Understanding React's useState and useEffect hooks
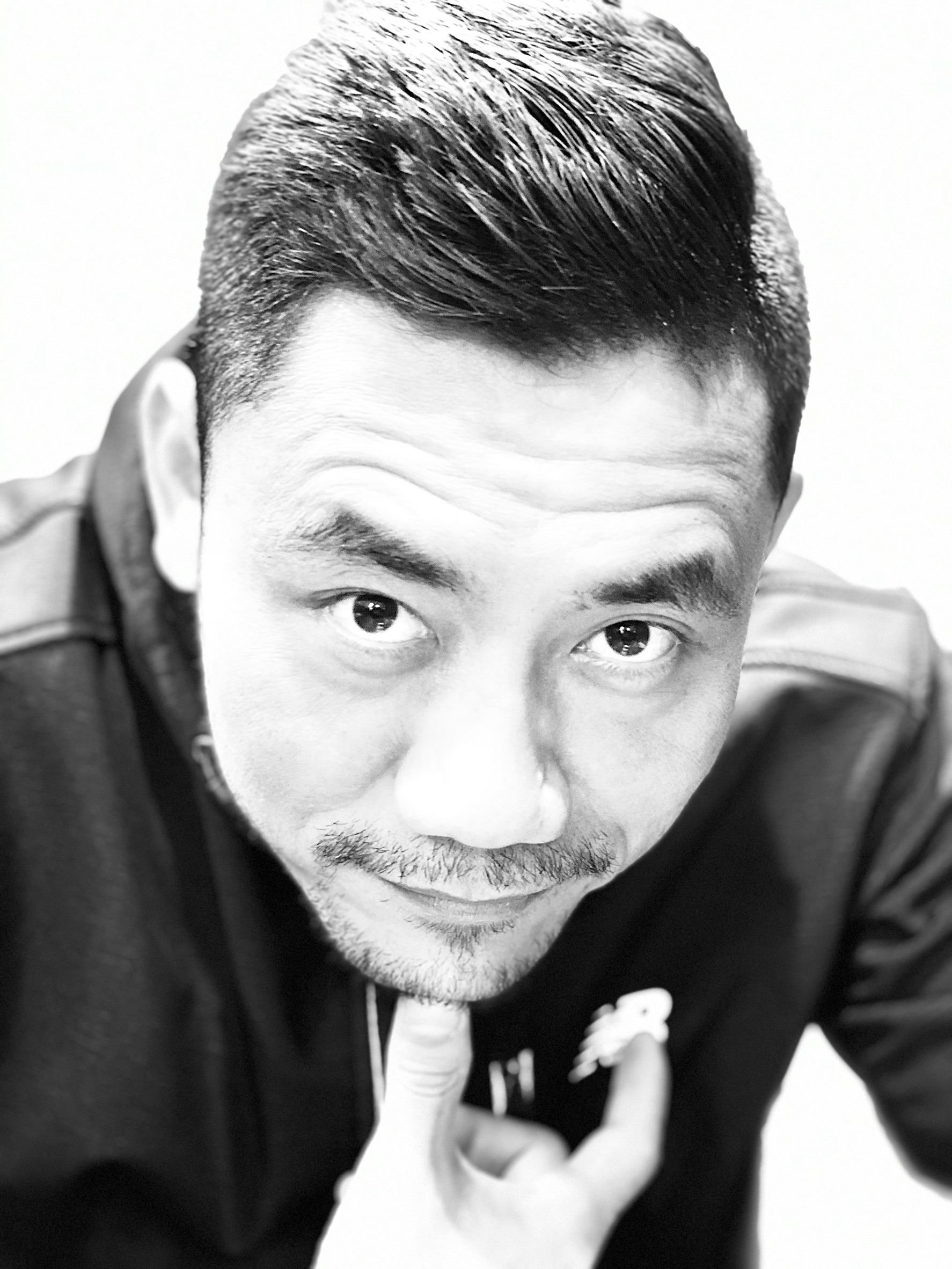
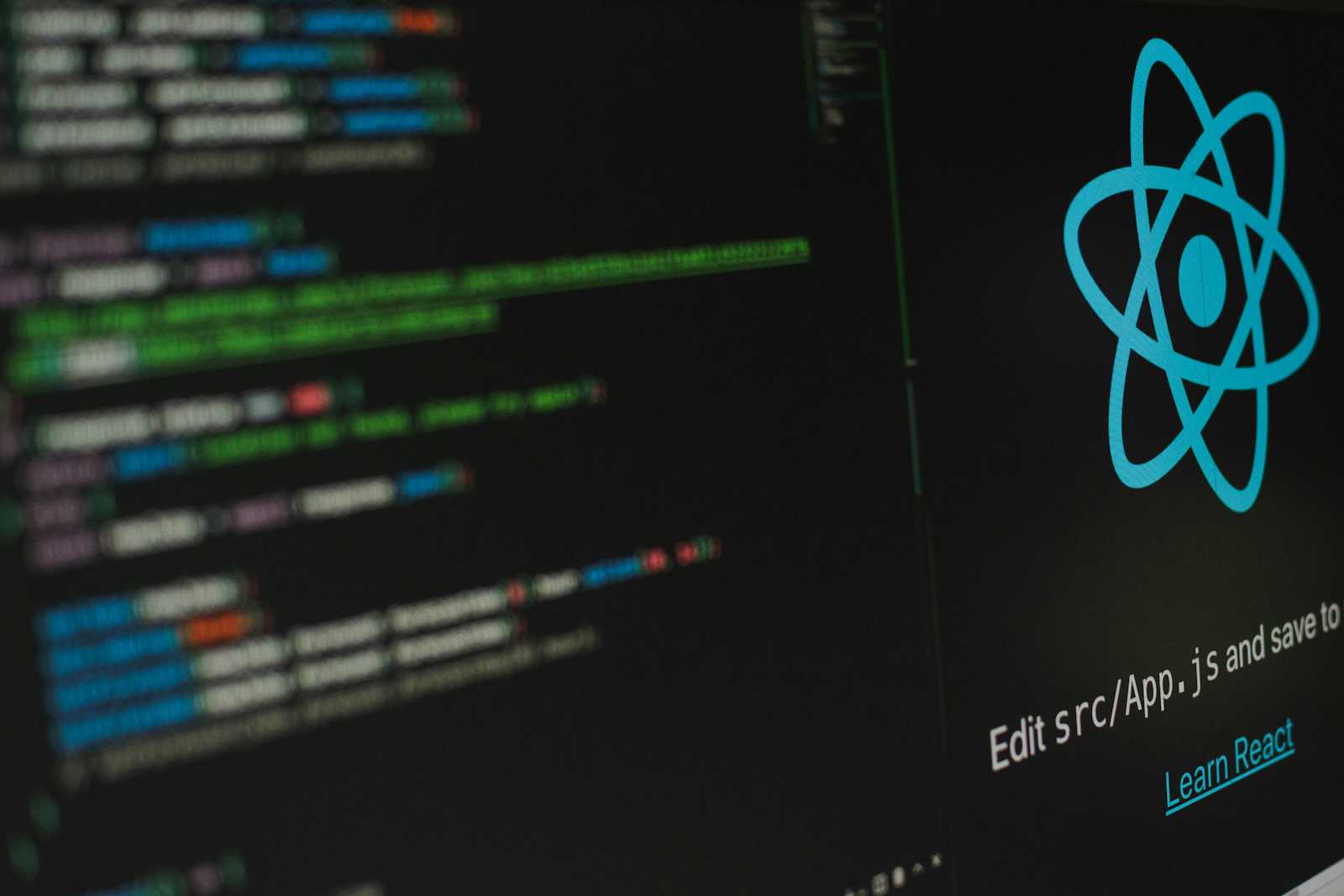
React is one of the most popular JavaScript libraries for building user interfaces.managing state and side effects in functional components became much easier and more intuitive. In this blog, we'll dive into two essential hooks: useState and useEffect, exploring their usage with code snippets and practical examples.
What are React Hooks?
Hooks are functions that let you "hook into" React state and lifecycle features from function components. They allow you to use state and other React features without writing a class. Two of the most commonly used hooks are useState and useEffect.
1. The useState Hook
The useState hook is used to manage state in a functional component. It allows you to add state variables to your component.
Basic Syntax
- state: The current state value.
- setState: A function that lets you update the state.
- initialState: The initial value of the state.
Example: Counter Component
Let's build a simple counter component to demonstrate useState in action.
In this example:
- We initialize the count state variable with 0.
- setCount is used to update the count state.
- Clicking the "Increment" button increases the count, and clicking "Decrement" decreases it.
2. The useEffect Hook
The useEffect hook allows you to perform side effects in your components. Side effects can include data fetching, subscriptions, or manually changing the DOM.
Basic Syntax
- Effect function: A function that contains the side-effect logic.
- Cleanup function (optional): A function that cleans up the side effect when the component unmount or before the effect is re-run.
- Dependencies: An array of values that the effect depends on. The effect will only re-run if one of these values changes.
Example: Fetching Data from an API
Let's create a component that fetches data from an API using useEffect.
In this example:
- The useEffect hook is used to fetch data from an API when the component mounts.
- The empty dependency array ([]) ensures that the effect runs only once after the initial render.
- The data state is updated with the fetched data, and loading is set to false once the data is loaded.
