Week 2: Diving Deeper into Terraform - Provider Lock Files, Resource Management, and Dependencies
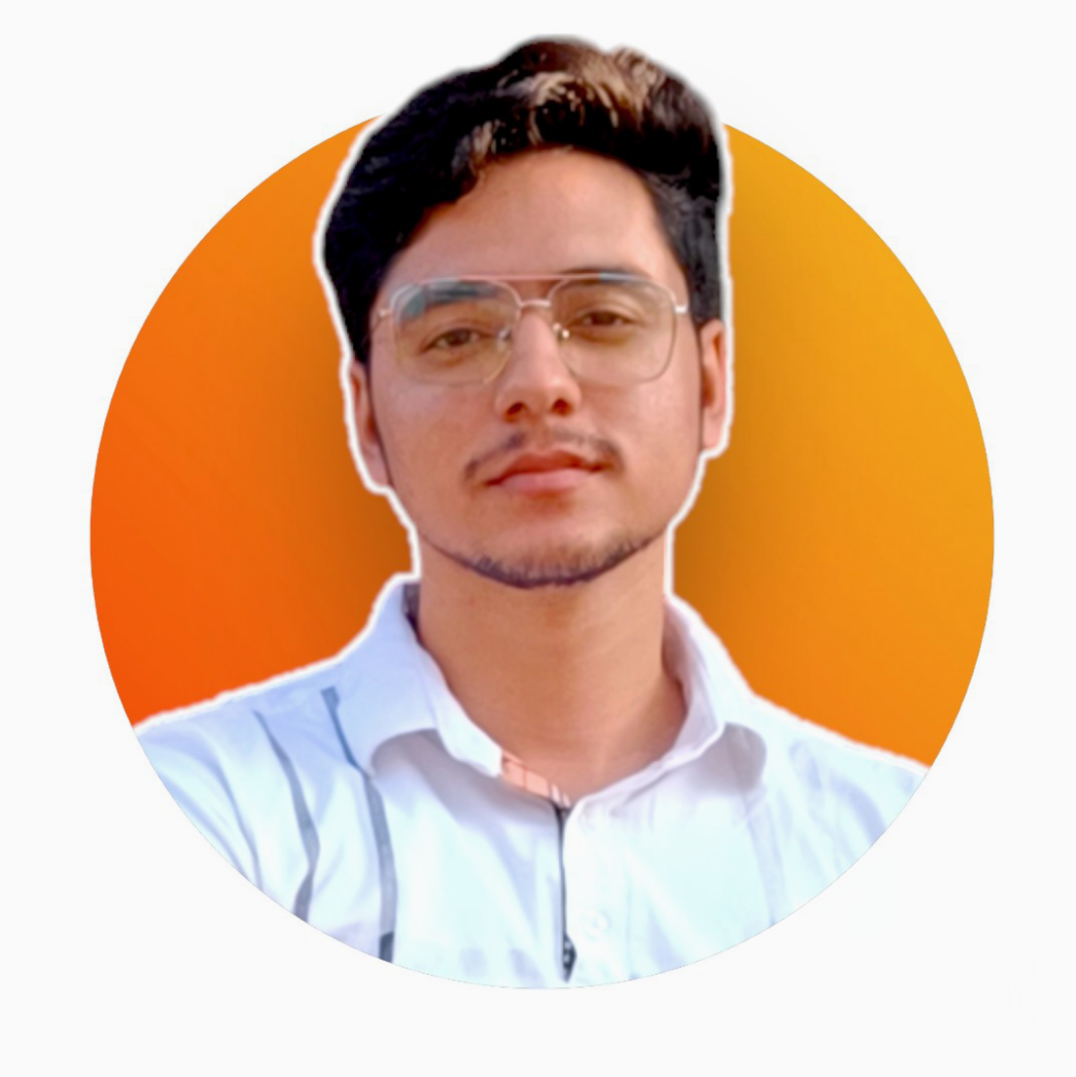
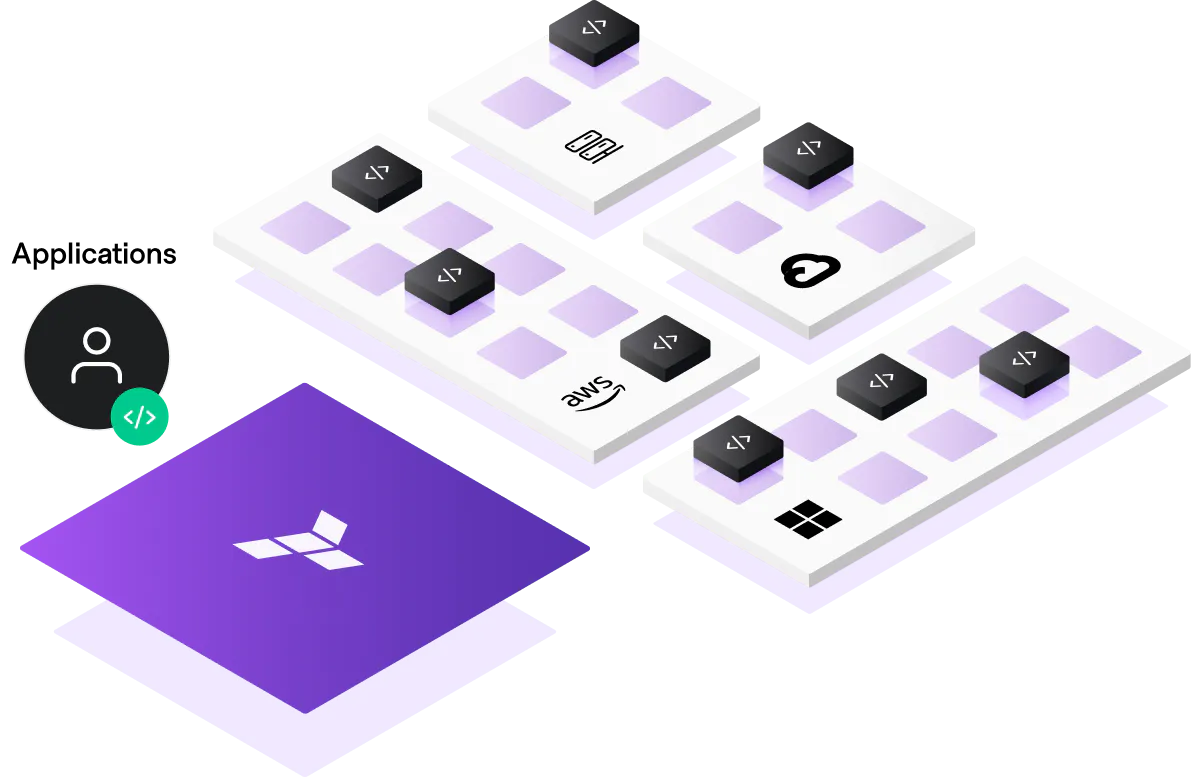
In the second week of my Terraform learning journey, I delved into some crucial aspects that form the foundation of managing infrastructure as code (IaC) with Terraform. This week, I focused on:
Terraform Provider Dependency Lock File
Terraform Resource Syntax, Behavior, and State
Terraform Resource Meta-Argument "depends_on"
Let's explore each of these topics in detail.
1. Terraform Provider Dependency Lock File
Introduction
When working with Terraform, ensuring that your infrastructure code is consistent and repeatable across different environments and teams is essential. The Provider Dependency Lock File, introduced in Terraform v0.14, plays a significant role in this.
The lock file ".terraform.lock.hcl" helps maintain consistent provider versions, ensuring that the exact version specified in your configuration is used during every "terraform init" run. This prevents issues that might arise from accidental upgrades or changes in provider versions.
