Building a Student Management System with Python and Streamlit: A Step-by-Step Guide
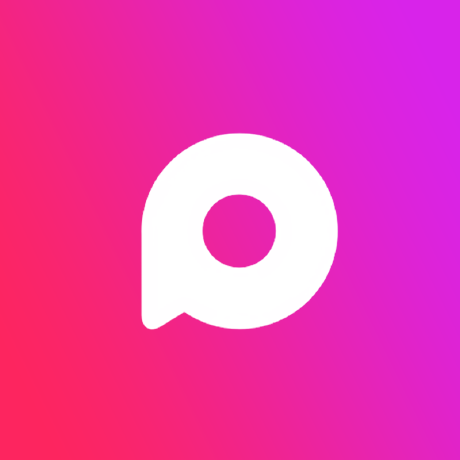
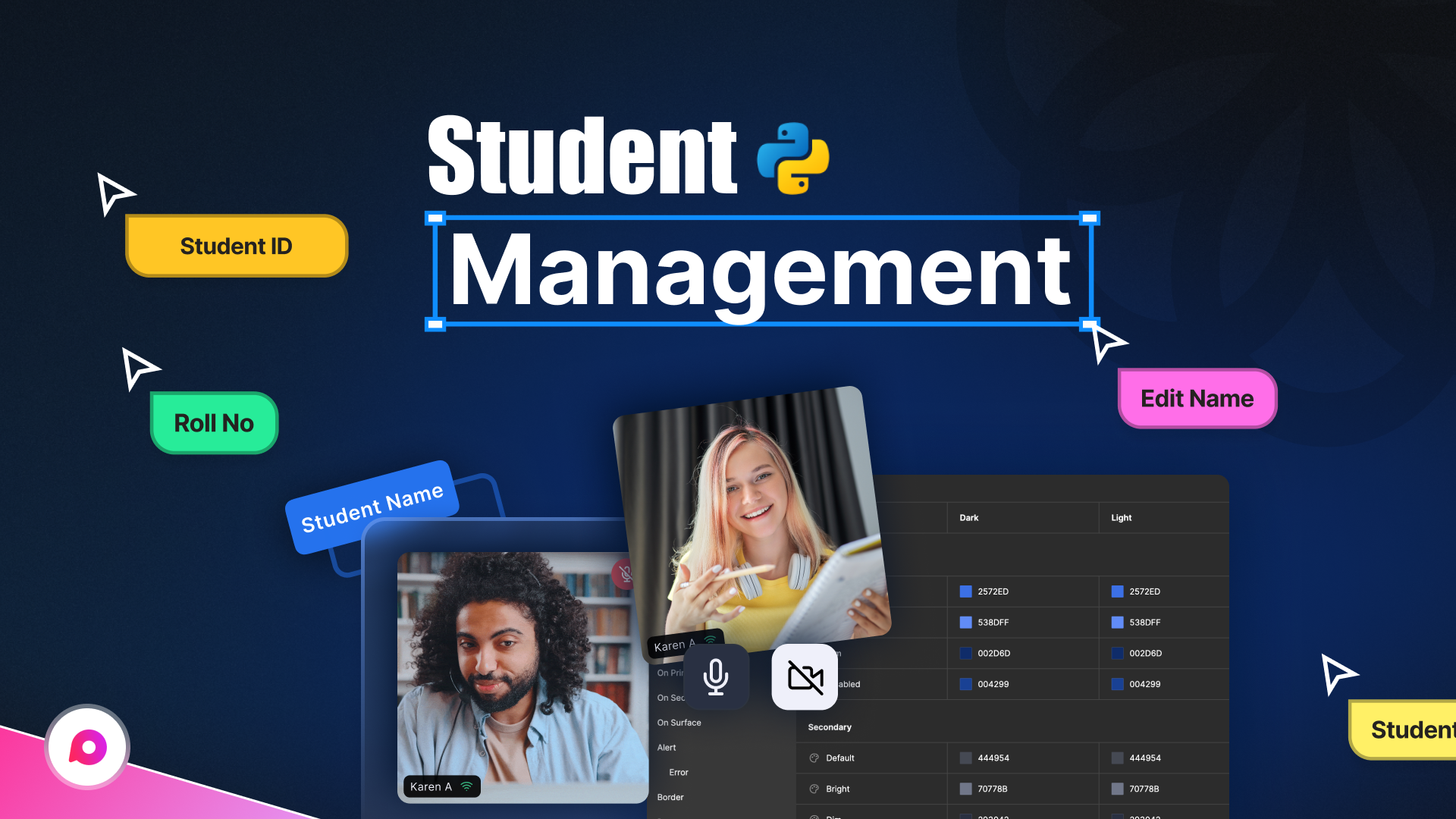
Introduction
Hey there, Python enthusiasts! 🎉 Today, we're going to dive into something really cool—building a Student Management System using Python and Streamlit. Whether you're a student, educator, or just someone looking to improve your Python skills, this project is a perfect way to get hands-on experience with web app development using Streamlit.
