Mastering Git & GitHub: A DevOps Essential 🚀 #Day9
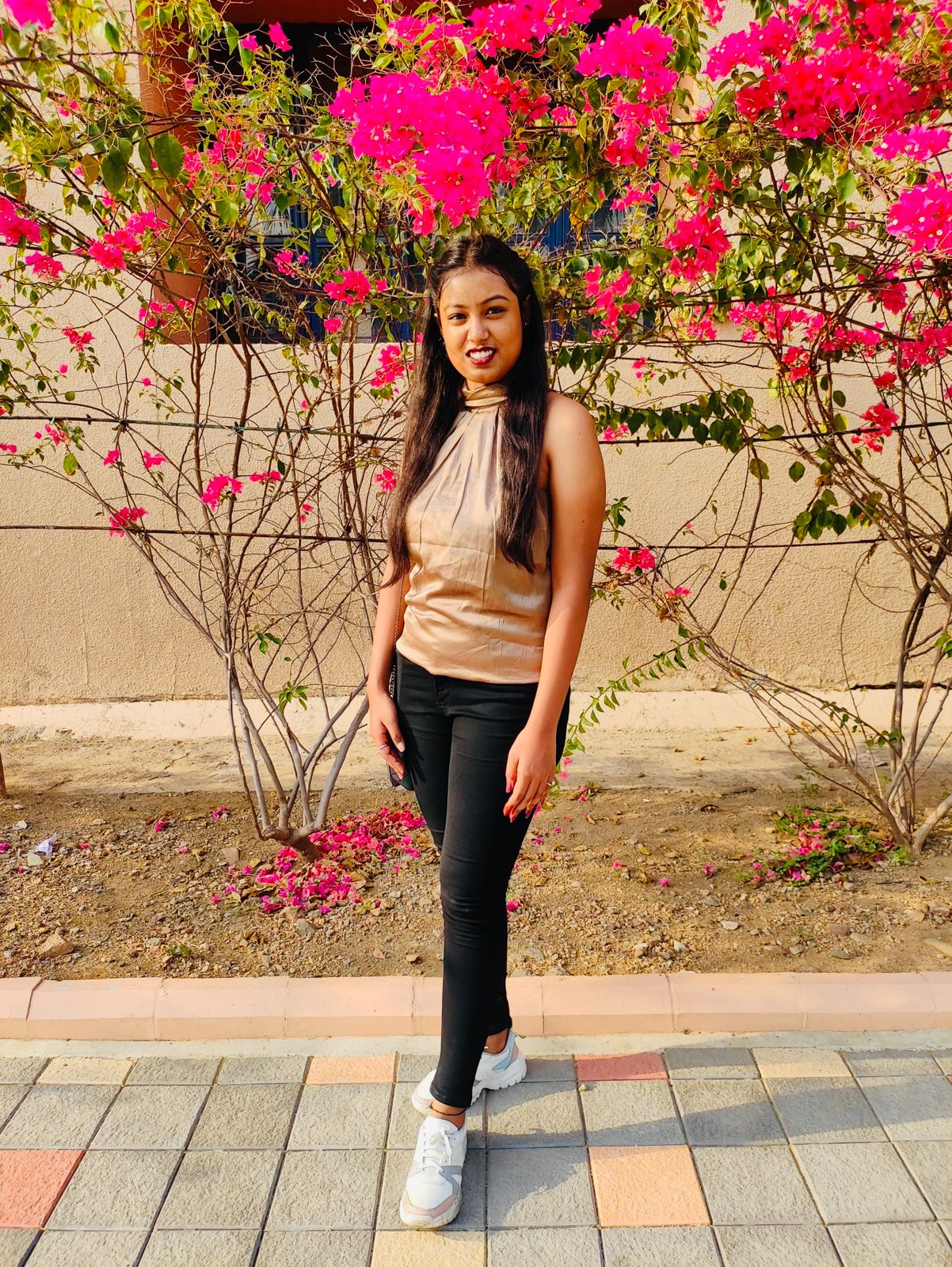
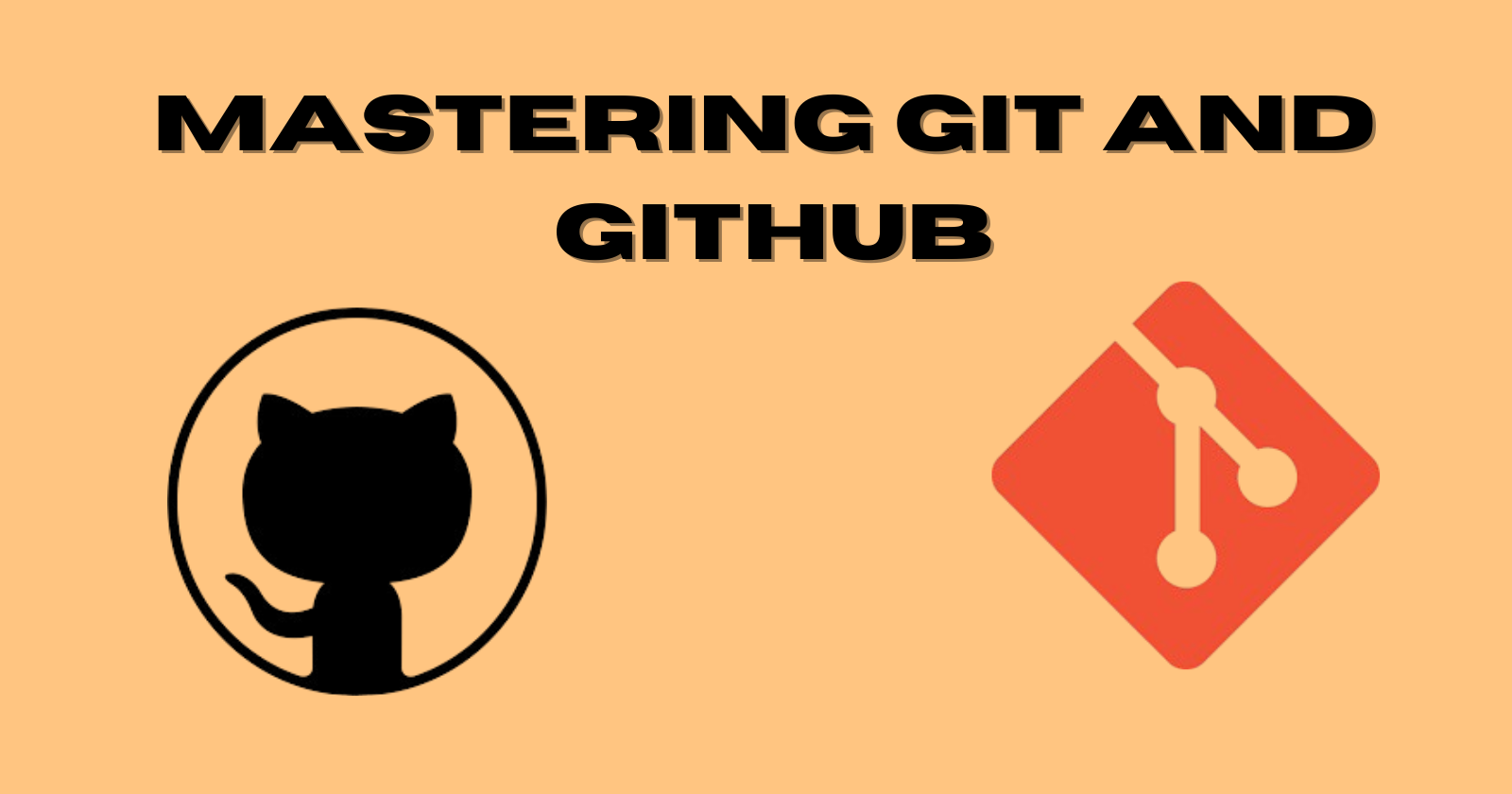
Understanding Version Control Systems (VCS)
A Version Control System (VCS) is a tool that helps track changes in files and coordinate work among multiple people. It allows you to save different versions of your project, track who made changes, and roll back to an earlier version if needed. VCS is essential in software development for managing code, documentation, and even configuration files.
Types of Version Control Systems
Centralized Version Control Systems (CVCS)
In a centralized VCS, there is a single server that contains all the project files and history. Developers check out files from the server to work on them, and then commit changes back to the server. The server is the single source of truth.
