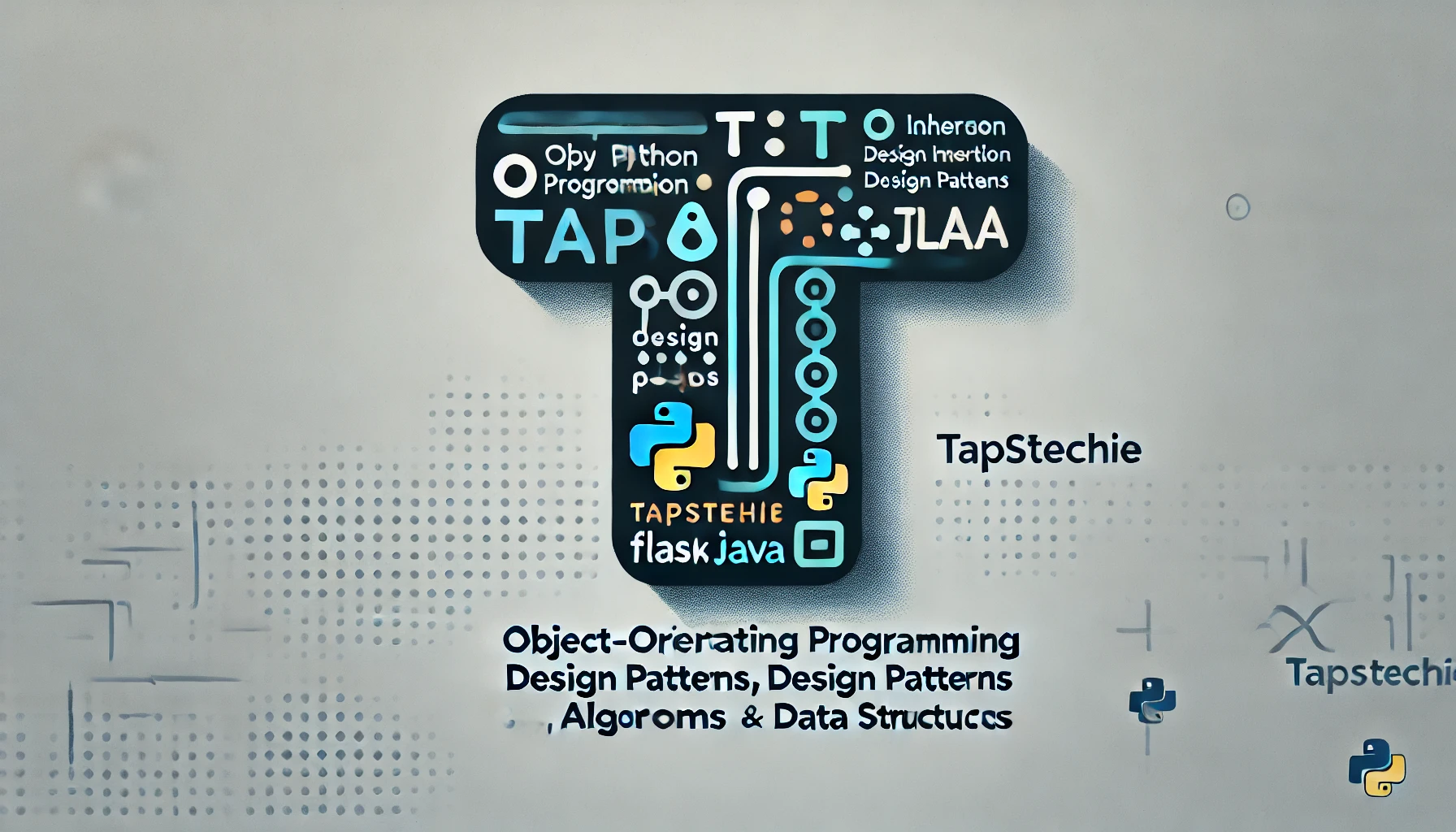
Introduction to Hypothesis
Testing is an essential part of software development, but writing exhaustive test cases can be both time-consuming and error-prone. Hypothesis is a powerful Python library that simplifies this process by automatically generating a wide range of test cases based on the properties you define. Whether you’re preparing for coding interviews or building complex APIs, Hypothesis can help you ensure your code is robust and handles all possible scenarios.