In this tutorial, I'll walk you through the entire process of setting up, coding, and deploying a fully functional student management system. By the end, you'll have a sleek web app where you can add, view, update, delete, and even export student records!
Why Streamlit?
Before we jump into the code, let me take a moment to talk about why I chose Streamlit for this project. Streamlit is a fantastic Python library that makes it incredibly easy to create web apps without any frontend experience. With just a few lines of code, you can have an interactive, responsive UI up and running. It's perfect for data scientists, machine learning engineers, and Python developers who want to create web apps without the hassle of learning HTML, CSS, and JavaScript.
Setting Up Your Project
Let's get started with setting up the environment. First, you'll need to install Streamlit if you haven't already. Open your terminal and run:
pip install streamlit
Next, create a new folder for your project called student_management
. Inside this folder, we’ll create two Python files:
app.py
- This will handle the user interface using Streamlit.db.py
- This will manage all our database operations using SQLite.
Step 1: Creating the Database Layer
We'll start by creating the database layer in db.py
. We’re using SQLite, a lightweight, file-based database that's perfect for small projects like this one.
Setting Up the Database
First, let's import the SQLite library and create a connection function:
import sqlite3
def create_connection():
conn = sqlite3.connect('students.db')
return conn
This function connects to a SQLite database file named students.db
. If the file doesn’t exist, SQLite will create it for us.
Creating the Students Table
Next, we'll create a function to set up the students table:
def create_table():
conn = create_connection()
cursor = conn.cursor()
cursor.execute('''
CREATE TABLE IF NOT EXISTS students (
id INTEGER PRIMARY KEY AUTOINCREMENT,
name TEXT,
age INTEGER,
gender TEXT,
course TEXT
)
''')
conn.commit()
conn.close()
This SQL command creates a table named students
with columns for the student's ID, name, age, gender, and course. The ID is an auto-incremented primary key.
CRUD Operations
We also need to create the basic CRUD (Create, Read, Update, Delete) operations:
- Add a Student:
def add_student(name, age, gender, course):
conn = create_connection()
cursor = conn.cursor()
cursor.execute('''
INSERT INTO students (name, age, gender, course) VALUES (?, ?, ?, ?)
''', (name, age, gender, course))
conn.commit()
conn.close()
- Get All Students:
def get_all_students():
conn = create_connection()
cursor = conn.cursor()
cursor.execute('SELECT * FROM students')
students = cursor.fetchall()
conn.close()
return students
- Update a Student:
def update_student(student_id, name, age, gender, course):
conn = create_connection()
cursor = conn.cursor()
cursor.execute('''
UPDATE students
SET name = ?, age = ?, gender = ?, course = ?
WHERE id = ?
''', (name, age, gender, course, student_id))
conn.commit()
conn.close()
- Delete a Student:
def delete_student(student_id):
conn = create_connection()
cursor = conn.cursor()
cursor.execute('DELETE FROM students WHERE id = ?', (student_id,))
conn.commit()
conn.close()
With these functions, we can perform all the basic database operations required for our student management system.
Step 2: Building the User Interface with Streamlit
Now that our database layer is ready, let's move on to creating the user interface in app.py
.
Setting Up Streamlit
Start by importing Streamlit and our database functions:
import streamlit as st
from db import create_table, add_student, get_all_students, update_student, delete_student
Let's initialize the database by calling create_table()
:
# Initialize Database
create_table()
Creating the App Layout
We'll create a sidebar menu that lets users choose between adding, viewing, editing, and deleting students:
st.title("Student Management System")
# Menu options
menu = ["Add Student", "View Students", "Edit Student", "Delete Student"]
choice = st.sidebar.selectbox("Menu", menu)
Adding Students
When the user selects "Add Student", we’ll display a form for entering student details:
if choice == "Add Student":
st.subheader("Add a New Student")
name = st.text_input("Name")
age = st.number_input("Age", min_value=1, max_value=100, step=1)
gender = st.selectbox("Gender", ["Male", "Female", "Other"])
course = st.text_input("Course")
if st.button("Add Student"):
if not name or not course:
st.error("Please fill all the fields.")
else:
add_student(name, age, gender, course)
st.success(f"Added {name} to the student database.")
