From Bubbles to Order: An Insight into the Bubble Sort Algorithm
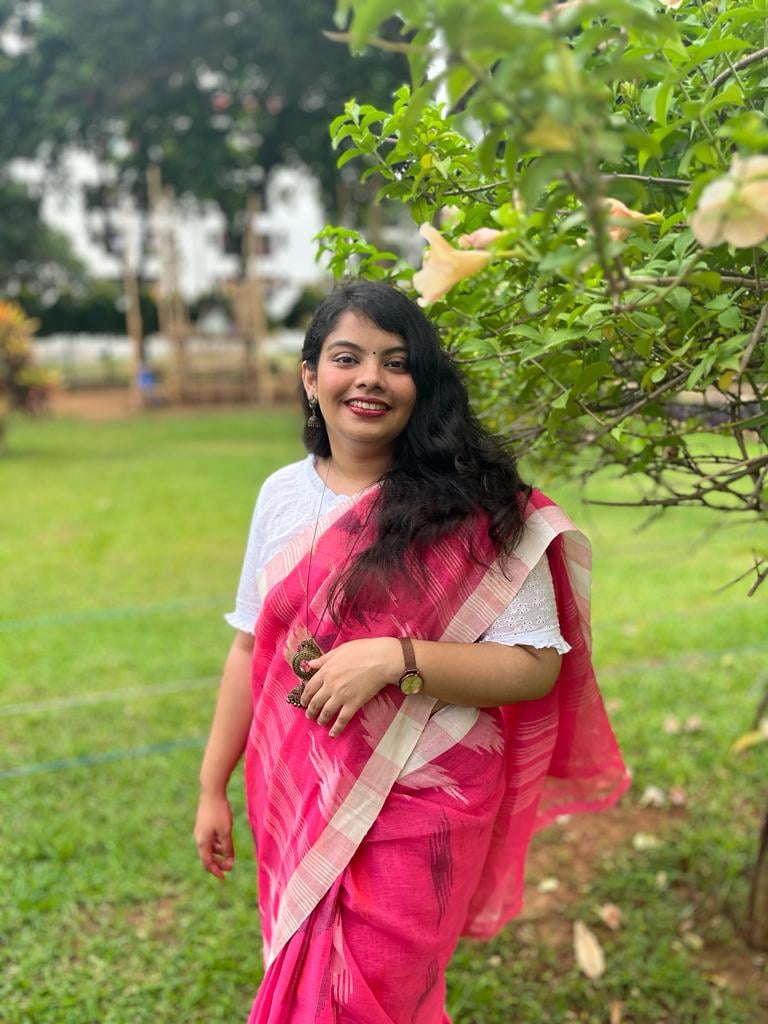
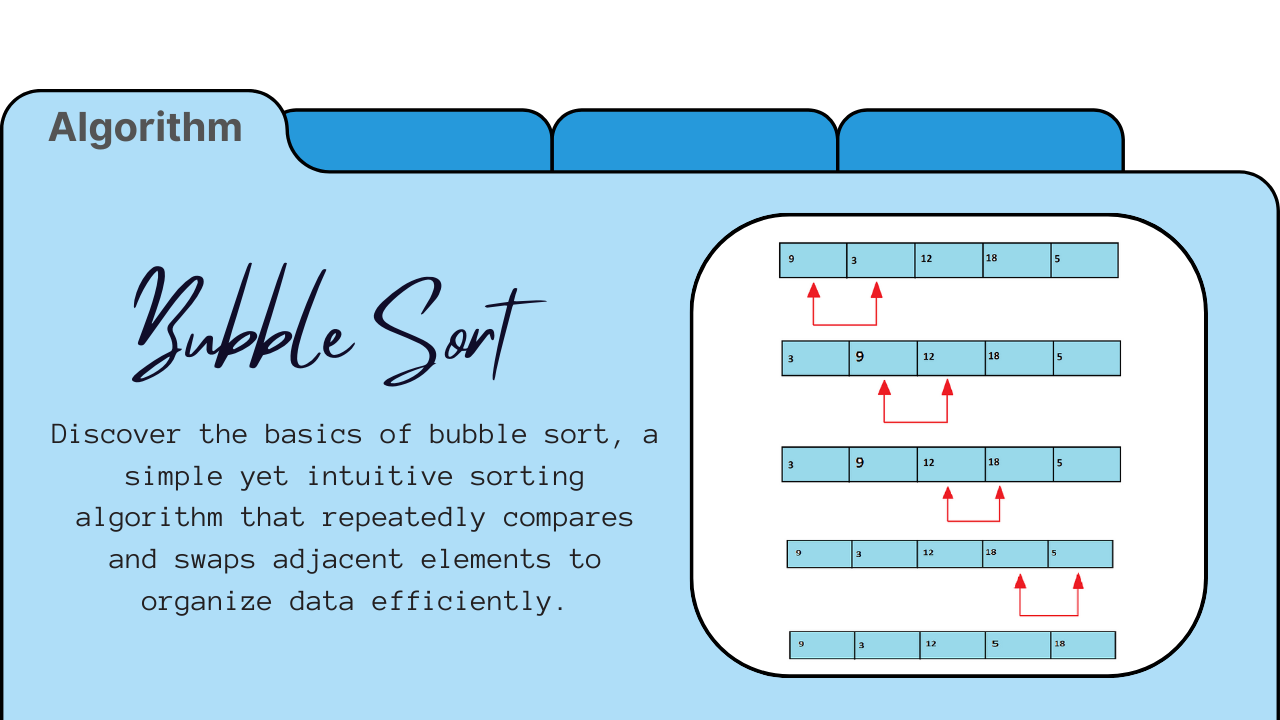
Bubble Sort is one of the simplest and most intuitive sorting algorithms. It methodically compares and swaps adjacent elements until the entire array is sorted.