Example: Subversion (SVN), Perforce, CVS
Distributed Version Control Systems (DVCS)
In a distributed VCS, every developer has a complete copy of the repository, including all history. Changes can be committed locally, and developers can work offline. When ready, changes can be pushed to a remote server or shared with others.
Example: Git, Mercurial, Bazaar
Comparison: Centralized vs. Distributed VCS
Feature | Centralized VCS (CVCS) | Distributed VCS (DVCS) |
Repository Structure | Single central repository | Full repository on every developer's machine |
Collaboration | Requires constant connectivity | Allows offline work |
Backup and Redundancy | Single point of failure | Full backup on every machine |
Speed | Slower, especially for large repositories | Faster, local operations are quick |
1. Introduction to Git and GitHub
What is Git?
Git is a version control system that tracks changes in your code over time. It records what was changed, who changed it, and when it was changed.
Why is Git crucial for DevOps engineers?
In DevOps, Git helps teams collaborate smoothly. Multiple people can work on the same project without interfering with each other's code. If something breaks, you can roll back to a previous stable version.
💡 Real-Time Example: Imagine you and your friends are writing a story together. Each person works on different chapters, and Git helps everyone keep their chapters organized and lets you combine them smoothly into one complete story.
What is GitHub?
GitHub is a cloud-based platform that hosts Git repositories. It allows developers to manage their code, collaborate with others, and track changes using Git’s version control capabilities, but with additional features that make it easier to work on projects together.
Key Features of GitHub:
Repository Hosting: Stores your Git repositories in the cloud, accessible from anywhere.
Collaboration: Multiple developers can work on the same project, fork repositories, and merge changes via pull requests.
Pull Requests & Code Reviews: Review and discuss changes before merging them into the main project.
Issue Tracking: Track bugs and tasks, assign issues, and monitor progress.
Project Management: Organize tasks with Kanban-style project boards.
Community & Networking: Showcase projects, contribute to open-source, and connect with others.
Automation: Automate tasks like testing and deployment with GitHub Actions.
Documentation: Create and host project documentation, such as README files.
Example: For a team project, everyone pushes code to GitHub, makes updates, and reviews changes through pull requests.
Key Differences Between Git and GitHub
Feature | Git | GitHub |
Purpose | Version control system | Repository hosting platform |
Where it Runs | Locally on your machine | Cloud-based |
Features | Tracks code changes, branching, merging | Pull requests, issue tracking, collaboration tools |
Usage | Command-line or GUI tools | Web-based interface, integrated with Git |
Who Can Use It? | Anyone who installs it | Users with GitHub accounts |
2. Setting Up Git: git config
and git init
Before diving into Git commands, you need to configure your identity and initialize a repository.
Configure Git with Your Details:
The git config
command sets your username and email address, which Git uses to track who made changes.
Set your username:
git config --global user.name "Your Name"
Set your email address:
git config --global user.email "you@example.com"
💡 Tip: The --global
flag ensures these settings apply to all your projects. You can also set them at the project level by omitting the --global
flag.
Initialize a Git Repository:
The git init
command sets up a new Git repository in your project directory.
Initialize a new repository:
git init
This creates a hidden
.git
folder that stores all version control information.
📂 Example: Imagine you start a new project for a website. Running git init
in that folder starts tracking changes, allowing you to save versions as you progress.
3. Key Concepts in Git
Repository (Repo): A project folder where all your files are stored.
📂 Example: A folder on your computer where your team stores the website files.
1.git add
This command stages your changes, preparing them to be committed. You can stage specific files or all changes at once.
# Stage a specific file
git add <file-name>
# Stage all changes
git add .
Example: If you updated a file named index.html
, you would run git add index.html
to track it.
2.git commit
This command saves the staged changes with a message describing what you’ve done. Commits are snapshots of your project.
# Commit with a message
git commit -m "Describe what changes were made"
Example: After making updates, you’d run git commit -m "Added new login feature"
.
3. git pull
This command fetches and integrates changes from the remote repository into your local branch. It ensures your code is up-to-date.
Pull Request (PR): A PR is when you ask your teammates to review your changes before merging them into the main project.
# Pull the latest changes from a branch
git pull origin <branch-name>
Example: If your team pushed updates to the main
branch, you’d run git pull origin main
to sync with their changes.
