How to Password-Protect a PDF Document Using Python
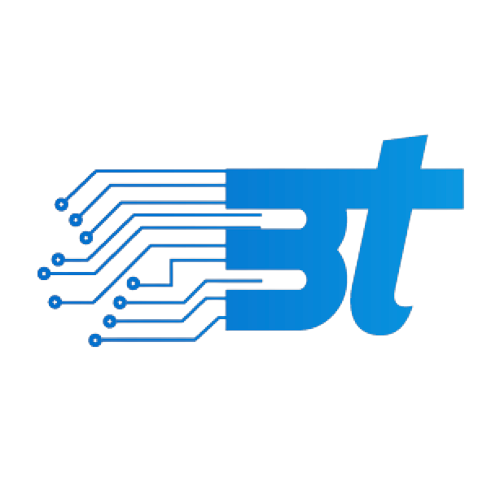
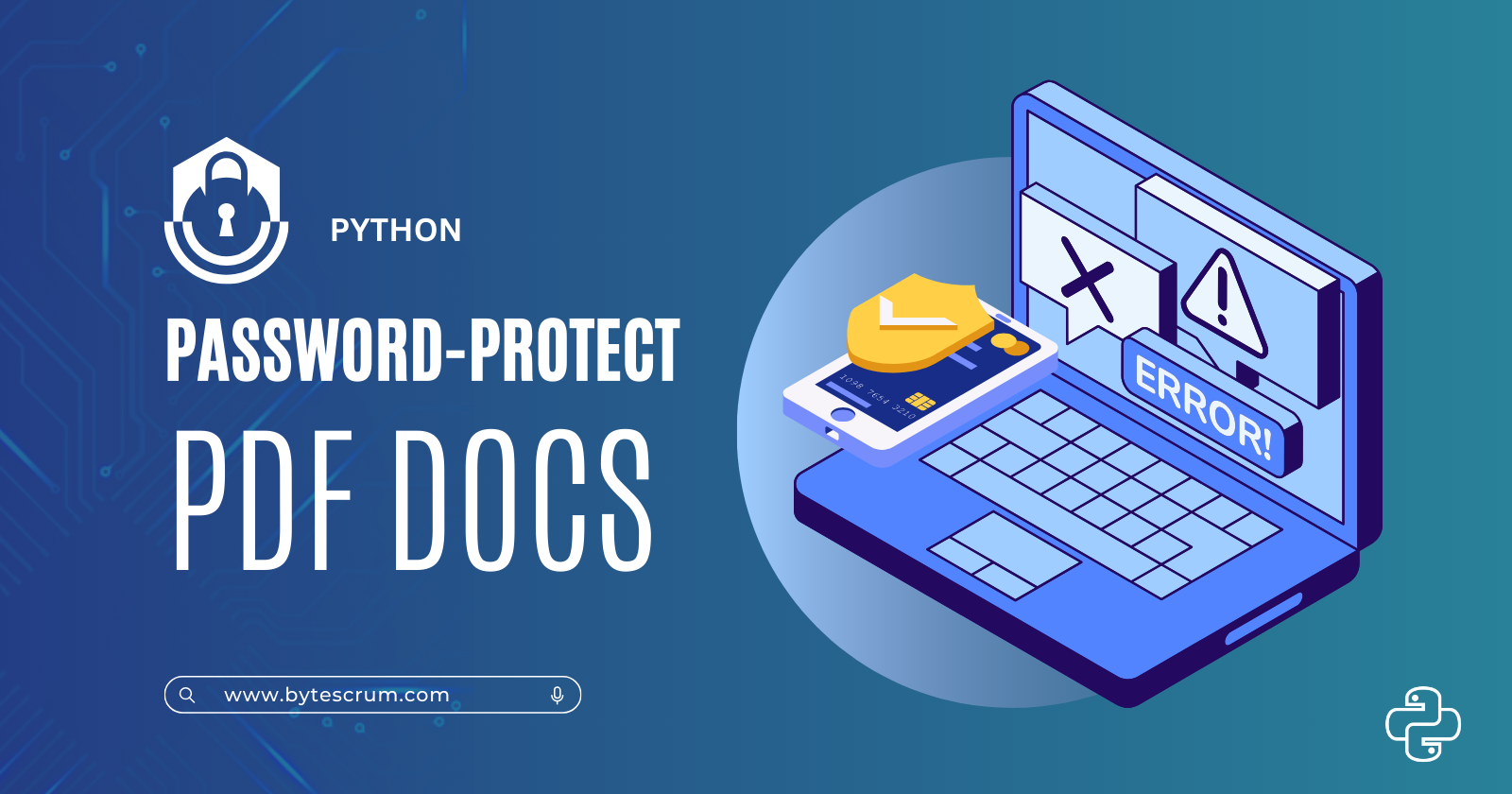
Password-protecting PDF documents is an essential practice for safeguarding sensitive information. By encrypting a PDF with a password, you can control who can open, view, and modify the document. Python offers several libraries that make it easy to add password protection to PDF files. In this guide, we’ll show you how to use PyPDF2
to encrypt a PDF with a password.
