How to Create PDF Reports Using Python
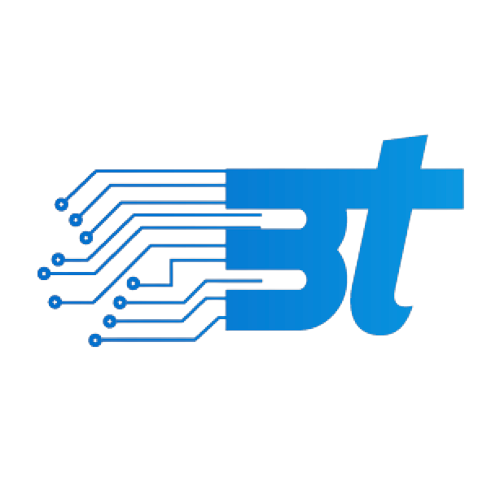

Generating PDF reports is a common requirement for many applications, whether it's for business reports, invoices, summaries, or documentation. Python offers several libraries that make it easy to create, modify, and manipulate PDF files programmatically. In this blog, we'll explore how to generate a PDF report using Python's ReportLab
library, a powerful tool for creating PDFs with custom formatting and graphics.
Why Generate PDF Reports?
PDF (Portable Document Format) is a universal format that preserves the layout and content of documents across different platforms and devices. PDFs are widely used in business and education for:
Professional Reports: Share formatted, printable documents.
Invoices: Generate bills or invoices dynamically from a database.
Data Summaries: Present summarized data in an easy-to-read format.
Documentation: Create manuals, guides, and reports.
Getting Started with PDF Generation in Python
To generate PDFs in Python, we'll use the ReportLab
library, which provides tools for creating PDF documents from scratch, including text, images, graphics, and tables.
Step 1: Install the ReportLab Library
First, you need to install the ReportLab
library if you haven't already. You can install it using pip:
pip install reportlab
Step 2: Basic PDF Creation
Here’s a simple example of creating a PDF report with some basic text:
from reportlab.lib.pagesizes import letter
from reportlab.pdfgen import canvas
def create_pdf(filename):
# Set up the canvas with a specific page size
c = canvas.Canvas(filename, pagesize=letter)
# Draw a string on the PDF at coordinates (x, y)
c.drawString(100, 750, "Hello, welcome to your PDF report!")
c.drawString(100, 730, "This report was generated using Python and ReportLab.")
# Save the canvas to create the PDF file
c.save()
if __name__ == "__main__":
create_pdf("simple_report.pdf")
In this code:
We use
canvas.Canvas
to create a canvas object for our PDF.drawString(x, y, text)
places text at the specified(x, y)
coordinates on the page.c.save()
saves the canvas and generates the PDF file.
Run this script to create a basic PDF named simple_report.pdf
.
Step 3: Adding More Content to Your PDF
Let’s enhance the PDF by adding a title, images, tables, and other formatted content.
from reportlab.lib.pagesizes import letter
from reportlab.pdfgen import canvas
from reportlab.lib import colors
from reportlab.platypus import Table, TableStyle
def create_pdf(filename):
# Create a canvas
c = canvas.Canvas(filename, pagesize=letter)
# Add a title
c.setFont("Helvetica-Bold", 18)
c.drawString(200, 800, "Monthly Sales Report")
# Add a subtitle
c.setFont("Helvetica", 12)
c.drawString(200, 780, "Report generated using Python and ReportLab")
# Add an image
c.drawImage("path_to_image.png", 100, 600, width=200, height=100)
# Create a sample data table
data = [
["Product", "Units Sold", "Revenue"],
["Product A", "100", "$1,000"],
["Product B", "150", "$1,500"],
["Product C", "200", "$2,000"]
]
# Define the table and its style
table = Table(data)
style = TableStyle([
('BACKGROUND', (0, 0), (-1, 0), colors.grey),
('TEXTCOLOR', (0, 0), (-1, 0), colors.whitesmoke),
('ALIGN', (0, 0), (-1, -1), 'CENTER'),
('FONTNAME', (0, 0), (-1, 0), 'Helvetica-Bold'),
('BOTTOMPADDING', (0, 0), (-1, 0), 12),
('BACKGROUND', (0, 1), (-1, -1), colors.beige),
('GRID', (0, 0), (-1, -1), 1, colors.black)
])
table.setStyle(style)
# Wrap the table and draw it on the canvas
table.wrapOn(c, 400, 400)
table.drawOn(c, 100, 500)
# Save the PDF
c.save()
if __name__ == "__main__":
create_pdf("detailed_report.pdf")
In this example:
Title and Subtitle: We set the font and size using
c.setFont()
and position the text withc.drawString()
.Adding Images: Use
c.drawImage()
to place an image on the PDF. Make sure to provide the correct path and specify the size.Creating Tables: We use the
Table
andTableStyle
classes fromreportlab.platypus
to create and style a data table. The table is drawn on the canvas withtable.drawOn()
.Styling: Table styles are defined to enhance the appearance, such as background colors, text color, alignment, padding, and grid lines.
Step 4: Advanced PDF Features
ReportLab offers many advanced features to customize your PDFs further:
Page Layout and Multi-Page PDFs: Control the layout of each page and create multi-page documents.
Graphics and Charts: Draw shapes, lines, and even create dynamic charts.
Interactive Elements: Add hyperlinks, bookmarks, and form elements to your PDFs.
Custom Fonts and Styles: Use custom fonts and styles to match your brand.
For more detailed documentation and examples, you can explore the ReportLab User Guide.
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
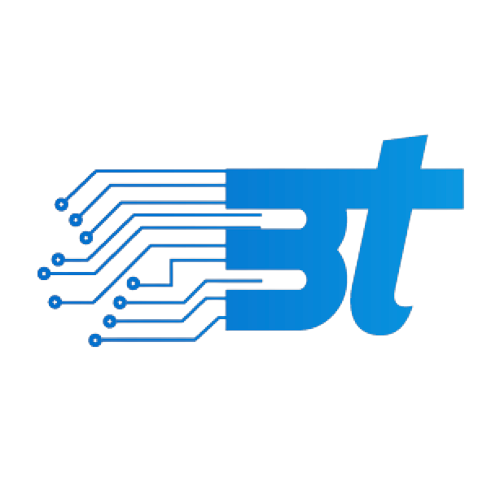
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.