React Performance Optimization: Debouncing and Throttling Explained

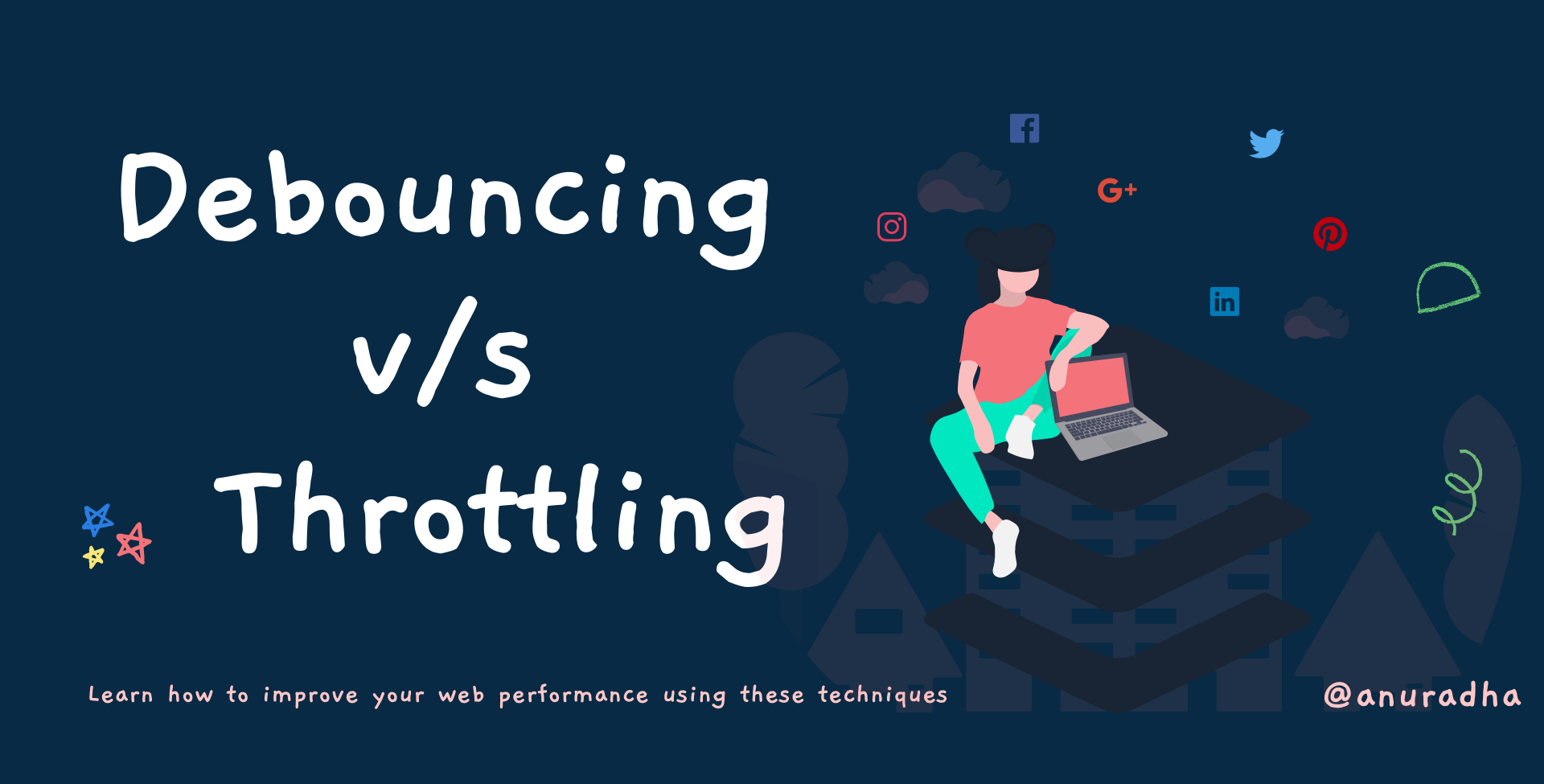
Introduction
Importance of Performance Optimization in React
In modern web development, user experience is paramount, and performance plays a crucial role in ensuring smooth interactions. React, as a popular JavaScript library, allows developers to build dynamic and responsive applications. However, with great power comes the need for careful performance optimization to avoid unnecessary re-renders, slow user interactions, and excessive resource usage. This article delves into two essential techniques for performance optimization in React: debouncing and throttling.
