Day 72: Mastering Pointers in C++ ๐ ๐
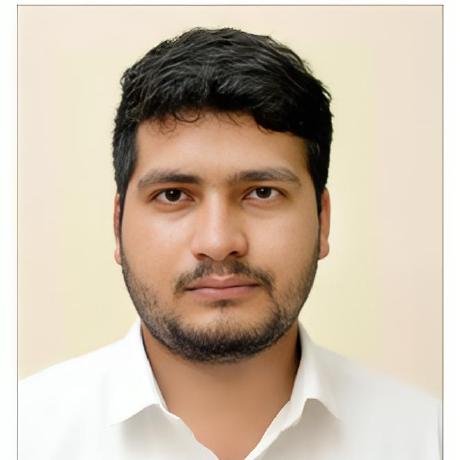
Table of contents
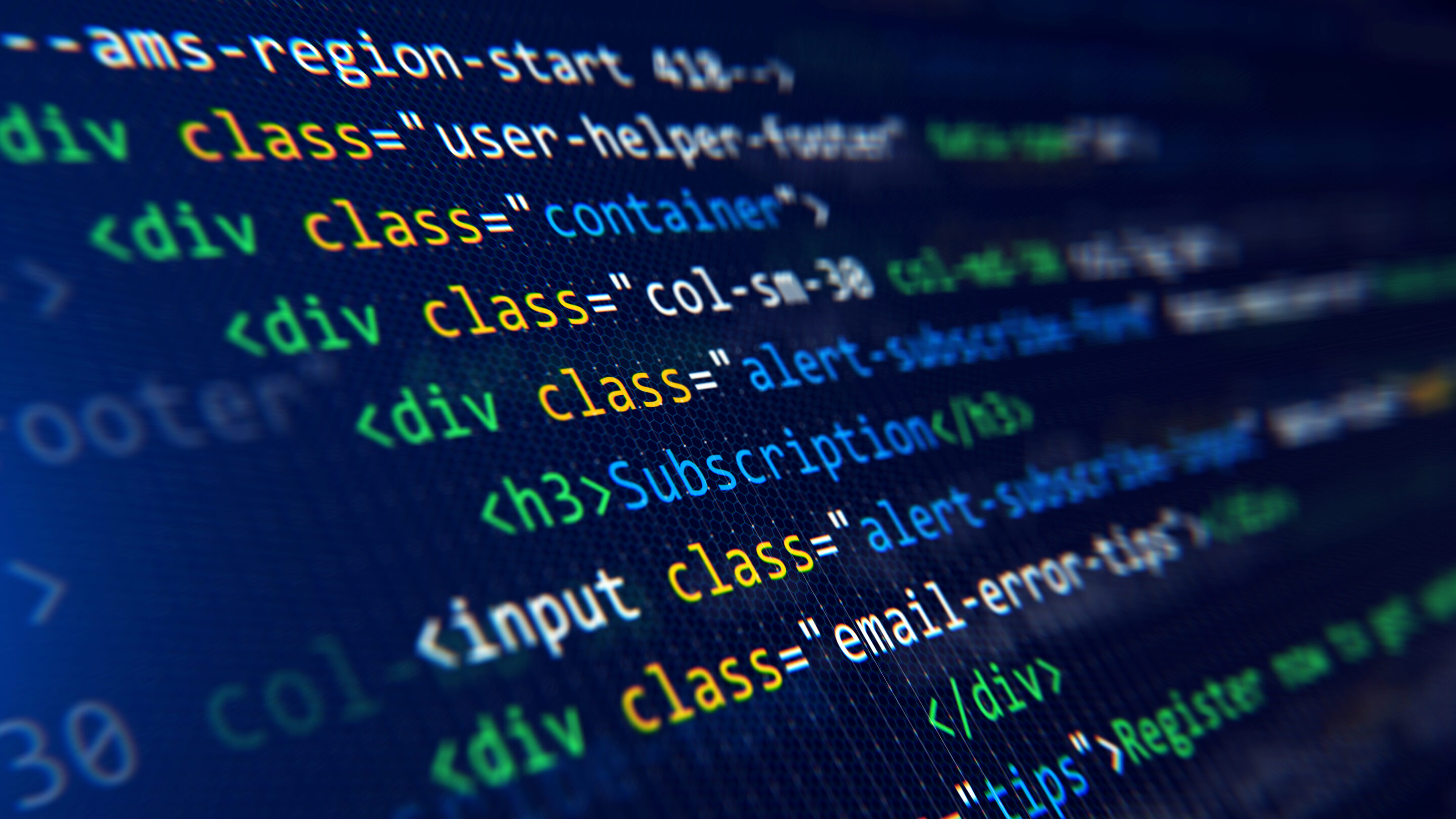
What are Pointers?
Pointers are variables that store the memory address of another variable. In C++, pointers are a powerful tool that enables dynamic memory management, efficient array manipulation, and the creation of complex data structures like linked lists, trees, and graphs.
Pointer Syntax:
int *ptr; declares a pointer ptr that can hold the address of an integer variable.
The * symbol is used both to declare a pointer and to dereference a pointer.
Pointer Example:
Dereferencing a Pointer: Dereferencing a pointer means accessing the value stored at the memory address the pointer holds.
Pointer Arithmetic: Pointers can be incremented or decremented to traverse through an array.
Dynamic Memory Allocation: Pointers are crucial for dynamic memory allocation using new and delete operators.
Pointer to Pointer: A pointer can point to another pointer, allowing multiple levels of indirection.
Pointers and Arrays: In C++, the name of an array is essentially a pointer to the first element of the array.
Pointers and Functions: Pointers can be passed to functions to modify the actual arguments or to pass large structures efficiently.
Null Pointer: A null pointer is a pointer that does not point to any memory location.
Dangling Pointer: A pointer that does not point to a valid object is known as a dangling pointer, which can occur when an object is deleted or goes out of scope.
Common Pointer Errors:
Dereferencing a Null Pointer: Leads to undefined behavior.
Memory Leaks: Occurs when dynamically allocated memory is not freed using delete.
Double Deletion: Deleting the same memory twice can cause a program to crash.
Best Practices:
Always initialize pointers.
Use nullptr instead of NULL for null pointers in modern C++.
Avoid raw pointers when possible; use smart pointers like std::unique_ptr or std::shared_ptr.
Pointers are foundational in C++ and mastering them is key to understanding the language's deeper concepts, such as dynamic memory allocation, data structures, and efficient memory management.
Subscribe to my newsletter
Read articles from Prashant Joshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
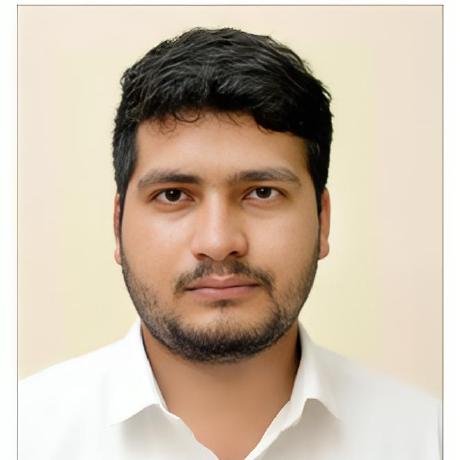
Prashant Joshi
Prashant Joshi
Fuelled by boundless passion for DevOps and cloud technologies โ๏ธโ๏ธโ๏ธ โ๏ธ, I'm on an exhilarating journey of mastery of Computer Application (MCA). I'm not just learning; I'm devouring DevOps principles and cloud tech, crafting the future through relentless innovation and automation. ๐