Viewing Students
For the "View Students" option, we’ll display all students or search for specific ones:
elif choice == "View Students":
st.subheader("View All Students")
search_query = st.text_input("Search by Name or Course")
if st.button("Search"):
students = search_students(search_query)
if students:
for student in students:
st.text(f"ID: {student[0]} | Name: {student[1]} | Age: {student[2]} | Gender: {student[3]} | Course: {student[4]}")
else:
st.error("No students found.")
else:
students = get_all_students()
for student in students:
st.text(f"ID: {student[0]} | Name: {student[1]} | Age: {student[2]} | Gender: {student[3]} | Course: {student[4]}")
Editing and Deleting Students
Finally, we’ll add functionality for editing and deleting student records:
elif choice == "Edit Student":
st.subheader("Edit Student Details")
student_id = st.number_input("Enter Student ID", min_value=1)
if st.button("Fetch Details"):
student = get_student_by_id(student_id)
if student:
name = st.text_input("Name", value=student[1])
age = st.number_input("Age", min_value=1, max_value=100, step=1, value=student[2])
gender = st.selectbox("Gender", ["Male", "Female", "Other"], index=["Male", "Female", "Other"].index(student[3]))
course = st.text_input("Course", value=student[4])
if st.button("Update Student"):
update_student(student_id, name, age, gender, course)
st.success(f"Updated {name}'s details.")
else:
st.error("Student not found.")
elif choice == "Delete Student":
st.subheader("Delete Student")
student_id = st.number_input("Enter Student ID", min_value=1)
if st.button("Delete"):
delete_student(student_id)
st.success(f"Deleted student with ID {student_id}.")
Step 3: Adding Extra Features
Search Functionality
Let’s add search functionality to make it easier to find students:
def search_students(query):
conn = create_connection()
cursor = conn.cursor()
cursor.execute("SELECT * FROM students WHERE name LIKE ? OR course LIKE ?", ('%'+query+'%', '%'+query+'%'))
students = cursor.fetchall()
conn.close()
return students
Export to CSV
We can also add an option to export student data to a CSV file:
import csv
def export_students_to_csv():
students = get_all_students()
with open('students_export.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['ID', 'Name', 'Age', 'Gender', 'Course'])
writer.writerows(students)
if st.sidebar.button("Export as CSV"):
export_students_to_csv()
st.success("Data exported successfully.")
Running the App
Once you’ve added all the features, run the app using:
streamlit run app.py
Your student management system is now live! You can add, view, edit, delete, search, and even export student data with just a few clicks.
Conclusion
And there you have it—a fully functional student management system built using Python and Streamlit. This project not only helps you understand the basics of
CRUD operations but also introduces you to web app development with Streamlit. Feel free to modify and expand this project to fit your needs.
If you found this tutorial helpful, don’t forget to share it with others! I’d love to hear your thoughts, so leave a comment below or reach out to me on YouTube.
Happy coding! 😊
Subscribe to my newsletter
Read articles from Proxlight directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
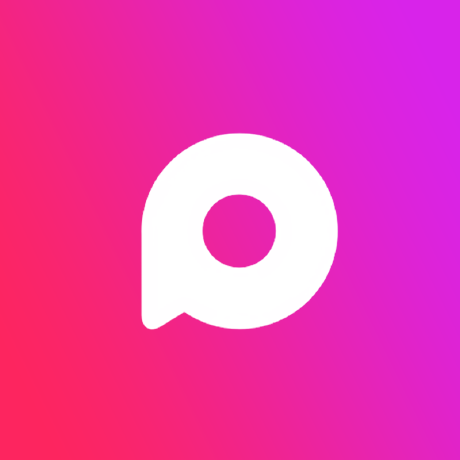
Proxlight
Proxlight
Do you need help with programming? You are not the only one. In this channel, we tackle gradual programming topics and end the videos on cool projects you can work on for practice to improve your skills on that given topic, step by step. Join us as we use fun and practical projects to grow our programming skills.😊💻.