Getting Started with Hypothesis
What is Hypothesis?
Hypothesis is a property-based testing library for Python. Instead of manually writing individual test cases, you define properties your code should satisfy, and Hypothesis automatically generates inputs to test those properties. This not only saves time but also helps uncover edge cases you might not have thought of.
Installation:
Before you start using Hypothesis, you need to install it along with pytest
, a popular testing framework that integrates seamlessly with Hypothesis.
pip install hypothesis pytest
Basic Usage: Testing Simple Functions
Let’s start with a simple example. Suppose you want to test a function that reverses a string. Instead of writing multiple test cases, you can define a property: reversing a string twice should return the original string.
from hypothesis import given
from hypothesis import strategies as st
def reverse_string(s: str) -> str:
return s[::-1]
@given(st.text())
def test_reverse_string(s):
assert reverse_string(reverse_string(s)) == s
Here:
@given(st.text())
tells Hypothesis to generate a wide range of strings.The test checks that reversing the string twice returns the original string.
Seeing the Test Cases in Action
You can observe how Hypothesis generates different test cases by adding a print
statement in your test function.
@given(st.text())
def test_reverse_string(s):
print(f"Testing with input: {s}")
assert reverse_string(reverse_string(s)) == s
When you run this with pytest
:
pytest -s
You’ll see the various strings that Hypothesis generates and tests.
Understanding Hypothesis Strategies
Hypothesis uses strategies to generate input data. Strategies are the building blocks of Hypothesis and can be used to generate basic types, complex data structures, and even custom data types.
Basic Types
Hypothesis provides strategies for generating a wide range of basic types:
st.integers()
: Generates random integers.st.text()
: Generates random strings.st.booleans()
: Generates random boolean values.st.floats()
: Generates random floating-point numbers.
Example:
@given(st.integers())
def test_is_positive(n):
assert n >= 0 or n < 0 # Trivial property just for demonstration
Complex Data Types
You can also generate more complex data structures like lists, dictionaries, and tuples.
Example: Generating lists of integers.
@given(st.lists(st.integers(), min_size=1))
def test_find_max(numbers):
assert max(numbers) == sorted(numbers)[-1] # Simplified property
Custom Data Types
Hypothesis allows you to create custom strategies using st.builds()
or st.composite()
. This is useful when you need to generate complex objects or domain-specific data.
Example: Generating a custom object.
from dataclasses import dataclass
@dataclass
class User:
name: str
age: int
user_strategy = st.builds(User, name=st.text(), age=st.integers(min_value=0))
@given(user_strategy)
def test_user_creation(user):
assert isinstance(user.name, str)
assert isinstance(user.age, int)
Using Hypothesis for Coding Interviews
When practicing for coding interviews, Hypothesis can help you automatically generate test cases for your solutions, ensuring they work across a wide range of inputs.
Example: Testing a function that finds the maximum number in a list.
def find_max(numbers: list) -> int:
return max(numbers)
@given(st.lists(st.integers(), min_size=1))
def test_find_max(numbers):
assert find_max(numbers) == max(numbers)
Advanced Usage: Testing APIs with Hypothesis
Hypothesis shines when testing more complex scenarios like APIs. You can use it to generate various inputs, including query parameters, path parameters, and request bodies, to ensure your API handles different cases correctly.