💻 Real-Time Example: Imagine you’re working on a website’s login page. You create a branch called login-feature
to work on it separately. Once finished, you test it and merge it back into the main branch without affecting the rest of the website.
4. git push
This command uploads your local commits to the remote repository, making your changes available to others.
# Push your changes to a branch
git push origin <branch-name>
Example: Once your code is ready, you’d run git push origin feature-branch
to share your work.
5. git log
This command shows the commit history, helping you track what’s been done and by whom. You can view detailed or simplified logs.
# Show the commit history
git log
# Show a simplified, one-line log for each commit
git log --oneline
Example: Use git log --oneline
to quickly review the commit history and see what changes were made.
4. Branching and Merging in Git 🌿
Branching is one of Git’s most powerful features. It allows you to work on a new feature, fix a bug, or experiment with code without affecting the main project.
Key Branching Commands:
git branch <branch-name>
: Create a new branch.git checkout <branch-name>
: Switch to a branch.git checkout -b <branch-name>
: Create a new branch and switch to it immediately.git merge <branch-name>
: Merge another branch into the current branch.git branch -d <branch-name>
: Delete a branch once it’s merged.Visualize Branching: Think of your main project as the trunk of a tree 🌳. Branches grow from the trunk whenever you want to work on something new. Once the work is done, branches merge back into the trunk, like new features being added to the main project.
Detailed Example:
Create a new branch:
git checkout -b feature-login
Switch back to the main branch:
git checkout main
Merge the feature branch into the main branch:
git merge feature-login
Delete the feature branch (optional):
git branch -d feature-login
5. Handling Merge Conflicts 🤔
Merge conflicts occur when two branches have conflicting changes. Git will flag these conflicts so you can decide which changes to keep.
Steps to Resolve Merge Conflicts:
Identify the conflict: Git will mark the conflicting code.
Manually edit the file: Choose which changes to keep or combine them.
Stage the resolved file:
git add <file>
Commit the resolution:
git commit -m "Resolved merge conflict"
💡 Real-Time Example: Imagine two friends are editing the same document. One changes the title while the other edits the content. When combining their changes, Git will flag this conflict so you can decide which title or content to keep.
6. Remote Repositories and Collaboration 🌐
Git works smoothly with platforms like GitHub, GitLab, and Bitbucket for remote collaboration.
Common Remote Repository Commands:
git fork <repo-url>
: Make a personal copy of someone else’s project.git clone <repo-url>
: Download a project from a remote repository to your computer.git push <remote> <branch>
: Upload your changes to the remote repository.git pull <remote> <branch>
: Fetch and merge changes from the remote repository.git fetch <remote>
: Get updates from the remote without merging them.git pull-request
(PR): Request to merge your changes into the main project.🛠️ Real-Time Example: If you find a cool open-source project on GitHub, you can fork it, make improvements, and then submit a pull request to share your work with the original creators.
7. Conclusion
Git is a must-have tool for any DevOps engineer. It simplifies teamwork, keeps your work organized, and helps automate deployments. Start practicing with small projects and get comfortable with Git by contributing to real-world repositories.