Combining useState and useEffect
Let's combine useState and useEffect to create a more interactive component. We'll create a simple search filter that fetches and displays data based on a query.
Example: Searchable Data List
In this example:
- The search state stores the search input value.
- The useEffect hook runs whenever the search changes, fetching data based on the search.
- If search is empty, no request is made.
- The component displays the filtered data or a loading message while the data is being fetched.
Conclusion
React's useState and useEffect hooks provide powerful tools for managing state and side effects in functional components. With useState, you can easily add and manage state, and with useEffect, you can handle side effects such as data fetching, subscriptions, and more.
By combining these hooks, you can create dynamic, interactive components that respond to user input and external data sources. Whether you're building simple components or complex applications, mastering these hooks is essential for any React developer.
Happy coding!
Subscribe to my newsletter
Read articles from Buddha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
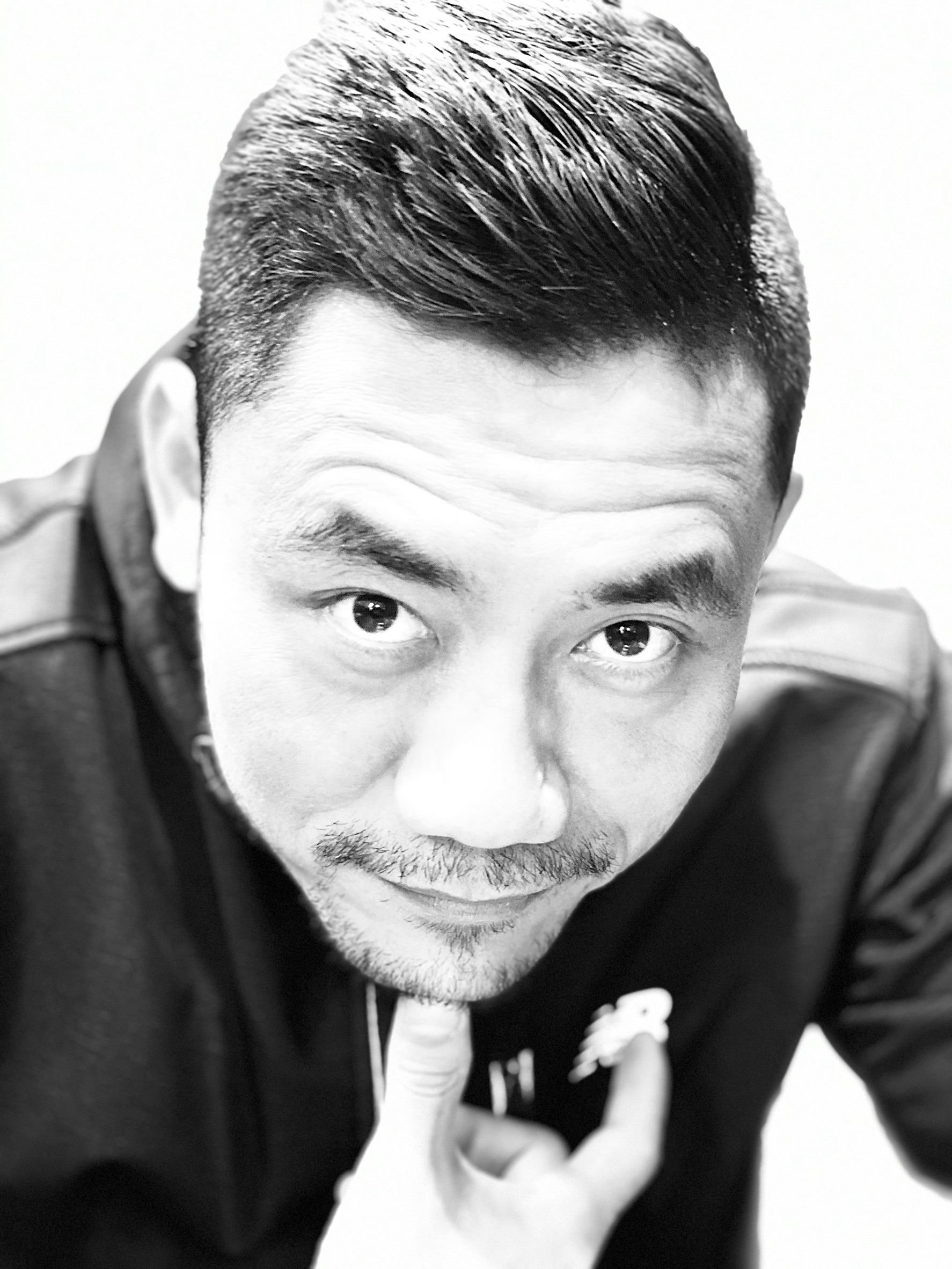