Step-by-Step Guide
Create or Review "versions.tf":
Start by defining the provider versions in your Terraform configuration. Here’s an example:terraform { required_version = ">= 1.0.0" required_providers { azurerm = { source = "hashicorp/azurerm" version = "1.44.0" } random = { source = "hashicorp/random" version = ">= 3.0" } } }
Create Resources:
Define your resources, such as an Azure Resource Group and a Storage Account:resource "azurerm_resource_group" "myrg" { name = "myrg-1" location = "East US" } resource "azurerm_storage_account" "mysa" { name = "mystorageaccount" resource_group_name = azurerm_resource_group.myrg.name location = azurerm_resource_group.myrg.location account_tier = "Standard" account_replication_type = "GRS" }
Initialize and Apply:
After defining your resources, run the following commands:terraform init terraform apply
The ".terraform.lock.hcl" file will be generated, capturing the exact versions of the providers used.
Upgrade Provider Versions:
To upgrade a provider, modify the version in "versions.tf" and run "terraform init -upgrade". Terraform will update the lock file with the new version:version = ">= 2.0.0"
Key Observations
The lock file captures the provider version, version constraints, and hashes.
Upgrading a provider might lead to breaking changes, so it’s essential to carefully review the changes and update your configuration accordingly.
2. Terraform Resource Syntax, Behavior, and State
Introduction
Terraform's core strength lies in how it manages resources. Understanding resource syntax, behavior, and state management is critical for effective infrastructure management.
Resource Syntax Overview
Terraform resources are defined using a specific syntax:
Resource Block: Defines a resource in Terraform.
Resource Type: Specifies the type of resource (e.g., azurerm_resource_group).
Resource Name: A local identifier for the resource.
Resource Arguments: Properties and configurations for the resource.
Meta-Arguments: Special arguments that control the behavior of the resource, such as "depends_on".
Example of a simple Azure Resource Group:
resource "azurerm_resource_group" "myrg" {
name = "myrg-1"
location = "East US"
}
Understanding Resource Behavior
Resources in Terraform can have different behaviors based on changes in your configuration:
Create: When a resource is first defined, Terraform will create it.
In-Place Update: If you modify a resource's properties, Terraform will update the resource in place.
Destroy and Recreate: Certain changes may require Terraform to destroy and recreate a resource, especially if the change is significant (e.g., renaming a resource).
Terraform State Management
The Terraform state file "terraform.tfstate" is a critical component. It stores the mapping between your configuration and the actual resources in your infrastructure. This file is essential for Terraform to understand the current state of your infrastructure and to plan any necessary changes.
Example of managing Terraform state:
terraform plan
terraform apply
These commands will use the state file to determine what changes need to be made to your infrastructure.
3. Terraform Resource Meta-Argument "depends_on"
Introduction
In complex infrastructure setups, certain resources depend on others. Terraform’s "depends_on" meta-argument is used to explicitly define these dependencies, ensuring that resources are created or destroyed in the correct order.
Example Scenario
Consider a scenario where you need to create a Public IP after a Virtual Network and Subnet are created. You can use "depends_on" to enforce this order:
resource "azurerm_virtual_network" "myvnet" {
name = "myvnet-1"
address_space = ["10.0.0.0/16"]
location = azurerm_resource_group.myrg.location
resource_group_name = azurerm_resource_group.myrg.name
}
resource "azurerm_subnet" "mysubnet" {
name = "mysubnet-1"
resource_group_name = azurerm_resource_group.myrg.name
virtual_network_name = azurerm_virtual_network.myvnet.name
address_prefixes = ["10.0.2.0/24"]
}
resource "azurerm_public_ip" "mypublicip" {
depends_on = [
azurerm_virtual_network.myvnet,
azurerm_subnet.mysubnet
]
name = "mypublicip-1"
resource_group_name = azurerm_resource_group.myrg.name
location = azurerm_resource_group.myrg.location
allocation_method = "Static"
}
Key Points
"depends_on" is necessary when a resource depends on another resource's behavior but doesn’t explicitly reference it.
It helps prevent race conditions where Terraform might try to create or destroy resources in the wrong order.
Conclusion
Week 2 of my Terraform journey has been a deep dive into managing provider versions, understanding resource behavior, and ensuring correct resource dependencies. These concepts are fundamental as I continue to build more complex infrastructure with Terraform. I'll be sharing more insights and configurations on my GitHub repository. Feel free to check it out and follow along!