Example: Testing a Simple Login API
Consider a Flask-based login API:
from flask import Flask, request, jsonify
app = Flask(__name__)
users = {"user1": "password123", "user2": "securepassword"}
@app.route('/login', methods=['POST'])
def login():
data = request.json
username = data.get('username')
password = data.get('password')
if not username or not password:
return jsonify({"error": "Username and password required"}), 400
if username in users and users[username] == password:
return jsonify({"message": "Login successful"}), 200
else:
return jsonify({"error": "Invalid username or password"}), 401
if __name__ == '__main__':
app.run(debug=True)
You can use Hypothesis to test this API by generating different login credentials:
import pytest
from hypothesis import given, strategies as st
from hypothesis.extra import requests as hyp_requests
API_URL = "http://localhost:5000/login"
valid_login_strategy = st.fixed_dictionaries({
"username": st.sampled_from(["user1", "user2"]),
"password": st.sampled_from(["password123", "securepassword"])
})
invalid_login_strategy = st.fixed_dictionaries({
"username": st.one_of(st.none(), st.text()),
"password": st.one_of(st.none(), st.text())
})
login_strategy = st.one_of(valid_login_strategy, invalid_login_strategy)
@given(login_strategy)
def test_login_api(data):
response = hyp_requests.post(API_URL, json=data)
if data["username"] in users and data["password"] == users[data["username"]]:
assert response.status_code == 200
assert response.json() == {"message": "Login successful"}
else:
assert response.status_code in [400, 401]
assert "error" in response.json()
Handling Path Parameters in API Testing
Suppose your API has an endpoint like /abc/<ID>/def
, and you need to test various ID
values:
API_URL_TEMPLATE = "http://localhost:5000/abc/{}/def"
id_strategy = st.text(min_size=1, max_size=5)
@given(id_strategy)
def test_api_with_path_parameter(id_value):
api_url = API_URL_TEMPLATE.format(id_value)
response = hyp_requests.get(api_url)
if id_value in valid_ids:
assert response.status_code == 200
else:
assert response.status_code == 404
This test ensures your API correctly handles both valid and invalid ID
values.
Testing Negative Scenarios
Hypothesis is particularly useful for testing negative scenarios, ensuring your code handles invalid or unexpected inputs gracefully.
Example: Testing for invalid login credentials.
invalid_login_strategy = st.fixed_dictionaries({
"username": st.one_of(st.none(), st.text().filter(lambda x: not x)),
"password": st.one_of(st.none(), st.text().filter(lambda x: not x))
})
@given(invalid_login_strategy)
def test_invalid_login(data):
response = hyp_requests.post(API_URL, json=data)
assert response.status_code == 400
assert "error" in response.json()
This test ensures that missing or empty fields result in the correct error responses.
Testing Service Methods and Database Interactions
Hypothesis can also be applied to test service methods and database interactions, ensuring that your backend logic handles various scenarios correctly.
Example: Testing a service method that interacts with a database.
def get_user_details(user_id: str) -> dict:
user = db.find_user_by_id(user_id)
if user:
return {"id": user.id, "name": user.name}
else:
return {"error": "User not found"}
@given(st.text(min_size=1, max_size=10))
def test_get_user_details(user_id):
result = get_user_details(user_id)
if user_id in db:
assert "name" in result
else:
assert result == {"error": "User not found"}
This ensures that the method behaves correctly whether the user exists in the database or not.
Conclusion
Hypothesis is a versatile and powerful tool that can greatly enhance your testing process, from simple coding exercises to complex API and database testing. By automating the generation of test cases and covering a wide range of scenarios, Hypothesis helps you ensure your code is robust, reliable, and ready for any challenge.
Whether you’re preparing for a coding interview, building a new API, or testing backend services, Hypothesis offers a comprehensive solution to make your testing process more efficient and effective.
This guide provides a detailed overview of how to use Hypothesis, from basic examples to more advanced scenarios, ensuring you have everything you need in one place. With Hypothesis, you can test with confidence, knowing that your code is thoroughly checked against a wide range of inputs and potential edge cases.
References
For more information about Hypothesis, check out the official Hypothesis documentation. This resource provides comprehensive details on all features, strategies, and advanced usage scenarios.
Subscribe to my newsletter
Read articles from Tarun Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Tarun Sharma
Tarun Sharma
Hi there! I’m Tarun, a Senior Software Engineer with a passion for technology and coding. With experience in Python, Java, and various backend development practices, I’ve spent years honing my skills and working on exciting projects. On this blog, you’ll find insights, tips, and tutorials on topics ranging from object-oriented programming to tech trends and interview prep. My goal is to share valuable knowledge and practical advice to help fellow developers grow and succeed. When I’m not coding, you can find me exploring new tech trends, working on personal projects, or enjoying a good cup of coffee. Thanks for stopping by, and I hope you find my content helpful!