Overview of Debouncing and Throttling
Debouncing and throttling are techniques used to control the rate at which a function is executed. They are particularly useful for managing expensive operations like API calls, event handling, and DOM updates that occur frequently due to user interactions. Understanding when and how to use these techniques can significantly improve the performance and responsiveness of your React applications.
Purpose of the Article
This article aims to provide a clear and practical understanding of debouncing and throttling in React. We’ll explore how each technique works, their use cases, and how to implement them effectively in your React projects.
Understanding Debouncing
Definition of Debouncing
Debouncing is a technique that delays the execution of a function until a specified amount of time has passed since it was last invoked. If the function is triggered again before the time has elapsed, the timer resets. This ensures that the function is executed only after a period of inactivity.
How Debouncing Works
Imagine a user typing into a search input field. Without debouncing, every keystroke would trigger an API call, leading to a flood of requests. With debouncing, the API call is delayed until the user stops typing for a moment, reducing the number of requests and improving performance.
Use Cases for Debouncing
Search Input Fields: When implementing a search feature that queries an API based on user input, debouncing prevents excessive API calls by waiting until the user has stopped typing.
Window Resizing: Debouncing the resize event handler ensures that layout recalculations or DOM updates are performed only after the user has finished resizing the window, rather than on every single pixel change.
Implementing Debouncing in React
Using Lodash's debounce
Function
Lodash, a popular JavaScript utility library, provides a debounce
function that is easy to use in React applications. Here’s how you can implement it:
javascriptCopy codeimport React, { useCallback } from 'react';
import { debounce } from 'lodash';
const SearchInput = ({ onSearch }) => {
const debouncedSearch = useCallback(
debounce((query) => onSearch(query), 300),
[]
);
const handleChange = (e) => {
debouncedSearch(e.target.value);
};
return <input type="text" onChange={handleChange} placeholder="Search..." />;
};
export default SearchInput;
In this example, the debounce
function ensures that the onSearch
function is called only after 300 milliseconds of inactivity.
Custom Debouncing Logic
If you prefer not to use a third-party library, you can implement debouncing with custom logic:
javascriptCopy codeimport React, { useState, useEffect } from 'react';
const useDebounce = (value, delay) => {
const [debouncedValue, setDebouncedValue] = useState(value);
useEffect(() => {
const handler = setTimeout(() => {
setDebouncedValue(value);
}, delay);
return () => {
clearTimeout(handler);
};
}, [value, delay]);
return debouncedValue;
};
const SearchInput = ({ onSearch }) => {
const [query, setQuery] = useState('');
const debouncedQuery = useDebounce(query, 300);
useEffect(() => {
onSearch(debouncedQuery);
}, [debouncedQuery, onSearch]);
return <input type="text" value={query} onChange={(e) => setQuery(e.target.value)} placeholder="Search..." />;
};
export default SearchInput;
This custom hook, useDebounce
, provides a reusable way to debounce any value in your React components.
Understanding Throttling
Definition of Throttling
Throttling is a technique that limits the execution of a function to once every specified period, regardless of how many times it is triggered. Unlike debouncing, throttling ensures that a function is called at regular intervals during continuous activity.
How Throttling Works
Consider a scenario where a user is scrolling through a long list of items. Without throttling, every tiny scroll movement could trigger an expensive operation like rendering additional items. Throttling ensures that this operation is performed at regular intervals, reducing the load on the browser.
Use Cases for Throttling
Scrolling Events: Throttling scroll event handlers helps maintain smooth scrolling by reducing the frequency of function calls.
Window Resize Events: Throttling ensures that layout recalculations or updates happen at controlled intervals during window resizing.