Check out my Terraform GitHub repository
Stay tuned for my Week 3 update, where I'll explore more advanced Terraform features and practices!
Subscribe to my newsletter
Read articles from Ketan Dogra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
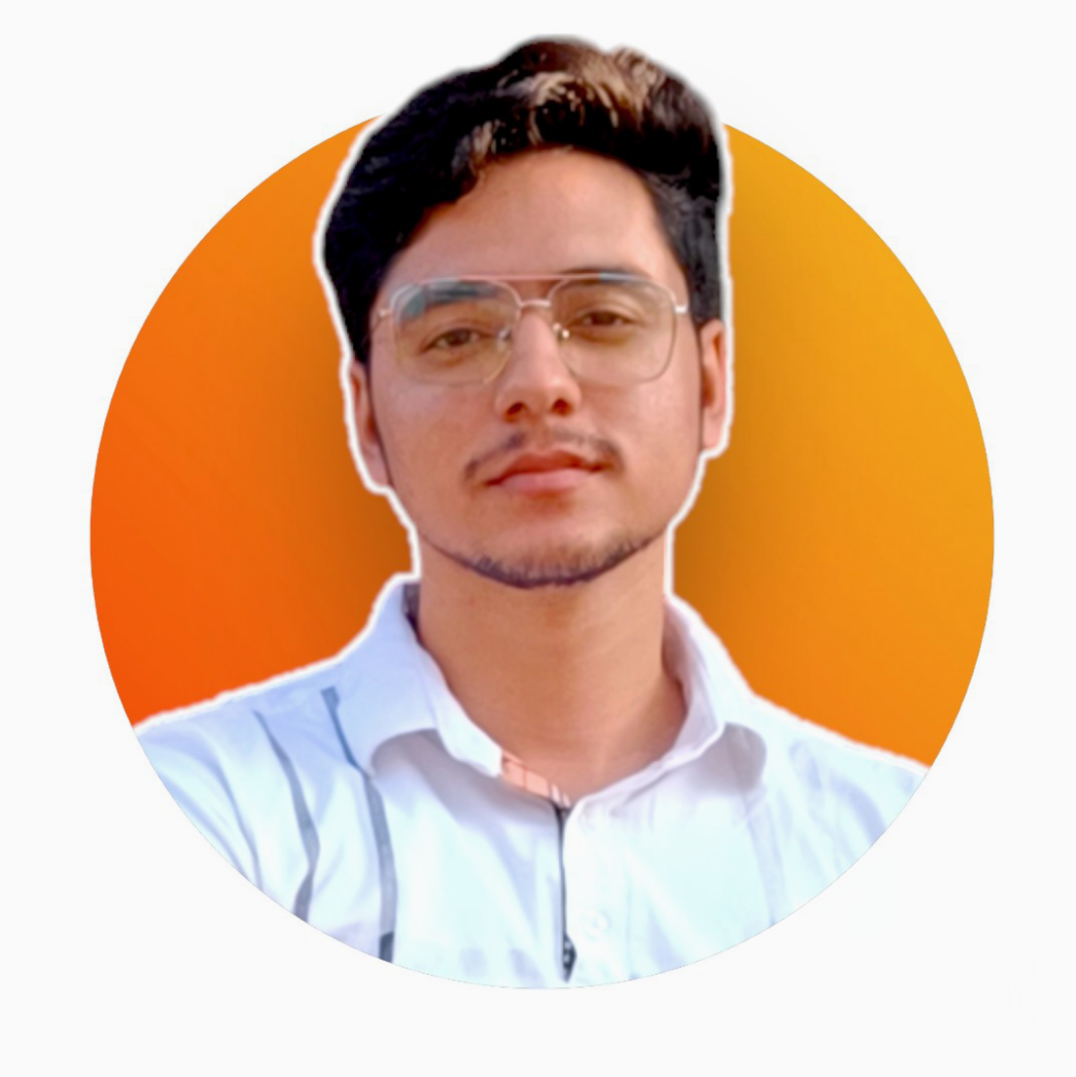
Ketan Dogra
Ketan Dogra
Full Stack Developer