Why Password-Protect PDF Documents?
Confidentiality: Ensure only authorized users can access sensitive information.
Integrity: Prevent unauthorized modifications to the document.
Compliance: Meet regulatory requirements for data protection.
Getting Started
To password-protect a PDF, you’ll need to install the PyPDF2
library, which provides tools for reading, merging, and encrypting PDF files.
Step 1: Install PyPDF2
Install the PyPDF2
library using pip:
pip install PyPDF2
Step 2: Encrypt a PDF with a Password
Here’s a simple script to add password protection to a PDF file:
from PyPDF2 import PdfReader, PdfWriter
def password_protect_pdf(input_pdf, output_pdf, password):
# Create a PdfReader object to read the input PDF
reader = PdfReader(input_pdf)
# Create a PdfWriter object to write the output PDF
writer = PdfWriter()
# Add all pages from the input PDF to the writer
for page_num in range(len(reader.pages)):
writer.add_page(reader.pages[page_num])
# Encrypt the PDF with the given password
writer.encrypt(password)
# Write the encrypted PDF to a new file
with open(output_pdf, "wb") as output_file:
writer.write(output_file)
print(f"Password-protected PDF saved as: {output_pdf}")
if __name__ == "__main__":
input_pdf = "example.pdf" # Path to the input PDF file
output_pdf = "protected_example.pdf" # Path to the output PDF file
password = "mypassword" # Password to protect the PDF
password_protect_pdf(input_pdf, output_pdf, password)
How This Script Works
Importing Libraries: The script uses
PdfReader
to read the input PDF andPdfWriter
to create a new PDF with encryption.Reading the Input PDF: The
PdfReader
object loads the input PDF file.Creating an Encrypted PDF:
The script iterates through all the pages of the input PDF using
reader.pages
.It adds each page to the
writer
object.writer.encrypt(password)
applies the password protection to the PDF.
Saving the Encrypted PDF: The encrypted PDF is saved to a new file with
writer.write(output_file)
.
Step 3: Run the Script
Replace the input_pdf
, output_pdf
, and password
variables with your desired values and run the script. The script will generate a password-protected PDF file with the specified password.
Additional Tips
Choosing a Strong Password: Use a combination of letters, numbers, and special characters to create a strong password.
Permissions: You can customize the permissions for the encrypted PDF, such as allowing printing or copying, by modifying the
encrypt()
method's arguments. For example,writer.encrypt(password, user_pwd=None, owner_pwd=None, use_128bit=True, permissions=None)
allows you to specify different user and owner passwords and set permissions.Batch Processing: You can modify the script to batch process multiple PDF files by iterating through a directory of PDF documents.
Conclusion
PyPDF2
library. By following the steps in this guide, you can ensure that your sensitive documents are secure and accessible only to those with the appropriate password. This is particularly useful for businesses and individuals who need to share confidential information securely.Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
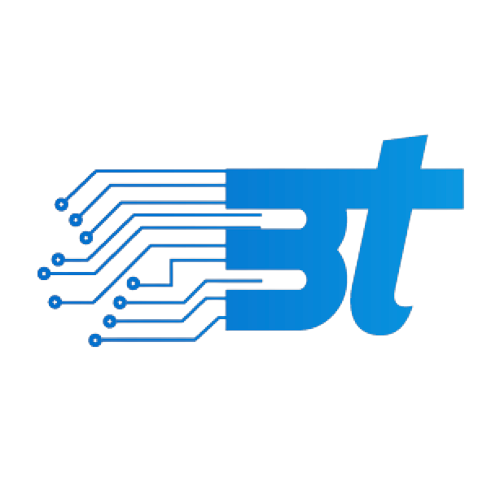
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.