Why Use Cloudflare Workers? Building Serverless Apps with Hono.js Explained
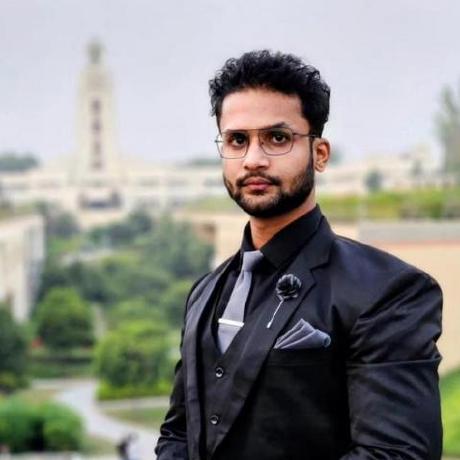
Table of contents
- Introduction
- What are Serverless Functions?
- What is Cloudflare Workers?
- When to Use Cloudflare Workers?
- Introducing Hono.js
- Deploying and Maintaining Cloudflare Workers with Hono.js
- More Advanced Example: Building a URL Shortener with Cloudflare Workers and Hono.js
- Pros and Cons of Using Cloudflare Workers
- Conclusion
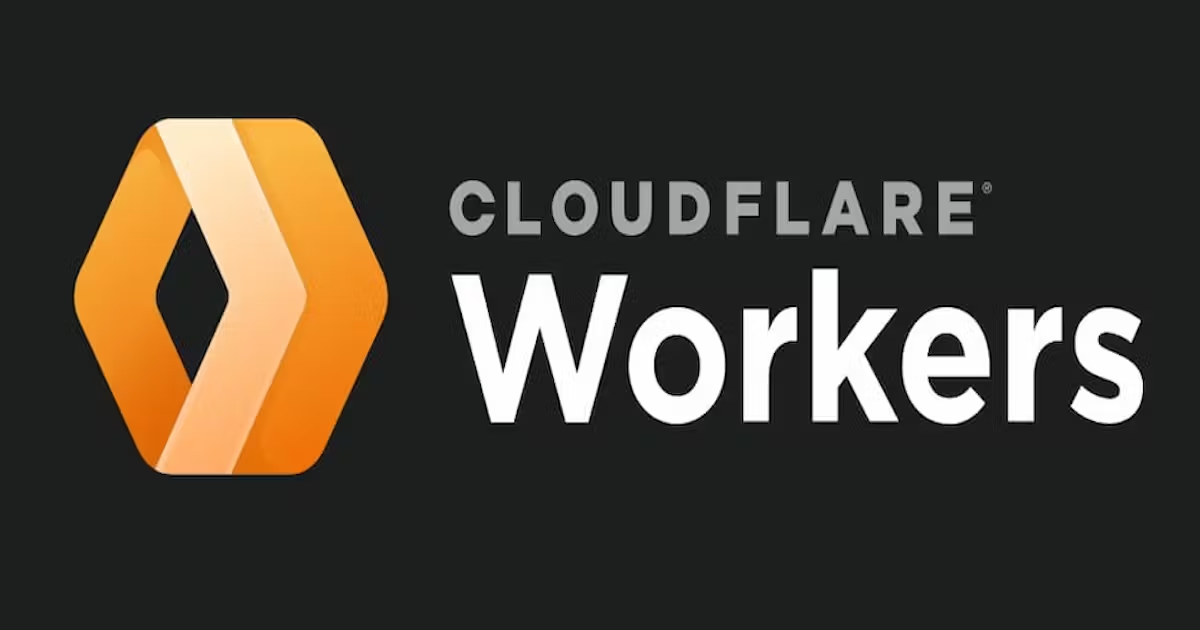
Introduction
The evolution of cloud computing has introduced us to the concept of serverless functions, a paradigm shift that allows developers to run code without managing servers. This approach brings scalability, flexibility, and cost-efficiency, making it increasingly popular among developers and enterprises. In this article, we will explore what serverless functions are, dive into Cloudflare Workers, understand when and why you should use them, and introduce Hono.js for building serverless applications. We’ll also cover the pros and cons of using these technologies and provide some practical code examples.
What are Serverless Functions?
Serverless functions, often referred to as Function-as-a-Service (FaaS), are cloud computing services that enable developers to execute code in response to events without the complexity of managing servers. In a serverless model:
No Server Management: Developers don't need to provision, scale, or maintain servers.
Automatic Scaling: Serverless platforms automatically scale the application up or down based on demand.
Pay-Per-Use Pricing: You pay only for the compute resources you use, typically billed per execution time and memory usage.
Serverless functions are ideal for tasks like HTTP request handling, file processing, data transformation, and event-driven workflows. They allow developers to focus on writing code, leaving infrastructure concerns to the cloud provider.
What is Cloudflare Workers?
Cloudflare Workers is a serverless platform that allows developers to run JavaScript, Rust, C, and C++ code at the edge, closer to the user. Unlike traditional serverless platforms that run in a centralized data center, Cloudflare Workers operate on Cloudflare's global network of data centers, ensuring low latency and high performance.
Key Features of Cloudflare Workers:
Edge Execution: Runs code close to the user, reducing latency and improving response times.
Scalability: Automatically scales to handle any volume of requests.
Security: Built on the same network that powers Cloudflare's security services, providing robust protection.
Integrated Caching: Leverage Cloudflare's caching capabilities to optimize performance.
Flexible Deployment: Easily deploy code globally with a few commands.
When to Use Cloudflare Workers?
Cloudflare Workers are suitable for scenarios where performance, scalability, and reduced latency are critical. Some common use cases include:
API Gateways: Quickly process and route API requests at the edge.
