Node.js Unveiled #5: Building Scalable APIs
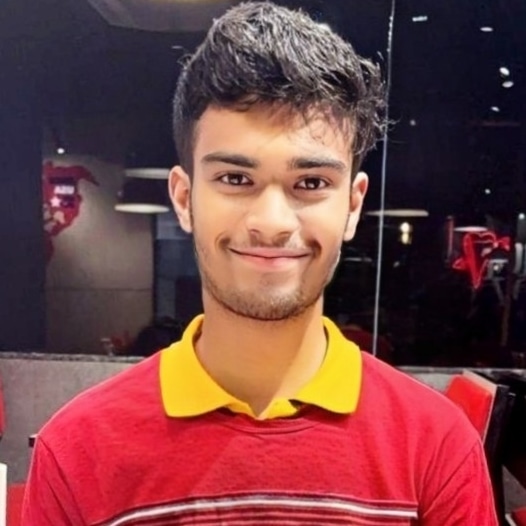
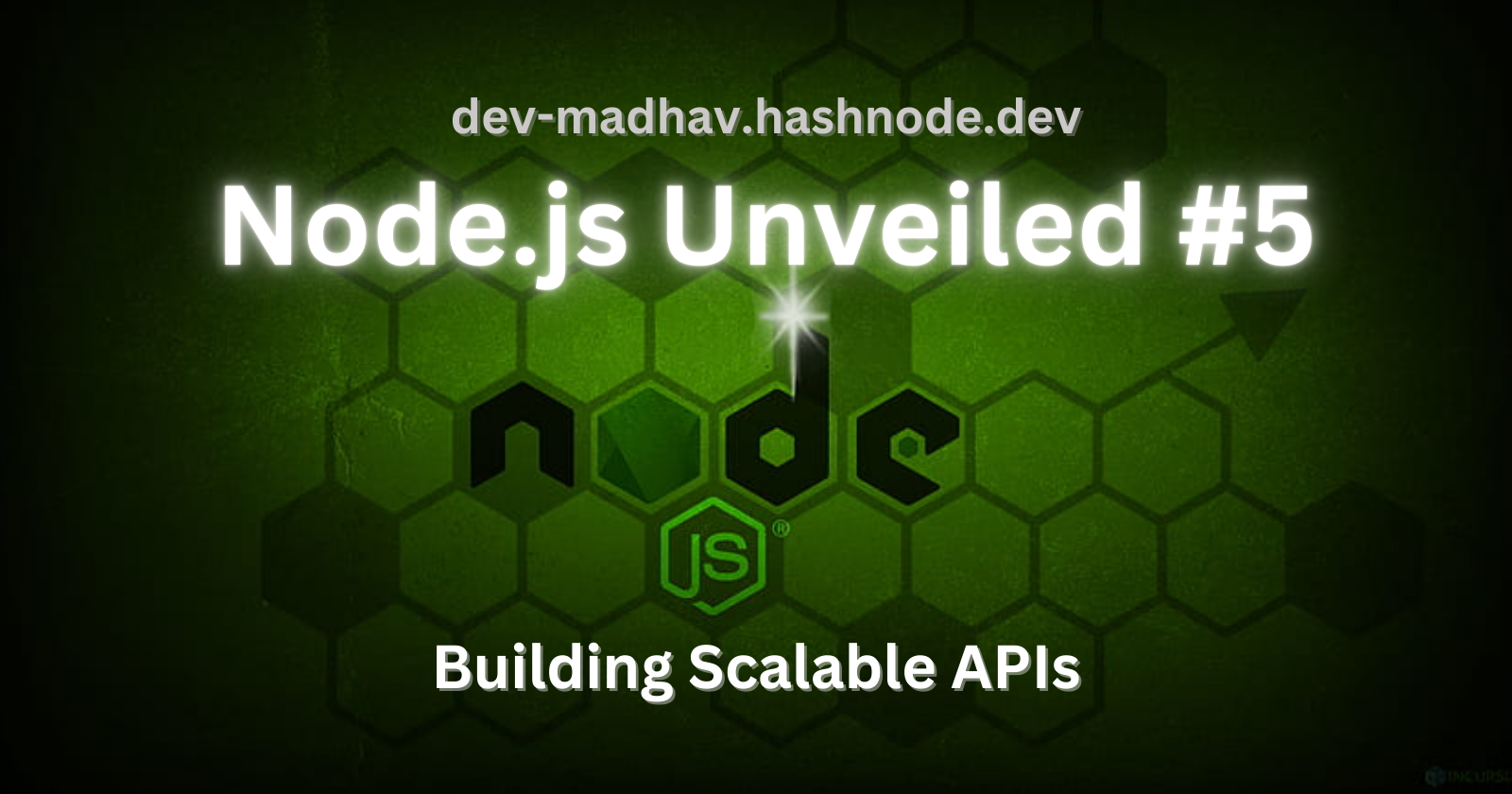
One of the key components of Node.js powered applications is well-designed APIs. RESTful APIs, provide a structured approach to building web services that are easy to understand, maintain, and scale.