Implementing Throttling in React
Using Lodash's throttle
Function
Similar to debouncing, Lodash offers a throttle
function for easy implementation:
javascriptCopy codeimport React, { useEffect } from 'react';
import { throttle } from 'lodash';
const ScrollListener = () => {
useEffect(() => {
const handleScroll = throttle(() => {
console.log('Scroll event triggered');
}, 200);
window.addEventListener('scroll', handleScroll);
return () => window.removeEventListener('scroll', handleScroll);
}, []);
return <div>Scroll down to see the effect.</div>;
};
export default ScrollListener;
In this example, the scroll event handler is throttled to execute once every 200 milliseconds.
Custom Throttling Logic
Throttling can also be implemented without external libraries:
P.S: Skip this one if its too hard to digest right now.
javascriptCopy codeimport React, { useEffect } from 'react';
const useThrottle = (callback, delay) => {
const lastCall = React.useRef(0);
return (...args) => {
const now = new Date().getTime();
if (now - lastCall.current > delay) {
lastCall.current = now;
callback(...args);
}
};
};
const ScrollListener = () => {
const handleScroll = useThrottle(() => {
console.log('Scroll event triggered');
}, 200);
useEffect(() => {
window.addEventListener('scroll', handleScroll);
return () => window.removeEventListener('scroll', handleScroll);
}, [handleScroll]);
return <div>Scroll down to see the effect.</div>;
};
export default ScrollListener;
This custom hook, useThrottle
, allows you to throttle any function in your React components.
Comparing Debouncing and Throttling
Key Differences
Debouncing: Delays function execution until a period of inactivity.
Throttling: Ensures function execution at regular intervals during continuous activity.
When to Use Debouncing versus Throttling
Use Debouncing: When you want to wait until a user has stopped performing an action before executing a function (e.g., search inputs).
Use Throttling: When you need to ensure consistent execution during an ongoing action (e.g., scroll or resize events).
Performance Implications
Both debouncing and throttling can significantly improve the performance of your React applications by reducing unnecessary function executions and preventing performance bottlenecks.
Real World example and usecases
https://css-tricks.com/debouncing-throttling-explained-examples/
This article right here is a must read for truly understanding the need and real life use case of debouncing and throttling.
Best Practices for Using Debouncing and Throttling
Choosing the Right Interval
Selecting the appropriate delay or interval is crucial. A too-short interval may negate the benefits of debouncing or throttling, while a too-long interval may make the application feel unresponsive.
Testing Performance Improvements
Always test the performance improvements gained from debouncing and throttling in a real-world scenario. Monitor the impact on user experience and adjust the intervals as necessary.
Avoiding Common Pitfalls
Overuse: Don’t apply debouncing or throttling to every event handler. Use them only where necessary.
Choosing the Wrong Technique: Understand the use case to choose the correct technique. For instance, using debouncing on a scroll event might make the user experience feel laggy, while using throttling on a search input could lead to unnecessary API calls.
Conclusion
Recap of Key Points
In this article, we've explored the importance of performance optimization in React and how debouncing and throttling can play a critical role in enhancing user experience. We discussed:
Debouncing: Delaying function execution until after a period of inactivity to prevent excessive calls.
Throttling: Limiting function execution to a set interval, ensuring consistent performance during ongoing user actions.
Implementation: How to implement these techniques in React using both Lodash and custom hooks.
Use Cases: Scenarios where each technique is most effective, including search inputs, scrolling, and window resizing.
Final Thoughts on Performance Optimization in React
Performance optimization is a crucial aspect of modern web development, and understanding when to apply debouncing and throttling can make a significant difference in the responsiveness of your React applications. By carefully choosing the right intervals and testing the effects, you can create a smoother, more user-friendly experience.
Additional Resources and Further Reading
By mastering these techniques, you’ll be better equipped to build performant and responsive React applications that delight users.
Subscribe to my newsletter
Read articles from Khalid Sayyed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Khalid Sayyed
Khalid Sayyed
Welcome to my blog! I’m an AI and Data Science undergrad with a knack for full-stack web development, machine learning, and working with LLMs. Proficient in C++, Java, JavaScript, and TypeScript, I’m into system design, microservices and creating scalable, user-friendly web apps. Follow along for some crazy tips, tricks and hacks for implementing complex services with minimal efforts. Here, I share my tech journey whilst trying to improving myself. My interests outside of code are anime, web novels, food, and swimming. Dive in and let's explore together!