Content Manipulation: Modify HTML, JSON, or other content types on-the-fly for personalization.
Authentication and Authorization: Handle authentication checks and token validation without hitting the origin server.
Rate Limiting: Implement rate limiting and request filtering at the edge to protect backend services.
Static Site Generation: Serve pre-rendered content directly from the edge for faster load times.
Introducing Hono.js
Hono.js is a small, fast, and intuitive web framework specifically designed for building serverless applications on Cloudflare Workers. It provides a familiar Express-like API, making it easy for developers to get started with building robust serverless applications.
Key Features of Hono.js:
Minimalist: Lightweight and fast, perfect for edge computing.
Express-Like API: Familiar syntax for those who have experience with Express.js.
Middleware Support: Extensible with middleware to add functionalities like authentication, logging, and more.
TypeScript Support: Built with TypeScript in mind, providing type safety and modern JavaScript features.
Deploying and Maintaining Cloudflare Workers with Hono.js
Let’s go through the steps to deploy a simple serverless function using Cloudflare Workers and Hono.js.
Step 1: Setting Up Your Environment
Install Wrangler: Cloudflare’s command-line tool for managing Workers.
npm install -g wrangler
Login to Cloudflare Account:
wrangler login
Create a New Project:
wrangler init my-worker cd my-worker
Step 2: Install Hono.js
In your project directory, install Hono.js:
npm install hono
Step 3: Create a Simple Worker with Hono.js
Create an index.ts
file and add the following code:
import { Hono } from 'hono';
const app = new Hono();
app.get('/', (c) => c.text('Hello, Cloudflare Workers with Hono!'));
app.get('/hello/:name', (c) => {
const name = c.req.param('name');
return c.text(`Hello, ${name}!`);
});
app.get('/json', (c) => c.json({ message: 'Hello, JSON response!' }));
export default app;
Explanation:
This code sets up a basic Hono application with three routes:
/
responds with a simple text message./hello/:name
responds with a personalized greeting based on the route parameter./json
responds with a JSON object, showcasing how to send structured data.
Step 4: Configure and Deploy
Configure Wrangler: Open
wrangler.toml
and add your account details.name = "my-worker" type = "javascript" account_id = "your_account_id" zone_id = "your_zone_id"
Build and Deploy:
npm run build wrangler publish
Your serverless function is now live and running on Cloudflare’s global edge network!
More Advanced Example: Building a URL Shortener with Cloudflare Workers and Hono.js
Here’s a more advanced example of how you might use Cloudflare Workers and Hono.js to build a basic URL shortener.
Backend: Cloudflare Worker
Install Dependencies:
npm install hono uuid
Code Implementation:
import { Hono } from 'hono'; import { v4 as uuidv4 } from 'uuid'; const app = new Hono(); const urlDatabase = new Map<string, string>(); // Endpoint to shorten a URL app.post('/shorten', async (c) => { const { url } = await c.req.json(); const id = uuidv4().slice(0, 6); urlDatabase.set(id, url); return c.json({ shortUrl: `https://yourdomain.com/${id}` }); }); // Endpoint to redirect to the original URL app.get('/:id', (c) => { const id = c.req.param('id'); const url = urlDatabase.get(id); if (url) { return c.redirect(url); } return c.text('URL not found', 404); }); export default app;
Explanation:
This code defines two main routes:
/shorten
accepts a POST request with a JSON body containing a URL. It generates a short ID and returns a shortened URL./:id
takes the short ID and redirects to the original URL stored in theurlDatabase
.
Pros and Cons of Using Cloudflare Workers
Pros:
Low Latency: Executes code close to the user, reducing latency significantly.
Scalable: Automatically scales to handle high traffic without manual intervention.
Cost-Effective: Pay only for the resources you use, making it economical for varying workloads.
Security: Leverages Cloudflare’s robust security features to protect your applications.
Ease of Use: Simplifies deployment and management with tools like Wrangler and frameworks like Hono.js.
Cons:
Cold Starts: Although rare, cold starts can add latency for the first request.
Limited Execution Time: Designed for short-lived functions; long-running processes may not be suitable.
Debugging Complexity: Debugging can be more challenging due to distributed nature.
Vendor Lock-In: Tightly integrated with Cloudflare, making it harder to migrate to other platforms.
Conclusion
Serverless functions and Cloudflare Workers offer a powerful combination for building scalable, performant, and cost-effective applications. With the introduction of Hono.js, building serverless applications has become even more accessible, providing developers with the tools they need to create robust and efficient edge-based solutions. Whether you're optimizing for low latency, improving scalability, or simplifying deployment, serverless and edge computing with Cloudflare Workers is a compelling choice.
By understanding the strengths and limitations of these technologies, you can make informed decisions on how to leverage them effectively in your projects. Happy coding! 🎉
Subscribe to my newsletter
Read articles from Swami Buddha Chaitanya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
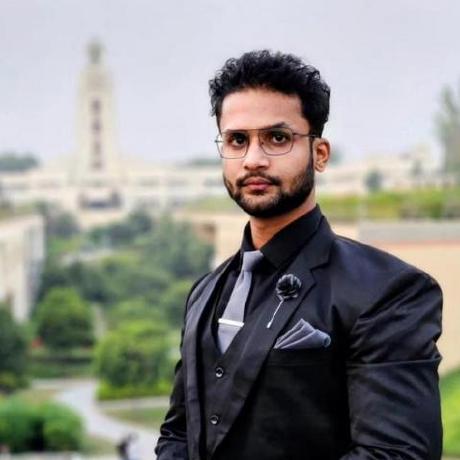