Subscribe to my newsletter
Read articles from Vaishnavi Modakwar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
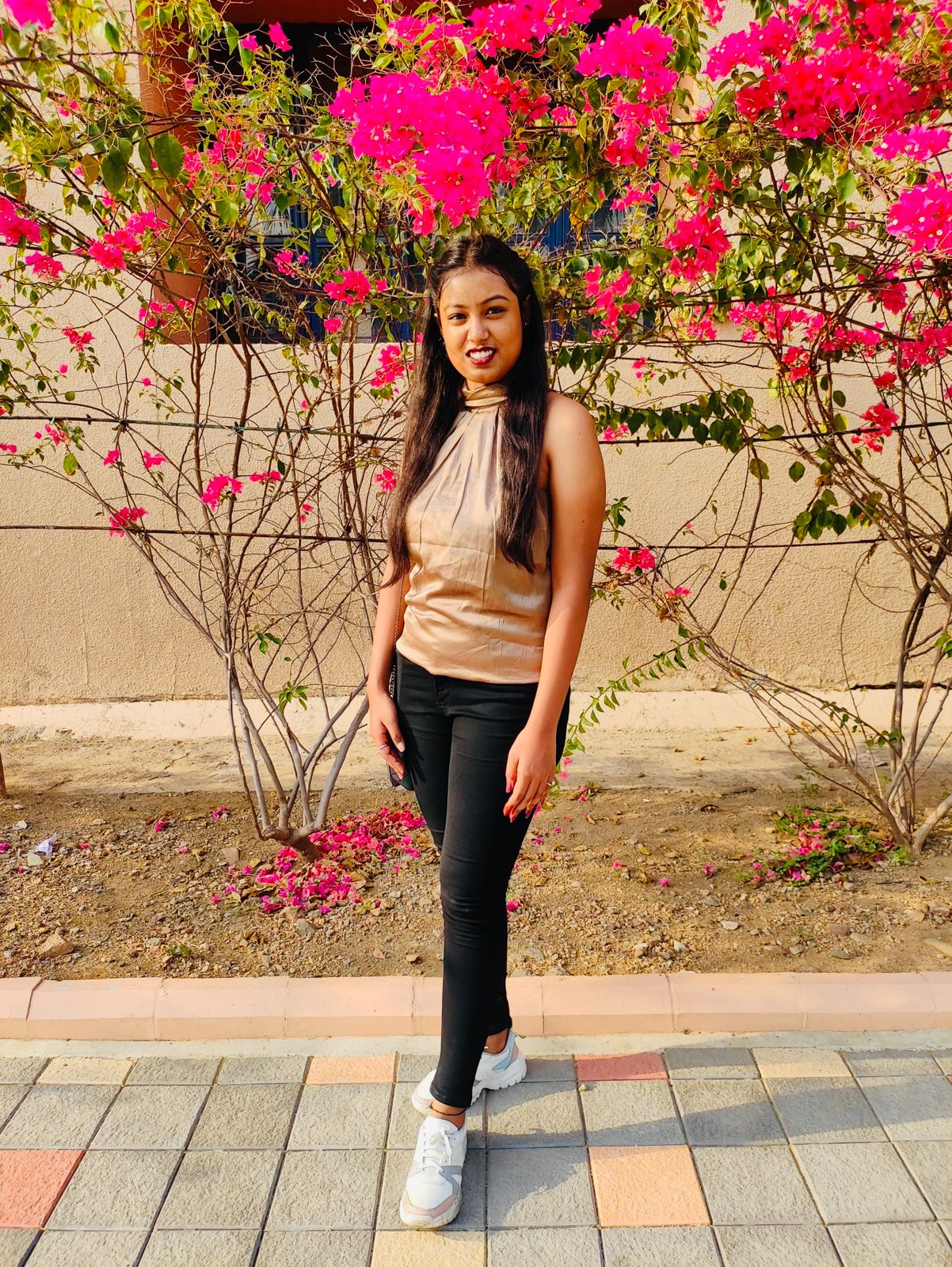
Vaishnavi Modakwar
Vaishnavi Modakwar
👋 Hi there! I'm Vaishnavi Modakwar, a dedicated DevOps and Cloud Engineer with 2 years of hands-on experience in the tech industry. My journey in DevOps has been fueled by a passion for optimizing and automating processes to deliver high-quality software efficiently. Skills: Cloud Technologies: AWS, Azure. Languages: Python, YAML, Bash Scripting. Containerization: Docker, ECS, Kubernetes. IAC: Terraform, Cloud Formation. Operating System: Linux and MS Windows. Tools: Jenkins, Selenium, Git, GitHub, Maven, Ansible. Monitoring: Prometheus, Grafana. I am passionate about demystifying complex DevOps concepts and providing practical tips on automation and infrastructure management. I believe in continuous learning and enjoy keeping up with the latest trends and technologies in the DevOps space. 📝 On my blog, you'll find tutorials, insights, and stories from my tech adventures. Whether you're looking to learn about CI/CD pipelines, cloud infrastructure, or containerization, my goal is to share knowledge and inspire others in the DevOps community. Let's Connect: I'm always eager to connect with like-minded professionals and enthusiasts. Feel free to reach out for discussions, collaborations, or feedback. Wave me at vaishnavimodakwar@gmail.com