Steps of Bubble Sort:
Compare Adjacent Elements: Starting from the beginning of the array, compare each pair of adjacent elements. If the elements are in the wrong order, swap them.
Repeat the Process: Continue this process for the entire array. Each pass ensures that the largest unsorted element moves to its correct position at the end of the array.
Reduce the Range: After each pass, reduce the range of the array to be sorted since the largest elements have already been placed in their final positions.
Continue Until Sorted: Repeat the process until no swaps are needed, indicating that the array is sorted.
Pictorial Explanation of the Algorithm:
First Pass:
Compare the first two elements.
Swap if the first element is greater than the second.
Move to the next pair and repeat until the end of the array is reached.
Subsequent Passes:
Each subsequent pass reduces the range of comparison since the largest elements "bubble" to their correct positions.
Continue until no swaps are required in a pass and all the elements in the array are sorted.
Advantages of Bubble Sort
Simplicity: Bubble Sort is easy to understand and implement and it's a great starting point for learning sorting algorithms.
Adaptive: If the array is already sorted or nearly sorted then it can terminate early, making it somewhat adaptive.
Disadvantages of Bubble Sort
Time Complexity: Bubble Sort has a time complexity of O(n^2) in the average and worst cases, which makes it inefficient for large datasets.
Inefficient for Large Arrays: Due to its quadratic time complexity, it is not suitable for large or complex datasets where more efficient algorithms are required.
Code Implementation
Here is a simple code implementation of Bubble Sort in Python:
def bubbleSort(arr):
n = len(arr)
for i in range(n):
# Flag to detect if any swapping happened
swapped = False
# Last i elements are already in place
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
swapped = True
# If no two elements were swapped, break
if not swapped:
break
def printList(arr):
for i in range(len(arr)):
print(arr[i], end=" ")
print()
if __name__ == '__main__':
arr = [64, 34, 25, 12, 22, 11, 90]
bubbleSort(arr)
print("Sorted array is:")
printList(arr)
This code illustrates the process of comparing and swapping adjacent elements until the array is fully sorted.
Summary
Bubble Sort, while simple and easy to grasp but not the most efficient choice for large datasets due to its O(n^2) time complexity. Its main advantage lies in its simplicity and ease of implementation. Despite its inefficiency for large arrays, mastering Bubble Sort provides a solid foundation for understanding more advanced sorting algorithms and their underlying principles.
Subscribe to my newsletter
Read articles from Mehreen Mallick Fiona directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
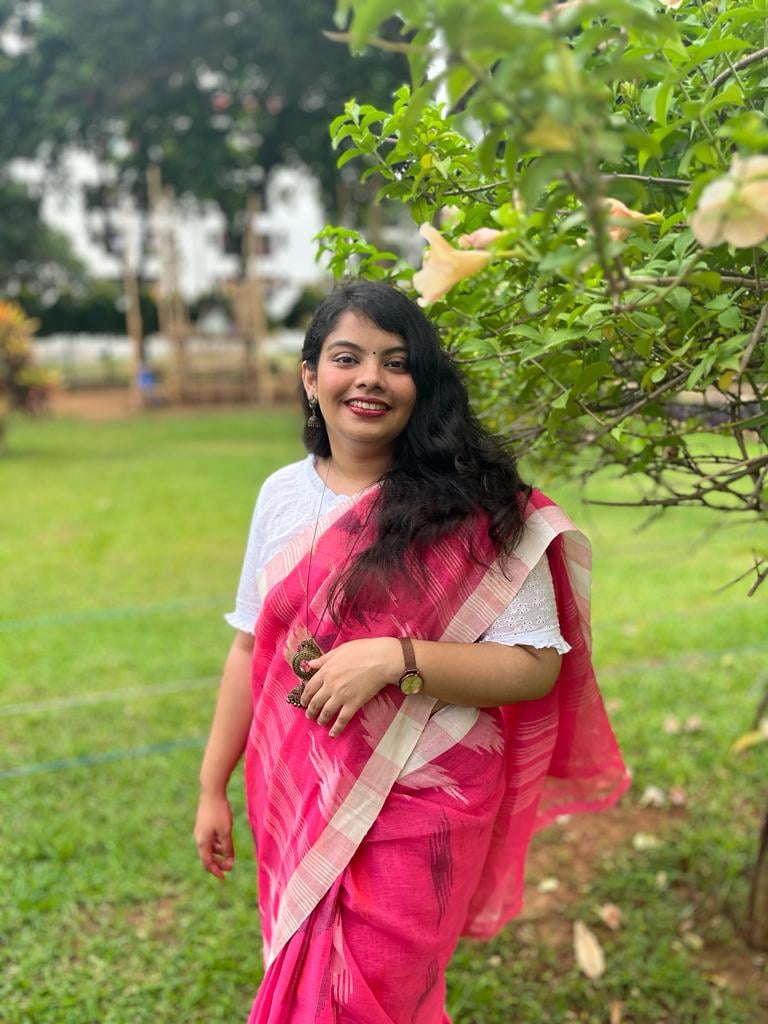
Mehreen Mallick Fiona
Mehreen Mallick Fiona
Hi, I'm Mehreen Mallick Fiona! ๐ I'm a passionate computer science and engineering undergraduate at BRAC University, diving deep into the world of technology and innovation. I'm particularly interested in cloud computing, front-end development, and AI-powered solutions. ๐ฉ๏ธ๐ป When I'm not coding or studying, I'm here to share my journey, learn from this amazing community, and collaborate on exciting projects. Let's connect and build something great together! ๐ Feel free to check out my latest projects and articles, and don't hesitate to reach out. I'm always open to new ideas and collaborations!