Key points of RESTful API design:
Resource-based: APIs should be centered around resources, such as users, products, or orders.
Cacheable: Responses can be cached to improve performance.
Layered: APIs should be layered to allow for flexibility and scalability.
Building RESTful APIs with Node.js:
A framework: Node.js offers several popular frameworks like Express.js. These provide a solid foundation for building RESTful APIs, handling routing, middleware, and error handling.
Define your resources: Identify the resources that your API will expose and the operations that can be performed on them (e.g., GET, POST, PUT, DELETE).
Design your routes: Create routes that map HTTP methods to specific actions on your resources. For example, a GET request to
/users/123
might retrieve the details of a specific user.Handle requests and responses: Use the framework's built-in mechanisms to handle incoming requests, process them, and send appropriate responses. This involves parsing request bodies, validating data, and formatting responses.
Consider security: Protect your API from security vulnerabilities by implementing measures like authentication, authorization, and input validation.
Example Express.js API
const express = require('express');
const app = express();
app.get('/users/:id', (req, res) => {
// Retrieve user data from a database or other data source
const user = { id: req.params.id, name: 'John Doe' };
res.json(user);
});
app.listen(3000, () => {
console.log('Server listening on port 3000');
});
*example code sourced form internet.
Introduction to GraphQL for Enterprise Data Management
While RESTful APIs are a popular choice, they can become complex and inefficient for handling complex datasets.
GraphQL, a query language for APIs, offers a more flexible and efficient approach. GraphQL allows clients to specify exactly what data they need, reducing over-fetching and under-fetching issues.
Key features of GraphQL:
Strongly typed: GraphQL uses a schema to define the structure of your data, making it easier to understand and use.
Declarative: Clients can specify the exact data they need, avoiding unnecessary data transfer.
Efficient: GraphQL can optimize queries to minimize network traffic.
Flexible: GraphQL allows for custom fields and types, making it adaptable to various use cases.
Example GraphQL API with Apollo Server:
const { ApolloServer, gql } = require('apollo-server-express');
const typeDefs = gql`
type User {
id: ID!
name: String!
}
type Query {
users: [User!]!
}
`;
const resolvers = {
Query: {
users: () => {
// Retrieve user data from a database or other data source
return [
{ id: '1', name: 'John Doe' },
{ id: '2', name: 'Jane Smith' }
];
}
}
};
const server = new ApolloServer({ typeDefs, resolvers });
server.listen().then(({ url }) => {
console.log(`๐ Server ready at ${url}`);
});
*example code sourced form internet.
By carefully considering these factors and following best practices, the healthcare organization can build a scalable, secure, and efficient API to meet their data management needs.
Subscribe to my newsletter
Read articles from Madhav Dhobley directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
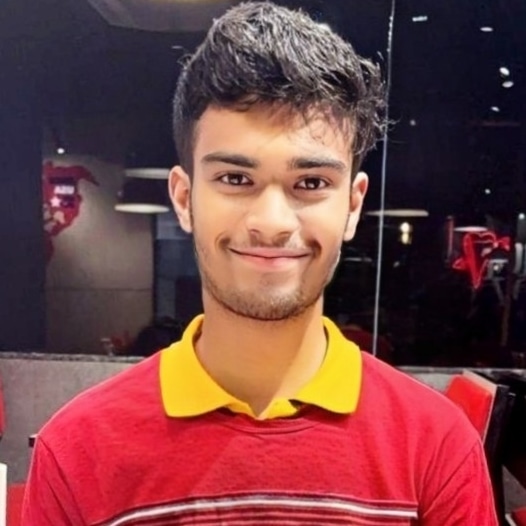
Madhav Dhobley
Madhav Dhobley
I am a dedicated React and Node.js developer with a good track record of successfully delivering guided projects in these cutting-edge technologies. My passion for creating dynamic and efficient web applications is evident in my hands-on experience with database management using MySQL and PostgreSQL, ensuring seamless integration and optimal performance.